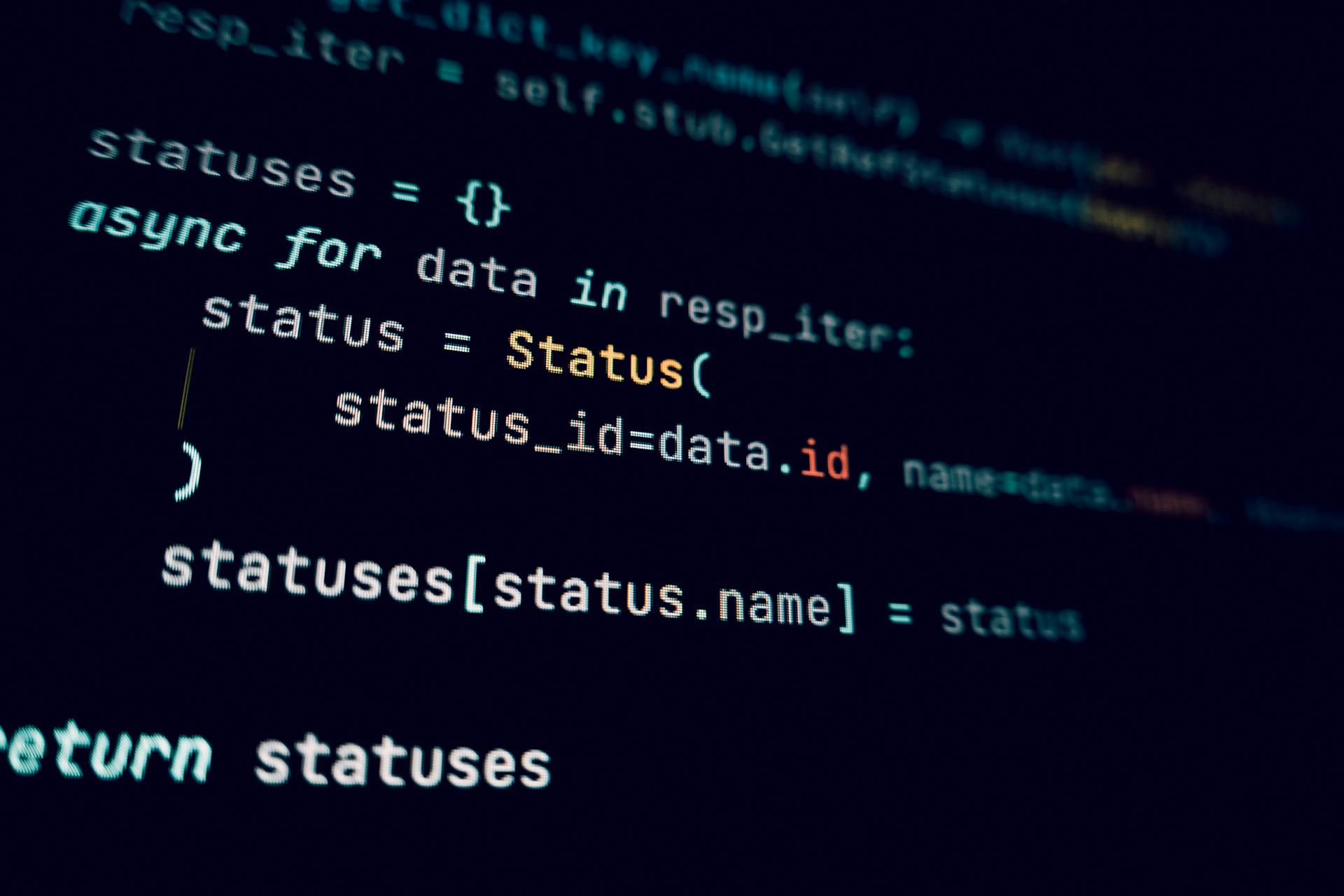
Building a Next JS REST API is a fantastic way to create a robust and scalable application. In this tutorial, we'll cover the basics of setting up a Next JS REST API using the Next JS API routes feature.
To get started, you'll need to create a new Next JS project using the `npx create-next-app` command. This will set up a basic Next JS project with a default API route.
Next, we'll explore how to create API routes using the `pages/api` directory. For example, you can create a simple API route to handle GET requests by creating a file called `hello.js` in the `pages/api` directory.
