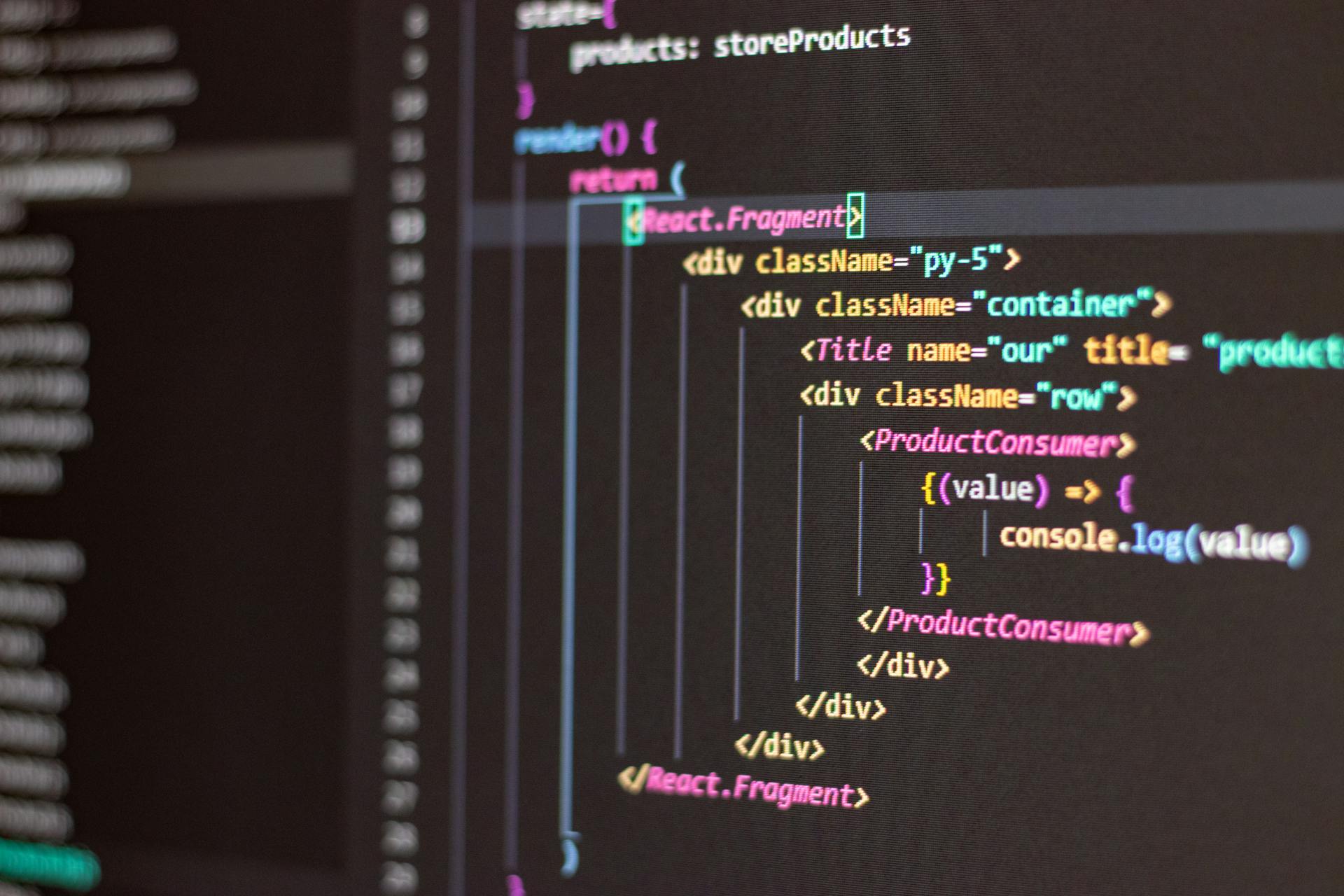
Next.js makes it easy to build server-rendered, statically generated, and performance-optimized websites. With Supabase, you can add authentication to your Next.js website in minutes. Supabase's API provides a simple and secure way to authenticate users.
Supabase is a PostgreSQL database with a RESTful API and a GraphQL API. This makes it easy to integrate with Next.js. Supabase's authentication features include email and password authentication, social media authentication, and more. Supabase also provides a built-in authentication system that can be easily integrated with Next.js.
FusionAuth is another popular authentication solution that can be used with Next.js. FusionAuth provides a robust and customizable authentication system that can be easily integrated with Next.js. With FusionAuth, you can add features like multi-factor authentication, passwordless authentication, and more to your Next.js website.
For your interest: Nextjs Website Template
Getting Started
You can add authentication in minutes with Clerk, making it a breeze to get started.
Clerk considers education a first-class feature, so you can choose a learning resource that resonates with you and start building something awesome.
