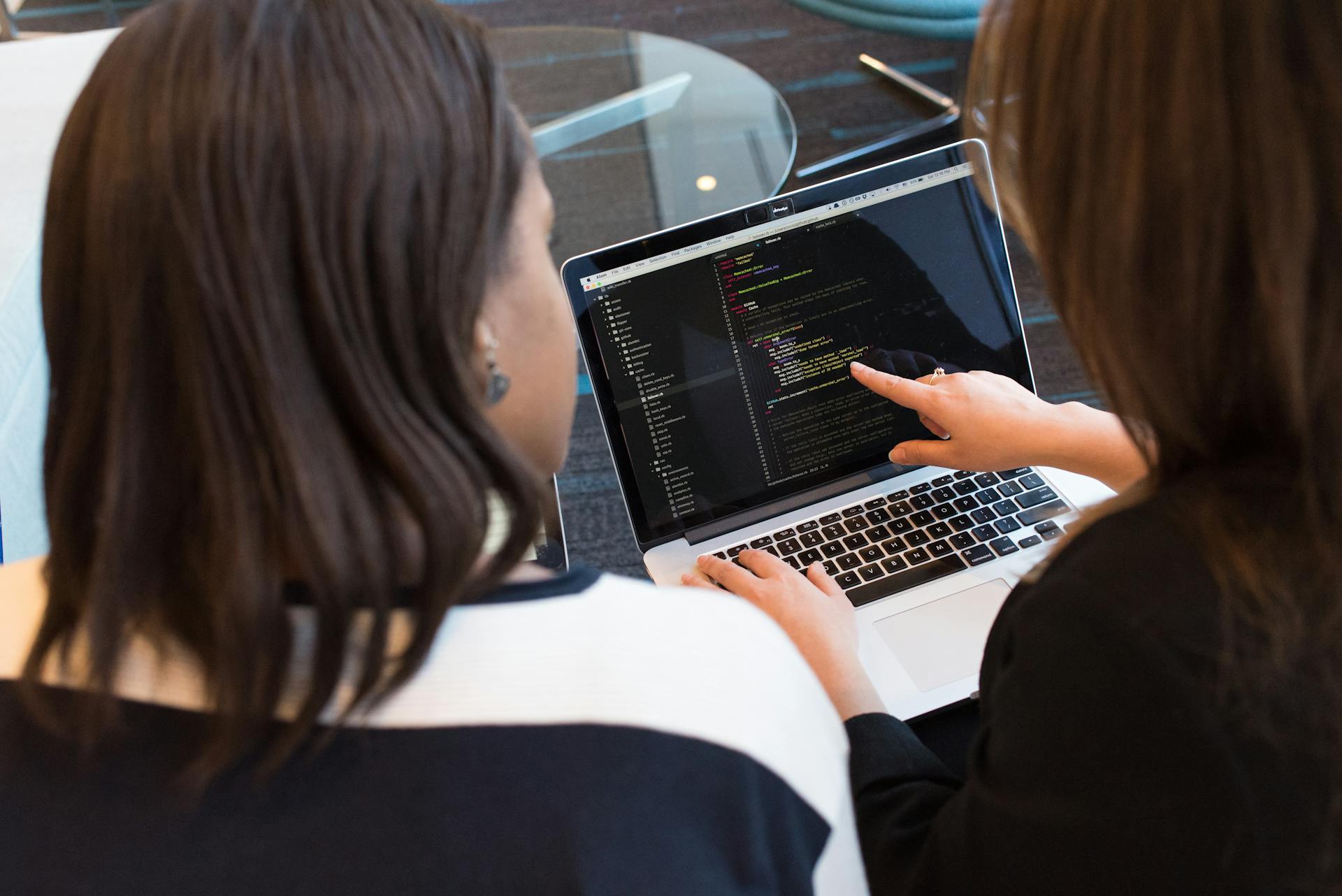
Packaging a Django project can be a daunting task, but it's a crucial step in making your project reusable and shareable with others. First, you need to create a setup.py file in the root directory of your project.
This file serves as the entry point for your project's packaging information and dependencies. In the setup.py file, you'll specify the project's name, version, and author, as well as any dependencies required to run the project.
To install the project, you'll use pip, a package installer for Python, which can be easily installed via the Python Package Manager.
Project Setup
To set up a Django project, start by creating a project folder, such as hello_django, on your file system.
