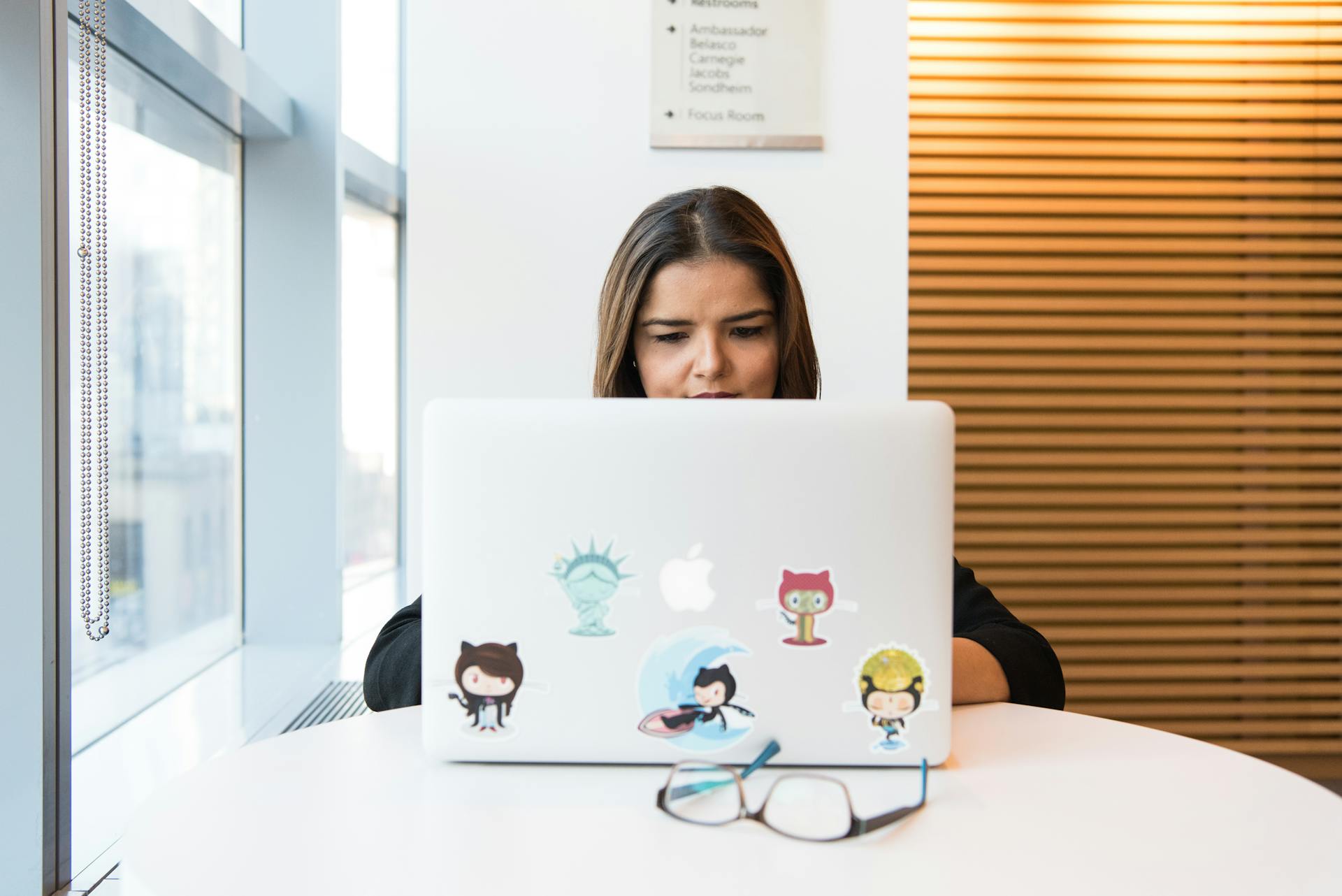
In Next.js, pagination is achieved through the use of APIs and data fetching. This allows for efficient data display and a seamless user experience.
Next.js provides built-in support for pagination through its API routes and getStaticProps method.
To implement pagination, you need to define a paginated API route that returns a limited number of items at a time. This can be achieved using the limit and skip parameters in your API route.
For example, you can use the following API route to fetch a limited number of items: `const items = await fetch('/api/items?limit=10&skip=0')`.
A different take: Nextjs Use Server
Setting Up Pagination
To set up pagination in Next.js, you'll need to create a new page for each page of data, with a unique URL for each one.
The `getStaticProps` function is used to fetch data for each page, and it's where you'll implement pagination logic.
Next.js provides a built-in `paginate` function that helps you break down large datasets into smaller chunks.
Worth a look: Nextjs App Route Get Ssr Data
Each page will have a unique `page` parameter, which is used to fetch the correct data from the API. For example, `page=2` will fetch the second page of data.
To link between pages, you'll need to create a pagination component that displays links to each page. This component will use the `page` parameter to determine which page to link to.
You might enjoy: Nextjs Link Component
Implementing Pagination
Implementing pagination in a Next.js application involves several key steps. To start, you'll need to prepare your Backend API to handle pagination, including providing a total count of items, the current page, and items of the page. Ideally, your backend should also support fetching items of a certain page, such as https://api.yourapp.com/posts?page=3.
To implement pagination in your Next.js application, you'll need to use URL query string parameters. This allows you to handle state across page reloads and facilitates sharing URLs with specific pages. Next.js's useRouter hook makes handling query parameters straightforward.
