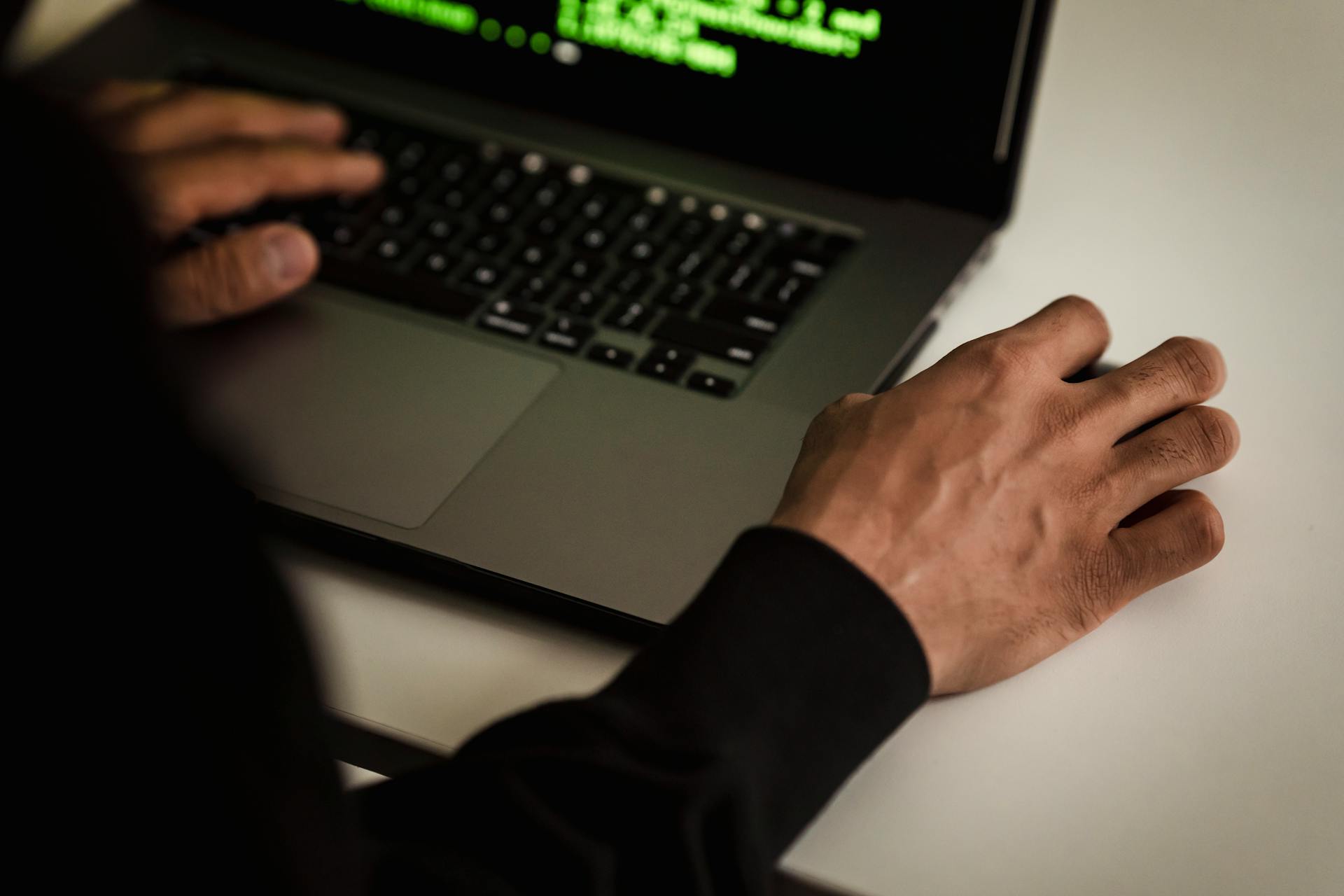
Nextjs Nested Layout is a powerful feature that allows you to create complex layouts within your Next.js application. This feature is particularly useful for building applications with a lot of nested content.
To get started with Nextjs Nested Layout, you'll need to create a new page component and use the `useRouter` hook to access the router object. This will allow you to navigate between different parts of your application.
Nextjs Nested Layout uses a hierarchical structure to organize your components, making it easier to manage complex layouts. This structure is composed of a main component and several child components.
By using Nextjs Nested Layout, you can create dynamic and responsive layouts that adapt to different screen sizes and devices. This is achieved through the use of flexible grid systems and responsive design principles.
Consider reading: Fluid Layout
Creating a Nested Layout
To create a nested layout in Next.js, you need to nest a new layout within the root layout. This is different from simply wrapping the active route within the root layout, which only works for a single layout throughout the application.
Readers also liked: Nextjs Layout
You can create a nested layout by creating a new layout file, such as components/DashboardLayout.js, which exports a component that returns a shared UI for specific pages.
