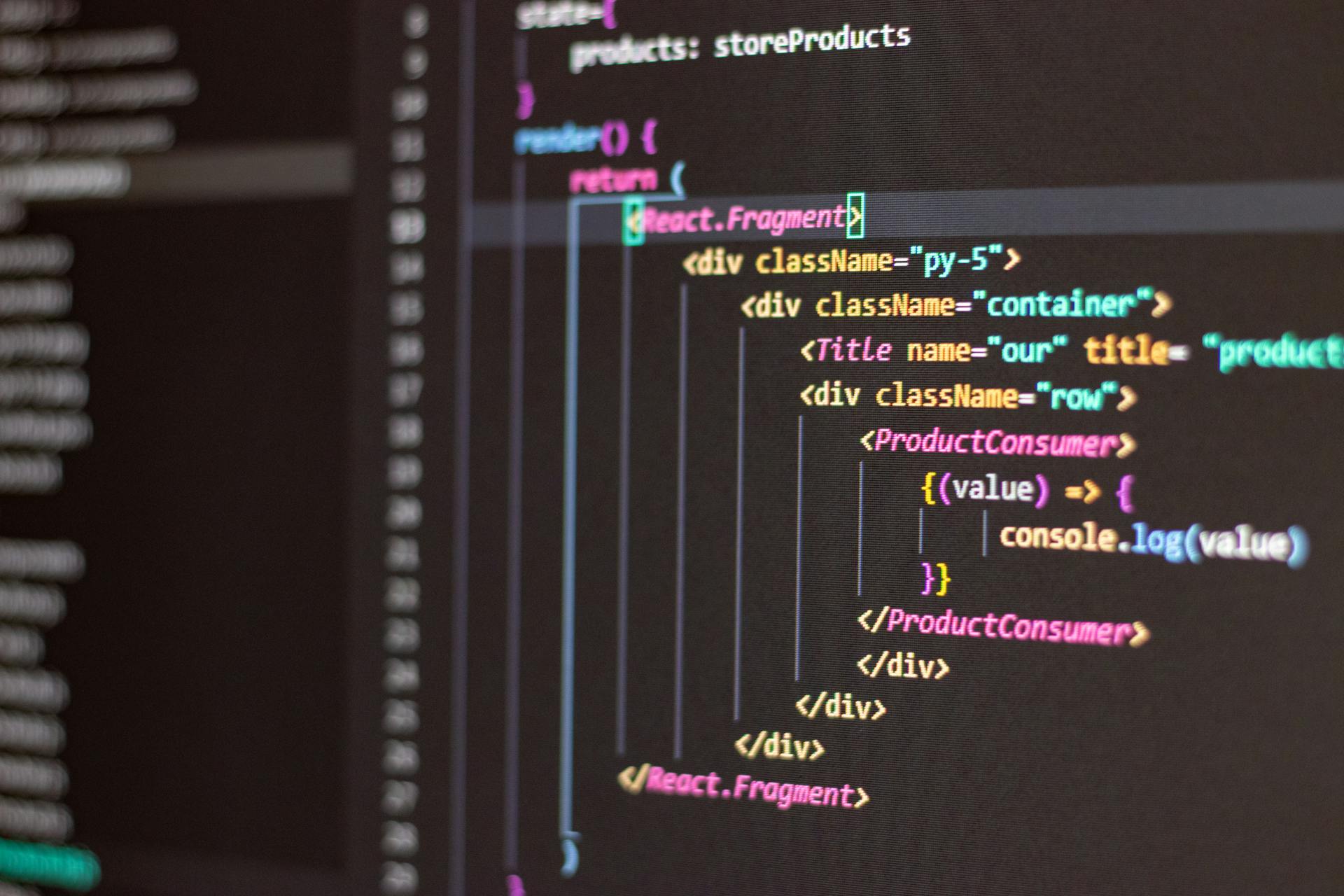
Next Js Provider for State Management and Authentication is a crucial aspect of building scalable and maintainable web applications.
Redux is a popular state management library that can be used with Next Js, providing a predictable and consistent way to manage global state.
Context API is another option for state management, offering a more lightweight and flexible approach.
Next Js provides built-in support for authentication through the use of Next Auth, a library that simplifies the process of adding authentication to your application.
Explore further: Next Js Component Library
Adding Authentication
To add authentication to your Next.js project, you'll need to create a GitHub OAuth app and obtain a Client ID and Client Secret. This is done by registering a new app on GitHub and filling out the form with your application's name, homepage URL, and authorization callback URL.
You can use other built-in OAuth providers that NextAuth supports, such as Google. To configure NextAuth.js, create a [...nextauth].js file in your pages/api/auth directory and add the following code, replacing the clientId and client secret with your own.
Worth a look: Next Js Stripe
Here's a list of the environment variables you'll need to set up:
- NEXTAUTH_URL: your project's base URL
- NEXTAUTH_SECRET: a long, random, and confidential string used for session encryption
- clientId and client secret: obtained from the GitHub OAuth app
You can create a NEXTAUTH_SECRET by running the command `openssl rand -base64 32` on your terminal. Then, create a .env.local file in the root folder of your application and store the environment variables. This ensures you don't expose your environment's secrets.
By following these steps, you'll be able to add authentication to your Next.js project using NextAuth.js.
API and Server-Side Rendering
Authentication and authorization are crucial for web applications, and Next.js offers a robust solution for handling them with server-side rendering.
In Next.js, authentication verifies a user's identity, while authorization determines what a user can access or do with the application.
