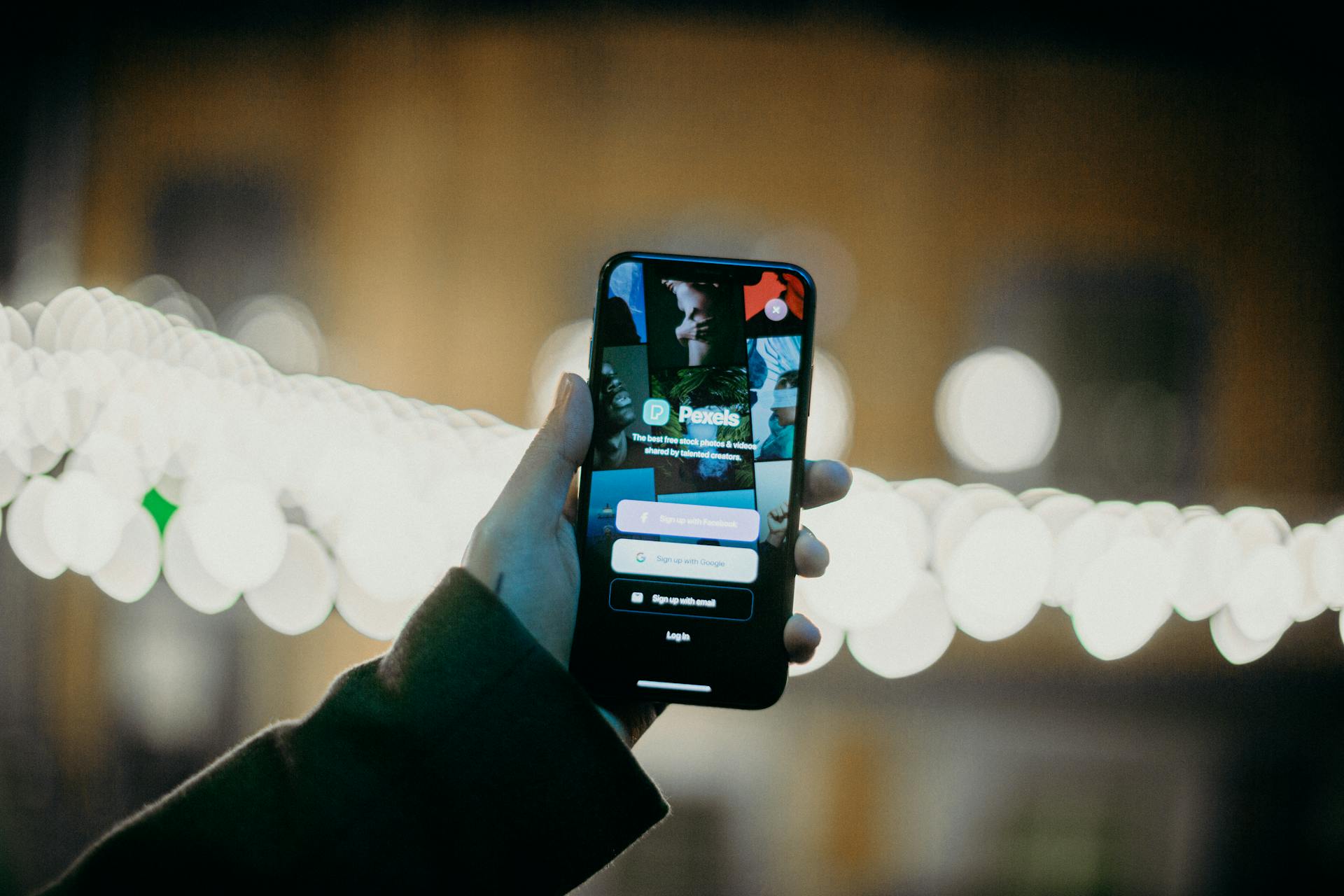
Next.js App Router API is a powerful tool that allows you to create fast and scalable web applications. It's designed to work seamlessly with Next.js, making it easy to build robust and efficient APIs.
