
Streamlining Django project deployment can be a daunting task, but packaging your project into an executable file can make it much easier. You can use tools like PyInstaller to create a single executable file that includes all the necessary dependencies.
This approach eliminates the need to install Python and other dependencies on the target machine, making it more convenient for users.
With PyInstaller, you can create a standalone executable file that can run on any Windows system without requiring Python to be installed.
You can also use tools like cx_Freeze to create a Windows executable file that includes all the necessary dependencies.
Preparing Your Project
To package your Django project into an executable, you'll need to prepare it first. This involves creating an entry-point script, which is a Python script that starts your project or application.
The entry-point script is usually a small script outside of your Django package that imports your package and runs the main function. You can create this script, for example, by calling main() to start up your project.
Readers also liked: Java Maven Package Aws S3
This entry-point script is crucial because it tells PyInstaller how to start your project. Luckily, if you're working on your own project, creating this script is straightforward because you're familiar with the code.
However, if you're working with someone else's code, it can be more challenging to find the entry-point. In this case, you can look at the setup.py file in the project's directory and search for a reference to the entry_points argument.
The entry-point script should call the same function mentioned in the entry_points argument. You can see an example of this in the Real Python feed reader project, where the entry-point script cli.py calls the same function mentioned in the entry_points argument.
Once you've created the entry-point script, you'll want to make sure it's correctly set up in your project directory. This means the entry-point script should be in the same directory as the reader package, and there should be no changes to the reader code itself.
However, be aware that you might also have hidden imports in your code, which are imports that are not explicitly mentioned in the entry-point script. These hidden imports can cause issues with PyInstaller, but don't worry, you can manually specify them later.
Packaging and Distribution
Packaging and distribution of a Django project into an executable file (EXE) is a crucial step in making it easily distributable to users. PyInstaller is a popular tool for achieving this, and it works well with Django projects.
PyInstaller can generate executables for Windows, Linux, and Mac with a simple command, and it abstracts the details of packaging your project's dependencies. Your users won't even know they're running a Python project because the Python Interpreter itself is bundled into your application.
To use PyInstaller, you'll need to have Python, Setuptools, and pywin32 dependency installed on Windows. Once installed, you can run the pyinstaller command from the terminal to convert your main.py file to an executable. The executable file will be found in the dist folder along with all its dependencies.
Here are the basic steps to package your Django project into an executable file with PyInstaller:
- Install PyInstaller via pip
- Run the pyinstaller command from the terminal to convert your main.py file to an executable
- Specify the --onefile option to generate a single executable with no dependencies
- Use the --icon option to specify your own icon for the executable
PyQt5 Packaging and Distribution
PyInstaller abstracts the details of packaging and distribution by finding all your dependencies and bundling them together. Your users won’t even know they’re running a Python project because the Python Interpreter itself is bundled into your application.
PyInstaller can create executables for Windows, Linux, and Mac with a simple command. It can also generate a single executable with no dependencies, making it easier to distribute, but take longer to initialize.
You can use PyInstaller to package PyQt5 applications for Windows with InstallForge, or for Linux with fpm. You can also use QResource to package data files with PyInstaller.
To package a PyQt5 application with PyInstaller, you need to have installed Python, Setuptools, and pywin32 dependency on Windows. You can then use the pyinstaller command to create an executable.
PyInstaller generates a few things of interest, including a *.spec file, a build/ folder, and a dist/ folder. The executable file will be found in the dist folder along with its dependencies.
Here are some common use cases for PyInstaller:
- Packaging PyQt5 applications for Windows with InstallForge
- Packaging PyQt5 applications into a macOS app with PyInstaller
- Packaging PyQt5 applications for Linux with PyInstaller and fpm
- Using QResource to package data files with PyInstaller
To create a distributable Windows installer, you can use InstallForge. You can also use the --onefile option to generate a single executable with no dependencies.
Hiding the Console Window
You can hide the console window that runs in the background when you run your packaged application. This is especially useful for GUI applications where you don't want the console window visible.
To turn off the console window, you can edit the .spec file and set console=False under the EXE block. Alternatively, you can re-run the pyinstaller command with the -w, --noconsole or --windowed configuration flag.
Running your application with the console window hidden can make it look more professional and user-friendly. It's a simple step that can make a big difference in the overall user experience.
By using the -w, --noconsole or --windowed flag, you can easily hide the console window without having to edit the .spec file. This is a quick and easy solution that can save you time and hassle.
If this caught your attention, see: How to Run Django Project
Freezing and Bundling
Freezing and bundling is a crucial step in packaging your Django project into an executable file. You can use tools like cx_Freeze, which is similar to PyInstaller and can generate executables for Windows, Linux, and Mac.
To freeze your project using cx_Freeze, you'll need to install it via pip and then run the command `python setup.py bdist_wheel` to create a wheel file. The `-c` option specifies the name of the file to convert and `--target-dir` specifies the directory where the executable file will be stored.
You can also use PyInstaller to bundle data files with your project. Simply tell PyInstaller to copy the files over by using the `--add-data` option, which accepts a list of individual file paths to copy over. The path separator is platform-specific, so use `;` on Windows and `:` on Linux or Mac.
If you need to bundle folders instead of individual files, you can use PyInstaller's latest versions to bundle folders just like you would files. This keeps the sub-folder structure intact, making it easy to manage your project's files.
Additional reading: Azure Data Engineer End to End Project
Cx Freeze
Cx Freeze is a tool that can generate executables for Windows, Linux, and Mac. You can install it via pip by running a simple command.