You'll then create a virtual environment within that folder, which will isolate your project's dependencies and make it easy to manage them. To do this, use the command `python3 -m venv .venv` (or `py -3 -m venv .venv` on Windows) to create a virtual environment named `.venv`.
Next, open the project folder in VS Code by running `code .` or by using the `File > Open Folder` command. Then, select the `Python: Select Interpreter` command from the Command Palette to choose the virtual environment in your project folder.
To activate the virtual environment, run `Terminal: Create New Terminal` from the Command Palette, which will automatically activate the virtual environment by running its activation script.
Here's a summary of the steps to create a project environment:
- Create a project folder, such as hello_django, on your file system.
- Use the command `python3 -m venv .venv` (or `py -3 -m venv .venv` on Windows) to create a virtual environment named `.venv`.
- Open the project folder in VS Code and select the `Python: Select Interpreter` command from the Command Palette.
- Activate the virtual environment by running `Terminal: Create New Terminal` from the Command Palette.
This will set up a self-contained environment ready for writing Django code.
Define the Requirements
Defining the requirements for your project is a crucial step in setting it up. You'll need to create a requirements.txt file that lists all the packages your project depends on.
This file should include packages like Django, psycopg2, and sqlalchemy, which are commonly used in web development. You can also use pip freeze to generate the file based on the exact libraries installed in your environment.
To create a requirements.txt file using pip freeze, activate your environment and run pip freeze > requirements.txt in the terminal. This will create the file in your project folder.
Anyone who receives a copy of your project can then reinstall the packages by running pip install -r requirements.txt. This ensures that everyone has the same environment and packages installed.
A Setup.cfg File
A setup.cfg file is an essential part of packaging a Django app. It contains configuration information used by the setup.py script to build the package.