The App Router API is built on top of the V2 API, which provides a robust and flexible way to handle API requests. This allows developers to create custom API routes and endpoints with ease.
Next.js App Router API supports both GET and POST requests, making it suitable for a wide range of use cases. This flexibility is a major advantage over traditional API frameworks.
Setting Up Next.js App Router API
To set up a Next.js App Router API, start by navigating to the pages/api directory in your project. If it doesn't exist, create it.
Create a new file in the pages/api directory with a name that matches the desired API route, such as users.js for a route handling user data. This file will contain the code for your API endpoint.
In this file, export a default function that will handle the route. This function takes two parameters: req (the incoming request object) and res (the response object).
Setting Up
To set up your Next.js App Router API, start by navigating to the pages/api directory in your project. If this directory doesn't exist, create it. Next, create a new file in this directory with a name that matches the desired API route, such as users.js for handling user data.
The file name will become the route path, so choose a name that accurately reflects the purpose of the API. For example, if you're creating a route to handle user data, the file name should be users.js.
In the file, export a default function that will handle the route. This function takes two parameters: req (the incoming request object) and res (the response object). The function should handle the route and send a response accordingly.
App Examples with TypeScript
Next.js 14's App Router is a game-changer for building robust applications.
To create GET & POST route handlers with ease, you can start by learning the basics of the App Router. Mastering Next.js 14's App Router will give you the skills to create efficient and scalable applications.
You can ensure type safety when typing API Routes with TypeScript by defining types for request and response objects. This is crucial for building robust applications.
TypeScript is a powerful tool that allows you to define types for request and response data. By doing so, you can catch errors early and improve your development workflow.
Whether you're fetching data from an external API with GET requests or handling form submissions with POST requests, understanding the App Router is essential.
Creating and Configuring Handlers
To create API routes in Next.js, you'll need to define them in the pages/api directory, with each file corresponding to a specific route.
When defining route handlers, you'll need to check the req.method property and respond accordingly. This involves checking if the incoming request is a GET, POST, or other type of request, and sending the appropriate response.
To handle GET requests, you can use the req.json() method to parse the JSON data from the request body. This is particularly useful when working with APIs that send JSON data.
Dynamic API routes can be created by using square brackets ([]) in the file name to define a parameter. For example, pages/api/users/[id].js would match requests to /api/users/1, /api/users/2, and so on.
To create a dynamic API route, you can use the following structure:
- Location: API routes reside in a dedicated folder named pages/api.
- File Naming: The file name determines the URL path for accessing the API route.
- Function Structure: Each API route file should export a default function that takes two arguments: req and res.
Here's an example of a simple GET handler that returns a plain text response:
This handler listens for GET requests to the /api/hello endpoint and responds with a plain text message.
Route handlers can be accessed from your frontend code using techniques like fetch or libraries like Axios. Here's an example using fetch:
Output:
Handling Requests and Responses
Handling requests and responses is a crucial aspect of building a robust Next.js API. To handle GET requests, you need to check the `req.method` property and respond accordingly.
You can use the `req.method` property to check if the incoming request is a GET request, and if it is, send a JSON response with a 200 status code. If the request method is not GET, you can set the `Allow` header to indicate that only GET requests are allowed, and return a 405 (Method Not Allowed) status code.
To handle POST requests, you need to access and process data from the request body. This can be done by accessing the `req.body` property, which contains the data sent in the request body.
Here are some ways to handle requests and responses in Next.js API:
- Handle GET requests by checking the `req.method` property and responding accordingly.
- Handle POST requests by accessing and processing data from the request body.
- Use request validation to ensure that incoming requests meet the required format, data types, and constraints.
- Use error handling to ensure that your API endpoints can handle unexpected errors and provide clear error responses.
Dynamic
In Next.js, dynamic API routes allow you to create flexible API endpoints that can handle different request parameters.
You can define a dynamic route file by creating a JavaScript file with square brackets `[]` in the filename inside the `pages/api` directory. For example, `pages/api/users/[id].js` will create a dynamic API route that accepts `id` as a parameter.
