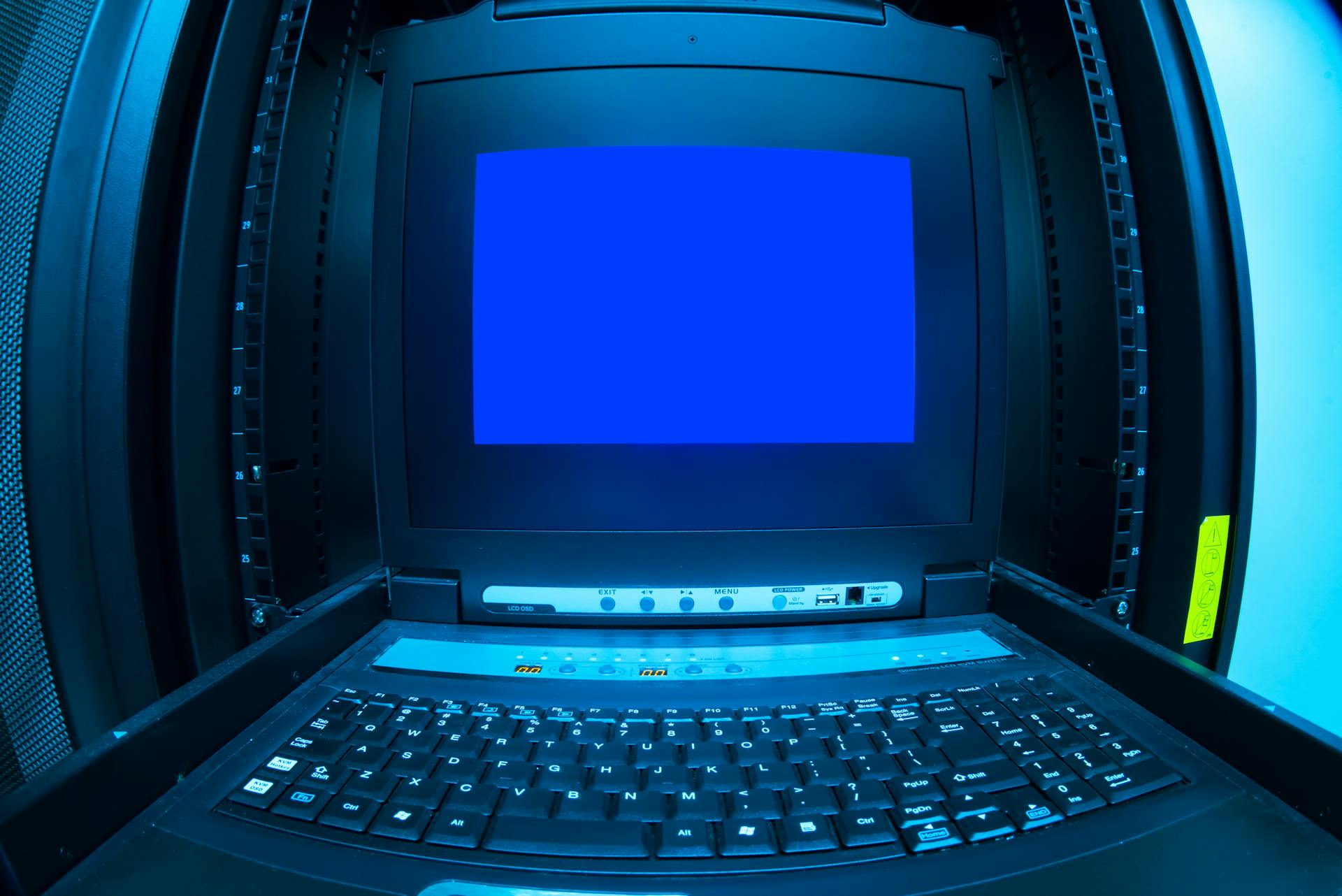
Azure Token Management and Authentication is a crucial aspect of securing your Azure resources. You can use Azure Active Directory (Azure AD) to manage tokens and authenticate users.
Azure AD provides a scalable and secure way to manage identities and access to Azure resources. It integrates with Azure services such as Azure Storage and Azure SQL Database.
To authenticate users, Azure AD issues tokens that contain user identity information. These tokens are used to access Azure resources without the need for traditional username and password combinations.