With Clerk, you can clone an auth starter template powered by Clerk and focus on building unique features, saving you time and effort.
Now go build something awesome with Clerk!
For your interest: Font Awesome Icons Next Js
Components and UI
Components are the building blocks of your application, and with next js auth, you can configure them to work seamlessly with authentication options.
You can embed these components directly in your application, making it easy to manage user sign-in, sign-up, and profile management.
Our components are designed to be beautiful, prebuilt, and customizable to match your brand. They include UI components for secure user sign-in, sign-up, profile management, organization management, and more.
By using these components, you can create a seamless user experience that's both secure and convenient for your users.
Suggestion: Next Js Components Folder
UI Building Blocks
We have a wide range of prebuilt UI components to authenticate and manage your users. These components are designed to work quickly out-of-the-box and can be customized to perfectly match your brand.
Our UI components include beautiful prebuilt components for secure user sign-in, sign-up, profile management, organization management, and more. They're perfect for building a seamless user experience.
You can easily embed these components directly in your application, making it convenient for your users to sign in and manage their accounts. Just configure your components with authentication options your users find convenient.
A unique perspective: Next Js Components
Authentication
Authentication is a crucial aspect of building a secure Next.js website. You can protect your pages using middleware, which runs code before an incoming request completes, allowing you to modify the response based on the nature of the incoming request.
To get started, you'll need to add the Auth0 configuration variables to your Next.js project. This includes the Auth0 Domain, Client ID, and Client Secret values, which can be obtained from the Auth0 Application Settings page.
You can then use the Auth0 Next.js SDK to implement user authentication in your Next.js application. This SDK uses React Context to manage the authentication state of your users on the client side.
To create a dynamic authentication API route, you'll need to create an API route under the src/app/api/auth/ directory. This route will handle the authentication flow of your Next.js application.
Here are the essential API routes that the Auth0 Next.js SDK creates for you:
- /api/auth/login
- /api/auth/callback
- /api/auth/logout
- /api/auth/me
These routes will handle the login, callback, logout, and user information retrieval processes for your Next.js application.
You can also use the Auth0 Next.js SDK to create a route handler for Auth confirmation. This will allow you to exchange a user's secure code for an Auth token when they click their confirmation email link.
To do this, you'll need to create a route handler for auth/confirm, which will use the Supabase client from @/utils/supabase/server.ts.
The Auth0 Next.js SDK also provides a UserProvider component that provides a UserContext to its child components. You can wrap your App Router Root Layout component with UserProvider to integrate Auth0 with your Next.js app.
By following these steps and using the Auth0 Next.js SDK, you can implement secure user authentication in your Next.js website.
Expand your knowledge: Firebase Auth in Nextjs
Security
Security is a top priority when building authentication websites with Next.js. Clerk commissions third-party testing and assessment based on the OWASP Testing Guide, the OWASP Application Security Verification Standard, and the NIST Technical Guide to Information Security Testing and Assessment.
Cross-Site Scripting (XSS) vulnerabilities are incredibly serious, but Clerk works to minimize attack surface area by using HttpOnly cookies for authenticated requests to their Frontend API, so that credentials cannot be leaked during XSS attacks.
Clerk handles the necessary configuration on your behalf by configuring cookies with the SameSite flag, protecting against most Cross Site Request Forgery (CSRF) attacks. Session fixation is a technique for hijacking a user session, but Clerk protects against this by resetting the session token each time a user signs in or out of a browser.
Clerk uses NIST guidelines to determine the character rules for passwords and contracts with HaveIBeenPwned to review prospective passwords. Additionally, Clerk leverages bcrypt, an industry standard hashing algorithm for storage.
Here are some security features provided by Clerk:
- Cross-Site Scripting (XSS) protection
- Cross Site Request Forgery (CSRF) protection
- Session fixation protection
- Password strength checking with NIST guidelines
- Password review with HaveIBeenPwned
- Bcrypt hashing for password storage
By using Clerk, you can take the security burden off your shoulders and focus on building a great user experience.
API Integration
API Integration is a crucial part of building Next.js auth websites. You can use the Auth0 Next.js SDK to implement user authentication in Next.js applications. This SDK allows you to create a dynamic authentication API route.
To create a dynamic authentication API route, you need to create an api directory under the src/app directory, followed by an auth directory and then an [auth0] directory. Inside the [auth0] directory, create a route.ts file, which is the path to your dynamic API route.
The handleAuth() method is the main way to use the server-side features of the Auth0 Next.js SDK. Running handleAuth() automatically creates 5 API routes for you to handle the essential features of user authentication in Next.js: /api/auth/login, /api/auth/callback, /api/auth/logout, and /api/auth/me.
