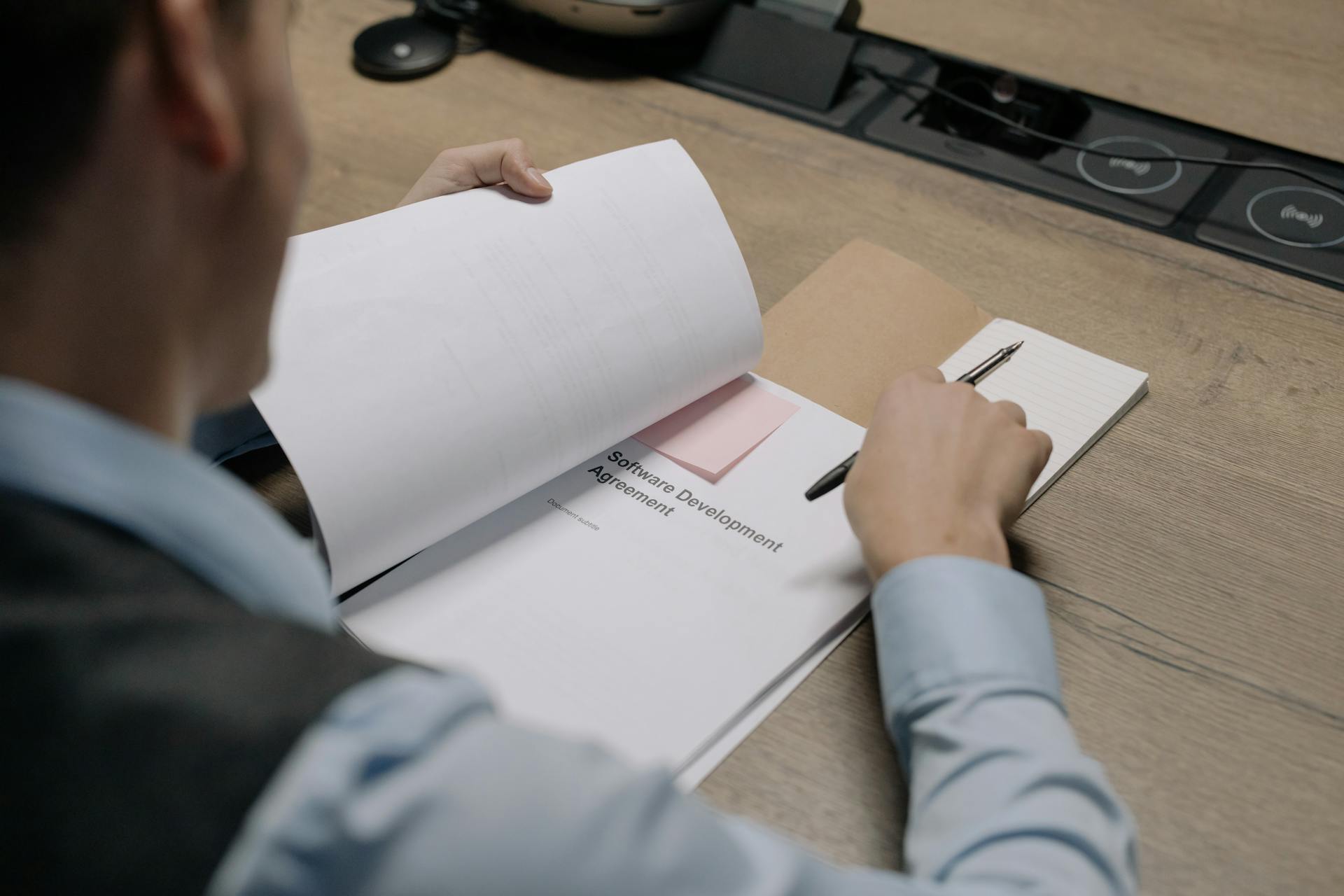
In a Next.js project, the `next.config.js` file is where you'll find configuration settings for your app. This file is where you can customize the behavior of Next.js.
The `pages` directory is where you'll store your route components. By default, Next.js looks for files in this directory to determine the routes of your app. For example, a file named `[id].js` in this directory will match any URL that has an ID in the path.
To get started with TypeScript in your Next.js project, you'll need to install the `@types/next` and `@types/react` packages. This will provide the type definitions for Next.js and React.
Next.js projects use the `tsconfig.json` file to configure the TypeScript compiler. This file specifies the settings for the compiler, such as the target JavaScript version and the module resolution.
