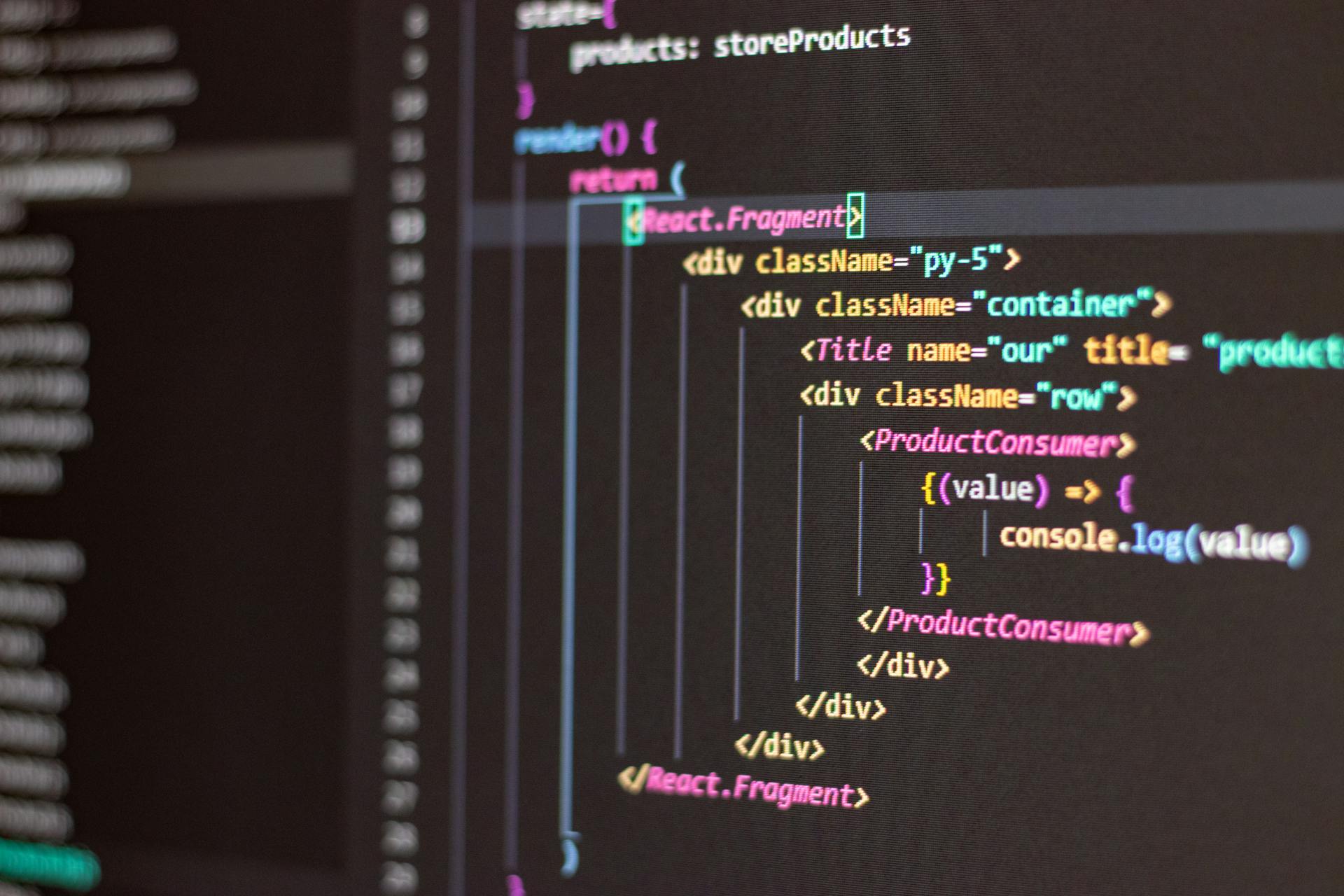
Next JS Protected Routes can be a bit tricky to set up, but don't worry, I've got you covered.
To create a Next JS Protected Route, you need to use the `getServerSideProps` method to check if the user is authenticated before rendering the page. This method runs on the server and allows you to fetch data and authenticate users before sending the response to the client.
