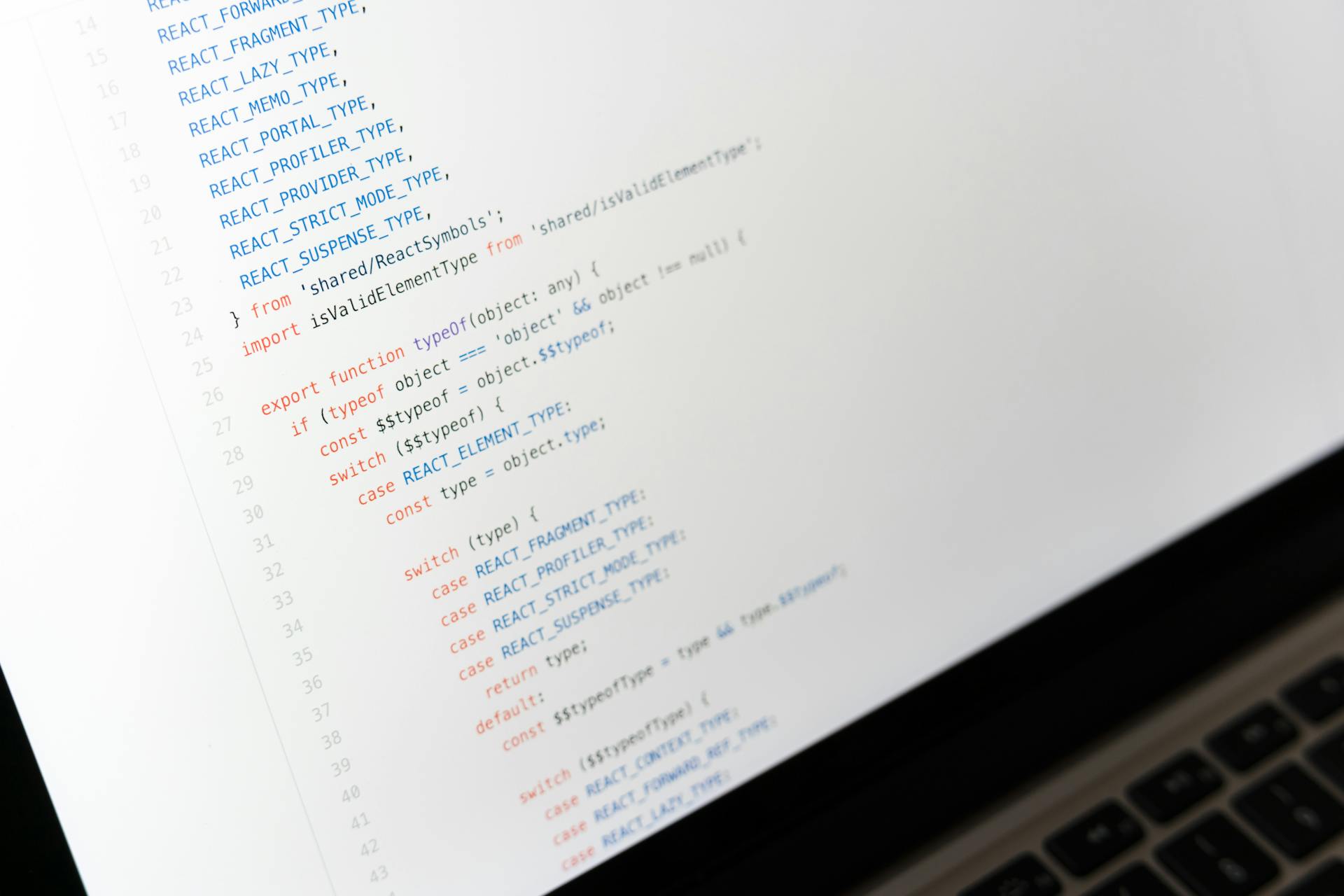
Integrating ESLint 9 with Next.js is a great way to improve code quality and catch errors early on. ESLint 9 offers several new features and improvements that can help you write cleaner, more maintainable code.
One of the key benefits of ESLint 9 is its ability to detect and report on more types of errors, including those related to asynchronous code. This is especially useful in Next.js applications, where asynchronous code is often used to handle API calls and other complex tasks.
By integrating ESLint 9 with Next.js, you can ensure that your code is free of common errors and follows best practices for coding style and security. This can save you time and headaches in the long run, and help you deliver high-quality applications to your users.