This ensures users' data are secure and private, and they get personalized experiences tailored to their accounts.
Render is a cloud platform that supports deploying Next.js applications, offering a hybrid hosting model that combines static hosting with serverless functions.
This gives you more flexibility and only charges you for actual usage, eliminating unnecessary costs.
Worth a look: Next Js Hosting
Authorization with Server-Side Rendering
Authorization with Server-Side Rendering is a crucial aspect of ensuring that users have access to the right information and features in an application.
Middleware functions in Next.js allow you to run code before a request is completed, and can be used for authentication, authorization, logging, error handling, and data parsing.
To restrict access to specific users, you can create a middleware function that checks the user's role obtained from the authentication token.
In Next.js, middleware is a function that runs before handling a request, allowing you to intercept and manipulate incoming HTTP requests and outgoing responses.
You can use the withAuth function from the next-auth/middleware package to define middleware that checks if the user is authorized to access a particular page.
The withAuth function takes a middleware function that takes a req as a parameter, and checks if the pathname of the requested URL matches a specific pattern.
If the user is not authorized, the middleware function returns a response with a message indicating permission denial.
Check this out: Next Js Function Handlers to Filter Data
To test this, you can set the token.role to "member" in the jwt callback function, and when a user clicks on the admin page, it should show Permission denied.
In a server-side component, you can access the user session using the getServerSession function, which fetches the user session on the server side before rendering the page.
If a user session is found, the user object is extracted from the session and passed as props to the Protected component.
A different take: Nextjs Pathname Server Component
Render
Render is a versatile cloud platform that offers great support for deploying Next.js applications.
It uses a hybrid hosting model that combines static hosting with serverless functions, giving you more flexibility.
You pay only for what you use with Render, so you don't have to worry about unnecessary costs.
This pay-per-use pricing is based on actual consumption of your resources.
Render includes developer-friendly features like automatic scaling and global HTTP/2 routing, which help improve productivity during the software development process.
A global content delivery network helps ensure your site loads quickly.
A fresh viewpoint: Nextjs Loader
Railway App
Railway App is a complete cloud platform that makes it easy to build, launch, and monitor applications. It provides quick deployments, easy scaling, and simple management.
You can deploy your app instantly with Railway.app, which means you don't need to wait for a long time to see your app live. This is especially useful for developers who want to test and iterate quickly.
Scaling your app with Railway.app is effortless, which means you can handle increased traffic without worrying about your app slowing down. This is due to Railway's ability to automatically adjust resources as needed.
Developers can easily trigger builds and deploy them to a live URL just by pushing code to a repository. This means you can automate the deployment process and focus on more important tasks.
Railway keeps your secrets safe and lets you access them easily through the terminal using the CLI. This is a big plus for developers who need to manage sensitive information.
Railway is a great choice for hosting Next.js applications due to its strong commitment to quality and developer-friendly features.
Recommended read: Deploy Nextjs on Render
The App Router
The App Router is a game-changer for Next.js developers. It was introduced in version 13 and is built on React Server Components.
The App Router supports shared layouts, which can be a huge time-saver for developers who need to reuse the same layout across multiple pages.
See what others are reading: Next Js Multi Tenant App
Using Sessions in Client-Side Pages
To use sessions in client-side pages, you need to add NextAuth's SessionProvider to your layout.
This is because, as we learned from Example 1, you can't access session data directly in client-side components or pages.
You need to wrap your children with the Providers component, which is imported from the client component provider.tsx.
This is because the layout.tsx is rendering in server mode, and SessionProvider is a client-side component, which can't be used in server mode.
You can create a client component provider.tsx with the use client directive to fix this issue.
Now you can access session data by using the useSession() hook in all your client pages.
Take a look at this: Iron Session Next Js Middleware
Hosting Providers
When choosing a hosting provider for your Next.js project, you have several options to consider. Back4app is a cloud hosting platform designed specifically for Next.js applications, offering a reliable and secure place to host your projects.
Back4app's interface is user-friendly and easy to understand, making it simple to control application deployments. You can also grow your hosting resources as your traffic and usage increase.
One great benefit of Back4app is its thorough automation system, which ensures client data is always protected and never compromised. Another advantage is the 24/7 customer support, which is a big plus for developers.
Netlify is another excellent option for deploying your Next.js project. It supports Incremental Static Regeneration, which speeds up builds by only re-building the pages that need updating.
Netlify is easy to use, allowing you to connect your GitHub, Bitbucket, or GitLab account to import any repository. You can also set up automatic deployments once your site is live, so your project will rebuild automatically every time you push code to a branch.
Here are some key hosting providers to consider:
- Back4app: A cloud hosting platform designed for Next.js applications, offering reliability, security, and user-friendly interface.
- Netlify: Supports Incremental Static Regeneration, easy to use, and offers automatic deployments.
- Vercel: A top choice for hosting Next.js applications, offering specialized services, serverless functions, and a global CDN for fast delivery.
- Linode: A leading cloud hosting provider offering fast and flexible VPS hosting with clear pricing and no hidden fees.
Sources
- https://www.makeuseof.com/react-context-nextjs-13-app-directory-state-management/
- https://blog.openreplay.com/authentication-and-authorization-with-ssr-in-nextjs/
- https://blog.back4app.com/next-js-hosting-providers/
- https://medium.com/@rezahedi/using-nextauth-authentication-provider-in-next-js-by-app-router-f50cb23282c9
- https://docs.sentry.io/platforms/javascript/guides/nextjs/
Featured Images: pexels.com