In Next JS, you can use the `next-auth` library to handle authentication, which provides a simple way to authenticate users and protect routes. By using `next-auth`, you can easily add authentication to your Next JS application.
To use `next-auth`, you'll need to install the library and configure it to work with your application. This involves setting up authentication providers, such as Google or GitHub, and handling the authentication flow.
Setting Up Protected Routes
To integrate protected routes with Next.js pages, you simply wrap the page component with the HOC (higher-order component) before exporting it. This will make the page a protected route, only accessible to authenticated users.
You might enjoy: Routing Next Js
You can apply the protected route HOC to specific pages in your Next.js application by wrapping the page component with the HOC before exporting it. This will ensure that only authenticated users can access the page.
Protected routes in Next.js can be implemented using the getServerSideProps function, which is called when the page is being pre-rendered on the server. This function returns an object that determines the action Next.js will take in rendering the component.
To protect a route in Next.js, you can check for the user session data in the getServerSideProps function. If a session is found and still valid, you can proceed to render the component. Otherwise, you can return a redirect property to redirect the user to the login page.
API routes in Next.js can also be protected using authentication. You can check for the user session data in the API route handler function. If a session is found and still valid, you can proceed to handle the request. Otherwise, you can return a "Non-authenticated user" error with a 403 status.
Here are some key facts to keep in mind when setting up protected routes in Next.js:
- Use the getServerSideProps function to check for user session data on the server-side.
- Wrap page components with the HOC to apply protected routes.
- Use the withSession utility function to get the session if it exists in API routes.
- Return a redirect property to redirect the user to the login page if no session is found.
- Return a "Non-authenticated user" error with a 403 status if no session is found in API routes.
By following these steps and key facts, you can effectively set up protected routes in your Next.js application to ensure that only authenticated users can access certain pages and API routes.
See what others are reading: Nested Routes in Next Js
Implementing Authentication
Implementing authentication in a Next.js application involves setting up a system to manage the user's auth state across the application. This typically includes creating a shared context that provides authentication data to your components and building custom hooks for easy access to authentication-related functionality.
To begin, you'll need to install necessary dependencies using npm or yarn, such as next-auth for handling authentication, jsonwebtoken for managing JSON Web Tokens (JWT), and any other libraries specific to your authentication provider or method.
Creating an authentication context allows you to share the user's authentication status and user data throughout your Next.js app without having to prop-drill. This can be achieved by designing a system that can conditionally render components based on the user's authentication status.
Here's an overview of the key components involved in implementing authentication in Next.js:
By using these components, you can create a robust and secure authentication system for your Next.js application.
Installing Necessary Dependencies
To implement authentication effectively, you'll need to set up a new Next.js application if you haven't already.
First, you'll need to install necessary dependencies using npm or yarn. These include packages like next-auth for handling authentication, jsonwebtoken for managing JSON Web Tokens (JWT), and any other libraries specific to your authentication provider or method.
You can use npm or yarn to install these dependencies quickly and efficiently. Next-auth and jsonwebtoken are two of the most commonly used packages for authentication and JWT management.
Installing these packages will help you manage the user's authentication status and secure your api routes, ensuring that only authenticated users can access them.
Related reading: Npm Next Js
Basic Project Structure
To get started with implementing authentication in your Next.js application, you'll need to set up the basic project structure. This involves creating specific directories to store your pages, API routes, React components, and more.
The pages directory is where you'll store your Next.js pages, including your login page, home page, and any other pages you plan to include in your app. Each file in this directory corresponds to a route in your application, such as pages/index.js for the home page.
Additional reading: Nextjs Pages
You'll also need to define your API routes in the pages/api directory. This is where you'll handle server-side logic, such as signing in and out, and checking the user's auth state.
Here's a simple directory layout to get you started:
- /pages - This directory contains your Next.js pages.
- /pages/api - This directory defines your API routes.
- /components - This directory stores your React components.
- /context - If you're using a context for authentication, you'll store your AuthContext here.
- /lib - This is a good place to put reusable libraries and functions.
- /middleware - If you're using Next.js middleware for route protection, you'll define it here.