This file defines the package requirements, such as the version of Python and Django that the package requires. To create a setup.cfg file, you need to open a text editor and add specific lines of code.
The setup.cfg file contains information about the package, including the name, version, description, author, license, and classifiers. It's a crucial step in the project setup process.
Once you've created the setup.cfg file, you can build the package using the setup.py script. Make sure to include the necessary package requirements to ensure smooth functionality.
Minimal App
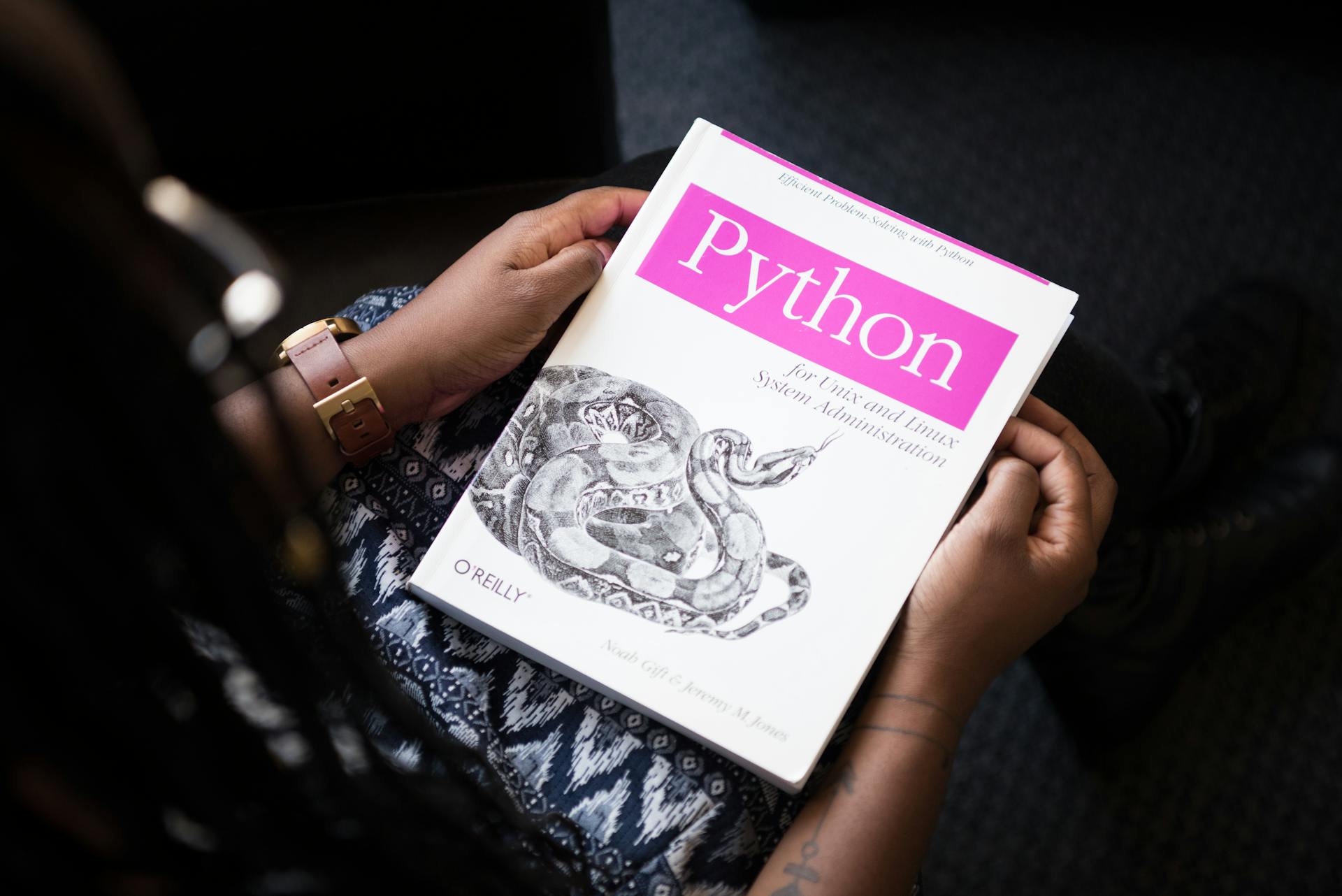
To create a minimal Django app, you'll need to create a Django project first. This project will serve as the container for your app. You can use the Django administrative utility, django-admin, which is installed when you install the Django package.
A Django project can contain multiple apps, each with its own independent function. To create a Django project, you'll use the django-admin utility. This utility is installed when you install the Django package.
You'll need to create a requirements.txt file to define the package requirements for your Django app. This file should include all the necessary packages, such as django, psycopg2, or sqlalchemy. You can use the pip install -r requirements.txt command to install all the packages.
A Django app is just a Python package that follows certain conventions. To create a minimal Django app, you'll need to create the app itself after creating the Django project. You can use the django-admin utility for this purpose as well.
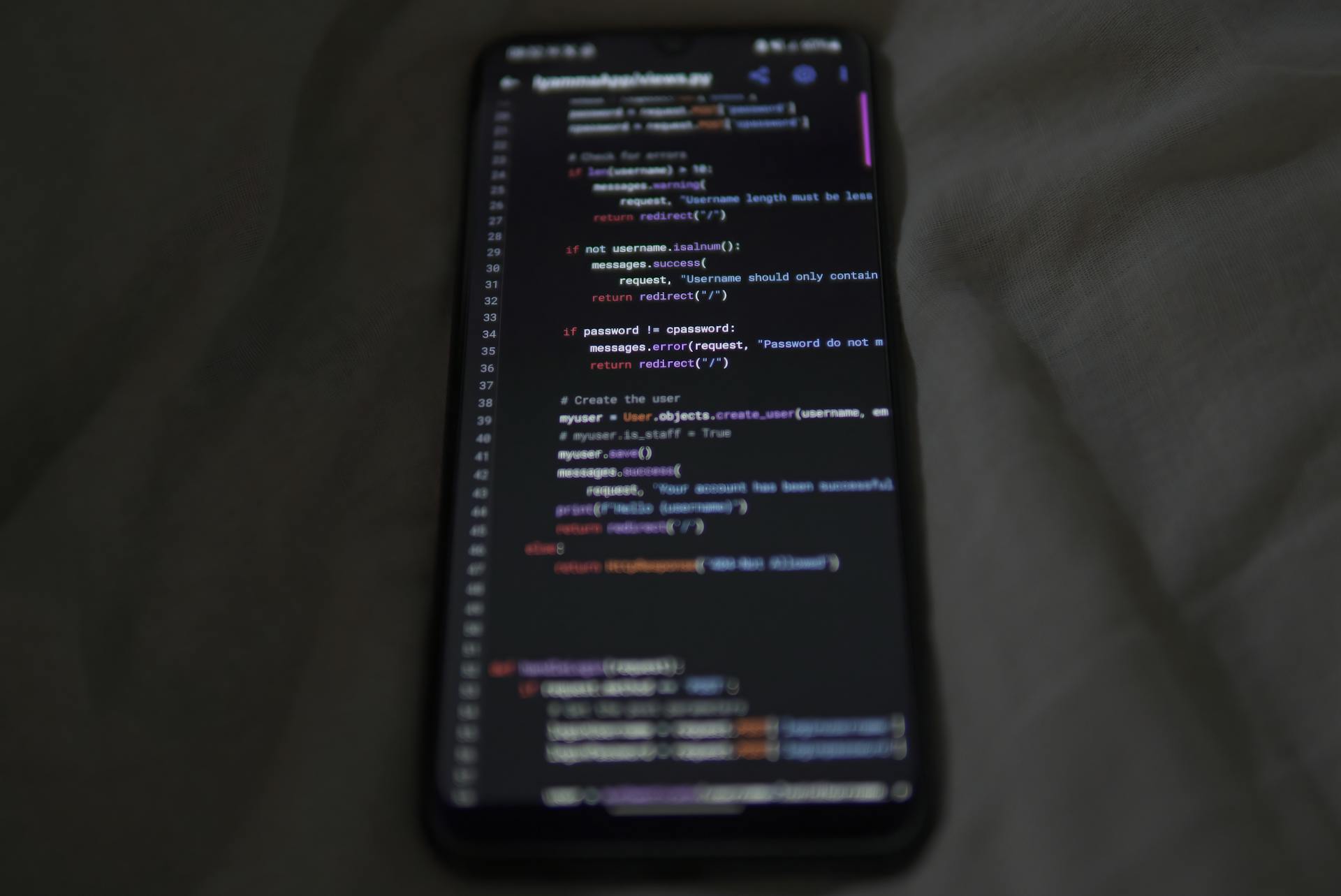
Here are the basic steps to create a minimal Django app:
- Create a Django project using the django-admin utility.
- Create a requirements.txt file to define the package requirements.
- Include the necessary packages, such as django, psycopg2, or sqlalchemy, in the requirements.txt file.
- Use the pip install -r requirements.txt command to install all the packages.
- Create the Django app itself using the django-admin utility.
Install Apache and WSGI
To set up your project for production, you'll want to install Apache and mod_wsgi. This is because Django works best with Apache and mod_wsgi for production sites.
Make sure you have Apache installed, as Django will work with any version that supports mod_wsgi. You can check the mod_wsgi documentation to determine which mode is right for your setup.
