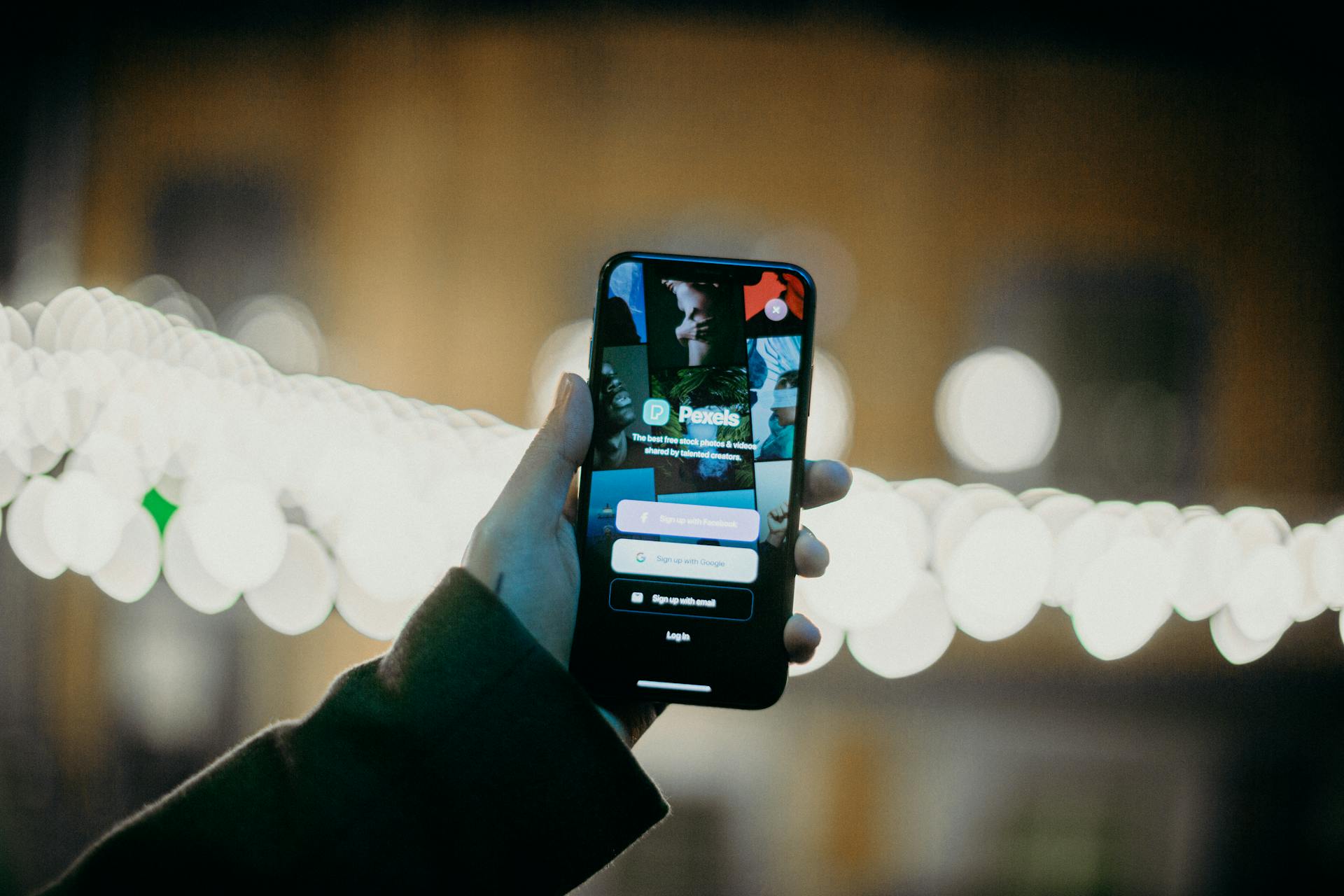
Managing Nextjs Client Session Tokens for Secure Applications requires careful consideration to ensure your application remains secure. To start, it's essential to understand that client session tokens are used to authenticate users and authorize access to protected routes.
Nextjs provides a built-in mechanism for managing client session tokens through the use of cookies. This approach is convenient but also poses security risks if not implemented correctly.
In Nextjs, client session tokens are typically stored in a cookie with a short expiration time, which helps prevent token theft. However, this also means the token must be refreshed periodically to maintain an active session.
If this caught your attention, see: Nextjs Session
Project Setup
To start a Next.js project, create a basic application in a directory of your choice, like "my-app".
Open your terminal and run the command `npx create-next-app my-app` to create the application. The contents of the working directory should then look like a standard Next.js project.
