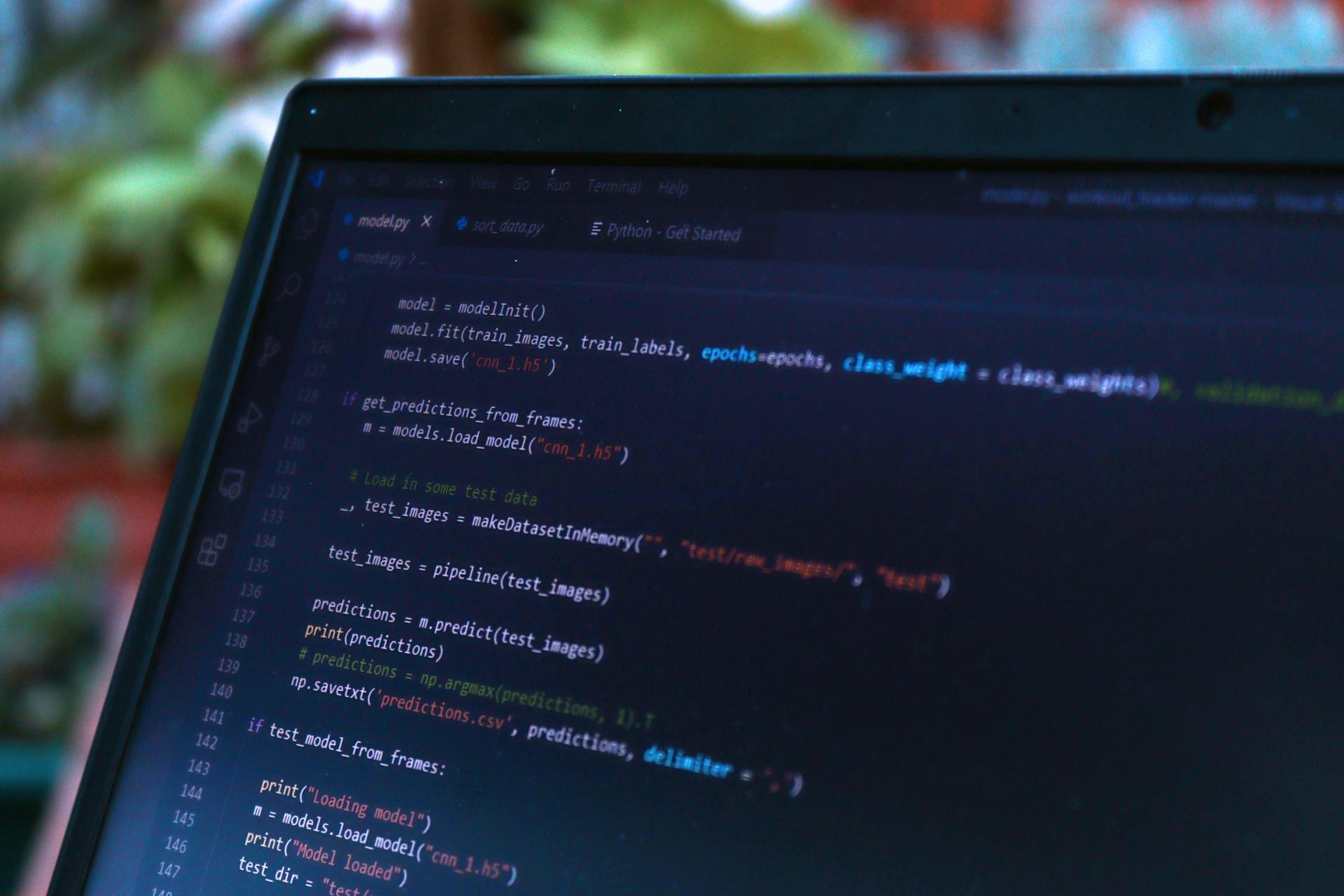
To install a Django project, you'll need to have Python and PIP installed on your computer. Python is the programming language that Django is built on, and PIP is the package manager that will help you install Django.
First, you'll need to install Python if you haven't already. You can download the latest version of Python from the official Python website.
You can then use PIP to install Django. This can be done by opening a terminal or command prompt and typing the command "pip install django".
Prerequisites
To start a Django project, you'll need a solid foundation. A server with Ubuntu 22.04 as its OS is the minimum requirement.
You'll also need to ensure you have the right user privileges. Specifically, you'll need to have either root privileges or be a non-root user with sudo privileges to make the necessary changes.
Here are the specific requirements in a concise list:
- A server with Ubuntu 22.04 as OS
- User privileges: root or non-root user with sudo privileges
Create Project
To create a Django project, you'll need to create a new virtual environment. This can be done using the command `python -m venv project`.
Next, activate the virtual environment by running `source project/bin/activate` on Linux or `project\Scripts\activate` on Windows.
Create a new Django project using the `django-admin startproject` command, followed by the project name, such as `django-admin startproject djangosite`.
Make sure to add your server IP address to the `ALLOWED_HOSTS` array in the `settings.py` file.
Create Project
To create a Django project, start by creating a new virtual environment with the command `python -m venv project`.
Create a new Django project by running `django-admin startproject djangosite` in the virtual environment.
Navigate to the `djangosite` directory and open the `settings.py` file to add your server IP address to the `ALLOWED_HOSTS` array.
