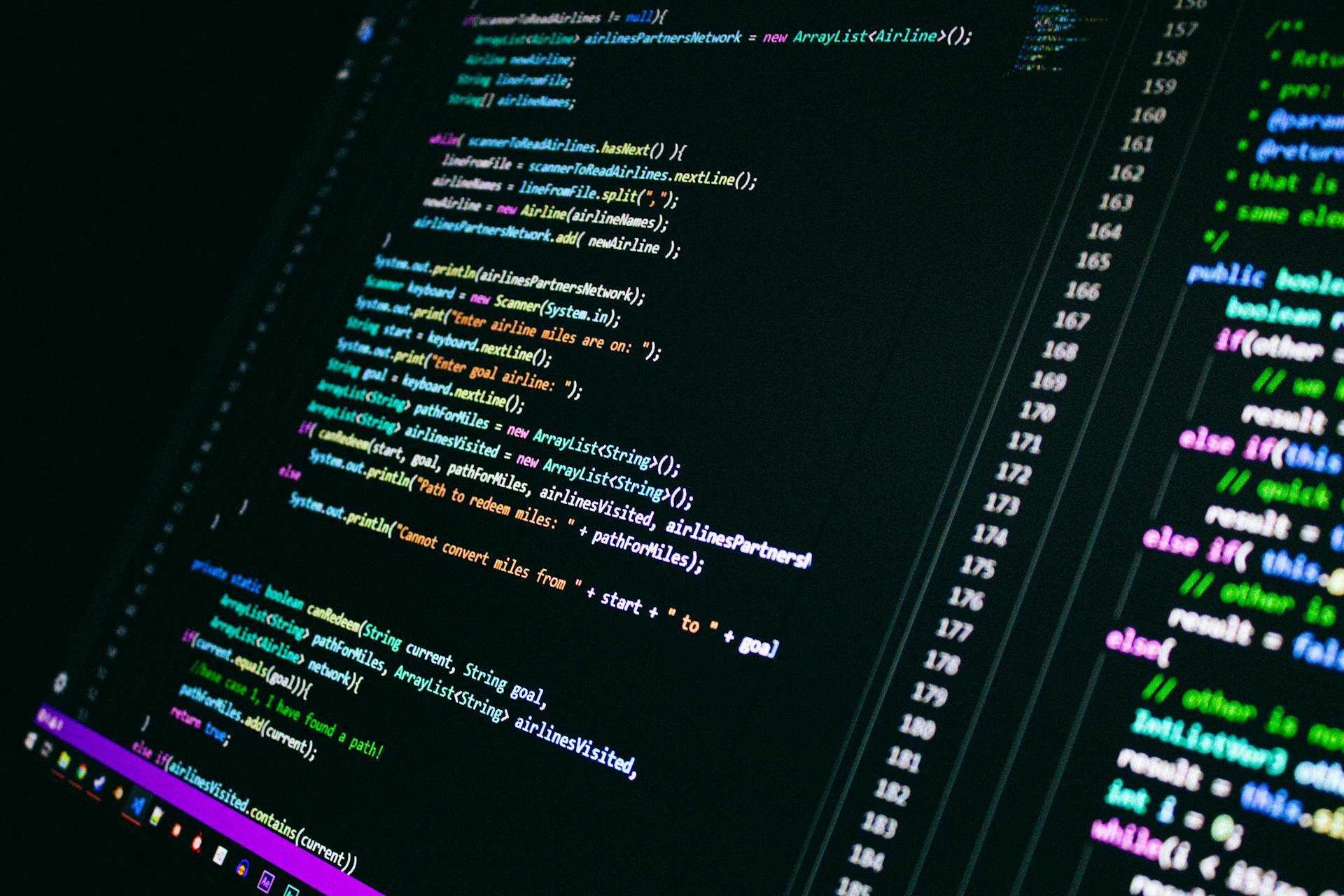
To set up the Next.js Context API, you'll want to create a new file in your project's directory, specifically for your context. This file will serve as the central hub for your app's state management.
In the Next.js Context API setup and implementation on GitHub, you can see an example of how to create a context file. The file is named `apiContext.js` and it imports the createContext function from the context module.
A context file typically exports a single value, which is an object that contains the state and functions for managing that state. This object is then passed down to components in your app through the use of the useContext hook.
In the GitHub example, the context file exports an object with a single property, `apiState`, which is an object that contains the current state of the API.
State Management in Next.js 13
State Management in Next.js 13 is made possible using the React Context API. This allows you to manage state across your application in a centralized way.
You can build a basic to-do app that utilizes React Context API for state management. This is a great starting point for learning state management in Next.js 13.
API Setup
To set up an API in Next.js, you'll need to create a new file in the `pages/api` directory. This is where all API routes live.
The `pages/api` directory is a special place in Next.js where API routes are stored, and it's where you'll find the `index.js` file that serves as the default API route.
In Next.js, API routes are functions that return data, and they're typically defined as `async` functions. For example, the `index.js` file might look something like this: `export async function handler(req, res) { return res.json({ message: 'Hello from Next.js!' }); }`
You can also use the `getServerSideProps` method to fetch data from an API and pass it to the page. This method is called on the server, so it's a great way to fetch data that's specific to the user.
The `getServerSideProps` method returns an object with a `props` property that contains the data fetched from the API. This data is then passed to the page as props.
To set up authentication for your API, you can use a library like Next-Auth. This library provides a simple way to authenticate users and protect your API routes.
Next-Auth integrates seamlessly with Next.js, and it provides a range of features that make it easy to manage authentication.
API Implementation
API Implementation is a crucial step in building a Next.js app. It involves creating a custom API using the Next.js Context API.
The Next.js Context API provides a simple way to manage global state. It's a built-in feature that allows you to share data between pages.
To implement a custom API, you need to create a new file in the `pages/api` directory. This is where Next.js looks for API routes by default.
In this file, you can use the `getServerSideProps` method to fetch data from an external API or database. This method is executed on the server, allowing you to handle requests and return data.
For example, if you have a file called `data.js` in the `pages/api` directory, you can use `getServerSideProps` to fetch data from it. This file can contain a simple API endpoint that returns data in JSON format.
Next.js also provides a built-in API route for handling HTTP requests. You can use the `fetch` method to make requests to external APIs or services.
In the example code, a GET request is made to the `https://jsonplaceholder.typicode.com/posts` endpoint. This endpoint returns a list of posts in JSON format, which is then used to populate the page.
By using the Next.js Context API and implementing a custom API, you can create a robust and scalable app that integrates with external services and databases.
Result and Next.js API Routes
Using Next.js API routes can be tricky, especially when it comes to handling user authentication tokens. API routes run in a server-side context, which means the token the server is expecting isn't what the client is supplying.
This tiny disparity is always present unless you "hard refresh". The execution context of API routes is server-side, which can be easy to forget.
To bypass this issue, the author used cookies-next to directly pass the cookie value to the HTTP request. This approach worked like a charm.
The problem with using nookies to export the token as a constant is that it provides a client-side method of accessing browser cookies, which is wrong in the context of API routes.
Sources
- https://www.makeuseof.com/react-context-nextjs-13-app-directory-state-management/
- https://episyche.com/blog/how-to-use-context-api-in-a-nextjs-app
- https://meje.dev/blog/persisting-auth-state-in-nextjs-context-and-cookies
- https://dev.to/shareef/context-api-with-typescript-and-next-js-2m25
- https://nextjs.org/docs/pages/api-reference/functions/get-static-props
Featured Images: pexels.com