To access dynamic parameters, you can use `req.query` or `req.params` depending on the route structure. This is useful for building APIs that require variable data, such as fetching specific resources based on IDs or filtering data based on query parameters.
Here's a step-by-step guide to creating a dynamic API route in Next.js:
- Define a dynamic route file with square brackets `[]` in the filename.
- Access dynamic parameters using `req.query` or `req.params`.
- Handle dynamic data using the parameter received in the request.
For example, visiting `/api/users/123` in your Next.js application will trigger a dynamic API route that responds with a JSON object containing the user data for ID 123.
Using Query and Form Data
Using query parameters is a great way to customize API responses in Next.js. You can access query parameters using the req.query object and use them to include specific data in your JSON responses.
In a GET request handler, you can extract query parameters from the URL and include them in the response. For example, if you access the API route /api/users?id=123, the response would include the id query parameter.
Fetching data from external APIs is another powerful use of GET handlers. You can extract query parameters, fetch data from an external API, and return it as a JSON response.
To validate and process form data in POST requests, you can check for the presence of required fields in the request body. If any field is missing, you can respond with an error message. This is crucial for handling form data securely and effectively.
Using Query Parameters
Using Query Parameters is a great way to customize your API responses. You can access query parameters using the req.query object.
In Next.js, you can automatically parse query parameters from the URL. This makes them available in the req.query object, which you can access in your GET request handlers. For example, if you access an API route with a query parameter like /api/users?id=123, the response would be a JSON object with the id included.
You can extract query parameters from the req.query object and include them in your JSON response. This is useful for customizing your API responses based on user input.
Form Data Validation and Processing
Form data validation is crucial when handling form data in POST requests. This is because it ensures that the data received is in the correct format and meets the necessary requirements.
Validation involves checking for the presence of required fields, such as name and email in the request body. If any field is missing, it responds with an error message.
You can use middleware functions to validate user input data, which helps prevent malicious data from being injected into your API. This is an essential step in maintaining the security of your application.
Request validation is also important, as it ensures that incoming requests meet the required format, data types, and constraints. You can use validation libraries like `joi`, `express-validator`, or write custom validation logic as per your application's needs.
Handling HTTP Methods
Handling HTTP Methods is a crucial part of building robust API endpoints in Next.js. To handle GET requests, you need to check the req.method property and respond accordingly, like in the example where the handler function sends a JSON response with a 200 status code if the request is a GET.
You can also check the request method to determine the correct response. For instance, if the request method is not GET, it sets the Allow header to indicate that only GET requests are allowed, and returns a 405 (Method Not Allowed) status code. This is a common pattern in API design.
To handle POST requests, you need to access and process data from the request body. This can be done by using req.json() to parse the JSON data from the request body, as shown in the example. You can then access and process the data as needed.
Handling Post Requests
Handling POST requests in Next.js API routes involves accessing and processing data from the request body. This requires checking the req.method property and responding accordingly.
To handle POST requests, you need to check the req.method property and respond accordingly. You can then use req.json() to parse the JSON data from the request body.
You can create a POST handler to handle form submissions or other POST requests by listening for POST requests to a specific endpoint, such as /api/submit. This handler reads the JSON body from the request and responds with the received data.
Handling and parsing JSON body data is a common task when dealing with POST requests. The request.json() method is used to parse the JSON data from the request body, and you can then log it to the console or respond with a success message.
Frequently Asked Questions
Is Next.js 13 ready for production?
Next.js 13 is now production-ready with the release of 13.4, allowing for incremental adoption of the App Router. Start exploring the future of Next.js today.
Sources
- https://nextjsstarter.com/blog/nextjs-api-routes-get-and-post-request-examples/
- https://nextjs.org/docs/pages/building-your-application/routing/api-routes
- https://www.geeksforgeeks.org/how-to-use-next-js-api-routes/
- https://blog.arcjet.com/testing-next-js-app-router-api-routes/
- https://www.wisp.blog/blog/nextjs-14-app-router-get-and-post-handler-examples
Featured Images: pexels.com