Azure AD also supports multi-factor authentication (MFA) to provide an additional layer of security. This can be particularly useful for sensitive resources that require an extra level of protection.
A different take: Manage Azure
Azure Token Validation
Azure Token Validation is a crucial step in ensuring the security and integrity of your Azure AD applications. You should validate both the signature and claims in the id_token to prevent token tampering and ensure the token was issued to your app.
To validate id_tokens, you can use open source libraries available in your preferred language, as recommended by Azure. These libraries will help you properly use the validation logic.
Before validating the claims in an id_token, you should check the following:
- The Audience claim to verify the id_token was intended for your app.
- The Not Before and Expiration Time claims to ensure the id_token hasn't expired.
- The Issuer claim to verify the token was issued by Azure AD.
- The Nonce to prevent token replay attacks.
You can also refer to the OpenID Connect specification for a full list of validations to perform on an id_token.
Azure Token Configuration
Azure Token Configuration is a crucial aspect of working with Azure services. You can configure Azure Token lifetimes to meet your industry's security and compliance requirements.
The lifetime of OAuth 2.0 bearer tokens used to gain access to protected resources can be set between 5 and 1440 minutes. This includes the default lifetime of 60 minutes. The lifetime of refresh tokens can be set between one day and 90 days. This determines the maximum time period before which a refresh token can be used to acquire a new access or ID token.
Additional reading: Azure Devops Personal Access Token
To configure these lifetimes, you can use Azure AD B2C properties. The following table summarizes the properties and their valid ranges:
These lifetimes can be set to meet specific use cases, such as allowing users to stay signed in to mobile applications indefinitely, or meeting industry security and compliance requirements.
App Secret
In Azure Active Directory, an App Secret is a string used for securing communication between an application and Azure AD. It's a credential that the application uses along with its client ID to authenticate itself.
This App Secret is a crucial component in Azure Token Configuration, as it enables the application to access Azure resources, such as APIs or other services, on behalf of a user or a system. It's also known as a client secret.
The App Secret is a string that should be kept confidential, as it's sensitive information that could compromise the security of the application and the resources it accesses.
Intriguing read: Azure App Insights vs Azure Monitor
Internal HTTP API
Internal HTTP API can be a bit tricky to navigate, but don't worry, I've got you covered. MSI_ENDPOINT is an alias for IDENTITY_ENDPOINT, and MSI_SECRET is an alias for IDENTITY_HEADER.
To find IDENTITY_HEADER and IDENTITY_ENDPOINT, you'll need to check your environment variables, specifically the "env" section. You can do this by checking the following URLs:
- https://graph.microsoft.com
- https://management.azure.com
- https://storage.azure.com
- https://vault.azure.net
These are the endpoints you'll need to use when making requests to Azure AD B2C. If you're using PowerShell, you can use the following commands to make a request:
- `curl "$IDENTITY_ENDPOINT?resource=https://management.azure.com&api-version=2017-09-01"-Hsecret:$IDENTITY_HEADER`
- `curl "$IDENTITY_ENDPOINT?resource=https://vault.azure.net&api-version=2017-09-01"-Hsecret:$IDENTITY_HEADER`
If you're using an Azure Function in Python, you can use the following code to make a request:
- `IDENTITY_ENDPOINT=os.environ['IDENTITY_ENDPOINT']`
- `IDENTITY_HEADER=os.environ['IDENTITY_HEADER']`
- `cmd='curl "%s?resource=https://management.azure.com&apiversion=2017-09-01" -H secret:%s'%(IDENTITY_ENDPOINT,IDENTITY_HEADER)`
- `val=os.popen(cmd).read()`
Remember to replace the placeholders with the actual values from your environment variables.
Pass-Through
Pass-Through allows you to pass the access token from an identity provider to your application. This is a convenient way to authenticate users without having to manage multiple authentication flows.
Azure AD B2C currently only supports passing the access token of OAuth 2.0 identity providers, which include Facebook and Google. For these providers, you can enable a claim in your user flow to pass the token through to your registered applications.
Intriguing read: Identity Provider Azure
To take advantage of passing the token as a claim, your application must be using a recommended user flow. This ensures that the access token is passed correctly and can be used for authentication.
