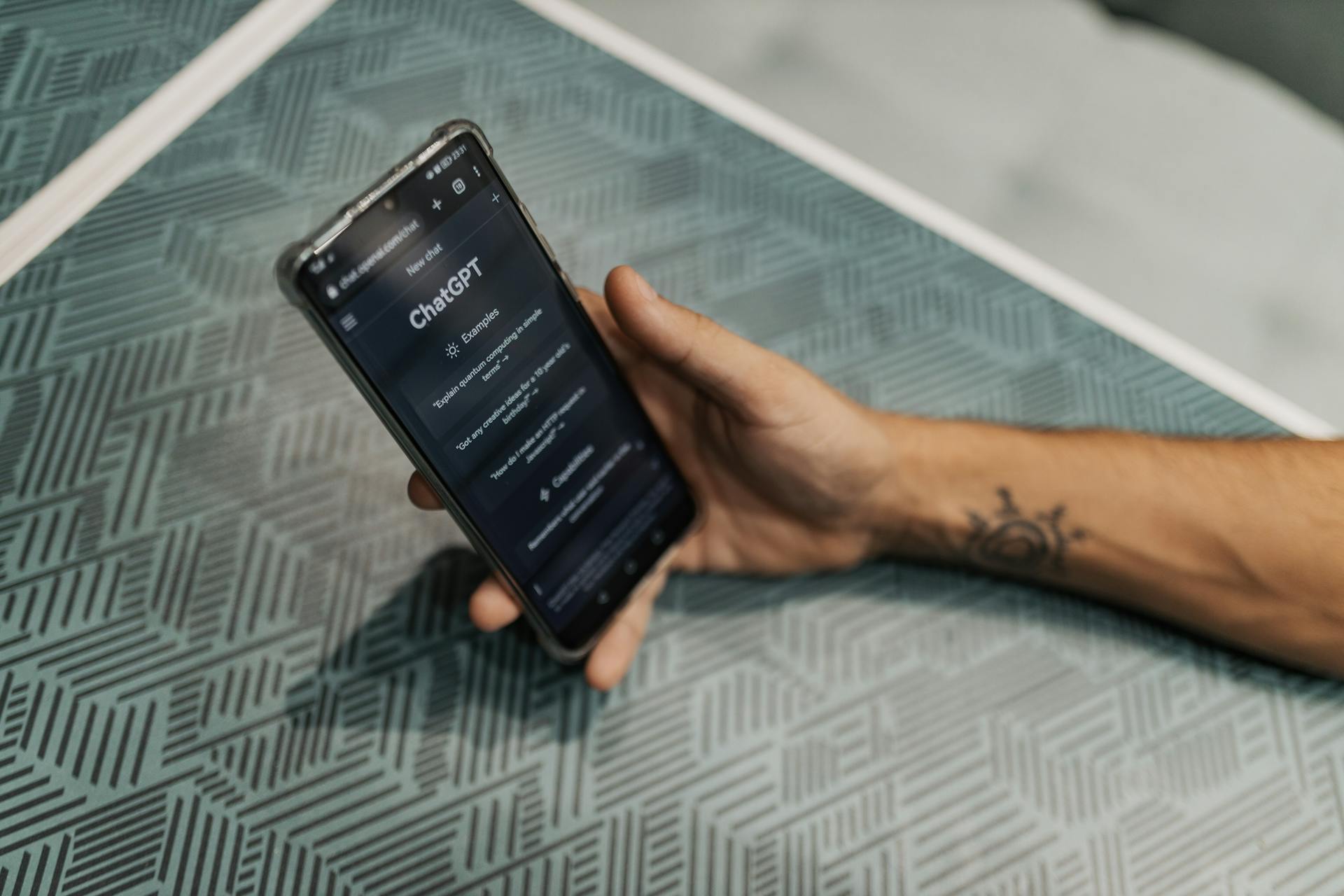
Building a Next JS Chat App with Real-Time Messaging is a project that requires some planning and setup.
First, you'll need to create a new Next JS project using the command `npx create-next-app my-chat-app`. This will give you a basic structure to work with.
