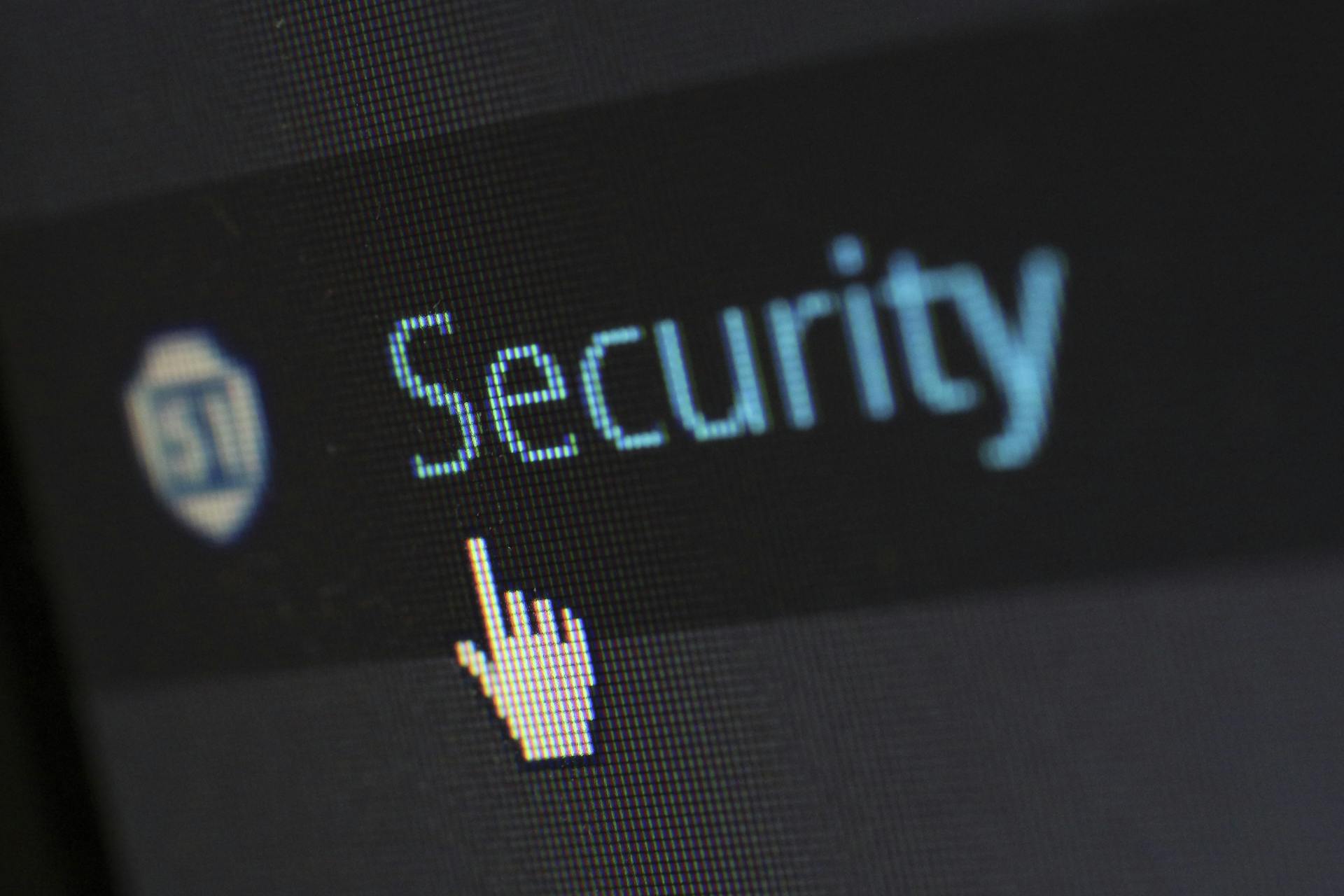
Next.js JWT authentication is a crucial aspect of building secure and scalable applications.
You can use the `next-auth` library to simplify the process of implementing JWT authentication in Next.js.