In each of the relevant page files, you need to apply a getLayout property and return the desired layout tree, nesting the new layout within the root layout.
Creating a nested layout in the Next.js App Router is incredibly easy and intuitive, as you can simply add a layout.js file inside the relevant folder to define the UI.
To achieve this, you'll need to use route groups to isolate specific route segments from shared layouts, allowing you to design personalized layouts for certain pages.
The children prop in the layout file serves as a placeholder for the page content, making it easy to render multiple views within a layout.
You can implement the root layout in the app router by overriding the app/layout.js file, which is used to initialize pages, and adding global CSS files or maintaining state when navigating between pages.
By using React components as the building blocks of your nested layouts, you can define new components that include shared layouts for specific sections of your application and use them to wrap relevant page components.
A unique perspective: Nextjs Can Layouts Be Server Components
Understanding the Concept
Nested layouts in Next.js are particularly useful for large applications with complex UI structures. They allow layouts to be embedded within each other.
The key to creating nested layouts is to understand how to compose React components to reflect the nested nature of your page's rendered markup. This means defining a specific structure for a group of pages or a particular section of your application.
Nested layouts enable you to define a structure for a user dashboard or an admin area, for example. Each nested layout can have its own layout file or be defined within the same js file, depending on the complexity and reusability requirements.
To create a nested layout, you start with your root layout and then add additional layout components that wrap around specific parts of your content. This ensures a consistent structure and style across your entire application.
The root layout in Next.js is implemented in the app router, specifically in the app/layout.js file. You can override this file to control page initialization and add global CSS files.
Curious to learn more? Check out: Tailwind Css Nesting
Implementing Nested Layout
To implement a nested layout in Next.js, you need to create multiple layout components and nest them as needed. Each layout component represents a specific section or module of the application.
You can start by defining a layout component that includes a header, main content area, and a footer. This layout component can be used as a starting point for your nested layouts. For example, you can define a LayoutOne component that includes a header, main content area, and a footer.
To create a nested layout, you can import both LayoutOne and LayoutTwo components and nest them within each other. This will result in a layout hierarchy that represents a dashboard page with a header, sidebar, and the main content area. By using nested layouts, you can create complex UI structures while keeping the codebase modular and maintainable.
Take a look at this: Next Js Components Folder
Implementing in Next.js
Implementing nested layouts in Next.js is a breeze, thanks to its flexible and modular architecture. You can create multiple layout components and nest them as needed, each representing a specific section or module of your application.
To start, you'll need to define a layout component, such as LayoutOne, which includes a header, main content area, and footer. This layout component represents one section of your application.
By importing and nesting these layout components within each other, you can create complex UI structures while keeping your codebase modular and maintainable. For example, you can nest LayoutOne within LayoutTwo to create a dashboard page with a header, sidebar, and main content area.
In Next.js, you can also implement the root layout in the app router by overriding the app/layout.js file. This allows you to add global CSS files and maintain state when navigating between pages.
To create a nested shared layout, you'll need to add a layout.js file inside the dashboard folder to define the UI. This layout will be nested within the root layout and will wrap all the pages in the /dashboard/* route segment, providing a more specific and targeted UI for those pages.
You can also use React components to create nested layouts. For instance, you can define a DashboardLayout component that includes the shared layout for the dashboard section and use it to wrap the relevant page components.
If this caught your attention, see: Nextjs Google Fonts
By using the children prop, you can pass page content within layouts and create a hierarchy of components that reflects the structure of your application's UI. This technique is essential for maintaining a clean and organized codebase, especially when dealing with nested layouts.
In some cases, you might need to create a custom layout for a specific route segment, like the Newsletter page. To do this, you can employ route groups to isolate that specific route segment from the shared layouts.
Finally, you can mix in client components to create a wrapper around the Link component and import that into your root layout. This allows you to use the benefits of server components throughout your application while still having a client component for the root layout.
Worth a look: Nextjs Components Library
Loading States
Loading States are a powerful feature that allows you to define a loading state at any level of your app.
You can define a loading state at any level of your app, and it will apply to any components further down the file tree. This means you can create a loading component at the root of the app that will apply to all other components.
Here's an interesting read: Nextjs Multi Tenant App
A loading state defined at the /app/games/ level will only be selected in or below this route.
This allows you to have different loading states for different parts of your app, making it easier to manage and customize.
You can include a simple loading component at the root of the app, which will apply to all other components, just like in the example.
For more insights, see: Next Js Chat
Managing Nested Layout
To create a desired layout tree in Next.js, you need to manage nested layouts. This allows you to create a layout hierarchy that reflects the structure of your user interface.
Nested layouts in Next.js enable granular control over different sections or route segments within your application. By managing nested layouts, you can provide a tailored experience for each part of your application.
To implement nested layouts, you need to create multiple layout components and nest them as needed. Each layout component represents a specific section or module of the application.
