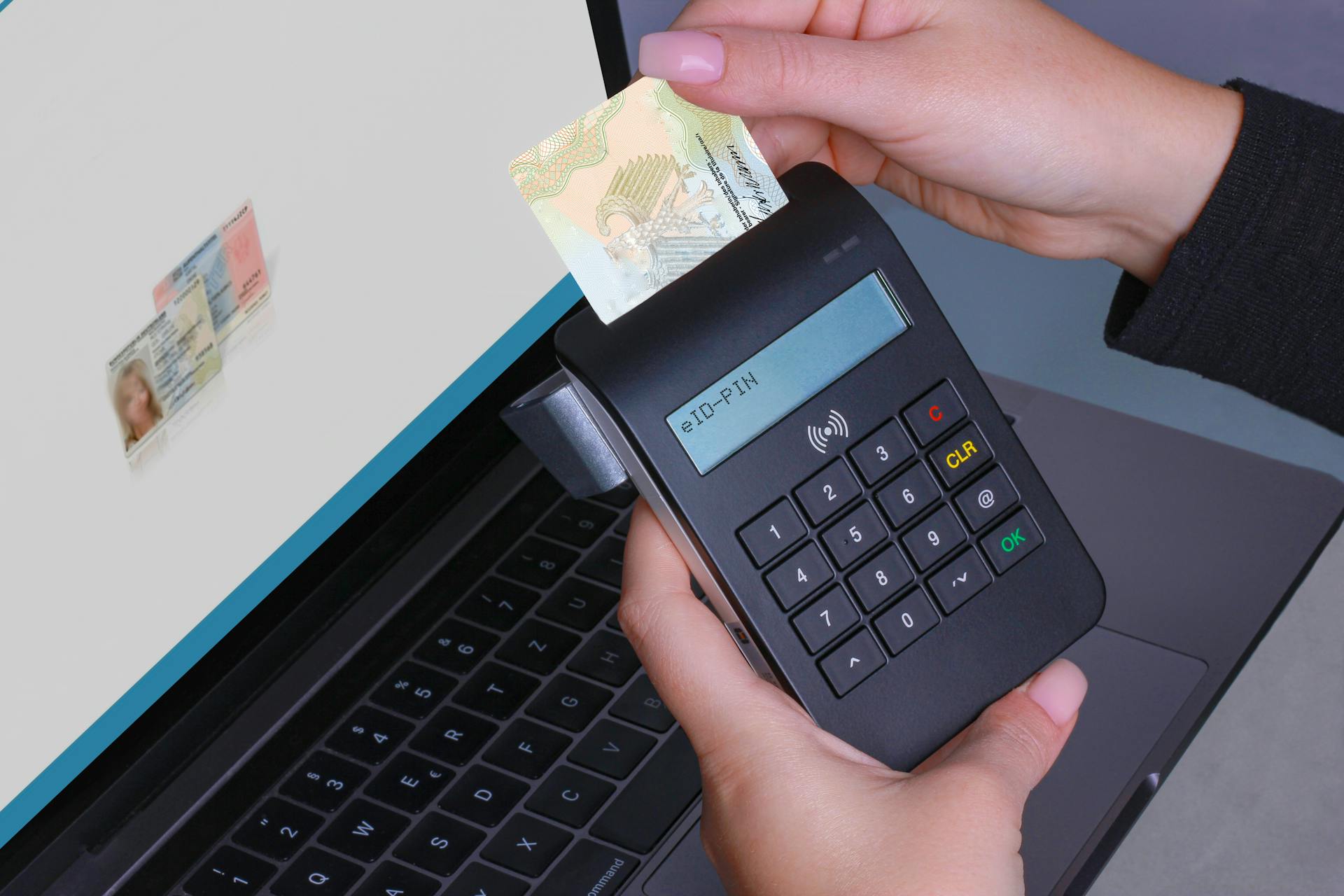
To store a login session in Next.js, you'll need to use a library like NextAuth.js, which simplifies the process by handling authentication for you.
NextAuth.js provides a simple API for storing user sessions, allowing you to easily manage and retrieve session data.
To get started, you'll need to install NextAuth.js and configure it in your Next.js project, which involves setting up a provider, such as Google or GitHub, to authenticate users.
By using NextAuth.js, you can focus on building your application without worrying about the complexities of authentication and session management.