The `next-auth` library provides a simple and efficient way to handle authentication, including token generation and validation.
To get started with JWT authentication in Next.js, you'll need to install the `jsonwebtoken` library and create a secret key for signing and verifying tokens.
The secret key should be kept secure and not shared with anyone.
Authentication Setup
To set up authentication in your Next.js app, you'll want to start by creating a hook to manage the authentication state. This hook will fetch and verify the JWT token present in cookies and set the authenticated user details to the auth state.
You can create a new folder in the src directory and name it hooks, then add a new useAuth/index.js file and include the code that manages the authentication state. This hook will allow other components to access and utilize the authenticated user's information.
The hook will conditionally render different content on the home route based on the authentication state of a user. You can use the hook to render a public home page with a link to the login page route when the user is not authenticated, and display a paragraph for an authenticated user.
To enforce authorized access to protected routes, you'll need to add a middleware to your Next.js app. This middleware code acts as a guard, checking to ensure that users are authenticated and authorized to access the routes, and redirecting unauthorized users to the login page.
The middleware code will be added to a new middleware.js file in the src directory. This will ensure that only authenticated users can access protected pages in your app.
In addition to the hook and middleware, you'll also need to set up an API route handler to authenticate users. This handler will receive HTTP requests sent to the authenticate route /api/users/authenticate and will authenticate the user using the authenticate() method.
On successful authentication, a JWT token will be generated and returned to the client application, which must include it in the HTTP Authorization header of subsequent requests to secure routes. This is handled by the fetch wrapper in your example app.
To verify the JWT token, you can use the verifyJwtToken function, which fetches and verifies the validity of the JWT token present in cookies. This function will be used in the useAuth hook to manage the authentication state.
The JWT token will be stored in the users.json file, which is used for simplicity in this example. However, in a production application, it's recommended to store user records in a database with hashed passwords.
Once the user is logged in, you can use the Auth Middleware in Apollo Client to set the Authorization header, which will be used to authenticate subsequent requests. The getToken() function will be used to get the auth token stored in the cookie and return the token.
The user service will handle communication from the React front-end to the backend API, containing methods for logging in and out of the app, and a method for fetching all users from the API. The API will return the user details and a JWT token, which will be published to all subscribers with the call to userSubject.next(user).
Protected Routes
To create protected routes in Next.js, you can use a Higher Order Component (HOC) to check for authentication before rendering the component. This is handled by creating a HOC that checks for authentication.
You can use an HOC to handle authorized queries, but to restrict access to pages like /articles at the route level, you need to make use of another HOC.
When a navigation is triggered on a protected route, a utility helper method is called to fetch the token from the cookie using the nextCookie module. If the token is not available, it will redirect to the /login page.
Protected components like articles can be wrapped using the withAuthSync HOC, which will take care of the redirects on the server.
Here are some key points to consider when creating protected routes:
- Use a HOC to check for authentication before rendering the component
- Use another HOC to restrict access to pages like /articles at the route level
- Call a utility helper method to fetch the token from the cookie when a navigation is triggered on a protected route
- Use the withAuthSync HOC to wrap protected components like articles
By following these steps, you can create protected routes in Next.js that ensure only authenticated users can access certain pages or resources.
Security and Best Practices
Token authentication is an effective security mechanism for Next.js applications, but it's not the only strategy to safeguard against unauthorized access.
To fortify applications against the dynamic cybersecurity landscape, adopt a comprehensive security approach that addresses potential security loopholes and vulnerabilities. This guarantees thorough protection.
JWTs are signed and optionally encrypted, making the token's content tamper-proof during transmission, and preventing unauthorized modifications.
Create a comprehensive security approach that includes token authentication, as it's an essential component to safeguard your applications from unauthorized access.
By managing the authentication state on the client side, you can fetch and verify the validity of the JWT token present in cookies using the verifyJwtToken function, and then set the authenticated user details to the auth state.
Handling state management using Redux Toolkit or employing a state management tool like Jotai guarantees components can get global access to the authentication state or any other defined state.