To use Cx Freeze, you need to install it first, and then run a command in the terminal where your main file is located. The command should include the -c option with the name of the file to convert and --target-dir to specify the directory where the executable file will be stored.
The -c option is used to specify the name of the file to convert, and --target-dir is used to specify the directory where Cx Freeze will store the executable file. You can combine options, such as adding --base-name=Win32GUI to hide the console on Windows.
For more advanced freezing, you might need to provide include and exclude paths, but for basic one-file scripts, Cx Freeze should work without any issues.
Bundling Data Folders
Bundling data folders is a crucial step in freezing and bundling your application. You can use PyInstaller to bundle folders just like you would files, keeping the sub-folder structure.
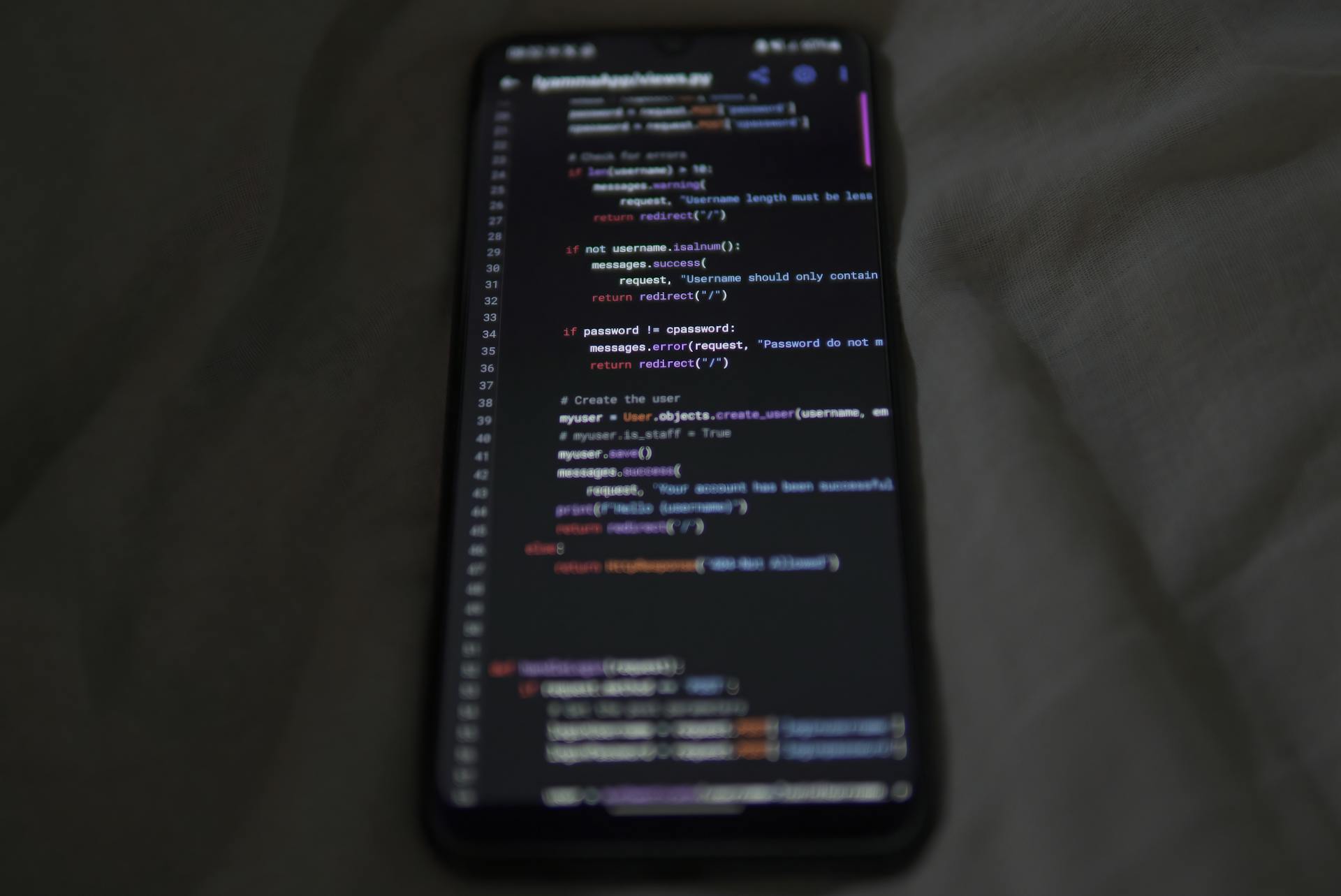
To bundle a folder, you can add it to your .spec file Analysis block. The paths should use the Unix forward-slash / convention, so they're cross-platform for macOS. If you're only developing for Windows, you can use \\ instead.
For example, if you have a subfolder named 'icons' containing your icons, you can add it to your .spec file like this: 'icons/'.
Alternatively, you can bundle your data files using Qt's QResource architecture. See our tutorial for more information.
If you run the build using this spec file, the icons folder will be copied across to the dist folder. The relative paths will remain correct in the new location, so your application will display the icons as expected.
For your interest: How to Add Sitemap to Django Project
Sources
- https://stackoverflow.com/questions/77123444/create-an-exe-file-from-a-django-project-with-pyinstaller
- https://pythonassets.com/posts/create-executable-file-with-pyinstaller-cx_freeze-py2exe/
- https://www.pythonguis.com/tutorials/packaging-pyqt5-pyside2-applications-windows-pyinstaller/
- https://docs.python-guide.org/shipping/freezing/
- https://realpython.com/pyinstaller-python/
Featured Images: pexels.com