Next, install Next-Auth by running `npm install next-auth` in the terminal.
Create an API route to use the NextAuth package by running the command `npx next generate api` in the pages/api folder.
Create a directory called "auth" inside the pages/api folder to hold the API route.
Inside the "auth" directory, create a file called [...nxtauth].js to hold the API route code.
Expand your knowledge: Nextjs 14 Api Routes
Implementing Authentication
Implementing authentication in Next.js is a crucial step in securing your application. You can use NextAuth.js, an open-source library designed for authentication in Next.js applications.
To start, you need to create a Next.js project and install NextAuth.js using the command `npx create-next-app my-app` and then `npm install next-auth`. NextAuth.js offers a secure and flexible way to handle user logins, signups, and authorization within your Next.js project.
You can configure NextAuth.js by creating a new file called `[...nextauth].js` in the pages directory. In this file, you can define the authentication options, such as the providers array, which includes the GoogleProvider module. You can pass your Client ID and Secret to the GoogleProvider module as environment variables.
You might enjoy: Auth Middleware Nextjs
Once you've configured NextAuth.js, you can use the `useSession` hook in your components to check if the user is authenticated. The `useSession` hook returns an object with two properties: `data` and `status`. The `data` property holds information about the authenticated user, and the `status` property can be either "authenticated", "unauthenticated", or "loading".
Here's a summary of the steps to implement authentication in Next.js:
- Create a Next.js project and install NextAuth.js
- Configure NextAuth.js by creating a new file called `[...nextauth].js`
- Define the authentication options, such as the providers array
- Use the `useSession` hook in your components to check if the user is authenticated
By following these steps, you can implement authentication in your Next.js application using NextAuth.js.
Authentication Flow
The OAuth flow is a crucial part of integrating authentication into your Next.js app using NextAuth. It involves multiple HTTP round trips between the client (your Next.js app) and the authorization server and resource server.
NextAuth handles all the HTTP round trips for you, but you need to register your app with the provider (such as Google) and obtain credentials (client ID and secret) to enable the interface.
Here's a breakdown of the OAuth flow:
Take a look at this: Backblaze Client
1. Client (Next.js app) requests authorization from the authorization server.
2. User grants or denies access.
3. Client redirects user to the authorization server for authentication.
4. User authenticates with the authorization server.
5. Authorization server redirects user back to the client with an authorization code.
6. Client exchanges the authorization code for an access token.
7. Client uses the access token to access the user's data on the resource server.
NextAuth generates all the necessary tokens for you and provides components to integrate into your frontend. You'll need to create a simple page for users to interact with to authenticate themselves, including sign-in and sign-out functionality, and route protection on the frontend.
To implement authentication in your Next.js app, you'll need to use the signIn, signOut, and useSession hooks provided by NextAuth. These hooks allow you to get the current user session, which contains information about the authenticated user and the expiry date of the issued JWE token.
For your interest: Next Js Use Client
Authentication in Next.js
Authentication in Next.js is a crucial aspect of building secure and user-friendly applications. Next.js offers two primary approaches to authentication: client-side authentication and server-side authentication.
Client-side authentication is a straightforward approach where users are authenticated on the client-side, typically using cookies or local storage. However, this approach has its limitations, as it can be vulnerable to cross-site scripting (XSS) attacks.
To implement client-side authentication, you can use NextAuth.js, an open-source library designed specifically for authentication in Next.js applications. NextAuth.js offers a secure and flexible way to handle user logins, signups, and authorization within your Next.js project.
Here are some key benefits of using NextAuth.js:
• Secure and flexible authentication
• Handles user logins, signups, and authorization
• Integrates with popular providers like Google and GitHub
• Supports various authentication strategies, including OAuth and JWT
By using NextAuth.js, you can simplify the authentication process and focus on building a robust and user-friendly application.
See what others are reading: Next Js Debug
In Next.js, authentication is typically handled using the `auth` function, which returns an object containing information about the user's session. This object can be used to determine whether the user is authenticated or not.
Here's an example of how to use the `auth` function in Next.js:
```javascript
import { auth } from 'next-auth';
const isAuthenticated = async () => {
const session = await auth.getSession();
return session ? true : false;
};
```
This code checks whether the user is authenticated by retrieving the session object using the `auth.getSession()` method. If the session object is present, the function returns `true`, indicating that the user is authenticated.
By using the `auth` function, you can easily determine whether a user is authenticated or not, making it easier to implement authentication and authorization logic in your Next.js application.
In addition to using the `auth` function, Next.js also provides a `withAuth` middleware function that can be used to restrict access to certain routes or pages based on the user's authentication status.