A fresh viewpoint: Nextjs Client Session Token
Prerequisites
To store a login session in Next.js, you need to set up a secret key that's used by both NextAuth and the middleware. The easiest way to do this is by setting the NEXTAUTH_SECRET environment variable.
This environment variable will be picked up by both the NextAuth config and the middleware config, making it a convenient and centralized way to manage your secret key.
Alternatively, you can provide the secret using the secret option in the middleware config, but we strongly recommend replacing the secret value completely with the NEXTAUTH_SECRET environment variable.
Expand your knowledge: Nextjs Middleware Matcher
Authentication Setup
To set up authentication in a Next.js app, you'll need to install NextAuth.js using yarn. Run the command `yarn add next-auth` to get started.
You'll also need to create a new file called `pages/api/auth/[...nextauth].js` to configure NextAuth.js. In this file, you'll define your authentication providers, such as credentials, magic links, or social media logins.
NextAuth.js requires a secret key to sign and encrypt tokens. You can generate a secret key using the command `npx next-auth snack`, or you can use a package like dotenv to load environment variables from a `.env` file.
To make the authentication session available throughout your Next.js app, you'll need to wrap your entire application with the SessionProvider. This component makes the authentication session available throughout your Next.js app.
Here's a list of the steps to set up the SessionProvider:
- Import the SessionProvider from `next-auth/react`.
- Wrap your entire application with the SessionProvider.
- Pass the session from `pageProps` to the SessionProvider.
Alternatively, you can use a tool like direnv to manage your environment variables. This allows you to define project-specific environment variables in a `.envrc` file.
To set up the Credentials provider, you'll need to create a new file called `pages/api/auth/[...nextauth].ts`. In this file, you'll define the `authOptions` and pass it to the default export. You'll also need to pass the fields `username` and `password` with their type and placeholder values.
Here's an example of the `authOptions` object:
```json
{
"providers": [
{
"credentials": {
"username": {
"type": "string",
"placeholder": "Username"
},
"password": {
"type": "string",
"placeholder": "Password"
}
}
}
]
}
```
See what others are reading: Can Amazon S3 Take in Nextjs File
Login/Logout
Logging in and out is a breeze with NextAuth.js. You can continue using the methods to signIn, signOut, useSession, and more just like you're used to.
The signIn function is called when the user clicks the "Login" button, initiating the authentication flow. This function is provided by NextAuth.js middleware.
Users who click the button to Sign in will be redirected to the NextAuth.js page to enter their username and password. You can customize this page or work with the API directly inside of your own page component if you want.
Here's an interesting read: Next Js 14 Redirect to Another Page Loading Indicator
The signOut function is called when the user clicks the "Logout" button, logging them out of the application. This is done using the signOut function from next-auth/react.
User data is stored in Grafbase, which is a user database. NextAuth.js prefers user data to be managed by a OAuth 1.0 or 2.0 service, but it provides a credentials provider for those who have an existing user database like Grafbase.
A fresh viewpoint: Best Next Js Database with Drizzle
Middleware and Routing
You can use a Next.js Middleware with NextAuth.js to protect your site. Middleware is a way to run logic before accessing any page, even when they are static.
To use middleware in Next.js, create a middleware.ts file at the root of your application or in the src directory. The matcher property in the config object dictates the paths to which the middleware function should be applied.
Here's a breakdown of how to protect routes with middleware:
- Create a middleware file (e.g., middleware.ts) and update it with the middleware code.
- Define the routes you want to protect in the config object.
- Export an auth instance from the middleware file without a database adapter attached.
This approach centralizes the logic for protecting routes, making it easier to manage and maintain your application.
Here's a comparison of protecting routes in Server Components and with middleware:
Setting Up Next
To set up NextAuth.js, you'll need to create a new file called [...nextauth].js in the pages/api/auth directory of your project. This file is where you'll define your NextAuth.js configuration.
First, make sure you have a Next.js project ready. You can create a new one by running the following command in your terminal: `npx create-next-app my-app`. Once you have your project set up, navigate into the project directory.
Next, install NextAuth.js using yarn by running `yarn add next-auth`. Now that NextAuth.js is installed, it's time to configure it.
Create a new file called [...nextauth].js in the pages/api/auth directory of your project, and add the following code: `export default NextAuth({ providers: [] });`. In this file, you'll specify the authentication providers you want to use, such as credentials, magic links, or social media logins.