In embedded mode, mod_wsgi operates similarly to mod_perl, loading Python code into memory when the server starts. This leads to significant performance gains over other server arrangements.
mod_wsgi can also run in daemon mode, spawning an independent process that handles requests. This can be beneficial for security and makes refreshing your codebase more seamless.
To use Django with mod_wsgi, you'll need to configure it properly, which is covered in the "How to use Django with mod_wsgi" article.
Installing a Distribution-Specific
Installing a Distribution-Specific Package is a viable option for getting Django up and running.
Check the distribution specific notes to see if your platform/distribution provides official Django packages/installers. Distribution-provided packages will typically allow for automatic installation of dependencies and supported upgrade paths; however, these packages will rarely contain the latest release of Django.
If you choose to go this route, you can expect a smoother installation process, but keep in mind that you may miss out on the latest features and bug fixes.
To get started, check the official Django documentation for distribution-specific notes. This will give you a clear idea of what to expect and how to proceed with the installation process.
Creating Project Directories & Environment
Creating a new project directory is a great first step in setting up your project. Create a main project directory and navigate into it. This should create an empty directory with the name project_tsukuyomi in your filesystem.
You'll want to install Django with pipenv to get started. Install Django with pipenv, and you'll notice two new files: Pipfile and Pipfile.lock.
To activate your virtual environment, use pipenv shell. This will give you a prompt indicating that you're running within the 'project_tsukuyomi' virtualenv.
Here's a quick rundown of what you should see:
Now you're ready to start creating your Django project and app. Remember to use pipenv shell to activate your virtual environment and ensure that you're working within the correct environment.
Adding Libraries to a Project
Incorporating Django libraries and packages into your project offers a range of significant benefits, which can enhance both the development process and the final product.