Take a look at this: File Upload Next Js Supabase
Configuration
Configuration is a crucial part of Next.js with TypeScript, allowing you to customize how your code is compiled and executed. You can configure absolute imports and module path aliases in tsconfig.json to make importing modules across your application easier.
TypeScript path aliases allow you to create shortcuts for absolute paths in your application, which can be resolved to the actual path. You can use the baseUrl and path options in tsconfig.json to configure path aliases. For example, you can create a path alias for all files in the MUIComponents folder for easy access.
The tsconfig.json file is where you'll find most of the compiler options, including the target version of JavaScript, library files to include, and strict option checking. Some common options include target, lib, allowJs, skipLibCheck, and strict.
Here's a summary of some common compiler options:
- target: specifies the version of JavaScript that the TypeScript code compiles into (e.g. "es5" or "es6")
- lib: specifies the list of library files to include in the compilation (e.g. "dom", "dom.iterable", and "next" together for a web project)
- allowJs: allows JavaScript file compilation, which can be helpful when introducing TypeScript gradually into an existing JavaScript codebase
If you're not using TypeScript in your project, you can use a jsconfig.json file instead.
Configure Authentication
To configure authentication for your Next.js app, you'll first need to set up your .env.local file correctly. This file should be added to the root of your project, and you'll need to copy values from .okta.env into it.
Related reading: Next Js Folder Structure
Next, create a new folder called auth under your pages/api folder if it doesn't exist yet. Inside this folder, add a file called [...nextauth].ts and add the provided code to it.
You'll also need to open pages/_app.tsx and replace the existing code with the default Next.js code wrapped in a Provider class from next-auth. This will enable you to share the session between pages more easily.
Finally, add a file called Unauthorized.tsx to handle cases where a user is not authenticated. To check if a user is authenticated, you can simply check if the session exists.
For your interest: Nextjs Session
Compiler Options
You can use a tsconfig.json file to configure your TypeScript project, and one of the most important sections is the compiler options. This is where you can specify how your TypeScript code should be compiled into JavaScript.
The target option determines the version of JavaScript that your code will compile into, with standard settings being "es5" and "es6".
Intriguing read: Nextjs Code Block
You can also specify the list of library files to include in the compilation with the lib option, which is often set to "dom", "dom.iterable", and "next" together for a web project.
Allowing JavaScript file compilation with the allowJs option can be helpful in introducing TypeScript gradually into an existing JavaScript codebase. This option can save you time and effort in the long run.
The skipLibCheck option can speed up your compilation process by avoiding the checking of declaration files (.d.ts).
Here are some of the most common compiler options:
You can also use the noEmit option to prevent the output files from being emitted by the compiler, which can be useful for projects where you're only concerned with type checking.
Configuring Absolute Imports and Module Path Aliases
Configuring Absolute Imports and Module Path Aliases is a crucial step in any application.
You can configure path aliases in tsconfig.json to create shortcuts for absolute paths in your application.
Broaden your view: Next Js Spa
TypeScript path aliases allow us to create aliases or shortcuts for absolute paths.
Next.js automatically supports path aliases, so we don't have to do many configurations.
To create a path alias, you can use the baseUrl and path options in tsconfig.json.
For example, you can use the baseUrl option to specify a root URL for your imports.
The path option lets you configure path aliases, which you can read more about here.
With path aliases, you can easily access modules across your application.
For instance, if you have a file importing a module with a path structure like /components/MUIComponents/ButtonGroup/, you can create a path alias for all files in the MUIComponents folder.
This way, you can use the alias in your application instead of writing an ugly code with the full path.
Related reading: Next Js Use Client
Building Components
In Next.js, you can create reusable components to build your application's UI. This is achieved by adding a new folder called components to the root of your project, where you can store shared components.
To start, add a new file called Movies.tsx and add the component code, which will be displayed on the Dashboard page for logged-in users. This component will display some of the most important movies in history to the user.
A good practice is to create a Header.tsx file, which is just a banner that sits on top of each page and has a login or logout button depending on the state of the user. You can check if a user is logged in by calling useSession() from next-auth, and present the user with the option to log out using the signOut() function if it does, or a login button that ties into the signIn() function if it does not.
You can tie everything together by creating your pages, such as the landing page and dashboard page. By default, Next.js should have added an index.tsx file, which you can replace with the code to display a landing page.
For more insights, see: Nextjs Landing Page
Create an App
To create an app, you can use the create-next-app task runner. This is done by running the command npx create-next-app in your console, and following the instructions to name your application. For example, if you name your application "movies", the runner will create a folder for you.