To generate a secret key for NextAuth.js, run the command `openssl rand -base64 32` in your terminal. You can also use a package like dotenv to load environment variables from a .env file. Install dotenv by running `yarn add dotenv`.
Check this out: Next Js Multi Tenant App
Protecting Routes with Middleware
Protecting routes with middleware is a powerful way to control access to certain pages in your Next.js application. You can use a middleware function to check if a user is authenticated before allowing them to access a particular route.
Next.js 12 introduced middleware, which allows you to run logic before accessing any page, even when they are static. This is useful for running authentication checks, request validation, and other tasks that require access to the request and response objects.
To use middleware in Next.js, you need to create a middleware file and update it with the necessary code. The middleware file should contain a function that takes the request and response objects as arguments and returns a boolean value indicating whether the request should be allowed to proceed.
You can get the withAuth middleware function from next-auth/middleware or create your own custom middleware function. The withAuth middleware function takes a config object as an argument, which defines the routes that should be protected.
A unique perspective: How to Use Reducer Api in Next Js 14
Here's an example of how you can use the withAuth middleware function to protect a route:
```javascript
import { NextApiRequest, NextApiResponse } from 'next';
import { withAuth } from 'next-auth/middleware';
export default withAuth({
callbacks: {
authorized: ({ token }) => {
if (token) {
return true;
}
return false;
},
},
})(async (req: NextApiRequest, res: NextApiResponse) => {
// Handle the request
});
```
This code creates a middleware function that checks if the user is authenticated by checking the token object. If the token is present, the request is allowed to proceed; otherwise, it is blocked.
You can also create a custom middleware function that uses the NextAuth.js API to check if the user is authenticated. Here's an example:
```javascript
import { NextApiRequest, NextApiResponse } from 'next';
import auth from 'next-auth';
export default async (req: NextApiRequest, res: NextApiResponse) => {
const session = await auth.getSession();
if (!session) {
return res.status(401).json({ error: 'Unauthorized' });
}
// Handle the request
};
```
This code creates a middleware function that checks if the user is authenticated by calling the getSession method of the NextAuth.js API. If the session is not present, the request is blocked with a 401 status code.
In summary, protecting routes with middleware is a powerful way to control access to certain pages in your Next.js application. You can use the withAuth middleware function or create your own custom middleware function to check if the user is authenticated before allowing them to access a particular route.
If this caught your attention, see: Nextjs 13 Middleware
User Management
You can require authentication for your entire site by adding a middleware.js file with a simple configuration.
This will secure all pages on your site, ensuring only authenticated users can access them.
If you only want to secure certain pages, you can export a config object with a matcher to specify which pages require authentication.
The default behavior is to redirect unauthenticated users to the sign-in page, allowing them to log in and access the secure pages.
Setting Up Prisma with PostgreSQL
To set up Prisma with PostgreSQL for NextAuth.js, you'll need to install the required dependencies. This will get your project off the ground and allow you to start working with Prisma.
First, you'll need to create a new Prisma schema file called schema.prisma. This file defines the structure of your database tables and the relationships between them. The schema example provided includes models for User, Account, Session, and VerificationToken.
You can extend the schema to include additional customer data by modifying the User model or creating a new model with a foreign key relationship to the User model. For example, you could add an Address model with a one-to-one relationship to the User model.
Suggestion: Nextjs File Upload Api
To set up the database connection, you'll need to add the database connection URL to your .env file. This will tell Prisma how to connect to your PostgreSQL database.
Once you've set up the schema and database connection, you can run Prisma migrations to create the necessary tables in the database. This is a crucial step in setting up your Prisma project.
The Prisma schema can be extended further to include any additional customer data fields or relationships based on your application's requirements. You can modify the User model or create new models to accommodate your needs.
Here's a simplified example of how you can add an Address model with a foreign key relationship to the User model:
```html
models:
User {
id String @id @default(cuid())
email String @unique
address Address?
}
Address {
id String @id @default(cuid())
userId String @unique
street String
city String
@relation(fields: [userId], references: [id], onDelete: Cascade)
}
```
This example shows how to add an Address model with a one-to-one relationship to the User model. The userId field in the Address model serves as the foreign key that references the id field in the User model.