Here's an example of how to use the `withAuth` middleware function in Next.js:
```javascript
import { withAuth } from 'next-auth/middleware';
Suggestion: Why Use Next Js
export default withAuth(async (req) => {
if (!req.user) {
return new Response('Unauthorized', { status: 401 });
}
// User is authenticated, proceed with the request
});
```
This code uses the `withAuth` middleware function to check whether the user is authenticated before proceeding with the request. If the user is not authenticated, the middleware returns an unauthorized response with a 401 status code.
By using the `withAuth` middleware function, you can easily restrict access to certain routes or pages based on the user's authentication status, making it easier to implement authentication and authorization logic in your Next.js application.
In summary, authentication in Next.js is a crucial aspect of building secure and user-friendly applications. By using NextAuth.js and the `auth` function, you can simplify the authentication process and focus on building a robust and user-friendly application. Additionally, using the `withAuth` middleware function can help you restrict access to certain routes or pages based on the user's authentication status.
Broaden your view: Next Js Protected Routes
Testing and Configuration
To test authentication in your Next.js application, you'll want to set up a small test to ensure everything is working as expected. This involves changing the homepage to check for an active user session.
NextAuth v5 makes it simple to get the session with a single function call auth(). This function runs as a React Server Component by default, so you're getting a check on authentication status on the server before responding with any data.
To protect all pages under the /protected route, you can leverage Next layouts and check if there is a currently active user session. If there is no session, you'll redirect the user to the sign in page.
You can add more information to the session object as needed, especially if you're managing users in a database. The session object will hold information about the session and user.
To configure NextAuth.js, you'll need to create a [...nextauth].js file in your pages/api/auth directory and add the required code. This includes configuring the GitHub provider with your clientId and client secret obtained from the GitHub OAuth app.
Using your own NEXTAUTH_SECRET variable is highly recommended for consistent session and token management in production environments. To generate your own NEXTAUTH_SECRET, enter the command openssl rand -base64 32 on your terminal.
For another approach, see: Next Js Session
NextAuth.js
NextAuth.js is an open-source library designed for authentication in Next.js applications. It offers a secure and flexible way to handle user logins, signups, and authorization within your Next.js project.
To start with NextAuth.js, you need to create a Next.js project by running the command `npx create-next-app my-app` on your terminal. This will set up a basic Next.js project for you to work with.
NextAuth.js can be installed in your project by running the command `npm install next-auth` or `yarn add next-auth` on your terminal. You can then configure it by creating a `nextauth.js` file in the `pages/api/auth` directory.
To configure NextAuth.js, you need to create a `nextauth.js` file in the `pages/api/auth` directory and add the following code:
```javascript
import NextAuth from 'next-auth';
import GitHub from 'next-auth/providers/github';
export default NextAuth({
// Configure one or more authentication providers
providers: [
GitHub({
clientId: process.env.GITHUB_ID,
clientSecret: process.env.GITHUB_SECRET,
}),
],
});
```
This code configures NextAuth.js with the GitHub provider. The `clientId` and `clientSecret` are obtained from the GitHub OAuth app you registered earlier.
NextAuth.js uses JSON Web Tokens (JWTs) to securely manage user sessions and authorization. However, these tokens are not persistent across server restarts, so it's recommended to use your own `NEXTAUTH_SECRET` variable for consistent session and token management.
You can generate your own `NEXTAUTH_SECRET` by running the command `openssl rand -base64 32` on your terminal. Then, create a `.env.local` file in the root folder of your application and store the environment variables.
Keywords
Keywords play a crucial role in Next.js client session token management. They help identify the session token in client-side code.
Some common keywords used in Next.js client session token management include 'session', 'token', 'client', and 'cookie'.
Worth a look: Azure Access Token
Frequently Asked Questions
How to getSession in next auth server?
To get a session in NextAuth.js on the server, call the getSession() method, which returns a promise with a session object or null if no session exists. This method can be called at /api/auth/session.
How to get token next auth?
To get a token with NextAuth, set the NEXTAUTH_URL environment variable and pass the same secret value to the getToken() function as specified in your auth API. This allows you to access the JWT cookie in your application.
Sources
- https://www.telerik.com/blogs/how-to-implement-google-authentication-nextjs-app-using-nextauth
- https://blog.logrocket.com/auth-js-client-side-authentication-next-js/
- https://stackademic.com/blog/authentication-in-next-js-with-auth-js-nextauth-5
- https://www.npmjs.com/package/next-auth
- https://blog.openreplay.com/authentication-and-authorization-with-ssr-in-nextjs/
Featured Images: pexels.com