Azure AD B2C receives an access token from an identity provider and uses it to retrieve information about the user. This process is seamless and transparent to the user.
You can enable a claim in your user flow to pass the token through to your registered applications. This is a simple and effective way to authenticate users and reduce the complexity of your authentication flows.
Configuration
Azure Token Configuration is a powerful tool that allows you to manage the lifetimes of security tokens emitted by Azure AD B2C. The configuration properties are used to control the access and ID token lifetimes, as well as the refresh token lifetimes.
The Access & ID token lifetimes can be set to a minimum of 5 minutes and a maximum of 1440 minutes, with a default of 60 minutes. This means you can choose how long you want users to stay signed in to your application.
For more insights, see: Azure Clientid
You can also set the Refresh token lifetime to a minimum of one day and a maximum of 90 days, with a default of 14 days. This determines how long a refresh token can be used to acquire a new access or ID token.
The Refresh token sliding window lifetime can be set to a minimum of one day and a maximum of 365 days, with a default of 90 days. This feature is only available when the switch is set to Bounded and is used to force users to reauthenticate after a certain period of time.
Here's a summary of the configuration properties:
Azure Token Claims
Azure Token Claims are essential for verifying the authenticity and legitimacy of tokens. They ensure that the token was intended for your application and hasn't expired.
The claims to be checked include the audience, not before and expiration time, issuer, and nonce. The audience claim verifies that the token was meant for your application, while the not before and expiration time claims ensure the token hasn't expired. The issuer claim verifies that the token was issued by Azure AD, and the nonce claim helps prevent token replay attacks.
Here are the expected values for these claims:
Claims
Claims are a crucial part of Azure token validation. They contain information about the user, the application, and the authentication process.
The Audience claim verifies that the ID token was intended to be given to your application. This is essential to prevent unauthorized access.
The Not Before and Expiration Time claims ensure that the ID token has not expired. This prevents tokens from being used after a certain time period.
The Issuer claim verifies that the token was indeed issued to your application by Azure AD. This ensures that the token is genuine and not tampered with.
The Nonce claim helps prevent token replay attacks by including a unique value in the token.
Here are the expected values for these claims:
These claims are essential for ensuring the security and integrity of your Azure token validation process.
Types of
Azure AD B2C supports the OAuth 2.0 authorization protocol, which makes use of both access_tokens and refresh_tokens. It also supports authentication and sign-in via OpenID Connect, which introduces a third type of token, the id_token.
Each of these tokens is represented as a "bearer token", a lightweight security token that grants the “bearer” access to a protected resource.
Bearer tokens do not have a built-in mechanism for preventing unauthorized parties from using them, so they must be transported in a secure channel such as transport layer security (HTTPS).
If a bearer token is transmitted in the clear, a man-in the middle attack can be used by a malicious party to acquire the token and use it for an unauthorized access to a protected resource.
Many of the tokens issued by Azure AD B2C are implemented as Json Web Tokens, or JWTs, which are a compact, URL-safe means of transferring information between two parties.
The information contained in JWTs are known as "claims", or assertions of information about the bearer and subject of the token.
Azure Token Lifetimes
Azure Token Lifetimes are a crucial aspect to understand when working with Azure tokens. They can help you develop and debug apps more effectively.
Id_Tokens are valid for 1 hour. This means your web app can use the same lifetime in maintaining its own session with the user.
A single refresh token is valid for a maximum of 14 days. However, it may become invalid at any time for any number of reasons.
Authorization codes are short-lived and should be immediately redeemed for access_tokens, id_tokens, and refresh_tokens when they are received. They are only valid for 5 minutes.
Here's a summary of the token lifetimes:
Azure Token Installation
To install the Azure token, you'll need to connect to your Azure account using the Az PowerShell module. This can be done by logging in with your credentials, which involves creating a PSCredential object with your username, password, and tenant name.
The Az PowerShell module provides two methods for logging in: using credentials and using a service principal secret. To use credentials, you'll need to create a PSCredential object with your username, password, and tenant name, and then use the Connect-AzAccount cmdlet to log in.
Check this out: How to Connect to Aks from Azure Portal
Alternatively, you can use a service principal secret to log in, which involves creating a PSCredential object with your app ID and secret, and then using the Connect-AzAccount cmdlet with the -ServicePrincipal parameter.