You can also add a --typescript or --ts flag to the create-next-app command to bootstrap a Next.js application with TypeScript. However, make sure you really know what you're doing before using this option.
To set up TypeScript support, you'll need to create a new file called tsconfig.json in the root of your application. Next.js will automatically populate this file with its default values, but you can edit them if you wish.
Here are the dependencies you should add at this point:
- next-auth, which makes setting up any type of authentication in Next.js simple
- Okta as an external provider, which you'll implement later
You can install next-auth with the command npm install next-auth.
You might like: Install Nextjs
Write Components and Pages
To write your components and pages in Next.js, start by creating a new folder called components in the root of your project. This will be a folder for your shared components. You can add a new file called Movies.tsx and add the code to display some of the most important movies in history to the user.
On a similar theme: How to Add Img to Nextjs
The Movies component will be displayed on the Dashboard page for logged-in users. You can use the signIn feature from the next-auth/client component to navigate the user to a list of providers that you implemented previously. This function will take the user to their login screen after they click on a provider.
To see if a user is logged in, you can check if the session exists by calling useSession() from next-auth. If it does, you present the user with the option to log out using the signOut() function. If it does not, you present the user with a login button that ties into the signIn() function.
You can tie everything together by creating your pages. The simplest way to do this is to create a new file in the pages directory called dashboard.tsx. This page will need to check if the user has access to the page and display the Unauthorized component if not and the Movies component if they are.
Check this out: Nextjs Middleware Firebase Auth
Testing and Debugging
In Next.js TypeScript, debugging errors can be a challenge, but it's not impossible. Reading the error message carefully is crucial to pinpointing the issue.
Type definitions are often the culprit behind TypeScript errors. Ensure all your types are well defined and imported to avoid any issues.
One simple trick is to use type assertions to tell TypeScript that you know what you're doing. This can often resolve the error.
Sometimes, updating your dependencies can make the errors disappear. Make sure to update TypeScript and your type definitions, such as @types/react, to the latest version.
Here are some tips to help you debug TypeScript errors in Next.js:
- Check Type Definitions: Ensure all your types are well defined and imported.
- Use Type Assertions: Use type assertions to tell TypeScript, "trust me, I know what I'm doing."
- Update Dependencies: Sometimes updating TypeScript or your type definitions (@types/react for example) will make the errors go away.
Test and Verify Functionality
Testing your application is crucial to ensure everything works as expected. Run npm run dev and navigate to http://localhost:3000 to start your application.
You should be presented with the home screen for the application. From there, click on the Movies link to test the navigation.
For another approach, see: How to Run Nextjs to Build
You'll receive a message telling you that you don't have access to the dashboard. Click Sign in and select Okta, then log in with your Okta credentials to test the authentication process.
If everything is set up correctly, you should be redirected to the dashboard page showing you some of the greatest movies in history. This is a crucial step in verifying that your application is safe and secure with Okta's authentication.
Consider reading: Next Js Admin Dashboard
Debug Errors
Debugging errors is a crucial part of the testing process. It's essential to read the error message carefully, as it often explains what's gone wrong and where.
TypeScript errors can be particularly frustrating, but there are a few tips to help you debug them. First, check your type definitions to ensure they're well defined and imported.
Use type assertions to tell TypeScript you know what you're doing, but be cautious not to ignore potential issues. Updating your dependencies, such as TypeScript or type definitions, can also resolve errors.
A strong type system is one of the primary benefits of TypeScript, helping catch potential errors and bugs during development. This leads to better code quality and reduces the likelihood of bugs.
TypeScript's compiler can detect type-related issues, like assigning a value of the wrong type to a variable or passing incorrect arguments to a function. It provides early warnings to ensure data types are consistent throughout a codebase.
Here are some tips to help you debug TypeScript errors:
- Check Type Definitions: Ensure all your types are well defined and imported.
- Use Type Assertions: Use type assertions to tell TypeScript, "trust me, I know what I'm doing."
- Update Dependencies: Sometimes updating TypeScript or your type definitions (@types/react for example) will make the errors go away.
Best Practices
Writing clean and maintainable code is essential in Next.js with TypeScript. This ensures better readability and less technical debt.
To achieve this, it's best to use type annotations. By implementing interfaces and types, you can make your code more self-explanatory and easier to understand.
Making functions small is also a good practice. This helps reduce complexity and improves the overall maintainability of your code.
Here are some specific best practices to keep in mind:
- Implement interfaces and types to make your code more self-explanatory.
