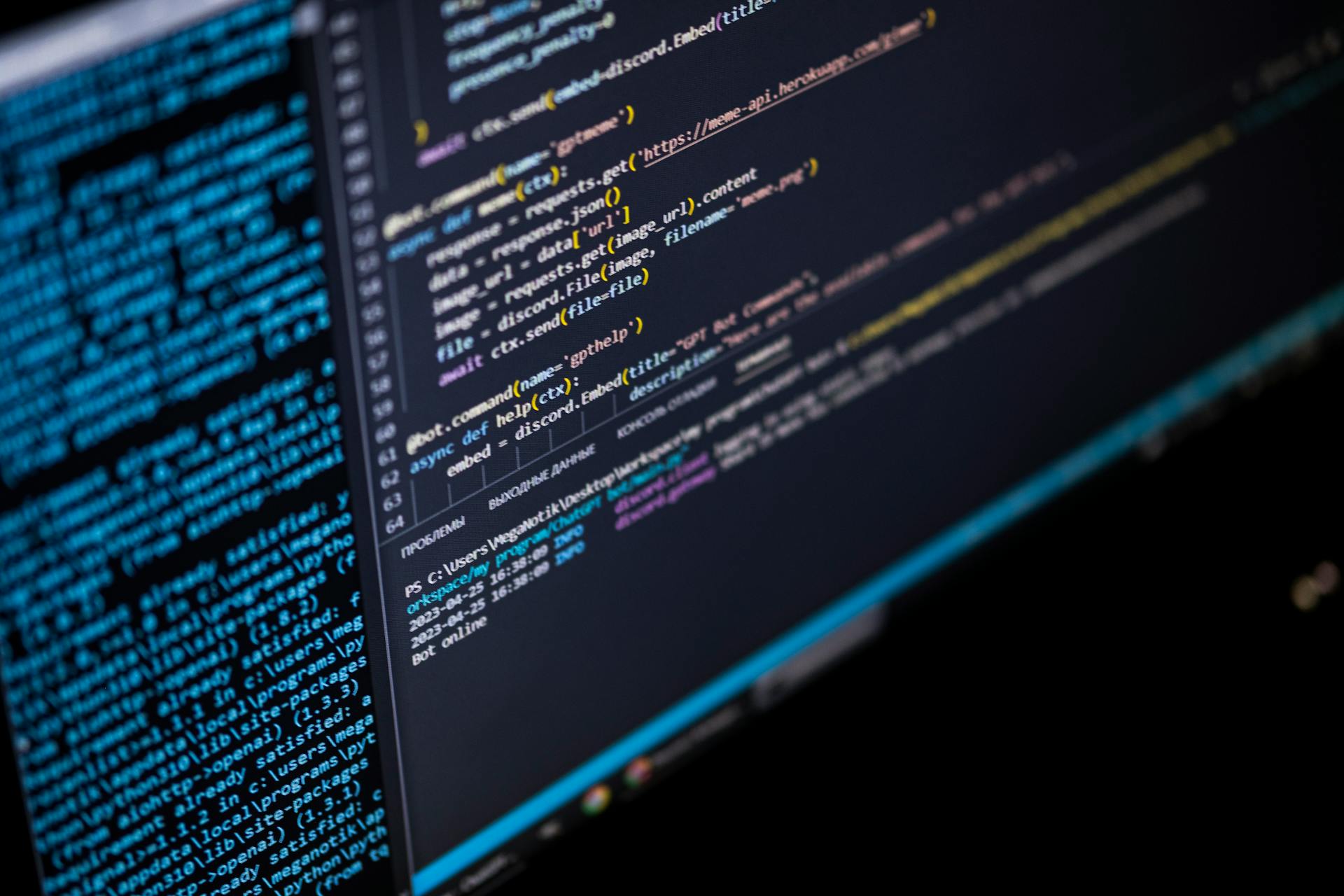
Setting up a Next.js project with Prettier, ESLint, and Tailwind CSS is a straightforward process that can be completed in a few steps.
First, you'll need to create a new Next.js project using the command `npx create-next-app my-app`. This will set up a basic Next.js project with all the necessary files and folders.
Next, you'll need to install the required dependencies, including Prettier, ESLint, and Tailwind CSS, using the command `npm install prettier eslint @eslint/eslintrc tailwindcss`.
With these dependencies installed, you can configure Prettier to format your code automatically.
On a similar theme: Next Js Using State Context
Setting Up ESLint
To set up ESLint, start by installing it, along with Prettier and some useful plugins, to get them working together seamlessly. This will help you maintain clean and consistent code quality in your Next.js project.
Next.js has built-in ESLint support, so you can initialize ESLint with a single command. This command creates a .eslintrc.json file in the root of your project, which includes some default rules.
Expand your knowledge: Nextjs Eslint 9
The .eslintrc.json file is where you'll configure ESLint to integrate with Prettier. Update it as follows:
- `extends: ["next", "next/core-web-vitals"]` uses the default ESLint config provided by Next.js and helps to lint for the Web Vitals metrics.
- `plugins: ["prettier"]` enables Prettier as an ESLint rule and displays formatting issues as ESLint errors.
