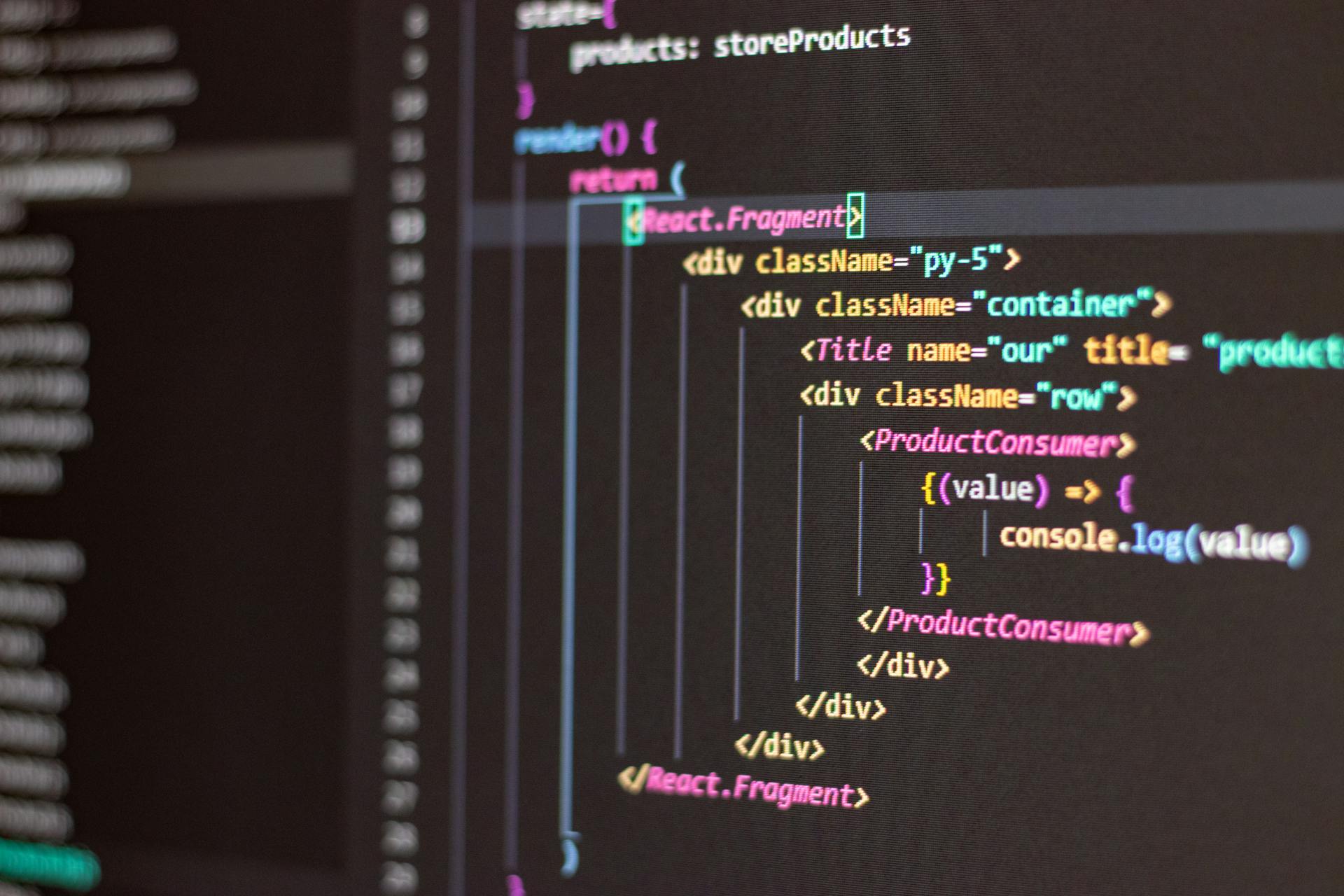
Building efficient apps with Next.js Edge Runtime and API interactions is a game-changer. By leveraging the power of Edge Runtime, developers can serve pages directly from the edge of the network, reducing latency and improving performance.
This approach is made possible by Next.js Edge Runtime's ability to run code at the edge, eliminating the need for server-side rendering. As explained in the article, Edge Runtime can handle complex logic and data fetching, making it an ideal solution for modern web applications.
With Next.js Edge Runtime, developers can also take advantage of API interactions, which enable seamless communication between the edge and the server. API interactions allow for real-time data exchange, making it possible to build highly responsive and dynamic applications.
