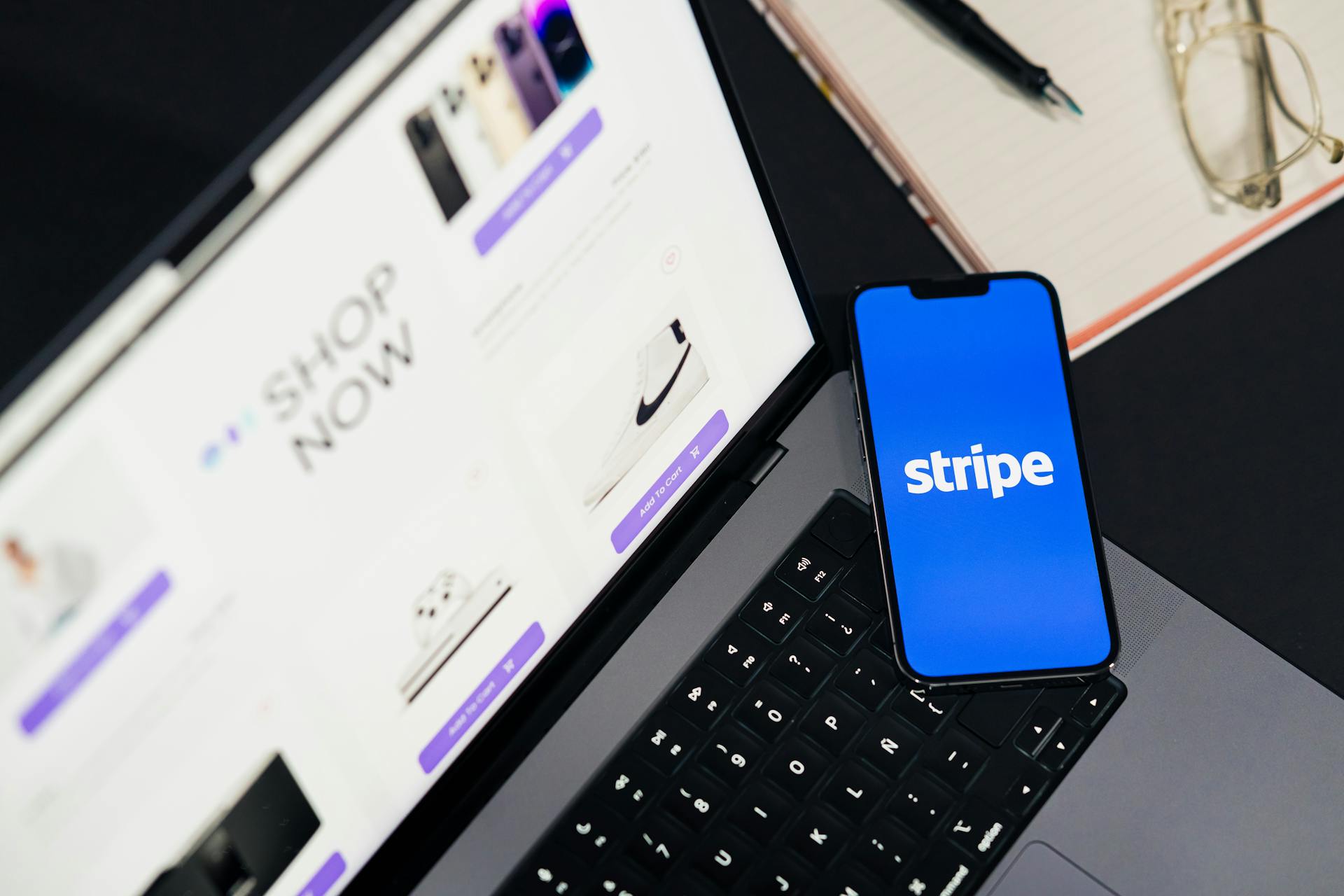
Adding Stripe to your Next.js app is a great way to accept payments online.
To get started, you'll need to install the Stripe library using npm or yarn.
First, create a Stripe account and obtain your API keys. You can find these in your Stripe dashboard under Developers > API keys.
Next, create a Stripe secret key and a Stripe publishable key, which you'll use to authenticate API requests.
