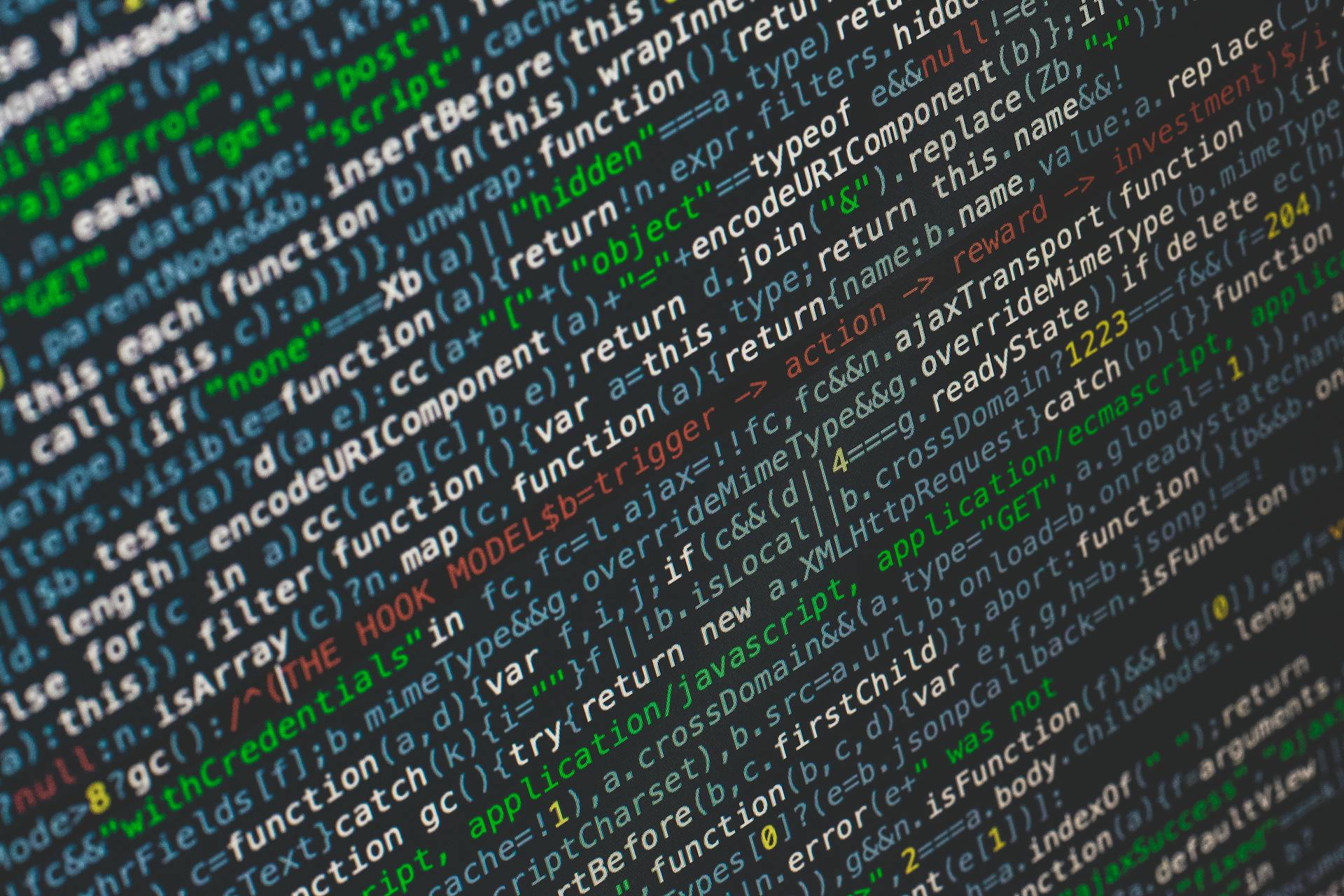
Next.js offers a wide range of customization options for ThemeProvider, allowing you to tailor the look and feel of your application to suit your needs.
You can change the theme's palette by passing a new theme object to the ThemeProvider component, as shown in the article section example, "Customizing the theme with ThemeProvider".