Discover more: How to Add Custom Font to Next Js
Accessing User Data
You can use the useSession hook to access the user's session data in your pages and components. This hook is provided by NextAuth.js and is used to conditionally render content based on the user's authentication status.
To use the useSession hook, you can import it from next-auth/react and use it in your components. For example, you can use it to check if a session exists and render the sign-in and sign-out links accordingly.
The useSession hook provides several benefits, including the ability to access the user's session data and render user-specific content. You can also use it to conditionally render content based on the user's authentication status.
Here are some ways to use the useSession hook:
- To check if a session exists and render the sign-in and sign-out links accordingly.
- To access the user's session data and render user-specific content.
- To conditionally render content based on the user's authentication status.
You can also use the unstable_getServerSession function from next-auth/next to fetch the user session on the server-side. This function is used in the getServerSideProps function to fetch the user session on the server-side and pass it as props to the component.
Here's an interesting read: Iron Session Next Js Middleware
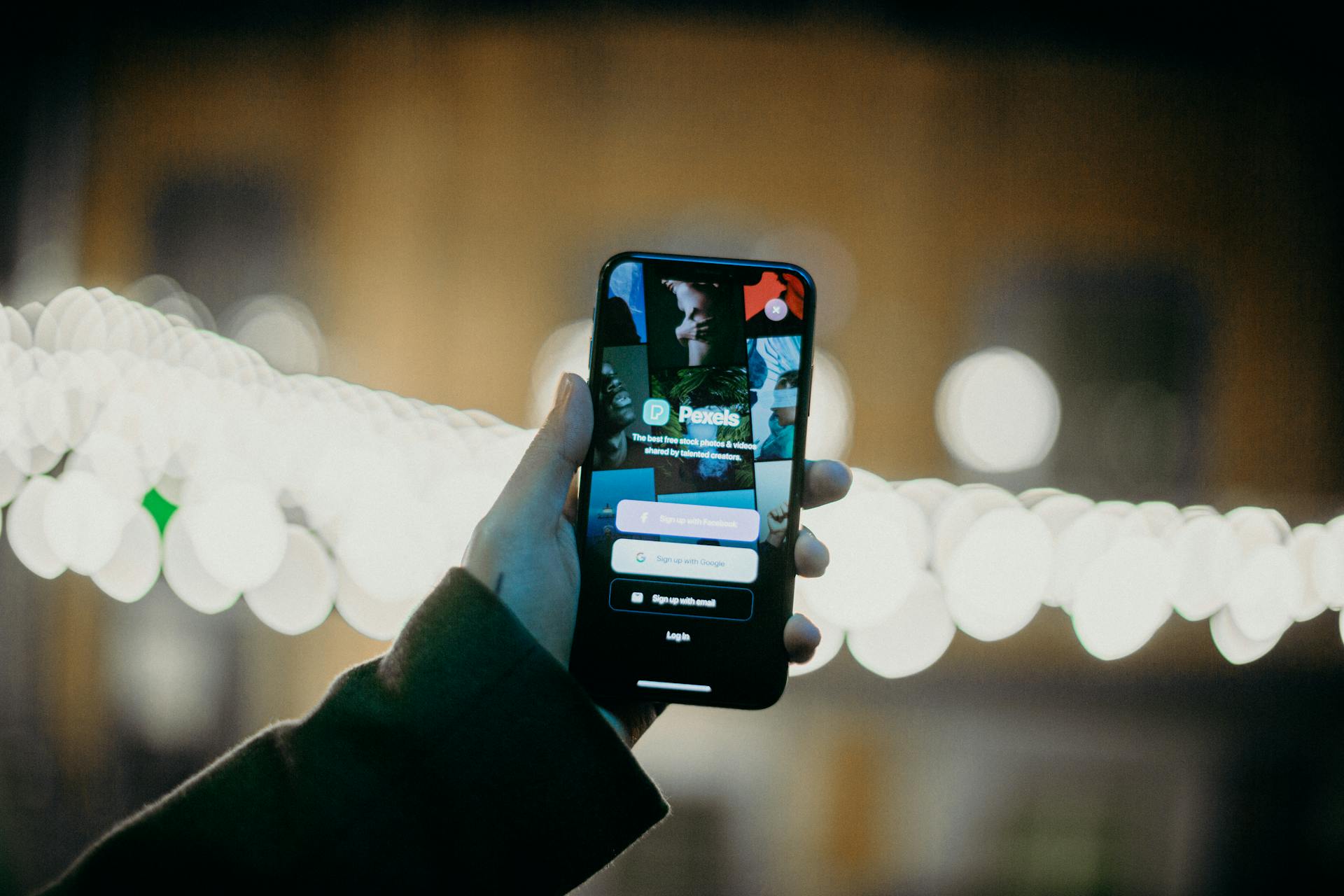
For example, you can use the unstable_getServerSession function to fetch the user session on the server-side and render user-specific content. If the user is not authenticated, you can redirect them to the login page.
