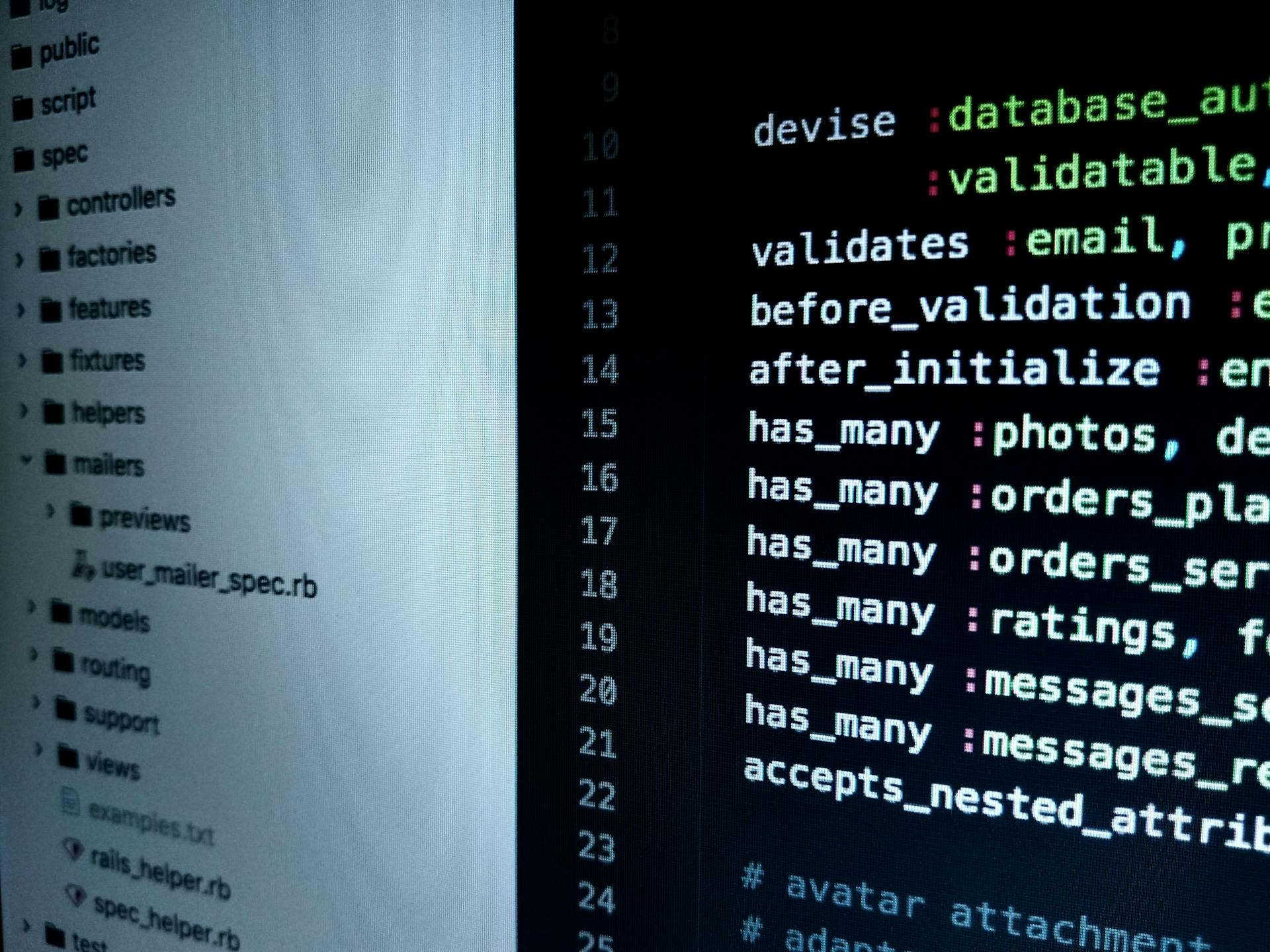
Next.js fetch cache is a powerful tool that can greatly enhance the performance of your application. By leveraging the browser's cache, Next.js fetch cache can reduce the number of requests made to your server, resulting in faster page loads and a better user experience.
One way to achieve this is by using the `fetch` API with the `cache` option set to `'force-cache'`. This tells the browser to always use the cached response, even if it's stale.
Using the `fetch` API with the `cache` option set to `'force-cache'` can lead to a significant reduction in requests made to the server, resulting in improved performance.
Next.js fetch cache also allows you to specify a custom cache key, which can be useful when dealing with dynamic data. By setting a unique cache key, you can ensure that the browser caches the correct response, even if the data changes.
Server-Side Techniques
Next.js 14 has equipped developers with an impressive array of server-side data fetching capabilities, particularly through its extended fetch API.