You can also integrate Next.js with an API server to access protected resources. Auth0 provides functionality to log in and log out users from your Next.js application, and you can use Auth0 to protect your API and delegate the authorization process to a centralized service.
To make secure API calls from Next.js, your application can authenticate the user, request an access token from Auth0, and pass that access token to your API as a credential. The API can then use Auth0 libraries to verify the access token and issue a response with the desired data.
Here's a quick summary of the API routes created by handleAuth():
- /api/auth/login
- /api/auth/callback
- /api/auth/logout
- /api/auth/me
By integrating your Next.js application with an API server, you can access protected resources and use Auth0 to verify user authentication.
Configuration and Setup
To configure your Next.js website for authentication, start by configuring Next.js with Auth0. This involves following a few steps to get started with the Auth0 Identity Platform quickly.
You'll need to add Auth0 configuration variables to Next.js, which includes getting the Auth0 Domain, Client ID, and Client Secret values from the Auth0 Application Settings page. The Client ID is an alphanumeric string that identifies your application, and the Client Secret protects your resources by only granting tokens to authorized requestors.
To set up environment variables, create a .env.local file in your project root directory and fill in your NEXT_PUBLIC_SUPABASE_URL and NEXT_PUBLIC_SUPABASE_ANON_KEY.
Check this out: Next Js Client Portal
Configure
To configure Next.js with Auth0, start by getting the Auth0 Domain, Client ID, and Client Secret values from the Auth0 Application Settings page. You'll need these values to allow your Next.js web application to use the communication bridge.
Each application is assigned a unique Client ID, which is an alphanumeric string, and it's used to identify the Auth0 Application to which the Auth0 Next.js SDK needs to connect. Think of it as your application's password, which must be kept confidential at all times.
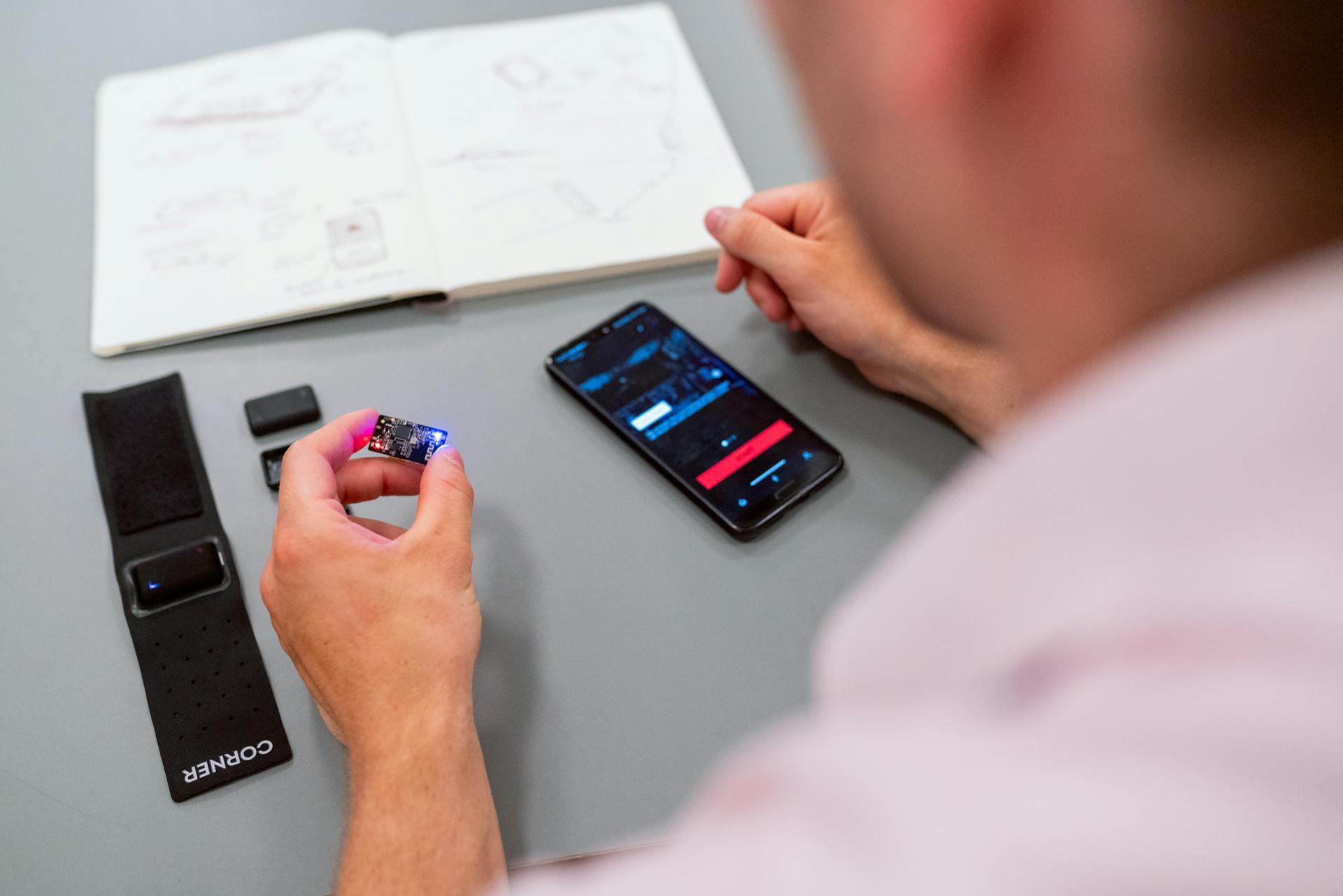
The Auth0 Client Secret protects your resources by only granting tokens to requestors if they're authorized. If anyone gains access to your Client Secret, they can impersonate your application and access protected resources.