For another approach, see: Can Nextjs Be Used on Traditional Web Application
Here's a breakdown of the steps to implement nested layouts:
- Create a new layout file called `components/DashboardLayout.js` that exports a component with a shared UI for pages.
- Apply the `getLayout` property to each page component under the `/dashboard/*` route and return the desired layout tree.
- In the `app` directory, define routes or nested routes with a `page.js` file to render the respective UI.
- Implement the root layout in the `app` router by overriding the `app/layout.js` file to control page initialization.
- Nest layout components within each other to create a complex UI structure while keeping the codebase modular and maintainable.
Here's an example of how to nest layout components:
- Define a layout component named `LayoutOne` with a header, main content area, and footer.
- Define another layout component named `LayoutTwo` with a sidebar and main content area.
- Import both `LayoutOne` and `LayoutTwo` components and nest them within each other to create a dashboard page with a header, sidebar, and main content area.
Customizing the Layout
You can create a nested layout for pages under the /dashboard/* route segments by making a new layout file called components/DashboardLayout.js. This file should export a component that returns a shared UI for these pages and uses the children prop to render their respective content.
To apply a custom layout to a specific route segment, you can isolate that segment from the shared layouts using route groups, as seen in the example of designing a personalized layout for the Newsletter page.
To implement the root layout in the app router, you'll use a specific file system structure, with the app/layout.js file being used to initialize pages. You can override this file to control page initialization, allowing you to add global CSS files and maintain state when navigating between pages.
Worth a look: 404 Nextjs
By wrapping the current page's component in a MyApp component, you can ensure that every page in your Next.js application uses the same root layout, providing a consistent structure and style across your entire application.
The children prop in the layout file serves as a placeholder for the page content, and you can render multiple views within a layout, as demonstrated by rendering the Settings page alongside the Notifications and Revenue metrics components.
For more insights, see: Next Js 14 Redirect to Another Page Loading Indicator
Utilizing Nested Layout Features
To create a nested layout for pages under the /dashboard/* route segments, you need to create a new layout file called components/DashboardLayout.js. This file should export a component that returns a shared UI for these pages and uses the children prop to render their respective content.
In each of the /dashboard/* page files, you need to apply a getLayout property on the page component and return the desired layout tree. For example, the /dashboard/account.js file will look like this: the DashboardLayout is nested within the RootLayout.
Worth a look: Framer Motion Page Transitions Nextjs Examples
You can also create a custom layout in the Next.js App Router by isolating a specific route segment from the shared layouts using route groups. This is useful when you want to design a personalized layout for a specific page, like the Newsletter page.
The children prop in the layout file serves as a placeholder for the page content, and you can use it to render multiple views within a layout. For instance, alongside the Notifications and Revenue metrics components, you can render the Settings page.
To implement the root layout in the app router, you can override the app/layout.js file to control page initialization and add global CSS files. This ensures that every page in your Next.js application uses the same root layout, providing a consistent structure and style across your entire application.
The children prop is a powerful feature in React that allows you to pass components as data to other components, enabling complex layout compositions. You can use it to nest page content within layouts, creating a hierarchy of components that reflects the structure of your application's UI.
For another approach, see: Next Js Fetch Data save in Context and Next Route
Integrating with Other Components
React components are the building blocks of your nested layouts. You can create a nested layout by defining a new React component that includes the shared layout for a particular section of your application.
To create a nested layout, you'll use a new React component to wrap the relevant page components. For example, you might create a DashboardLayout component that includes the main navigation and footer, and adds a Sidebar specific to the dashboard section.
A client component can be used to mix in with other components, but it's not recommended to make the entire root layout component a client component. This is because a server component can only import other server components.
You can create a wrapper around the Link component and import that into your root layout. This allows you to use a new hook called useSelectedLayoutSegment to determine which URL segment you're currently on.
Replace instances of Link in your root layout with your newly created NavLink component. This gives you the benefits of server components throughout your application.
You might enjoy: Nextjs Pathname Server Component
Use Cases and Best Practices
Nested layouts are ideal for applications with diverse UI sections, such as dashboards with sidebars, headers, and different content areas.
They allow for compartmentalizing layout designs, making it easier to manage and update specific sections of the application without affecting the overall layout.
Nested layouts provide flexibility in designing complex UI structures and enable developers to create customized layouts tailored to specific application requirements.
This flexibility is especially useful when building dashboards or applications with multiple content areas, as it allows for a high degree of customization and control.
Suggestion: Next Js Movie Streaming Ui
Sources
- https://blog.logrocket.com/guide-next-js-layouts-nested-layouts/
- https://www.builder.io/blog/layouts-in-nextjs-14-visual
- https://purecode.ai/blogs/nextjs-layout
- https://www.dhiwise.com/post/exploring-nextjs-root-layout-for-scalable-web-application
- https://blog.mechanicalrock.io/2022/10/27/next-js-layouts-by-example.html
Featured Images: pexels.com