Save and close the `settings.py` file, then migrate the Django database with the command `python manage.py migrate`.
Next, create a superuser to log into the Django admin interface by running `python manage.py createsuperuser`.
Start the Django project with the command `python manage.py runserver YOUR_SERVER_IP_ADDRESS:8000`.
with PIP
Creating a Django project can be a bit overwhelming, but don't worry, I've got you covered. First, you need to install Django using PIP, which is the recommended method for global system usage.
To start, check which PIP version is already installed with the command `pip3 -V`. Unless you need a specific version of PIP for another application, upgrade PIP to the latest version with the command `python3 -m pip install --upgrade pip`.
Next, install Django using the command `python3.10 -m pip install Django`. Once installed, ensure Django works by verifying the version with `python3.10 -m django --version`.
Here's a quick rundown of the steps:
After installing Django, you can start creating your project. Remember to create a new virtual environment, activate it, and then create a new Django project.
Install and Configure
To install Django, you can use the PIP command to install it via the PyPI repository. This method allows you to install the specific version of Django for your project.
You can install Django version 3.0.0 by running the command: `pip3 install Django==3.0.0`. After the successful installation, verify the Django version with the command: `django-admin --version`.
Alternatively, you can use virtualenv to create an isolated Python environment for your project. To do this, install the Python virtualenv package using the command: `sudo python3 -m pip install virtualenv`. Then, create a new Python virtual environment named venv using the command: `virtualenv venv`.
Set Up Python
To set up Python, you'll want to start by checking which PIP version is already installed. pip3 -V will give you the current version. Unless you need a specific version of PIP for another application, it's a good idea to upgrade PIP to the latest version with python3 -m pip install --upgrade pip.
By default, Python 3 comes pre-installed on Ubuntu 20.04, but you'll need to create a symbolic link of the Python3 binary from /usr/bin/python3 to /usr/bin/python. You can do this with the command:
You can verify the python command by running python -V, which will give you the Python version.
To install Pip, you can run the command: python3 -m pip install pip. After installation, create a symbolic link of the pip3 binary from /usr/bin/pip3 to /usr/bin/pip.
Verify the pip command by running pip -V, which will give you the Pip version.
Here's a quick reference guide to setting up Python and Pip:
Ubuntu 20.04
Ubuntu 20.04 is a popular Linux distribution that comes with Python 3 pre-installed, but you'll need to create a symbolic link to access it.
You can create a symbolic link by running the command `sudo ln -s /usr/bin/python3 /usr/bin/python`. This will allow you to use the `python` command instead of `python3`.
Python 3 comes with Pip pre-installed, but you'll need to install it separately. You can do this by running the command `sudo apt update && sudo apt install python3-pip`.
After installing Pip, you'll need to create another symbolic link to access it. Run the command `sudo ln -s /usr/bin/pip3 /usr/bin/pip`.
Once you've completed these steps, you can verify the Python and Pip versions by running the commands `python --version` and `pip --version`.
If you're planning to install Django, you can do so using the default repository by running the command `sudo apt update && sudo apt install python3-django`.
Using PIP
Using PIP to install Django is a popular and recommended method. It's easy to upgrade to the latest version of PIP and then install Django.
To start, check which version of PIP is already installed by running the command `pip3 -V`. You can then upgrade to the latest version using `python3 -m pip install --upgrade pip`. This will ensure you have the most up-to-date PIP package manager.
Once PIP is upgraded, you can install Django using the `python3.10 -m pip install Django` command. This will install the Django framework on your system.
To ensure Django is working correctly, you can verify the version by running the command `python3 -m django --version`. This will display the version of Django that was installed.
Here's a step-by-step guide to installing Django using PIP:
- Check the current PIP version: pip3 -V
- Upgrade PIP to the latest version: python3 -m pip install --upgrade pip
- Install Django: python3.10 -m pip install Django
- Verify the Django version: python3 -m django --version
Database and Migrations
Now that you have your Django project set up, it's time to think about the database. The default database for a Django project in PyCharm is SQLite, which is a great choice for small projects.
To create tables in the database for your models, you'll need to use migrations. Migrations are human-editable files that store changes to your data models.
To start the migration process, open a terminal tab and type `makemigrations` followed by Enter. This will create a new migration file in the `todo/migrations` directory.
The new migration file will be named something like `0001_initial.py`. This file contains the changes to your data model.
Once you've created the migration file, you can apply the changes to your database by typing `migrate` and pressing Enter. This will create the tables in your database for your models.
Here's a step-by-step guide to migrating your database:
- Open a terminal tab and type `makemigrations` followed by Enter.
- Run the command `migrate` to apply the changes to your database.
After running `migrate`, you should see a message indicating that the migration was successful. This means that the tables have been created in your database for your models.
Sources
- https://www.rosehosting.com/blog/how-to-install-django-web-framework-on-ubuntu-22-04/
- https://www.inmotionhosting.com/support/edu/control-web-panel/cwp-admin/server-software/install-django-cwp/
- https://cloudinfrastructureservices.co.uk/how-to-install-django-on-ubuntu-20-04/
- https://www.jetbrains.com/help/pycharm/creating-and-running-your-first-django-project.html
- https://pypi.org/project/Django/
Featured Images: pexels.com