Additional reading: Nextjs App vs Pages
Dynamic Routing
Dynamic routing is a game-changer for Next.js applications, allowing you to access individual data with efficiency by adding custom parameters to your URLs. You can create dynamic routes for files, folders, and even catch-all paths.
To create a dynamic route, you use bracket syntax, like [id].js, and Next.js will automatically know it's a dynamic route. This means you can render a plethora of posts from an API without having to create a route for each one.
Dynamic routes can also be extended to catch all paths by prefixing them with an optional catch-all route. For example, pages/blog/[...id].js matches /blog/1, /blog/1/2, and so on. This is a great way to handle complex routes without having to create multiple routes.
See what others are reading: Nextjs Client Side Cookies
Dynamic Routing Basics
Dynamic routes can be created by adding custom parameters to your URLs using bracket syntax, like [id].js, which Next will automatically know is a dynamic route. This allows you to access individual data with efficiency.
Dynamic routes are not limited to files alone; you can create dynamic routes for folders too. This means you can have multiple dynamic route segments.
The useRouter hook has a query object property that exposes dynamic route parameters. This query object is usually empty during pre-rendering if the page is not using server-side rendering.
Routing to /post/123 will give the query object {"id": "123"}.
Here's an example of how to create a dynamic route:
- Create a file named pages/post/[id].js
- The file will automatically know it's a dynamic route and will expose the dynamic route parameter in the query object.
Dynamic routes can be extended to catch all paths by prefixing a dynamic route with three dots, like [...id].js. This will match paths like /blog/1, /blog/1/2, and so on.
Here are some examples of dynamic routes:
It's good practice to not have more than one dynamic route inside a folder level.
Simulating Webhooks and Callbacks
Simulating webhooks and callbacks is a crucial aspect of testing your application's behavior when receiving asynchronous events from third-party services or APIs. This allows you to ensure your application responds correctly to these events.
To simulate webhooks, you can use a mock API server to test your application's behavior. This is exactly what you would do when learning how to simulate webhooks or callbacks in your mock API server.
By simulating webhooks and callbacks, you can test your application's ability to handle asynchronous events from third-party services or APIs. This is particularly important when developing applications that rely on external services.
A mock API server can be used to simulate webhooks and callbacks, allowing you to test your application's behavior in a controlled environment.
Explore further: Why Are Apis Important
Working with Endpoints
To create API endpoints with Next.js, you simply need to create a function within the /pages/api directory. For example, let's create an API handler that returns "Hello World" by creating a file at /pages/api/hello-word.ts and exporting a default function that handles the request.
Readers also liked: Next Js Create App
You can handle different HTTP methods within the same API route by checking the req.method property. This allows you to respond differently based on the method, such as using a switch statement to determine the response.
API endpoints are global, meaning they will respond to any HTTP method (GET, POST, PUT, DELETE, etc.) with the same response. However, if you want to respond differently based on the method, you will need some logic like a switch statement.
Next.js functions are extremely easy to write, but it takes some mastery to write more complex controllers. This is why it's often recommended to write the logic outside of the functions and simply use them for the routing logic.
Here are the three situations when you want to execute an HTTP function within components:
- To fetch the initial component data
- To continually fetch data from the same component when some dependent property changes
- To make a request to respond to the user's interaction (for example, submitting a form)
To create API routes, you need to create a new directory called ‘api’ inside the app directory, which is the route for all the API endpoints. In this directory, you can create files for different API endpoints, such as ‘GET’ and ‘POST’ requests.
Related reading: Next Js Src Directory
Here are the key features of Next.js API routes:
- Serverless Functionality: Next.js API routes are serverless functions, which means they scale automatically and are only invoked when needed, leading to efficient resource utilization.
- Built-in Middleware Support: Customize API behavior with built-in middleware, enabling tasks like request parsing, CORS handling, and more.
- Easy to Set Up: With no additional configuration required, setting up API routes is straightforward, making it accessible for developers of all skill levels.
Managing Data
You can manage data in your Next.js application by using API endpoints to fetch and update data. This is especially useful when you need to retrieve data to display in components.
To fetch initial component data, you can use the `useApiRequest` hook, which takes two parameters: `path` and `method`. The hook returns an array with a `callback` function to execute the fetch request and the current state of the request.
To fetch data on changes, you can add a dynamic dependency to `useEffect`, which is the path of the API endpoint to request.