To set up your single-page application, enter the "Domain" and "Client ID" values in the corresponding fields. You'll also need a session secret to sign the session ID cookie of your Next.js web application. Use the following command to generate a random secret key and copy the output to paste into the input box.
The AUTH0_BASE_URL is the base URL of your application, which you'll need to specify when working with Auth0. It's a good practice to have different tenants for your different project environments, such as a production tenant, which gets higher rate limits than non-production tenants.
Curious to learn more? Check out: Iron Session Next Js Middleware
Install Supabase Packages
To set up Supabase, you'll need to install the required packages. Install the @supabase/supabase-js package and the helper @supabase/ssr package.
Consider reading: Install Nextjs
These packages will provide the necessary tools for your application. The @supabase/supabase-js package is a JavaScript library for interacting with Supabase, while the @supabase/ssr package is a helper package for server-side rendering.
Copy the middleware code for your app to get started. This code will allow you to connect your application to Supabase.
Broaden your view: Can Nextjs Be Used on Traditional Web Application
Supabase and Middleware
To protect your Next.js website with Supabase, you'll need to install the Supabase packages, specifically @supabase/supabase-js and @supabase/ssr. This allows you to leverage the power of middleware to refresh expired Auth tokens and store them securely.
You'll want to create a middleware.ts file at the root of your project, which will handle the authentication process. This middleware is responsible for refreshing the Auth token by calling supabase.auth.getUser, passing the refreshed token to Server Components, and storing it in the browser.
To refresh the Auth token, the middleware calls supabase.auth.getUser, which sends a request to the Supabase Auth server to revalidate the token. This is a crucial step, as it ensures the token is valid and not spoofed.
Worth a look: Nextjs Auth Providers
Server Components can't write cookies, so the middleware takes care of storing the refreshed token in the browser using response.cookies.set. This way, the token is replaced with the new one, ensuring the user remains authenticated.
To protect pages, you should always use supabase.auth.getUser() to verify the user's session. Never trust supabase.auth.getSession() inside server code, as it may not revalidate the Auth token.
Here's a summary of the middleware's responsibilities:
- Refresh the Auth token by calling supabase.auth.getUser
- Pass the refreshed token to Server Components using request.cookies.set
- Store the refreshed token in the browser using response.cookies.set
By following these steps and using middleware to handle authentication, you can ensure your Next.js website is secure and protected with Supabase.
Error Handling and Pages
When you're dealing with authentication errors, it's essential to understand how to handle them effectively. You can pass error query parameters to the default or overridden error page.
There are four types of errors that can occur: Configuration, AccessDenied, Verification, and Default. These errors are usually caused by issues like restricted access or expired tokens.
Here are the specific error types and their causes:
- Configuration: There is a problem with the server configuration. Check if your options are correct.
- AccessDenied: Usually occurs when you restricted access through the signIn callback, or redirect callback
- Verification: Related to the Email provider. The token has expired or has already been used
- Default: Catch all, will apply if none of the above matched
For example, if you encounter a configuration error, you'll see an error page like this: /auth/error?error=Configuration. Similarly, if none of the above error types match, the default error page will be displayed: /auth/signin?error=Default.
Related reading: 404 Nextjs
Frequently Asked Questions
What website uses next JS?
Next.js is used by popular platforms like Netflix, Nike, and Hulu, but it's also suitable for smaller businesses and projects
Is Next Auth free?
NextAuth.js is free and open source, making it a cost-effective solution for authentication needs
Is next auth OAuth?
Yes, NextAuth.js supports OAuth, specifically versions 1.0, 1.0A, 2.0, and OpenID Connect, making it a versatile authentication solution.
Is Clerk Next Auth?
Clerk supports authentication with Next.js, enabling seamless user verification. Learn more about Clerk's Next Auth capabilities.
Featured Images: pexels.com