For example, your project directory might look like this, with each file in the pages directory corresponding to a route in your app.
Creating a Context
Creating a Context is a crucial step in implementing authentication in Next.js. To share the user's authentication status and user data, you can use React's Context API.
You'll need to create a new file for your authentication context, such as AuthContext.js. This file will define your context and a provider component.
The provider component, AuthProvider, is used to wrap your application in _app.js, providing the authentication state to all components. This eliminates the need for prop-drilling.
Additional reading: Next Js Fetch Data save in Context and Next Route
Implementing Secure Authorization
Implementing secure authorization in a Next.js application is crucial for protecting routes and data. This involves creating a system to manage user permissions and access control.
A higher-order component (HOC) can be used to wrap sensitive components and utilize the authentication hook to determine access. This HOC can conditionally render components based on the user's authentication status.
To secure API routes, you can validate the session by checking the user's authentication status. For example, you can use middleware to protect routes and ensure only authenticated users can access them.
Proper OAuth implementation streamlines the user experience and reduces vulnerability risks. This involves storing OAuth tokens securely, using HTTP-only cookies, or encrypting tokens server-side.
To implement logout mechanics, you can use the signOut() method and redirect users after logout. Clearing frontend app state on logout is also recommended.
Next.js offers two common authentication strategies: static generation and server-side authentication. Choosing the right strategy depends on your application's requirements.
To create Next.js API routes, you need to create a subfolder "api" in the "app" directory. Each API route must be protected using middleware to ensure only authenticated users can access them.
Here's an example of how to protect API routes using middleware:
By implementing these measures, you can ensure secure authorization in your Next.js application and protect your routes and data from unauthorized access.
Integrating Supertokens
Integrating Supertokens is a crucial step in creating protected routes in Next.js. You'll need to create a higher-order component (HOC) for protected routes and integrate it with your Next.js pages.
To start, you'll need to create a new file /app/api/auth/[...path]/route.ts. This file will contain the SuperTokens API routes that you'll use to handle authentication. You can add the following code to this file to ensure that SuperTokens is always initialized when any of the API routes are called.
Here's a step-by-step guide to setting up Supertokens:
1. Create a new file /app/api/auth/[...path]/route.ts and add the following code:
- We call ensureSuperTokensInit to make sure SuperTokens is always initialized when any of the SuperTokens API routes are called.
- getAppDirRequestHandler is a helper function that the SuperTokens SDK provides, which adds request parsing, error handling, etc. so that you don't have to manually check for individual API results.
Once you've set up the SuperTokens backend SDK, you can verify that it's working correctly by visiting http://localhost:3000/api/auth/signup/email/[email protected] in your browser.
Next, you'll need to add Supertokens to the frontend of your application. You can use the pre-built UI provided by supertokens-auth-react, or you can build your own UI using the functions exposed by the SDK.
To add the pre-built UI, you'll need to create a new file /app/config/frontend.ts and add the following code:
* Create a /app/components/supertokensProvider.tsx file and add the following contents to it:
+ We check for window not being undefined because we only want to initialize the SuperTokens React SDK on the client side.
+ The SuperTokensWrapper component adds some context to the rest of our application so that we can access the session from anywhere.
Finally, you'll need to modify the /app/layout.tsx file to include the SuperTokensProvider component.
With Supertokens set up on both the backend and frontend, you can now create protected routes in your Next.js application. To do this, you'll need to create a new file /app/auth/[[...path]]/page.tsx and add the following code:
- SuperTokens.canHandleRoute returns true if SuperTokens exposes a component for the current browser URL.
- ThirdPartyEmailPasswordPreBuiltUI are all the pre-built UI components that can handle a specific set of routes.
Now, if you visit http://localhost:3000/auth in your browser, you should see the login page of your project.
Route Protection and Security
Route protection is a crucial aspect of Next.js development, ensuring that sensitive routes and data are accessible only to authorized users.
To protect routes in Next.js, you can use the `getServerSession` function, which handles JWT token validation automatically. This function checks if a user is logged in before allowing access to the API route.
You can also use middleware to handle authentication logic, making it easier to protect API routes without having to re-implement auth logic repeatedly.