To add a custom theme, you need to create a new theme object and pass it to the ThemeProvider component, like this: `ThemeProvider theme={myCustomTheme}>`.
Readers also liked: Nextjs Theme
Material UI Setup
To start using Material UI in a Next.js project, you need to install Material UI Core, which offers a range of reusable UI components for web application development. This is the heart of Material UI, allowing you to harness the power of the Material Design system.
Material UI Core is installed independently of Material UI Icons, which is another package that supplies a compilation of icons in accordance with Material Design principles. You'll need to install both packages to get the most out of Material UI.
Worth a look: Shadcn Ui Nextjs
Here are the steps to install Material UI Core and Icons:
- Material UI Core: the core of Material UI, offering reusable UI components.
- Material UI Icons: an independent package supplying a compilation of icons in accordance with Material Design principles.
Once you've installed Material UI Core and Icons, you'll need to configure your Next.js application to utilize them. This involves modifying the _app.js file, which is responsible for initializing pages and is executed both on the server-side and client-side.
Worth a look: Next Js Client Side Rendering
Material UI Installation
To install Material UI, you need to install Material UI Core, which is the heart of Material UI, offering a range of reusable UI components for web application development.
Material UI Core can be installed as a separate package, allowing us to harness the power of the Material Design system.
Here are the steps to install Material UI Core and Icons:
- Install Material UI Core, a collection of React UI components that adheres to Google’s Material Design guidelines.
- Install Material UI Icons, an independent package that supplies a compilation of icons in accordance with Material Design principles.
By installing these two packages, we can create modern and responsive web applications that not only look good but also provide a seamless user experience.
Create a CSS Color File
To create a CSS color file, start by creating a new file called /styles/colors.css. This file will store all the colors used in your Material UI setup, both for Light and Dark modes.
For your interest: Apply Css Nextjs
In this file, you can define variables for your colors and apply them to the root element using the :root pseudo-class. This will set the colors for Light mode.
For Dark mode, you can use the [data-theme='dark'] attribute to apply different colors. This attribute is added and updated whenever the user toggles the theme, thanks to the next-themes library that we installed earlier.
A different take: Next Js Strict Mode
On Change
To implement a smooth transition between light and dark themes, we can utilize the next-themes library, which simplifies the process with convenient hooks and components.
The onChangeTheme function is used to change the current theme, receiving the theme name as a parameter. This function is a crucial part of managing the theme switch.
With the NextThemeProvider provider, you can set the theme for your app and access the current theme using a hook. This provider also includes a function to change the theme, making it easy to implement a theme switcher.
The system theme name for the current page can be accessed using the NextThemeProvider provider. This allows you to keep track of the current theme and make adjustments as needed.
A fresh viewpoint: Nextjs Usecontext
Components
Creating a Theme Switcher component is the first step to implementing a theme provider in Next.js. This component will allow users to toggle between light and dark modes.
To create the Theme Switcher component, you'll need to create a component folder and inside it, create a ThemeSwitcher.jsx file. In this file, you can use the useTheme hook from next-themes to fetch the current theme and control theme toggling.
You can use two SVG toggle icons to beautify the toggle button, or use your own. The Theme Switcher component will now switch between dark and light mode. However, you may not see any significant changes because you haven't added any styles for dark or light versions yet.
To add styles for dark and light versions, you'll need to import the ThemeSwitcher in the app/layout.js file and add it before the children. This will enable the theme switching functionality.
Here's a step-by-step guide to creating the Theme Switcher component:
- Create a component folder and inside it, create a ThemeSwitcher.jsx file.
- Import the useTheme hook from next-themes in the ThemeSwitcher.jsx file.
- Use the useTheme hook to fetch the current theme and control theme toggling.
- Add two SVG toggle icons to beautify the toggle button.
- Import the ThemeSwitcher in the app/layout.js file and add it before the children.
By following these steps, you'll be able to create a functional Theme Switcher component that allows users to toggle between light and dark modes.
Customization
Customization is where you can get really creative with your ThemeProvider Next.js app. You can style the application in different ways for each theme using utility classes.
