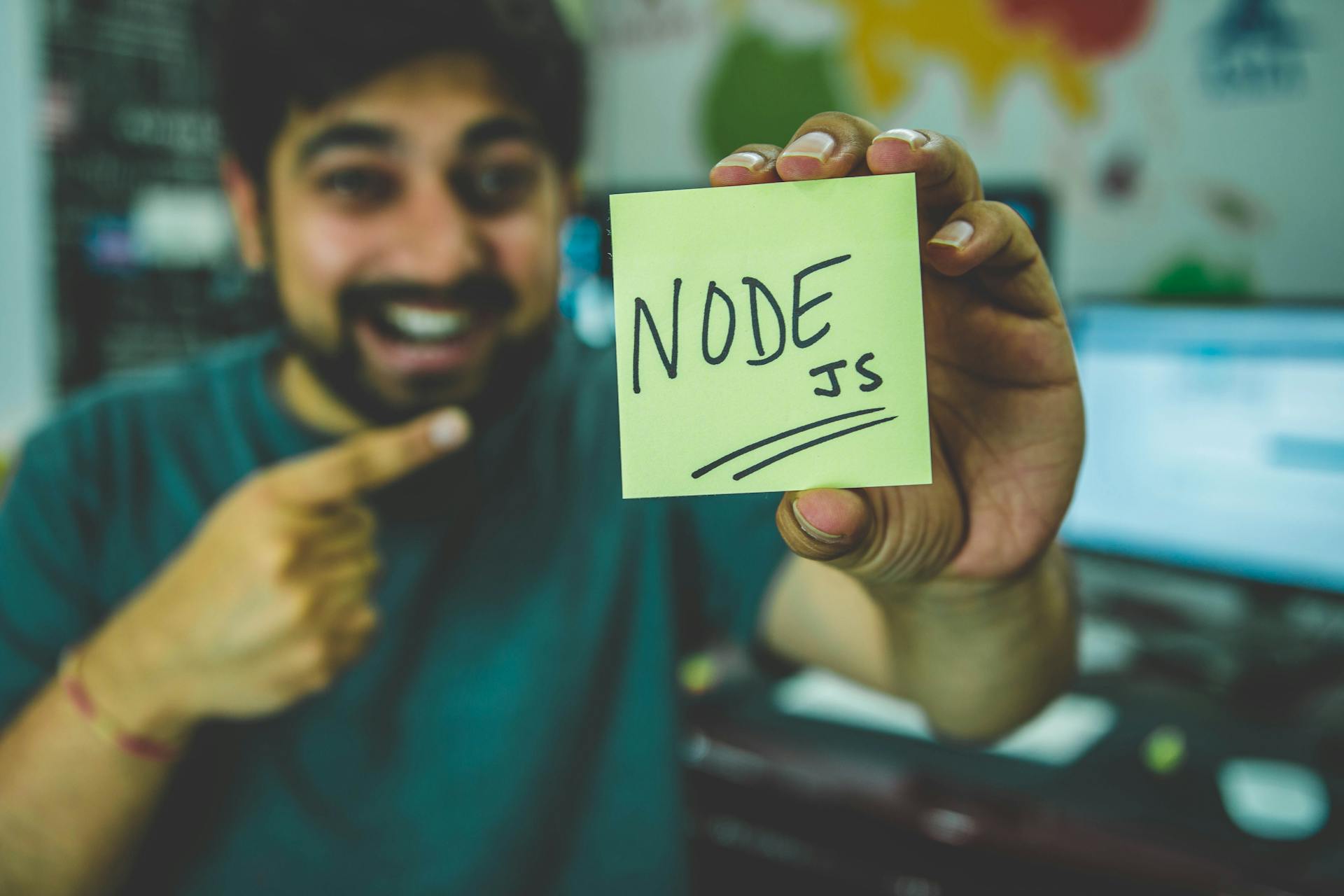
Getting the current URL in a Next.js app is a common requirement for many web applications. You can use the `useRouter` hook from the `next/router` package to get the current URL.
The `useRouter` hook returns an object with several properties, including `pathname`, `query`, and `asPath`. These properties contain the current URL's path, query string, and as-path, respectively.