In Next.js, API routes can be protected by validating the session. For example, you can create a middleware file that applies to specific routes, such as `/api/users` and `/api/admin`.
To implement seamless redirection post-authentication, you can store the intended destination before redirecting the user to the login page. After successful authentication, you can redirect the user back to their intended destination using the `router` object from Next.js.
Here are some key takeaways for protecting routes in Next.js:
- Use `getServerSession` to check if a user is logged in before allowing access to API routes.
- Implement middleware to handle authentication logic and protect API routes.
- Store the intended destination before redirecting the user to the login page for seamless redirection post-authentication.
- Use the `router` object from Next.js to redirect the user back to their intended destination after successful authentication.
By following these best practices, you can ensure that your Next.js application is secure and protected from unauthorized access.
Using NextAuth.js
NextAuth.js is an open-source authentication library designed specifically for Next.js applications, handling login flows and session management seamlessly.
It supports many popular providers like Google, Facebook, Twitter, GitHub, and more, allowing users to sign in with their existing accounts.
To add OAuth-based authentication with NextAuth.js, you can follow the steps outlined in the documentation.
With NextAuth.js, you get a full-featured authentication system out of the box, handling social login, email and password authentication, encrypted sessions, and custom database integration.
Here are some key benefits of using NextAuth.js:
- Social login with Google, Facebook, Twitter, etc.
- Email and password authentication
- Encrypted sessions + JWT tokens
- Custom database integration
By using NextAuth.js, you can easily implement secure social login using its authentication flow, with just a few lines of code.
This library provides a robust and scalable solution for authentication in Next.js applications, making it a great choice for building secure and user-friendly applications.
Explore further: Auth Middleware Nextjs
Managing User Sessions
You can persist user sessions after login using NextJS iron-session, a popular solution that handles encryption and storage of session data.
On a similar theme: Nextjs Client Session Token
To get started, install the iron-session library, which allows you to store session data in a cookie on the client side in an encrypted format.
One of the benefits of using iron-session is stateless sessions, which means no need for server-side session storage.
You can customize session options like cookie name, TLS settings, and more as needed.
This persists user sessions across requests, and you can also store custom user data in sessions, enable offline access with refresh tokens, and more.
Here are some key points to keep in mind when implementing iron-session:
- Server-side session handling is required
- Session data is stored in a cookie on the client side
- Session data is encrypted for security
- Session options can be customized as needed
Best Practices and Troubleshooting
Implementing OAuth authentication in Next.js applications can be a bit tricky, but don't worry, we've got you covered. Integrating OAuth authentication provides many benefits, such as simplified login flows and access to external API data.
Properly storing access tokens is crucial for security, so make sure to store them securely. This can be done by using a secure storage solution like a library or a framework that handles token storage for you.
To manage user sessions, consider implementing a system that revokes tokens when a user logs out. This ensures that even if an attacker gets access to a user's token, they won't be able to use it after the user logs out.
OAuth authentication introduces additional security considerations, so it's essential to handle errors and exceptions properly. This includes logging and reporting errors to identify and fix issues quickly.
Next.js OAuth authentication can be customized to fit your application's needs, so don't be afraid to experiment and try out different approaches. Remember to test thoroughly and ensure that your implementation is secure and reliable.
Admin Access Control
Admin Access Control is a crucial aspect of securing your Next.js API. Restricting admin routes is now a standard practice, as the API routes are now protected.
In Next.js, this protection is built-in, so you don't need to worry about implementing additional security measures. The API routes are automatically secured.
To ensure that only authorized personnel can access admin routes, you can use the built-in Next.js API protection.
Sources
- https://www.dhiwise.com/post/implementing-next-js-protected-routes-a-step-by-step-guid
- https://daily.dev/blog/authentication-in-nextjs
- https://supertokens.com/blog/adding-login-to-your-nextjs-app-using-the-app-directory-and-supertokens
- https://nextjsstarter.com/blog/nextjs-oauth-integration-your-step-by-step-guide/
- https://blog.tericcabrel.com/protect-your-api-routes-in-next-js-with-middleware/
Featured Images: pexels.com