To add Tailwind to your application, use the following commands: npm install tailwindcssnpx tailwindcss init
With Tailwind, you can easily style the site using utility classes. Simply use the "dark:" prefix before any Tailwind class to set a style that will be visible in dark mode. For example, using "dark:bg-white" will create a white background for light mode and a black background for dark mode.
Explore further: Next Js Tailwind Spinner Loading Page
Style the Application
To style your application, you can use a library like Tailwind. This allows you to easily add dark mode-specific styles using the "dark:" prefix before any Tailwind class.
With Tailwind, you can create a white background for light mode and a black background for dark mode by using the "dark:bg-black" class. This makes it easy to customize your app.
You might like: Nextjs App Router Tailwind Loading Page
You can also use Tailwind utility classes to style your application. For example, you can use the "dark:text-white" class to set text color to white in dark mode.
You can customize the app using Tailwind utility classes, or you can use your own styles. The key is to use the "dark:" prefix to apply styles specifically to dark mode.
Tailwind makes it easy to implement and maintain dark mode-specific styles by generating new classes for items in dark mode.
Explore further: Nextjs Server Rendering Tailwind
Integration with Next.js
Integration with Next.js is a breeze when you have the right tools. To get started, you need to install Material UI, a collection of React UI components that adheres to Google's Material Design guidelines.
To configure Material UI components in your Next.js project, you'll need to follow a few steps. First, import the ThemeProvider in your app/layout.js file, then wrap the children with the ThemeProvider. This will allow you to use Material UI components in your Next.js project.
Here's an interesting read: Nextjs Ui
You can also use the next-themes library to simplify the implementation of dark mode. This library provides convenient hooks and components that manage the underlying logic, making it easy to switch between light and dark themes. To use it, you'll need to install it via npm and create a custom ThemeProvider component.
Here are the steps to create a custom ThemeProvider component:
Material UI and Next.js Integration
Material UI and Next.js Integration is a powerful combination that allows you to create stunning web applications with a seamless user experience.
To get started, you'll need to install Material UI, a collection of React UI components that adheres to Google's Material Design guidelines. This will give you access to a range of pre-built components suitable for production deployment.
You can install Material UI using npm or yarn, and then configure it in your Next.js project by modifying the _app.js file. This file is responsible for initializing pages and is executed both on the server-side and client-side.
Recommended read: Next Js Npm Install
Once you've installed Material UI, you'll need to configure it to work with Next.js. This involves setting up the Material UI theme and managing style injection through Material UI's caching mechanism.
To simplify the implementation of dark mode, you can use the next-themes library. This library provides convenient hooks and components that manage the underlying logic, allowing you to easily switch between light and dark themes.
Here's a summary of the steps to integrate Material UI with Next.js:
- Install Material UI using npm or yarn
- Configure Material UI in your Next.js project by modifying the _app.js file
- Set up the Material UI theme and manage style injection
- Use the next-themes library to simplify the implementation of dark mode
By following these steps, you can create a modern and responsive web application with a beautiful user interface.
Pages/_app.tsx
In Next.js, the _app.tsx file is crucial for initializing pages and is executed both on the server-side and client-side. It's responsible for setting up the Material UI theme and managing style injection through Material UI's caching mechanism.
To configure _app.js for Material UI, you'll need to modify the file to utilize the theme and caching mechanism. This involves installing Material UI Core and Icons, and then modifying the _app.js file to set up the theme.
On a similar theme: Nextjs Server Actions File Upload
You can add the TamaguiProvider to the _app.tsx file to manage style injection. This involves passing the appDir boolean to @tamagui/next-plugin. By doing so, you'll ensure that the application uses the correct theme functions.
Here are the specific steps to configure _app.js for Material UI:
- Install Material UI Core and Icons
- Modify the _app.js file to set up the Material UI theme
- Add the TamaguiProvider to the _app.tsx file
- Pass the appDir boolean to @tamagui/next-plugin
Sources
- https://purecode.ai/blogs/material-ui-nextjs/
- https://tamagui.dev/docs/guides/next-js
- https://blog.logrocket.com/getting-started-mui-next-js/
- https://staticmania.com/blog/guide-to-creating-a-darklight-mode-toggle-in-next-js
- https://webtech-note.com/posts/how-to-implement-light-dark-mode-css-vars-next-js
Featured Images: pexels.com