Once you're logged in, you can get a token using the Get-AzAccessToken cmdlet. This cmdlet takes a resource URL as an argument, and returns an access token that you can use to authenticate with Azure.
Here are the steps to get a token:
- Use the Get-AzAccessToken cmdlet with the -ResourceUrl argument set to the URL of the resource you want to access (e.g. https://graph.microsoft.com).
- Use the Get-AzAccessToken cmdlet with the -ResourceTypeName argument set to the name of the resource type you want to access (e.g. MSGraph).
Azure Token Testing
Azure Token Testing is a crucial step in ensuring that your Azure applications are functioning correctly. You can test Azure tokens by using the az cli command az account get-access-token.
To get an access token using az cli, you can use the command az account get-access-token, or az account get-access-token --resource-type aad-graph. You can also use az login to login with credentials, and then use az account get-access-token to get the access token.
Related reading: Azure Account
Alternatively, you can use Azure Powershell to test Azure tokens. To login with credentials, you can use the command Connect-AzAccount -Credential $creds, where $creds is a PSCredential object created with the username, password, and tenant name. To get an access token, you can use the command (Get-AzAccessToken -ResourceUrl https://graph.microsoft.com).Token.
You can also test your logic app by manually starting the workflow and checking the outputs of the actions. To do this, select Run on the designer toolbar, and then review the outputs after the workflow runs successfully.
Here are the steps to get an access token using az cli and Azure Powershell:
Azure Token User Management
Azure Token User Management is a crucial aspect of working with Azure tokens. You can log in to Azure using the az cli with your credentials, which involves using the az login command with your username and password.
To get a token, you can use the az account get-access-token command. Alternatively, you can use the az ad signed-in-user show command, which is the Whoami equivalent.
Consider reading: How to Use Windows Azure
When working with Azure PowerShell, you can log in using your credentials by converting your password to a secure string and creating a new PSCredential object. This can be done using the ConvertTo-SecureString and New-Object cmdlets.
You can also log in using a service principal secret by creating a new PSCredential object with the secret and specifying the tenant ID. To get a token, you can use the Get-AzAccessToken cmdlet with the resource URL or resource type.
Issue a user access token by adding a new action in Azure and selecting the "Issue a user access token" action. You can then specify the token scope, such as VoIP or chat, and use the User ID output from the previous Create a user step.
To revoke user access tokens, you can add a new action in Azure and select the "Revoke user access tokens" action. You can then specify the User ID to revoke all user access tokens for that user.
You might enjoy: Application Id Azure
Azure Token Azure AD B2C
Azure AD B2C is a key part of Azure token, emitting several types of security tokens in each authentication flow.
Azure AD B2C emits several types of security tokens, including those used in authentication flows.
These tokens have a specific format and security characteristics, which are crucial for ensuring their integrity and authenticity.
The format of these tokens is described in the Azure AD B2C Preview: Token Reference document.
Each type of token contains unique information, such as the user's identity and authentication details.
This information is essential for various purposes, including authentication, authorization, and account management.
Broaden your view: Azure Auth Json Website Azure Ad Authentication
Frequently Asked Questions
What is an Azure token?
An Azure token is a JSON Web Token (JWT) that contains information about the user, used for authentication and secure access to resources. It's a digital token that verifies a user's identity and grants them access to protected resources.
How to get the Azure access token?
To get the Azure access token, request an authorization code by launching a browser window and logging in with your Azure user credentials. Use this code to acquire the Microsoft Entra ID access token.
What is the difference between Azure ID token and access token?
ID tokens provide user information, while access tokens grant authorization to access protected resources. Use ID tokens for UX and data storage, and access tokens for authentication and API calls
Sources
- https://swisskyrepo.github.io/InternalAllTheThings/cloud/azure/azure-access-and-token/
- https://learn.microsoft.com/en-us/azure/communication-services/quickstarts/identity/access-tokens
- https://github.com/Huachao/azure-content/blob/master/articles/active-directory-b2c/active-directory-b2c-reference-tokens.md
- https://learn.microsoft.com/en-us/azure/api-management/validate-azure-ad-token-policy
- https://learn.microsoft.com/en-us/azure/active-directory-b2c/tokens-overview
Featured Images: pexels.com