In Next.js applications, it's essential to conditionally render different content based on the authentication state of a user, such as displaying a public home page with a link to the login page route when the user is not authenticated.
Token Management
Token Management is crucial in Next.js JWT authentication. You'll need to generate a token after a successful login process, which involves implementing token verification logic.
To verify tokens, you'll use the jwtVerify function from the Jose module. This function compares the decoded token signature to the expected signature, allowing the server to verify the token's validity and authorize user requests.
You'll also need to store the token securely on the client-side, which can be done using localStorage or cookies.
Implement Token Revocation
Implementing token revocation is a crucial step in ensuring the security of your application. This mechanism allows you to invalidate tokens before they expire, thereby preventing unauthorized access.
You can implement token revocation by creating a middleware that checks for token validity. As mentioned in the article, you can create a new middleware.js file and add the code to handle token revocation.
For enhanced security, consider implementing token revocation as part of your authentication flow. This can be done by adding a token revocation endpoint to your API, which can be called when a user logs out or when a token is compromised.
By implementing token revocation, you can significantly reduce the risk of unauthorized access to your application. This is especially important for applications that handle sensitive user data.
To get started with token revocation, you can follow the example provided in the article and create a new middleware.js file with the code to handle token revocation.
Token Expiration & Refresh
JWTs can have an expiration time to enhance security, which can be set during token generation.
This is done to prevent tokens from being used indefinitely and to force users to re-authenticate after a certain period.
To implement token refresh, you should issue a refresh token alongside the access token.
When a token expires, users should refresh it without the need to re-authenticate.
Here's a summary of the token expiration and refresh process:
In the context of a Next.js client app, token expiration and refresh can be implemented by using a middleware to manage the authentication state and token verification logic.
By implementing token expiration and refresh, you can enhance the security of your application and provide a better user experience.
Implementation Details
To implement a Login API Endpoint in Next.js, you need to create a new folder called api inside the src/app directory. Inside this folder, add a new file called route.js.
The primary task for this API is to verify login credentials passed in POST requests using mock data. This is done to test the API's functionality without relying on actual user data.
The API generates an encrypted JWT token associated with the authenticated user details upon successful verification. This token is then sent to the client in the response cookies.
The API returns a failure status response if the login credentials are invalid. This ensures that the client is notified of the authentication failure.
Example API
The Example API is a crucial part of the Next.js JWT auth example. It contains two routes/endpoints: /api/users/authenticate and /api/users. The first one is a public route for authenticating username and password, on success returns a JWT token and basic user details. The second one is a secure route that returns all users for requests contain a valid JWT token.
User data is stored in a JSON flat file (/data/users.json) to keep the example simple and focused on authentication. In a production application, data should be stored in a database (e.g. MySQL, MongoDB, PostgreSQL etc).
The API routes are as follows:
- /api/users/authenticate - public route for authenticating username and password, on success returns a JWT token and basic user details.
- /api/users - secure route that returns all users for requests contain a valid JWT token.
This API setup allows for secure authentication and authorization, making it a great starting point for building a Next.js JWT auth API.
Deploy On Now
To deploy your Next.js app on now.sh, you can use the serverless target. This means you don't need to worry about setting up and managing servers, as now.sh takes care of it for you.
The JWT server also needs to be deployed, and its URL must be configured inside your Next.js app. This is a crucial step to ensure seamless integration and authentication.
You can deploy your app to the cloud using now, which can be downloaded from the provided link. This is a convenient and efficient way to get your app up and running quickly.
Sources
- https://nextjs.org/docs/app/building-your-application/authentication
- https://www.makeuseof.com/token-authentication-nextjs-using-jwt/
- https://jasonwatmore.com/post/2021/08/04/next-js-11-jwt-authentication-tutorial-with-example-app
- https://clouddevs.com/next/jwt-and-token-based-authentication/
- https://hasura.io/blog/add-authentication-and-authorization-to-next-js-8-serverless-apps-using-jwt-and-graphql
Featured Images: pexels.com