- Make functions small to reduce complexity and improve maintainability.
- Do not use the 'any' type, as it can lead to type errors and make your code harder to maintain.
By following these best practices, you can write clean and maintainable code in Next.js with TypeScript, making it easier to work with and understand your codebase.
Type Safety and Error Detection
Type safety is a crucial aspect of Next.js with TypeScript. TypeScript's strong type system helps catch potential errors and bugs during development, leading to better code quality. This reduces the likelihood of bugs and makes our code easier to maintain.
TypeScript's compiler can detect type-related issues, like assigning a value of the wrong type to a variable or passing incorrect arguments to a function, and provide early warnings. This is a game-changer for developers, as it allows us to catch errors before they even make it to production.
Here are some ways TypeScript helps with type safety in Next.js:
- Ensures consistent data types throughout a codebase
- Catches potential errors and bugs during development
- Provides early warnings for type-related issues
By enforcing strict typings, TypeScript helps us prevent errors and bugs from making it to production. This is especially important in Next.js, where we can use features like statically typed links to prevent typos and other errors when using next/link.
With TypeScript, we can create interfaces for our data structures, like the Post object in Next.js. This helps ensure that our data is consistent and predictable, making it easier to maintain and debug our code.
If this caught your attention, see: Next Js Fetch Data save in Context and Next Route
Benefits and Features
Using Next.js with TypeScript is a powerful combination that offers numerous benefits for developers. It enhances their experience and the quality of the applications they create.
TypeScript's static typing system helps catch errors early, making it easier to maintain and refactor codebases. This is especially reassuring when working on large projects with multiple developers contributing.
With TypeScript, you'll benefit from better autocompletion, code navigation, and refactoring features in modern IDEs. It's a delight to work with code that's easier to understand and navigate.
TypeScript ensures that code adheres to defined types, making applications more reliable and less prone to runtime errors. This added security brings peace of mind, knowing your application is better protected against unforeseen issues.
Here are some key benefits of using TypeScript with Next.js:
- Static typing system helps catch errors early
- Better autocompletion, code navigation, and refactoring features
- Ensures code adheres to defined types
- Increases reliability and reduces runtime errors
Both Next.js and TypeScript are designed to support large-scale applications, making them a perfect match for growing projects. TypeScript's type system enforces a SOLID structure that promotes best practices, making it easier to scale the codebase without compromising on maintainability or quality.
Troubleshooting
Troubleshooting is a crucial part of working with Next.js and TypeScript. Read error messages carefully to pinpoint issues in your code.
Check Type Definitions, ensuring all types are well defined and imported, can resolve many errors. This includes importing necessary type definitions, such as @types/react.
Use Type Assertions to tell TypeScript you know what you're doing, but be cautious not to overuse them. Updating Dependencies, like TypeScript or your type definitions, can also resolve errors.
Disabling Errors in Production
If you happen to have TypeScript errors while running next build, Next.js will fail the build, but you can disable the type checks if you wish.
You can disable the type checking step by enabling the ignoreBuildErrors option in next.config.js.
This can be done to avoid failing the build, but it's generally better to address the errors first.
Enabling the ignoreBuildErrors option will allow your build to proceed, even with TypeScript errors present.
Common Issues and Workarounds
Troubleshooting can be a frustrating experience, but there are some common issues that can be easily resolved with the right approach. Reading error messages carefully is key to pinpointing and fixing issues in your code.
Many error messages will explain what has gone wrong and where, so take the time to read them carefully. This will save you a lot of time and effort in the long run.
Check your type definitions to ensure they are well defined and imported. This is a common issue that can be easily fixed by reviewing your code.
Use type assertions to tell TypeScript to trust you when you're sure of what you're doing. This can be a lifesaver when you're working with complex code.
Updating your dependencies, such as TypeScript or type definitions, can also resolve errors. This is especially true if you're using a library like @types/react.
Here are some common issues and their workarounds:
Frequently Asked Questions
Should I use TypeScript with NextJS on Reddit?
Yes, using TypeScript with NextJS is highly recommended for developing robust and maintainable business applications on Reddit, as it provides strong type safety and scalability
Sources
- https://strapi.io/blog/introducing-the-new-strapi-starter-with-nextjs13-tailwind-and-typescript
- https://developer.okta.com/blog/2020/11/13/nextjs-typescript
- https://refine.dev/blog/next-js-with-typescript/
- https://blog.logrocket.com/using-next-js-with-typescript/
- https://prismic.io/blog/nextjs-typescript
Featured Images: pexels.com