Here are some benefits of using the unstable_getServerSession function:
- You can fetch the user session on the server-side and render user-specific content.
- You can redirect users to the login page if they are not authenticated.
In summary, accessing user data is an essential part of user management. You can use the useSession hook to access the user's session data and render user-specific content, and you can use the unstable_getServerSession function to fetch the user session on the server-side and render user-specific content.
A unique perspective: Nextjs Session
Social Login
Social login is a fantastic way to make your app more user-friendly and convenient. With social login, users can sign in using their existing accounts from popular platforms like Google, Twitter, and Facebook.
To add social login options to your Next.js app, you'll need to install the necessary provider packages. This involves installing packages for each provider you want to include, such as Google, Twitter, and Facebook.
For another approach, see: Nextjs Stripe
You'll also need to update your pages/api/auth/[...nextauth].js file to include the desired providers. This is where you specify which providers you want to enable for social login.
To get started, you'll need to obtain the necessary API keys and secrets from the respective developer platforms. For Google, you'll need to create a new project in the Google Developer Console and set up OAuth 2.0 credentials. This will give you a client ID and client secret that you'll need to add to your .env.local file.
Here are the API keys and secrets you'll need for each provider:
Once you have the necessary keys and secrets, you can add them to your .env.local file. This will allow NextAuth.js to authenticate users using the social login providers.
With social login set up, users can click on a provider's login button to sign in. This will redirect them to the provider's login page, where they can authenticate using their existing account credentials. After successful authentication, the user will be redirected back to your app's callback URL, where they'll be logged in and ready to go.
For your interest: Next Js Provider
Magic Links via Email
Magic Links via Email is a secure and popular alternative to passwords and usernames. It's made easy to implement with Auth.js and the Email Provider.
To get started, you'll need to configure a database to securely store and manage the unique tokens sent to users for authentication. This ensures that tokens are valid, not reused, and can be easily revoked if necessary.
A database is required for token management, but the session management strategy can still be set to jwt, allowing for stateless sessions, reduced database load, and improved scalability.
To set up a database, you can adopt the MongoDB adapter, which has adapters for various databases including SQL, Postgres, and more. Create a new file at lib/mongo-client.ts with the code from the MongoDB docs to make sure that you pass the adapter a MongoClient that is already connected.
The Email Provider for magic links requires adding it to the providers array in your authOptions configuration. This is done by importing the EmailProvider and adding it to the providers array.
Take a look at this: Next Js Database
In the EmailProvider configuration, the from option specifies the sender's email address, maxAge sets the expiration time for the magic link, and sendVerificationRequest is a custom function that sends the login email.
A simple HTML email template with the login link can be constructed using the necessary information from the params object. This information is then used to send the email to the user using a custom sendEmail function.
For reliable email delivery, it's recommended to use a service like Postmark, Amazon SES, or Mailgun, rather than a popular choice like Nodemailer.
After sending the login link, you can display a verification page to the user, informing them that a login link has been sent to their email and providing instructions on what to do next.
To complete the magic link authentication flow, create a login page where users can enter their email address to receive the magic link. The signIn function from next-auth/react is called with the “email” provider and the entered email address, and the callbackUrl parameter specifies the page to redirect to after successful authentication.
Additional reading: 404 Nextjs
Sources
- https://next-auth.js.org/configuration/nextjs
- https://nextjs.org/docs/pages/building-your-application/authentication
- https://blog.logrocket.com/auth-js-client-side-authentication-next-js/
- https://www.pedroalonso.net/blog/authentication-nextjs-with-nextauth/
- https://grafbase.com/guides/username-and-password-authentication-with-next-auth
Featured Images: pexels.com