To enable real-time messaging, you'll need to install the `socket.io` package using npm or yarn. This package will allow you to establish a connection between the client and server.
With `socket.io` installed, you can start building your chat app by creating a new page for the chat interface.
If this caught your attention, see: Next Js vs Create React App
Set Up Project
To set up your project, start by creating a new NEXT.js project using the command `npx create-next-app my-chat-app`. Then, change into the project directory using the command `cd my-chat-app`.
You can find your App ID in the Settings tab of the TalkJS dashboard, and for this tutorial, we recommend using the App ID for TalkJS's Test Mode, which has built-in sample users and conversations.
Install the required packages by running `npm install @talkjs/react talkjs` or `yarn add @talkjs/react talkjs` in your app.
To make your environment variable accessible in the browser, prefix it with `NEXT_PUBLIC_` as Next.js recommends.
For another approach, see: Next Js React
Server and Messaging Setup
To set up the server, you'll need to create a server.js file in the root directory of your application and add the necessary code snippet to it. This will involve setting up a simple server using Next.js to wrap an Express application server.
You'll also need to load the necessary middlewares for the Express server and configure Pusher using the credentials you added to your environment variables. This is done by adding the body-parser middleware and using the credentials to set up Pusher.
To make the chat application work, you'll need to define the messaging routes. This involves creating the /message and /messages routes, where the /message route fetches the chat payload from req.body and uses the sentiment module to calculate the overall sentiment score of the chat message.
Explore further: Express Js Next Params
Server Setup
To set up the server, you'll need to create a server.js file in the root directory of your application.
Create a server.js file in the root directory of your application and add the following code snippet to it to set up the server: We will go ahead to set up a simple server using Next.js to wrap an Express application server.
We will also load the necessary middlewares for the Express server. Next.js will be used to wrap an Express application server, making it a great choice for building server-rendered applications.
A different take: Next Js Server Side Api Call
Defining Messaging Routes
To get our chat app up and running, we need to define the messaging routes. We'll create two routes: /message and /messages. The /message route will handle sending a single chat message, while the /messages route will fetch all chat history messages.
We'll add the body-parser middleware to fetch the chat payload from req.body. This will help us process the incoming message. The sentiment module will also be used to calculate the overall sentiment score of the chat message.
The chat history will be stored in an in-memory store, which will be useful for new clients that join the chat room to see previous messages. Whenever the Pusher client makes a POST request to the /messages endpoint on connection, it will get all the messages in the chat history in the returned response.
To trigger real-time magic, we'll add the chat to the chat history messages and then trigger a new-message event on the chat-room Pusher channel, passing the chat object in the event data. This will enable our chat app to update in real-time as messages are sent and received.
If this caught your attention, see: Nextjs App Router Tailwind Spinner Loading Page
Building the Chat App
To build a chat app, you need to create a Next.js project using create-next-app. This will give you a basic project structure to work with.
You'll also need to install the PubNub JavaScript SDK using npm to integrate real-time functionality into your chat app.
The first step in building the chat app is to create the Chat component. This component will handle sending and receiving messages. You can use React Hooks and CSS to create components for the chat input and message list.
To implement real-time chat functionality, you can use the PubNub SDK to send messages and subscribe to channels (chat groups and threads) to process received messages.
Here's a list of the key components you'll need to build:
- Chat Input Component: This component will handle user input for sending messages.
- Chat Messages Component: This component will display incoming messages.
- Chat Interface: This component will handle sending and receiving messages.
Here's a simple table to illustrate the components you'll need:
To implement private messaging, you'll need to establish private rooms or namespaces for individual users. You can use a unique identifier (e.g., user ID) to achieve this.
In addition to private messaging, you'll also need to implement access control and permission systems to ensure message privacy.
The final step in building the chat app is to style the user interface using CSS or a component library like Material-UI or Bootstrap. This will give your app a polished look and feel.
With these steps completed, you'll have a fully functional real-time chat application built on Next.js and powered by PubNub.
Advanced Features and Security
As you build your next.js chat app, you'll want to consider advanced features to enhance user experience and security. Advanced Features and Security are crucial for a robust chat app.