Setup and Configuration
To get started with adding Stripe to your Next.js app, you'll need to configure your Stripe API keys. Create a .env.local file in your project's root directory and add your API keys, including the publishable and secret keys from your Stripe dashboard.
First, install the required Stripe packages by running the command in your Next.js project: "Install Stripe Dependencies". This will provide the necessary tools to integrate Stripe Checkout into your Next.js application.
To process the checkout, you'll need to install the @stripe/stripe-js library using NPM. This library is required to create a checkout session.
Expand your knowledge: Nextjs Icons Library
To create a Stripe checkout session, you'll need to initialize a new Stripe instance using the secret API key. You can do this by creating a checkout session object to the handler function and passing the items.
Here are the required parameters for the checkout session object:
- payment_method_types: The payment method types the checkout session accepts.
- line_items: A list of items the user is purchasing.
- mode: The mode of the Checkout Session. If the checkout items include at least one recurring item, pass “subscription”.
- success_url: The URL Stripe will redirect users if the payment is successful
- cancel_url: The URL Stripe will redirect users if they cancel the payment.
Before you can create a checkout session, you'll need to sign up and create products in the Stripe dashboard. This includes setting up your Stripe dashboard and creating a product.
Payment Integration
Stripe is a payment processing platform that allows you to add a pre-built checkout page to a website and accept and manage online payments.
To integrate Stripe into your Next.js application, you'll need to create a Stripe account and save the secret key into the .env.local file, which authenticates and enables you to access Stripe from the application.
You can create a product on Stripe by selecting Products from the top menu and clicking the Add Product button to create a new product, providing the product name, price, description, and payment option, and selecting one-time as the payment option.
Here's an interesting read: Next Js Font
To add Stripe checkout to your Next.js application, you can install the Stripe Node.js library and create an API endpoint - api/payment within the Next.js application.
The checkout-session.js file should use a try/catch to redirect users when an error occurs during checkout.
You can create a checkout session for a product and return the session URL, which is the link where payments for a product are collected, and you need to redirect users to this URL.
To redirect users to the checkout page, you'll need to create a function within the index.tsx file that retrieves the session URL from the API endpoint.
Stripe supports a variety of payment methods, including credit and debit cards, Apple Pay, and Google Pay.
A fresh viewpoint: Next Js Get Url
Components and Integration
To create a checkout component, you'll need to install the required Stripe packages by running `npm install stripe @stripe/stripe-js` in your Next.js project. This will provide the necessary tools to integrate Stripe Checkout.
Create a new file in your /components directory named /components/checkout.js and call the loadstripe function from the @stripe/stripe-js library, passing the publishable key as an argument. This will return a promise that resolves with a newly created Stripe object once Stripe.js has loaded.
Integrate the checkout component into your Next.js application by calling the checkout component on the cart page and adding a checkout button that triggers the handleCheckout function when clicked. This will initiate the Stripe Checkout process.
On a similar theme: Next Js Components Folder
Setting Up Webhook
To set up a webhook, you'll need to create a new API route in your Next.js application. Create a new file pages/api/webhooks.js and add the code that sets up a webhook handler.
The code listens for the checkout.session.completed event, which is triggered when a payment is successful. You can add more cases to handle additional event types as needed.
To receive webhook events, you need to add the webhook secret to your .env.local file. This involves copying the webhook signing secret from your Stripe dashboard and pasting it into your .env.local file.
Additional reading: Can Amazon S3 Take in Nextjs File
You can test the webhook locally using tools like ngrok, which exposes your local server to the internet. Once you have ngrok installed, run the command ngrok http 3000 to get a public URL that forwards to your local server on port 3000.
Update your webhook endpoint in the Stripe dashboard to point to this URL, followed by /api/webhooks. This allows Stripe to send requests to your webhook handler when events are triggered.
With the webhook component set up, your Next.js application is now equipped to handle Stripe events and further enhance your project's functionality.
Explore further: Save Api Data on Local Storage Next Js
Create a Component
To create a component, start by creating a new file in the /components directory, such as components/CheckoutButton.js. This file will contain the code for the CheckoutButton component.
The code initializes the Stripe instance using the publishable key and defines a handleCheckout function, which fetches the checkout session from the API and redirects the user to the Stripe Checkout page. The handleCheckout function is triggered when the checkout button is clicked.