Here are some best practices for efficient data handling:
- Pagination: Implement pagination to limit the data sent in a single response.
- Data Caching: Cache data that doesn't change often at the server level to reduce database load and improve response times.
By following these practices, you can improve the performance of your API and the experience for end-users.
Error Handling and Security
Error Handling and Security is crucial for a Next.js REST API. You can't just build an API and expect it to be secure, you need to implement proper error handling and security measures.
Explore further: Nextjs Error Boundary
To protect sensitive endpoints, implement authentication and authorization checks, such as using JWT (JSON Web Tokens) or session-based authentication mechanisms. This will ensure that only authorized users can access your API.
Input validation is also essential to prevent SQL injection, cross-site scripting (XSS), and other common web vulnerabilities. You can use libraries like Joi or Yup for schema validation to validate all incoming data.
Here's a quick rundown of some key security measures:
- Authentication and Authorization: Implement JWT or session-based authentication mechanisms.
- CORS (Cross-Origin Resource Sharing): Configure CORS policies to restrict which domains can access your API.
- Rate Limiting: Implement rate limiting to prevent abuse and ensure the availability of your API.
- Input Validation: Validate all incoming data using libraries like Joi or Yup.
Handle Errors Gracefully
Handling errors in a way that's both informative and user-friendly is crucial for a smooth experience. Implement a consistent structure for error responses to avoid confusing clients. This should include a standardized error message and a status code that accurately reflects the nature of the error.
A try/catch block is your best friend when it comes to catching exceptions and responding with the right error messages and status codes. This helps prevent the application from crashing and provides a clear indication of what went wrong.
Here's an interesting read: Nextjs Error Page
Validation errors can be particularly frustrating for users, but providing detailed error messages can help them correct their requests. This is where validation errors come in – make sure to validate request data and provide clear instructions on what needs to be fixed.
Here are some key takeaways for handling errors gracefully:
- Implement consistent error responses with a standardized error message and status code.
- Use try/catch blocks to catch exceptions and respond with error messages and status codes.
- Provide detailed error messages for validation failures to help clients correct their requests.
Secure Your
Securing your API is crucial to prevent unauthorized access and protect sensitive data. Implementing authentication and authorization checks is a must, and you can use JWT (JSON Web Tokens) or session-based authentication mechanisms to get started.
Authentication and authorization are like having a bouncer at the door - it ensures only authorized people get in. Consider using libraries like Joi or Yup for schema validation to protect against common web vulnerabilities.
CORS (Cross-Origin Resource Sharing) policies are essential to restrict which domains can access your API. This prevents unauthorized access from other domains and keeps your data safe.
Expand your knowledge: Save Api Data on Local Storage Next Js
Rate limiting is another important security measure that prevents abuse and ensures the availability of your API. Limiting the number of requests a user can make within a certain timeframe helps maintain API availability.
Input validation is also critical to prevent SQL injection, cross-site scripting (XSS), and other common web vulnerabilities. Make sure to validate all incoming data to keep your API secure.
Here are some key security measures to implement:
- Authentication and Authorization: Implement authentication and authorization checks using JWT or session-based authentication.
- CORS (Cross-Origin Resource Sharing): Configure CORS policies to restrict which domains can access your API.
- Rate Limiting: Implement rate limiting to prevent abuse and ensure API availability.
- Input Validation: Validate all incoming data using libraries like Joi or Yup for schema validation.
Frequently Asked Questions
Is Next.js good for REST API?
Yes, Next.js is well-suited for building REST APIs due to its built-in API routes support. This makes it an easy solution for creating server-side rendered applications with robust API functionality.
Can I use Next.js for backend?
Yes, you can use Next.js with an existing backend, but it's not a custom server. Next.js includes its own server, but you can still integrate it with your existing backend.
Sources
- https://mattermost.com/blog/dynamic-routing-in-next-js-using-rest-and-graphql-apis/
- https://makerkit.dev/blog/tutorials/how-to-call-api-nextjs
- https://mockoon.com/tutorials/nextjs-api-call-and-mocking/
- https://kapsys.io/user-experience/exploring-next-js-api-routes-for-server-side-operations
- https://www.bytestechnolab.com/blog/build-rest-apis-in-nextjs-with-prisma-orm-full-guide/
Featured Images: pexels.com