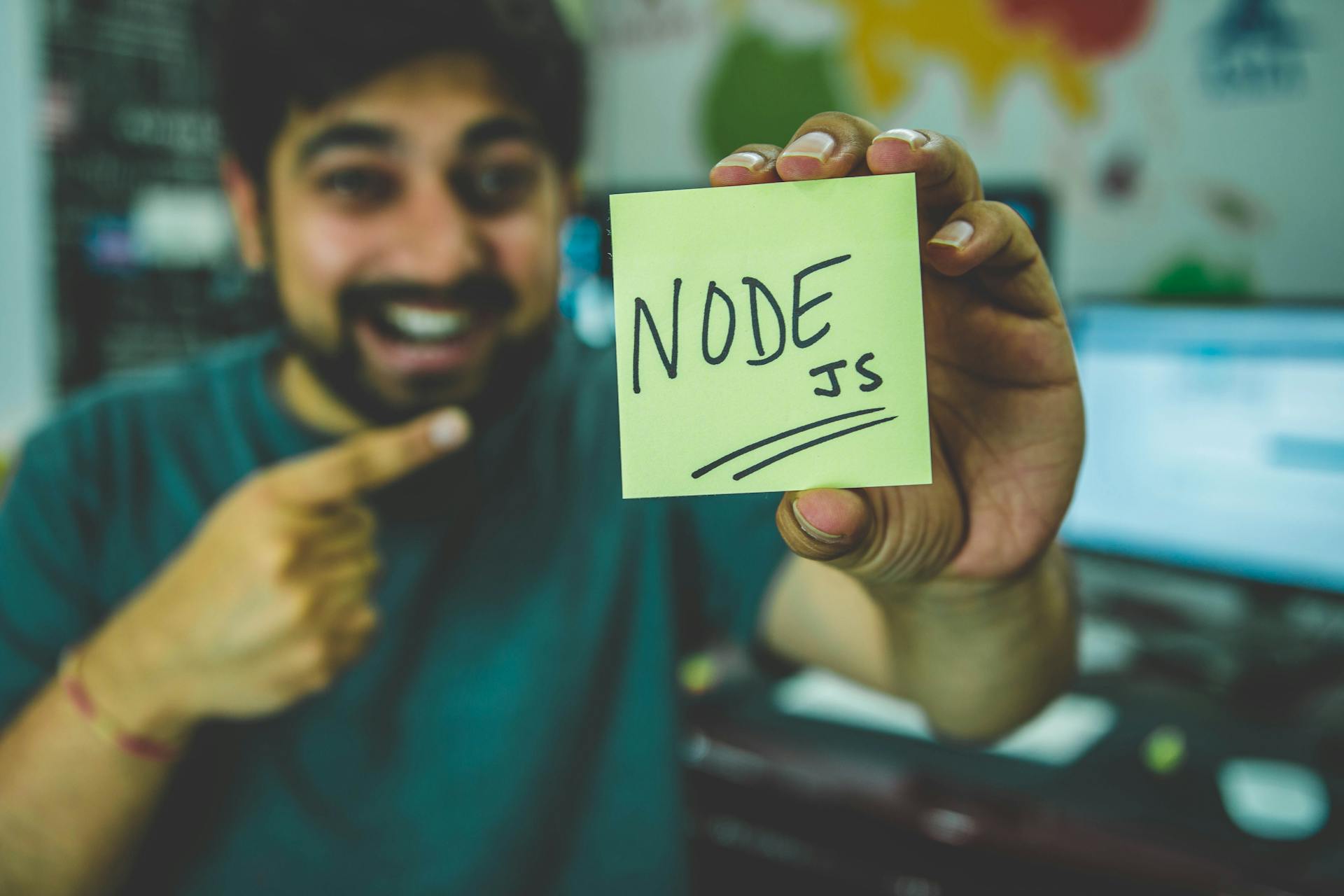
In Next.js, pathname navigation and routing are handled through the use of the `pathname` property in the `useRouter` hook.
The `pathname` property returns the current URL's pathname, which is the part of the URL that comes after the domain name and before any query parameters. For example, if the URL is `https://example.com/about/team`, the pathname would be `/about/team`.
Next.js also provides a way to access the URL's query parameters through the `query` object, but the `pathname` property is what's used for navigation and routing.
With the `pathname` property, you can easily get the current URL's pathname and use it to determine which page or component to render.
You might enjoy: Useeffect Nextjs
Pathname Manipulation
You can retrieve the current pathname without a potential locale prefix by calling usePathname.
The returned pathname will correspond to an internal pathname template, where dynamic params will not be replaced by their values.
You can use path.resolve() to resolve a sequence of paths or path segments into an absolute path.
Consider reading: Nextjs Get Pathname
The method processes the given sequence of paths from right to left, prepending each subsequent path until an absolute path is constructed.
For example, given the sequence of path segments: /foo, /bar, baz, calling path.resolve('/foo', '/bar', 'baz') would return /bar/baz.