If this caught your attention, see: Next Js Fetch Data save in Context and Next Route
Embracing Node.js
Next.js 14 has taken a significant leap forward in its server-side rendering capabilities, allowing developers to create dynamic web applications with impressive performance boosts. This is thanks to the introduction of hybrid pages that support both static generation and server-side rendering.
Broaden your view: Nextjs Server Rendering Tailwind
The streamlined SSR process in Next.js 14 reduces memory usage significantly, which leads to improved cold start times. This is especially beneficial for applications that scale to handle a large volume of requests.
Developers should now design functions that are succinct and single-purpose, allowing for rapid execution and easy debugging. This approach to server-side code enhances the reusability of components and promotes modularity.
The optimized server actions in Next.js 14 are strategically designed for efficiency, prioritizing memory optimization. This proves especially beneficial for applications that scale to handle a large volume of requests.
Optimizing Apps with Middleware
The Edge Runtime in Next.js 14 is a game-changer for web applications, but it's not the only tool in your optimization toolkit. Middleware in Next.js 14 is a powerful lever for optimizing applications, enabling developers to interject custom logic that can dramatically affect performance.
Middleware can be used to implement caching strategies, security protocols, and even geolocation-based content delivery, which can significantly reduce latency by serving localized resources. By serving localized resources, you can create a tailored content experience that's faster and more responsive to users.
If this caught your attention, see: Nextjs Middleware Firebase Auth
However, implementing middleware also introduces additional complexity, which can offset the performance gains. Developers must consider the geographic distribution of their user base and the impact of maintaining an ever-evolving set of location-specific content and rules.
To mitigate this complexity, developers can use efficient caching of authorization tokens and judicious use of the Next.js API routes. This can help maintain a balance between secure practices and swift response times.
Middleware can also be used for A/B testing, which involves intercepting requests and directing users to different versions of a page to test features or user experience paths. This practice provides valuable insights into user behavior and preferences, but it can also lead to increased development and testing overhead.
To keep middleware lean and focused, developers should implement performance metrics to monitor the impact of each middleware function. Techniques such as conditionally executing middleware based on route patterns or employing server-side caching can help maintain performance while leveraging the benefits middleware has to offer.
Additional reading: Nextjs Use Server
Code Migration and Compatibility
Migrating to Next.js 14 requires careful attention to code migration and compatibility challenges. The shift from familiar Node.js patterns to the new Edge runtime environment poses unique obstacles.
A common challenge is the exclusion of legacy Node.js APIs, which are not supported in the Edge context. This means developers must seek scalable alternatives for server-dependent functionalities.
Replacing filesystem operations with compatible services is crucial. Middleware must also be updated to align with Edge's design philosophy, which emphasizes lightweight and decomposed logic blocks for maximum efficiency.
Transforming standard middleware to Edge middleware involves breaking it down into isolated logic blocks. This refactoring may require moving from server-handled API endpoints to modular Edge Functions.
Careful planning is necessary to avoid disruptions during the migration process. Testing should not be an afterthought, as techniques like Unit testing and Integration testing can uncover non-obvious problems.
Automated test suites and tools like Lighthouse scores can help monitor performance and identify areas for improvement.
Discover more: Next Js vs Node Js
Data and API Interactions
Data and API Interactions are crucial in Next.js Edge Runtime. Employing Incremental Static Regeneration (ISR) for pages that require frequent updates without compromising on performance is an advanced pattern.
Caching strategies in the Edge Runtime are paramount for performance. Leveraging the built-in cache-control headers for static assets can vastly reduce needless data fetching.
API interactions with care is critical when leveraging the Edge Runtime, where running server-side tasks is not as straightforward as with a traditional Node.js server. Complex data fetching tasks now move closer to the client, employing Edge Functions.
Streaming responses allow for sending partial data to the client as it is fetched or processed, highly beneficial for handling large datasets or long-running operations.
Here are some best practices for integrating third-party services or APIs in the Edge Runtime:
- Choose lightweight and HTTP-focused libraries over traditional Node.js libraries.
- Encapsulate integrations within Edge Functions.
- Handle error scenarios and fallbacks to maintain reliability.
To add server-rendered data in your Next.js project using the App Router, you can edit a Next.js component to add a server-side operation to render data in the component. By default, Next.js components are Server Components that can be server-rendered.
Consider reading: How to Add Img to Nextjs
Here's an example of how to add server-rendered data:
- Open the app/page.tsx file and add an operation that sets the value of a server-side computed variable.
- Import unstable_noStore from next/cache and call it within the Home component to ensure the route is dynamically rendered.