Defining package requirements is crucial when creating a Django app. This involves creating a requirements.txt file that includes all the necessary packages, such as the django package.
To create a requirements.txt file, you can use the pip freeze command to generate the file based on the exact libraries installed in the activated environment. This involves running pip freeze > requirements.txt in the terminal.
Libraries and packages provide pre-written code for common functionalities, allowing you to avoid reinventing the wheel. This accelerates the development process by reducing the amount of time needed to build features from scratch.
Developing complex functionalities from scratch can be costly in terms of both time and resources. Utilizing existing Django libraries and packages helps minimize development efforts, which can significantly reduce project costs.
Some popular Django framework packages include the Django REST Framework, which can be installed via the Python pip command pip install djangorestframework.
A Project Environment
To set up a project environment for your Django project, start by creating a virtual environment. This can be done by running the command `python3 -m venv .venv` in your terminal, which will create a virtual environment named `.venv` based on your current interpreter.
The virtual environment is a self-contained environment that allows you to install packages without affecting the global Python environment. It's a good practice to use a virtual environment for each project to avoid conflicts and make it easy to manage dependencies.
To activate the virtual environment, run the command `source .venv/bin/activate` on Linux/macOS or `.venv\Scripts\Activate.ps1` on Windows. Once activated, your terminal will display the name of the virtual environment, indicating that you're running within it.
Next, install Django in the virtual environment by running `python -m pip install django`. This will install the latest version of Django and make it available for use in your project.
Here's a list of steps to create a project environment:
- Create a virtual environment using `python3 -m venv .venv`.
- Activate the virtual environment using `source .venv/bin/activate` (Linux/macOS) or `.venv\Scripts\Activate.ps1` (Windows).
- Install Django using `python -m pip install django`.
- Update pip in the virtual environment using `python -m pip install --upgrade pip`.
By following these steps, you'll have a self-contained environment ready for writing Django code.
Database Management
Database management in Django is a breeze. You work with your database almost exclusively through the models you define in code, making it easy to represent the objects in that database using models.
To manage your database, you'll need to run the makemigrations and migrate commands. Makemigrations generates scripts in the migrations folder that migrate the database from its current state to the new state, while migrate applies the scripts to the actual database.
The general workflow is to make changes to the models in your models.py file, run python manage.py makemigrations, and then run python manage.py migrate to apply the scripts to the actual database.
Here are the basic settings you'll need to define in your project's settings file:
- ENGINE – The type of database you're using, such as 'django.db.backends.sqlite3', 'django.db.backends.postgresql', or 'django.db.backends.mysql'
- NAME – The name of your database, which will be a file on your computer if you're using SQLite
If you're using a database other than SQLite, you'll also need to define additional settings such as USER, PASSWORD, and HOST.
Types of Databases
Django includes a db.sqlite3 file for an app's database by default, which is suitable for development work. However, it's not recommended for higher volumes, as it's limited to a single computer.
SQLite works fine for low to medium traffic sites with fewer than 100 K hits/day, according to the SQLite documentation. For production-level sites, consider using a database like PostgreSQL, MySQL, or SQL Server.
Here are some popular database options for Django:
- PostgreSQL
- MySQL
- SQL Server
These databases are officially supported by Django and can handle higher volumes than SQLite. If you're planning to deploy your site in a production environment, it's recommended to use one of these databases.