Here's a summary of the plugins you'll need to install:
- eslint: Lints your JavaScript/TypeScript code.
- prettier: Automatically formats your code.
- eslint-config-prettier: Disables ESLint rules that would conflict with Prettier.
- eslint-plugin-prettier: Integrates Prettier into ESLint so that Prettier errors show up as ESLint errors.
Configure ESLint
Configure ESLint by running the following command in your Next.js project: `npx eslint --init`. This creates a `.eslintrc.json` file in the root of your project, which includes some default rules.
The default ESLint config provided by Next.js is used by default. If you want to use a custom config, you can specify it in the `extends` field.
Here's an example of how to update the `.eslintrc.json` file to integrate Prettier:
- `extends`: `next`
- `extends`: `next/core-web-vitals`
- `plugins`: `prettier`
- `rules`: `prettier/enabled`: `true`
This setup does the following:
Linting and Formatting
Linting and formatting are crucial steps in maintaining clean and consistent code quality. Next.js provides built-in ESLint support, so you can initialize ESLint with a single command. This command creates a .eslintrc.json file in the root of your project, which includes some default rules.
To integrate Prettier with ESLint, you can update the .eslintrc.json file to include the following configuration:
- next: Uses the default ESLint config provided by Next.js.
- next/core-web-vitals: Helps to lint for the Web Vitals metrics.
- prettier: Enables Prettier as an ESLint rule and displays formatting issues as ESLint errors.
This setup does the following:
- Uses the default ESLint config provided by Next.js.
- Helps to lint for the Web Vitals metrics.
- Enables Prettier as an ESLint rule and displays formatting issues as ESLint errors.
By following these steps, you can ensure that your code is both linted and formatted correctly, making it easier to maintain and collaborate on your project.
Running ESLint in VS Code
If you're using Visual Studio Code, you can enable automatic linting by installing the ESLint and Prettier VS Code extensions.
Installing these extensions is a great idea, especially if you don't want to format your file manually every time.
To run ESLint in VS Code, you'll need to modify your workspace settings. Open your workspace settings (.vscode/settings.json) and add the following:
- Open your workspace settings (.vscode/settings.json)
- Add the configuration to run ESLint and Prettier every time you save a file
This configuration will run ESLint every time you save a file, making your code cleaner and more maintainable.
Linting and Formatting
Linting and formatting are essential tools for maintaining clean and consistent code quality. You can integrate ESLint and Prettier with Next.js to achieve this.
Next.js has built-in ESLint support, so you can initialize ESLint with `npx eslint --init`. This command creates a `.eslintrc.json` file in the root of your project, which includes some default rules.
To integrate Prettier with ESLint, update the `.eslintrc.json` file as follows: `extends: ['next', 'next/core-web-vitals', 'prettier']`. This setup enables Prettier as an ESLint rule and displays formatting issues as ESLint errors.
You might like: Nextjs Server Actions File Upload
You can also install ESLint, Prettier, and some useful plugins to get them working together. The plugins include `eslint-config-prettier` to disable ESLint rules that conflict with Prettier and `eslint-plugin-prettier` to integrate Prettier into ESLint.
Here are some essential plugins to install:
- eslint: Lints your JavaScript/TypeScript code.
- prettier: Automatically formats your code.
- eslint-config-prettier: Disables ESLint rules that would conflict with Prettier.
- eslint-plugin-prettier: Integrates Prettier into ESLint so that Prettier errors show up as ESLint errors.
To run ESLint and Prettier automatically, you can install the ESLint and Prettier VS Code extensions. Then, modify your workspace settings to run ESLint and Prettier every time you save a file.
Prettier is also a great tool for formatting code, and it's 100% compatible with TypeScript Next JS apps. You can configure Prettier to follow your styling preferences, and it will take care of the rest by indenting your code, adding space or semi-colons, and replacing single quotes with double quotes (or vice-versa).
Related reading: Nextjs Code Block
Tailwind CSS Styling
To get started with Tailwind CSS, you need to install the required packages: tailwindcss, postcss, and autoprefixer.
You'll then run a command to initialise the Tailwind project, creating the tailwind.config.js and postcss.config.js files.
See what others are reading: Next Js Tailwind Spinner Loading Page
A new tailwind.config.js file has been created, and you'll update it to remove unused styles using the purge property.
The jit compiler is enabled to improve performance.
You'll apply Tailwind styles to the project by overwriting the globals.css file inside the styles folder.
Remove all other CSS files since you won't need them if you use Tailwind CSS.
Make sure the globals.css file is included in the _app.tsx file inside pages.
You can now start working on your next amazing project, using a battle-tested and production-ready setup.
Related reading: Apply Css Nextjs
Sources
- https://staticmania.com/blog/how-to-integrate-eslint-and-prettier-with-next-js-for-code-quality
- https://www.sandromaglione.com/articles/create-nextjs-project-with-typescript-eslint-prettier-tailwindcss
- https://creativedesignsguru.com/how-to-set-up-eslint-and-prettier-with-next-js-typescript/
- https://www.jakeprins.com/blog/how-to-use-eslint-and-prettier-in-your-next-js-application-with-typescript
- https://stackoverflow.com/questions/62060275/next-js-with-prettier-formatting
Featured Images: pexels.com