- Update the Home component in app/pages.tsx to render the server-side data.
To create an API route, you can use Route Handlers provided by Next.js. You can fetch these APIs in Client Components.
Here's an example of how to create an API route:
- Create a new file at app/api/currentTime/route.tsx.
- Add a handler function to return data from the API.
- Create a new file at app/components/CurrentTimeFromAPI.tsx.
- Add a client component that fetches the API in this file.
The Client Component fetches the API with a useEffect React hook to render the component after the load is complete.
API Routes and Configuration
API routes in Next.js Edge Runtime are created using Route Handlers, which can be used to fetch APIs in Client Components. This is a powerful feature that allows you to create dynamic and interactive web applications.
To create an API route, you need to add a new file at `app/api/currentTime/route.tsx` and add a handler function to return data from the API. This function should return a JSON response using `NextResponse.json()`.
API routes can be configured to handle GET requests, and can be used to fetch data from third-party services or APIs. However, it's essential to choose lightweight and HTTP-focused libraries over traditional Node.js libraries that may rely on unsupported APIs or file system access.
Here's an interesting read: Nextjs Backend
Here are some key things to consider when creating API routes:
- Create a new file at `app/api/currentTime/route.tsx` for the Route Handler.
- Add a handler function to return data from the API using `NextResponse.json()`.
- Use a `useEffect` hook to fetch the API in Client Components.
- Use the `use client` directive to identify Client Components.
Adding an API Route
Adding an API Route is a crucial step in creating a robust and dynamic application. You can create API routes in Next.js using Route Handlers.
To add an API route, you'll need to create a new file at `app/api/currentTime/route.tsx`, which holds the Route Handler for the new API endpoint. This file should contain a handler function that returns data from the API.
Here's a step-by-step guide to creating an API route:
- Create a new file at `app/api/currentTime/route.tsx` and add a handler function to return data from the API.
- Use the `NextResponse` object to return a JSON response with the desired data.
- Make sure to set the `dynamic` property to `'force-dynamic'` to ensure that the API route is always fetched dynamically.
- Use the `GET` method to handle HTTP requests to the API route.
- Return a JSON response with the desired data, such as the current time.
Here's an example of what the handler function might look like:
```typescript
import { NextResponse } from 'next/server';
export const dynamic = 'force-dynamic';
export async function GET() {
const currentTime = new Date().toLocaleTimeString('en-US');
return NextResponse.json({
message: `Hello from the API! The current time is ${currentTime}.`
});
}
```
Once you've created the API route, you can fetch it in a Client Component using the `fetch` API or a library like Axios. Make sure to use the `useEffect` hook to render the component after the load is complete.
A unique perspective: Next Js Dynamic
Set Environment Variables
Setting environment variables is crucial for Next.js, especially when using Azure Static Web Apps. Next.js uses environment variables at build time and at request time to support both static page generation and dynamic page generation with server-side rendering.
To set environment variables, you need to do it within the build and deploy task, as well as in the Environment variables of your Azure Static Web Apps resource. This ensures that your application can access the variables it needs to function properly.
Next.js uses environment variables at both build time and request time, which is essential for static page generation and dynamic page generation with server-side rendering. This is a unique aspect of Next.js that sets it apart from other frameworks.
Suggestion: Nextjs Static Html
Frequently Asked Questions
Does Next JS middleware run on Edge?
Yes, Next JS middleware is unique in that it runs on the edge, which offers faster performance and improved security. This edge-based approach sets Next JS apart from other frameworks.
What is the difference between Vercel runtime edge and Node?
Vercel's Edge runtime is a lightweight JavaScript runtime that exposes a subset of Web Standard APIs, whereas Node.js is a full-fledged runtime that builds and bundles dependencies into a Serverless Function. The key difference lies in their scope and functionality, with Edge being more limited but optimized for edge computing.
How do I check my edge runtime?
To check if your function is running on the Edge runtime, look for the globalThis.EdgeRuntime property. This property can help you validate your function's runtime in tests or use different APIs as needed.
Sources
- https://nextjs.org/docs/pages/api-reference/edge
- https://www.nextjs.cn/docs/api-reference/edge-runtime
- https://nextjs-ja-translation-docs.vercel.app/docs/api-reference/edge-runtime
- https://borstch.com/blog/development/exploring-edge-and-nodejs-runtimes-in-nextjs-14
- https://learn.microsoft.com/en-us/azure/static-web-apps/deploy-nextjs-hybrid
Featured Images: pexels.com