Access Database via Models
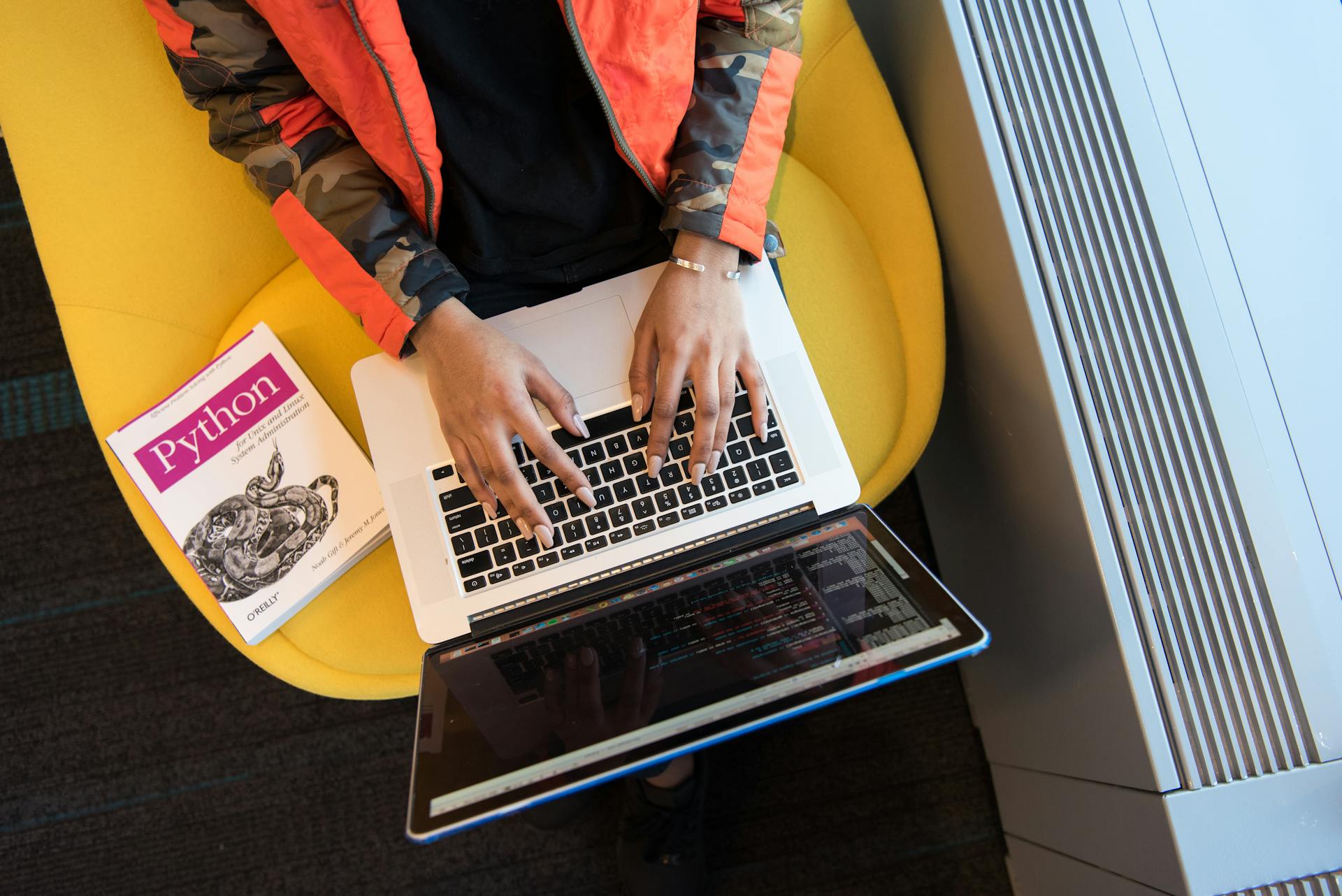
Accessing your database via models is a fundamental aspect of Django's database management system. You can work with your database almost exclusively through the models you define in code.
To represent objects in the database, you place model classes in an app's models.py file. These classes are derived from django.db.models.Model and are typically named after the database object they represent, such as a table.
A model class can include methods that return values computed from other class properties. For example, a model class can include a __str__ method that returns a string representation of the instance.
The fields in a model class are defined using types from django.db.models, such as CharField, TextField, and IntegerField. Each field takes attributes, like max_length, blank=True, null=True, and choices.
Here's a breakdown of the field types:
You can work exclusively with your model classes to store and retrieve data. Django handles the underlying details, making it easy to manage your database.
Frequently Asked Questions
Where do I deploy my Django project?
You can deploy your Django project on popular platforms like AWS, Azure, Google Cloud, Hetzner, DigitalOcean, or Heroku, each offering unique features and scalability options. Choose the one that best fits your project's needs and get started with deployment.
Sources
- https://anovin.mk/tutorial/how-to-correctly-package-a-django-app/
- https://code.visualstudio.com/docs/python/tutorial-django
- https://www.techforceservices.com/blog/django-framework-packages-for-web-development/
- https://docs.djangoproject.com/en/5.1/topics/install/
- https://python.plainenglish.io/setting-up-a-basic-django-project-with-pipenv-7c58fa2ec631
Featured Images: pexels.com