Expand your knowledge: Next Js Function Handlers to Filter Data
A similar approach is taken when creating a checkout component in the /components directory, by calling the loadstripe function from the @stripe/stripe-js library, passing the publishable key as an argument. This returns a promise that resolves with a newly created Stripe object once Stripe.js has loaded.
In the checkout component, a function is added to create a checkout session using Axios to call the API in the /api folder to retrieve the checkout session. The checkout button is then added in the return statement, triggering the handleCheckout function when clicked.
A fresh viewpoint: Nextjs Ui Library
Integrate the Component
To integrate the Checkout component into your Next.js application, create a new file called CheckoutButton.js and add the code that initializes the Stripe instance using the publishable key and defines a handleCheckout function. This function fetches the checkout session from the API and redirects the user to the Stripe Checkout page.
The handleCheckout function is a crucial part of the Checkout component, as it allows users to complete the payment process and redirects them to the Stripe Checkout page.
See what others are reading: Nextjs Pathname Server Component
Next, integrate the CheckoutButton component into pages/index.js, which will render the Checkout component on the page. This is where the magic happens, and users can start the payment process.
After integrating the Checkout component, you'll need to create success and error pages to handle the user experience after the Stripe Checkout process. These pages will provide a seamless experience for users, regardless of the outcome of their payment attempt.
Here's an interesting read: Next Js Pages vs App
Creating a Session
Creating a Session is a crucial step in integrating Stripe with your application. This involves creating a checkout session object that describes the item the customer is purchasing.
To create a checkout session, you need to define the checkout parameters. These include payment_method_types, which specify the types of payment methods the checkout session accepts, such as card. You also need to include metadata, which is a set of key-value pairs that can be attached to an object, and in this case, we add the image of the item to be displayed on the Stripe Checkout Page.
Broaden your view: Store Login Session Nextjs
A list of items the customer is purchasing is also required, which in our case contains only one item. Additionally, you need to specify the success_url and cancel_url, which are the URLs to which the customer will be redirected after the payment is successful or when they cancel the payment.
Here's a list of the required checkout parameters:
- payment_method_types: A list of the types of payment methods (e.g. card) this Checkout Session is allowed to accept.
- metadata: Set of key-value pairs that you can attach to an object. Here we have added the image of the item which will be displayed on the Stripe Checkout Page.
- line_items: A list of items the customer is purchasing. In our case, the list contains only one item.
- success_url: The url/page to which the customer will be redirected after the payment is successful.
- cancel_url: The url/page to which the customer will be redirected when they cancel the payment.
Once you have defined the checkout parameters, you can create the checkout session and return its unique id. This id will be used to initiate the Stripe Checkout Session.
A unique perspective: Nextjs Session
Installing npm Package
Installing the npm package for Stripe is a crucial step in integrating Stripe Checkout into your Next.js application. You'll need to install the required Stripe packages by running a command in your project.
These packages provide the necessary tools to integrate Stripe Checkout into your Next.js application. To do this, run the command mentioned in Example 2.
You can install the Stripe npm package by running a single command. This command is mentioned in Example 2.
Here's an interesting read: Can Nextjs Be Used on Traditional Web Application
The Stripe npm package includes the necessary tools to create a checkout session object and pass the items to the handler function. This is essential for processing the checkout.
To process the checkout, you need to install the @stripe/stripe-js library using NPM. This library is required for creating a checkout session object.
Here's a list of the necessary packages to install:
- stripe
- @stripe/stripe-js
Make sure to install these packages to successfully integrate Stripe Checkout into your Next.js application.
Frequently Asked Questions
How do I redirect to the Stripe checkout page?
To redirect to the Stripe checkout page, use Stripe's redirectToCheckout method to securely collect payment information from your customers. This will send them to a Stripe-hosted page, then back to your website after the purchase is complete.
Sources
- https://www.mtechzilla.com/blogs/how-to-integrate-stripe-checkout-in-next-js-a-comprehensive-guide
- https://www.makeuseof.com/stripe-checkout-next-js-application-add/
- https://articles.wesionary.team/getting-started-with-stripe-checkout-next-js-and-tailwind-css-a292c270b3ff
- https://trigger.dev/blog/achieve-nextjs-mastery-build-a-sales-page-with-stripe-and-airtable
- https://www.saasstarterkit.com/blog/Stripe
Featured Images: pexels.com