To get the current URL, you can simply call the `pathname` property of the `useRouter` hook. For example, `const currentUrl = useRouter().pathname;`.
You might enjoy: Next Js Get Pathname
Getting the Current URL
You can get the current URL in a Next.js component using various methods. To get the current URL in a Next.js Pages Router component, use the useUrl hook from the nextjs-current-url package. This hook provides the current URL in a format that's easy to work with.
The useUrl hook returns an object with several properties, including href, protocol, hostname, pathname, search, and hash. The href property contains the complete URL, including the protocol, hostname, port number, path, query parameters, and fragment identifier. This is useful for building dynamic links and styles based on the current page.
Recommended read: Nextjs Components Library
You can also use the usePathname hook to get the current path in a Next.js layout. This hook is a part of Next.js and requires opting in your component to use it as a client component. The usePathname hook returns the current pathname, which you can use to dynamically style links based on the current path.
To get the current URL in a Next.js app router component, you can use the useUrl hook from the nextjs-current-url library. This hook works similarly to the one used in Pages Router components, providing the current URL in a format that's easy to work with.
In some cases, you might need to get the current URL in the getServerSideProps function of a Next.js page. In this case, you can use the getUrl function from the nextjs-current-url library. However, note that you won't be able to get the hash/fragment identifier in getServerSideProps because the browser never sends it to the server.
Here's a summary of the properties returned by the useUrl hook:
- href: the complete URL, including the protocol, hostname, port number, path, query parameters, and fragment identifier.
- protocol: URL's protocol scheme, like https: or mailto:. Doesn't include the //.
- hostname: the URL's domain name without the port number.
- pathname: the URL's path and filename without the query and fragment identifier.
- search: the URL's query parameters. Includes the ?
- hash: the fragment identifier, the part after the #.
Keep in mind that using middleware to access the current page's path on the server is also an option. This involves setting a custom header with the current request's pathname and then accessing it in your component. However, Vercel recommends against this approach due to performance implications.
Using Next.js Built-in Functions
You can use the usePathname hook to retrieve the current pathname without a potential locale prefix. This hook is a solution provided by Next.js to get the current page path.
To use the usePathname hook, you need to opt in your component to use it as a client component. This can be done by adding the "use client" directive to the top of your layout.tsx file.
The usePathname hook gives you the current pathname, which can be used to dynamically style links based on the current path. For instance, you can use Tailwind to apply different styles to links based on their current path.
The pathname will reflect the current path on first load and whenever you change pages. You can use this hook to determine if a path is active and apply styles accordingly.
Readers also liked: Next Js Client Portal
Redirect
If you want to interrupt the render and redirect to another page, you can invoke the redirect function.
Take a look at this: Next Js 14 Redirect to Another Page Loading Indicator
The redirect function wraps the redirect function from Next.js and localizes the pathname as necessary. A locale prop is always required, even if you’re just passing the current locale.
permanentRedirect is supported too.
To work around TypeScript's limitation with control flow analysis, you can add an explicit type annotation to the redirect function.
Intriguing read: Next Js Function Handlers to Filter Data
Using Hooks
You can use the `usePathname` hook to retrieve the current pathname without a potential locale prefix.
The `usePathname` hook returns the pathname corresponding to an internal pathname template if you're using the pathnames setting, but dynamic params won't be replaced by their values.
To get the current URL, you need to use `useEffect`.
This is because pages render on both the server and client in Next.js, so the `window` object isn't available when the server renders the page.
You'll get a "window is not defined" error if you try to use `window` before hydration, which is why `useEffect` is used to wait till mounting/hydration is done.
You might like: How to Use Reducer Api in Next Js 14
Sources
- https://nextjs.org/docs/pages/api-reference/components/link
- https://spacejelly.dev/posts/how-to-style-active-links-in-next-js-app-router
- https://next-intl-docs.vercel.app/docs/routing/navigation
- https://medium.com/@codingbeautytutorials/nextjs-get-current-url-e4e1373333b2
- https://nextjs.org/docs/pages/api-reference/functions/use-router
Featured Images: pexels.com