If no path segments are passed, path.resolve() will return the absolute path of the current working directory.
Zero-length path segments are ignored, and trailing slashes are removed unless the path is resolved to the root directory.
Here are some key points about path.resolve():
- It resolves a sequence of paths or path segments into an absolute path.
- It processes the given sequence of paths from right to left.
- It ignores zero-length path segments.
- It removes trailing slashes unless the path is resolved to the root directory.
GetStaticPaths
GetStaticPaths is a crucial function in Next.js that allows you to pre-render pages at build time. It's used to fetch data for pages that don't have a direct URL, such as pages that are dynamically generated.
By using GetStaticPaths, you can specify the paths for which your page should be pre-rendered. In the example of a blog, this could be used to pre-render pages for each individual blog post.
See what others are reading: Next Js Blog
Use Pathname
You can retrieve the current pathname without a potential locale prefix by calling usePathname. This method is useful when you want to get the pathname without any extra prefixes.
If you're using the pathnames setting, the returned pathname will correspond to an internal pathname template, and dynamic params will not be replaced by their values.
To break down a pathname into its significant elements, you can use the path.parse() method. This method returns an object with properties that represent the dir, root, base, name, and ext of the path.
The path.parse() method ignores trailing directory separators and returns an object with the following properties:
- dir: a string representing the directory of the path
- root: a string representing the root of the path
- base: a string representing the base of the path
- name: a string representing the name of the path
- ext: a string representing the extension of the path
Path.resolve([...paths])
The path.resolve() method is a powerful tool for resolving a sequence of paths or path segments into an absolute path. It's often used to construct a final path by combining multiple path segments.
The method processes the given sequence of paths from right to left, with each subsequent path prepended until an absolute path is constructed.
For instance, if you pass the sequence of path segments: /foo, /bar, baz, the method would return /bar/baz because 'baz' is not an absolute path but '/bar' + '/' + 'baz' is.
If no path segments are passed, the method will return the absolute path of the current working directory.
Zero-length path segments are ignored, so you don't have to worry about empty strings causing issues.
The resulting path is normalized and trailing slashes are removed unless the path is resolved to the root directory.
Navigation and Routing
You can use the Link component to wrap next/link and localize the pathname as necessary. This component can be used to create active links in your application.
The useSelectedLayoutSegment hook from Next.js allows you to detect if a given child segment is active from within the parent layout. You can match this internal pathname against an href to determine if the link is active.
If you need to create a component that receives an href prop, you can compose the props from Link with the ComponentProps type. This will allow you to forward the href prop to Link internally.
A unique perspective: Nextjs Links
The href prop of the wrapping component will be strictly typed based on your routing configuration if you're using the pathnames setting. If you need to link to unknown routes, you can disable type checking on a case-by-case basis.
You can use useRouter from Next.js to navigate programmatically. Next-intl provides a convenience API that wraps useRouter and localizes the pathname accordingly.
Redirects and Retrieval
Redirects in Next.js allow you to interrupt the render and redirect to another page using the redirect function, which wraps the Next.js redirect function and localizes the pathname as necessary.
The redirect function requires a locale prop, even if you're just passing the current locale. This is a requirement, not an option.
To avoid issues with TypeScript's control flow analysis, you can add an explicit type annotation to the redirect function. This is a workaround for the limitation in TypeScript's ability to narrow types correctly after calling redirect.
You might enjoy: Next Js with Typescript
Redirect
To redirect to another page, you can invoke the redirect function, which wraps the redirect function from Next.js and localizes the pathname as necessary.
A locale prop is always required, even if you're just passing the current locale.
You can use permanentRedirect as well.
However, TypeScript has a limitation with control flow analysis, which can make it difficult to narrow types correctly after calling redirect.
To work around this limitation, you can add an explicit type annotation to the redirect function.
The [email protected] release brought the first release of the navigation APIs, which include these redirect functions.
Explore further: Next Js 14 Redirect to Another Page Loading Indicator
Get
A redirect is essentially a permanent or temporary change of address for a web page or resource, allowing you to move or update content without breaking existing links.
Redirects can be used to update URLs, merge websites, or even retire old content.
A 301 redirect is a permanent redirect, informing search engines and users that the old URL has been replaced by a new one.
A unique perspective: Next Js Update
This type of redirect is ideal for updating URLs or moving content to a new location.
Temporary redirects, on the other hand, are used for maintenance or testing purposes, indicating to users and search engines that the content will be available again soon.
These redirects are often used during website updates or when fixing broken links.
Sources
- https://nextjs.org/docs/pages/api-reference/functions/get-static-paths
- https://next-intl-docs.vercel.app/docs/routing/navigation
- https://nodejs.org/api/path.html
- https://nextjs.org/docs/pages/api-reference/functions/use-router
- https://nextjs.org/docs/app/building-your-application/routing/linking-and-navigating
Featured Images: pexels.com