Getting Started
To get started with Next.js and ESLint 9, you'll need to install and configure ESLint. You can do this by running a single command in your terminal.
The command to install and configure ESLint is straightforward: you can use the ESLint command with the --init option to set up a basic configuration.
After installing ESLint, you can specify a shareable config hosted on npm using the --config option and the package name.
Once you have ESLint set up, you can run it on any file or directory by using the ESLint command with the path to the file or directory.
Configuration
If you're coming from a version of Next.js before 9.0.0, you'll need to see the migration guide. After running npm init @eslint/config, you'll have an eslint.config.js (or eslint.config.mjs) file in your directory. This file will contain some default rules configured like this: "no-unused-vars": "warn", "no-undef": "warn".
The error level of a rule can be one of three values: "off" or 0 to turn the rule off, "warn" or 1 to turn the rule on as a warning, or "error" or 2 to turn the rule on as an error.
The recommended configuration, like this: "js.configs.recommended", contains configuration to ensure that all of the rules marked as recommended will be turned on. You can also use configurations created by others by searching for "eslint-config" on npmjs.com.
Here's a breakdown of the error levels:
Your eslint.config.js configuration file will also include a recommended configuration, which contains configuration to ensure that all of the rules marked as recommended will be turned on.
Prettier Setup
To set up Prettier, create a Prettier configuration file (.prettierrc) to specify your preferred formatting rules. You can adjust these settings to match your style guide.
Add scripts to your package.json file to make running Prettier checks easier. This will simplify the process of formatting your code.
To integrate Prettier with ESLint, modify the .eslintrc.json file. Update it as follows:
- next: Uses the default ESLint config provided by Next.js.
- next/core-web-vitals: Helps to lint for the Web Vitals metrics.
- prettier: Enables Prettier as an ESLint rule and displays formatting issues as ESLint errors.
This setup does the following: it enables Prettier as an ESLint rule, displays formatting issues as ESLint errors, and integrates Prettier with the default ESLint config provided by Next.js.
Integration and Support
Next.js ESLint 9 integrates well with TypeScript, a popular language for large and complex applications. You can enable TypeScript support by running Create-next-app with the --typescript flag.
To get started, you'll need to add some specific codes to your .eslintrc.json file. This will apply Next.js ESLint rules along with TypeScript-specific lint rules from next/typescript.
Support for TypeScript
Support for TypeScript is a breeze with Next.js, thanks to the create-next-app command. You can enable TypeScript support by running create-next-app --typescript, which will automatically apply Next.js ESLint rules and TypeScript-specific lint rules.
To add TypeScript support manually, you can modify your .eslintrc.json file to include the necessary codes.
Running in VS Code
Running in VS Code can be a game-changer for developers. Installing the ESLint and Prettier VS Code extensions is a great first step.
If you're using Visual Studio Code, you can enable automatic linting and formatting on save by installing these extensions. This will help keep your code clean and consistent.
To take it to the next level, you'll need to modify your workspace settings. Open your workspace settings (.vscode/settings.json) and add the following configuration:
- ESLint and Prettier will run every time you save a file.
This configuration is what makes the magic happen, allowing you to focus on writing code rather than worrying about formatting and linting.
Lint
ESLint is a must-have in all JavaScript projects, and it's become a real crutch for developers.
A linter has several purposes, including standardizing code and detecting syntax errors. It can catch errors like wrong variable declarations and import errors.
ESLint is particularly useful in React environments, where it's often used in conjunction with other plugins.
In a React environment, you can install ESLint with main plugins used in the project. You'll also need to install dedicated packages if you're using Typescript.
To configure ESLint, you'll need to create an .eslintrc.json file at the root of your project. This file will contain declarations for your plugins, as well as overrides for specific file types.
The overrides key in the .eslintrc.json file allows you to define an additional configuration for certain file types, such as Typescript files.
You'll also need to create an .eslintignore file at the root of your project to specify which files to ignore, such as those from node_modules.
Sources
- https://staticmania.com/blog/how-to-integrate-eslint-and-prettier-with-next-js-for-code-quality
- https://soykje.gitlab.io/en/blog/nextjs-typescript-eslint
- https://eslint.org/docs/latest/use/getting-started
- https://dev.to/celest67/nextjs-with-eslint-3gl5
- https://blog.linotte.dev/eslint-9-next-js-935c2b6d0371
Featured Images: pexels.com