To implement authentication, you can use techniques like JSON Web Tokens (JWT) or session-based authentication to secure your real-time connections. This is because Socket.io doesn't provide built-in authentication mechanisms, so you'll need to handle authentication manually.
Securing your real-time connections is a top priority, so take the time to implement authentication logic that works for your app.
Discover more: Nextjs 14 New Features
Handling Authentication
Authentication is a critical aspect of real-time applications, and Socket.io doesn't provide built-in mechanisms, so you'll need to implement your own logic.
You can use techniques like JSON Web Tokens (JWT) to secure your real-time connections, as mentioned in the documentation.
In fact, JWT is a popular choice for authentication due to its simplicity and efficiency.
For more insights, see: Next Js Jwt
Scaling the Application
Scaling your real-time application is crucial to handle a larger number of users and connections.
As your user base grows, a scalable backend infrastructure becomes essential. Consider using a load balancer to distribute incoming connections across multiple servers.
A load balancer ensures that no single server is overwhelmed, preventing downtime and improving overall performance. It's a simple yet effective way to scale your application.
To handle large-scale real-time events efficiently, utilize a message queue system like RabbitMQ or Redis. These systems help manage high volumes of data and prevent bottlenecks.
By implementing a scalable infrastructure, you can ensure your application remains responsive and reliable, even under heavy loads.
Take a look at this: Can Nextjs Be Used on Traditional Web Application
Best Practices and Tools
Building a next.js chat app requires a solid foundation of best practices and the right tools.
Next.js is built on top of Node.js, which means you can leverage its power to create a robust and scalable chat app.
To ensure a seamless user experience, consider using PubNub's real-time infrastructure capabilities.
PubNub allows you to easily develop a full-stack instant messaging app, making it a great choice for your next.js chat app.
Advanced features and best practices are essential for building a successful chat app.
By exploring advanced features and best practices, you can take your next.js chat app to the next level.
For your interest: Best Next Js Database with Drizzle
Socket.io and PubNub Integration
To integrate real-time messaging into your Next.js chat app, you have two options: Socket.io and PubNub. For Socket.io, you can start by installing the Socket.io client library using the command `npm install socket.io-client`. Once installed, create a new file named `socket.js` in the root of your project directory and add the following code.
For more insights, see: Next Js Client Portal
Socket.io can be configured in your application to handle real-time messaging. To get started with PubNub, sign up for a free account to obtain your API keys, which are essential for integrating PubNub's real-time messaging services into your chat app.
PubNub offers a comprehensive documentation that provides guides and tutorials to help you integrate PubNub into your chat app. You can choose a PubNub SDK that supports your chosen framework, such as JavaScript or Node.js, to handle messaging while your framework controls the app state management.
Here's a comparison of the steps to integrate Socket.io and PubNub into your Next.js chat app:
By following these steps, you can choose the best option for your Next.js chat app and integrate real-time messaging using Socket.io or PubNub.
Example and Demo
If you want to see a Next.js chat app in action, check out PubNub's Chat SDK web chat demo, which showcases features like message threads, reactions, quoting, and more.
You can also learn how to make a messaging app yourself by following the tutorial or checking out the code repo on GitHub.
PubNub offers a range of example projects to inspire your chat app project, including a group chat app, a web-based chat app, a chatbot app, and a customer support chat app.
Here are some examples of real-time chat apps built using Next.js and PubNub:
- Group Chat App: Allows users to join different chat rooms, send messages, and see who's online.
- Web-Based Chat App: Enables users to send messages and share files with others.
- Chatbot App: Integrates AI-powered chatbots, such as OpenAI's GPT, to answer user queries and provide assistance.
- Customer Support Chat App: Connects users with support agents in real-time, allowing them to resolve issues quickly and efficiently.
Sources
- https://pusher.com/tutorials/chat-sentiment-analysis-nextjs/
- https://www.cometchat.com/tutorials/how-to-build-a-chat-app-with-nextjs
- https://clouddevs.com/next/real-time-applications/
- https://talkjs.com/resources/add-chat-to-a-nextjs-app-with-talkjs/
- https://www.pubnub.com/blog/how-to-build-a-next-js-real-time-chat-application/
Featured Images: pexels.com