A unique perspective: Is Next Js Backend
To build the pagination component, create a new file named Pagination.js in the components directory of your Next.js app. This component will manage the current page state and render buttons for navigating between pages. The handlePageChange function updates the current page and modifies the URL to reflect the new page.
The pagination component will also need to display next and previous buttons. For the Previous button, display this when not on page 1. If on page 2, output '/blog' as the href value. Otherwise, pass an object which takes a pathname and query value. The pathname is '/blog', and for the query, set page to pageNum - 1.
Here's a summary of the pagination component's key features:
- Uses URL query string parameters to handle state across page reloads
- Displays next and previous buttons based on the current page number
- Updates the URL to reflect the new page when the user selects a different page
- Uses the useRouter hook to handle query parameters
To integrate search functionality with pagination, modify the data fetching logic to consider the current search term when making API requests. Ensure that both search term and page number are used to fetch the relevant data. This can be achieved by updating the getServerSideProps function to handle both search and pagination parameters.
Data Fetching and Display
In a Next.js app, fetching data can be efficiently handled using server-side methods like getServerSideProps or getStaticProps.
You can use getServerSideProps to fetch data on each request, which is particularly useful for paginated data. The getServerSideProps function allows you to fetch data from an API endpoint, including the total number of pages.
The data and pagination details are passed as props to the Home component, making it easy to render the data on the index page. The Pagination component can be used to render the pagination controls.
To display data on the index page, you'll need to map through the data items and render them as a list or grid. This involves using a component like the Home component to render the data.
The getServerSideProps function is a powerful tool for fetching data in a Next.js app, and it's especially useful for paginated data. By using this function, you can ensure that your data is always up to date.
In the end, the goal is to render the data on the index page in a way that's easy to understand and use. The Pagination component can help with this by providing pagination controls.
You might like: Nextjs Loading
Handling Pagination State
Handling Pagination State is crucial for a seamless user experience in your Next.js application. It involves managing the current page and updating it based on user interactions, which can be achieved using state hooks and router methods.
Updating the Pagination Component is essential to handle state changes and update the current page accordingly. This can be done by ensuring your pagination component can handle state changes.
Using URL Parameters to Manage State is a great approach to preserve pagination state across page reloads and share URLs with specific pages. Next.js's useRouter hook makes handling query parameters straightforward.
The current page is derived from the query parameters, and the handlePageChange function updates the query parameters to reflect the new page. This is done by modifying the pagination component to read and update the query parameters.
Fetch Data Based on Query Parameters is another crucial step, where you use getServerSideProps to fetch data based on the current page from the query parameters. This ensures that the data is fetched accordingly based on the current page.
If this caught your attention, see: Next Js Url Params
Here's a step-by-step guide to handling pagination state:
1. Update the Pagination Component to handle state changes.
2. Use URL Parameters to manage state across page reloads.
3. Modify the pagination component to read and update the query parameters.
4. Fetch data based on query parameters using getServerSideProps.
By following these steps, you can effectively handle pagination state in your Next.js application and provide a smooth user experience.
A unique perspective: Nextjs Usecontext
Customizing Pagination Behavior
Customizing pagination behavior is crucial to fit the specific needs of your application. This involves handling edge cases, customizing the appearance, or adding additional functionality.
To handle edge cases, you'll want to ensure that navigating beyond the first or last page is properly managed. This is done by ensuring that edge cases are handled correctly.
Customizing the appearance of the pagination component is also a great way to tailor it to your application's needs. This can be achieved using CSS modules or styled-components.
Adding features like showing the total number of pages, current page indicator, and more can enhance the user experience.
See what others are reading: Edge Runtime Nextjs
Customizing Behavior
Customizing pagination behavior is all about tailoring the logic to fit your application's specific needs. This might involve handling edge cases, customizing the appearance, or adding additional functionality.
Handling edge cases is crucial to ensure that users can navigate beyond the first or last page without issues. To achieve this, you need to ensure that edge cases are properly managed.
Customizing the appearance of the pagination component is a great way to make it blend in with your application's design. You can use CSS modules or styled-components to achieve this.
Adding features like showing the total number of pages or a current page indicator can enhance the user experience. This can be done by adding additional functionality to the pagination component.
Here are some ways to customize pagination behavior:
- Handling edge cases
- Customizing the appearance
- Adding features like showing the total number of pages or a current page indicator
Optimizing for Performance
Optimizing for Performance is crucial when building a robust pagination system in your Next.js application. This ensures your application remains responsive and efficient, even with large datasets.
Readers also liked: Nextjs Spa
Server-Side Data Fetching is a technique that fetches data efficiently and reduces the load on the client. This can be achieved using getServerSideProps or getStaticProps.
Client-Side Data Fetching with Caching is another technique that minimizes the number of API requests. Libraries like react-query or SWR can be used to implement client-side data fetching with caching mechanisms.
Debouncing Search Inputs is essential to minimize the number of API requests when users type in the search field. This can be implemented by using debouncing techniques.
Here are some techniques to optimize pagination for performance:
- Use Server-Side Data Fetching with getServerSideProps or getStaticProps.
- Implement Client-Side Data Fetching with caching using libraries like react-query or SWR.
- Debounce search inputs to minimize API requests.
Sources
- https://everythingcs.dev/blog/nextjs-server-components-pagination-searching-drizzle-orm/
- https://docs.directus.io/blog/implementing-pagination-and-infinite-scrolling-in-next-js
- https://www.dhiwise.com/post/ultimate-guide-to-nextjs-pagination-for-beginners
- https://vpilip.com/how-build-simple-pagination-in-nextjs/
- https://ste.digital/blog/simple-server-side-pagination-next-js
Featured Images: pexels.com