Server-side data fetching in Next.js 14 can be achieved through native fetch, which allows for straightforward implementation and reduced server workload, especially when fetch requests are memoized.
Native fetch benefits from React's stability to deny unnecessary data fetching, ensuring that once data is fetched and stored in state, it will not be re-fetched unless the component is unmounted and remounted.
Server-side data fetching via third-party libraries also has its place in Next.js 14, often offering more granular control or additional features beyond what the native fetch provides.
Implementing effective caching strategies involves understanding the trade-offs between immediate consistency and performance benefits of serving stale content.
getServerSideProps is another built-in method in Next.js that allows you to fetch data on each request, useful when you need to fetch dynamic data that changes frequently.
Use getStaticProps for fetching static data that doesn't change frequently, allowing Next.js to generate static HTML files and cache the data for a longer period.
Use getServerSideProps for fetching dynamic data that changes frequently, allowing Next.js to re-render the page on each request and cache the data for a shorter period.
The decision around caching duration and revalidation triggers directly affects user perceived latency and server workload, with shorter-lived caches ensuring data freshness but increasing server activity.
Client-Side Strategies
SWR and React Query are two popular libraries designed for data retrieval, which can be used in conjunction with Next.js to streamline the data fetching lifecycle.
These libraries offer robust strategies for caching and revalidating content, playing a critical role in rendering a seamless user experience and fostering scalable applications.
SWR, for instance, embodies the "stale-while-revalidate" principle by treating the cache as the primary source of truth and revalidating it asynchronously.
React Query, on the other hand, leans on a configuration-first approach, adjusting query behaviors through options, and features automatic background refreshes and pagination.
Libraries like SWR and React Query cache effectively and reduce the quantity of duplicate requests, ensuring efficient use of network and server resources, which directly correlates with an application's ability to scale.
The impact of these libraries on scalability cannot be overstated, allowing developers to focus on crafting the application's core functionality and leading to more maintainable and evolvable codebases.
However, it's essential to consider the potential risks of over-reliance on these tools, such as misconfiguring caching strategies or failing to effectively manage cache invalidation, which can lead to outdated data being served to users.
By understanding the library's caching mechanisms and judiciously implementing revalidation logic, developers can ensure data consistency and reliability.
Caching Internals and Configuration
Next.js 14 introduces a more sophisticated handling of information retrieved from network requests, incorporating advanced caching configurations that developers can leverage to optimize their applications.
Developers can specify caching behavior using the next.config.js configuration file or directly within specific API routes, where cache-control headers inform how fresh or stale a piece of data is allowed to be before it's considered necessary to fetch it again.
The stale-while-revalidate header is particularly useful for this, as it provides a way to serve up-to-date data after serving the cached version.
Configuring caching in Next.js may look like the following in an API route: In this configuration, data from the /api/data endpoint is cached for 10 seconds (s-maxage=10) and will be revalidated in the background once it becomes stale.
Internals and Configuration
Next.js 14's caching mechanism allows developers to specify how data should be cached and the conditions under which it should be revalidated. This is done through a configurable caching system that can be set up in the next.config.js configuration file or directly within specific API routes.
The caching system in Next.js 14 is dynamic, adjusting to the application's data fetching patterns. This means that developers can specify caching behavior based on the application's needs.
Next.js 14 introduces advanced caching configurations that can be used to optimize applications. Developers can leverage these configurations to control how and when stale data is refreshed.
The stale-while-revalidate header is particularly useful for this, as it provides a way to serve up-to-date data after serving the cached version.
Configuring caching in Next.js may look like the following in an API route: data from the /api/data endpoint is cached for 10 seconds and will be revalidated in the background once it becomes stale.
Developers should simulate different network conditions and user behaviors to understand how their caching setup will perform in the real world.
Prisma Accelerate
Prisma Accelerate is a connection pooler and global cache that makes your database queries faster, especially in Serverless and Edge environments.
It's used to speed up your app, and you can get started with it in a Next.js app.
Advanced Revalidation Patterns
In modern web applications, ensuring data freshness while maintaining performance can be a challenging task. Next.js 14 offers advanced revalidation patterns that allow developers to fine-tune how their application handles data fetching, caching, and revalidation.
Time-based revalidation is particularly useful for data that does not change frequently. By specifying revalidation intervals, applications can limit update checks to a reasonable frequency, balancing server load and data freshness.
You can implement revalidation at a set interval in a Next.js application by using the next.revalidate option of fetch to set the cache lifetime of a resource, such as giving it a 1-minute lifetime.
On the other hand, on-demand revalidation enables applications to respond promptly to events that should trigger a data refresh, like user actions or external system notifications. This type of revalidation is particularly useful in a content management system (CMS) that updates and necessitates immediate data revalidation.
To implement event-driven revalidation in Next.js, you can use cache tags, which allow you to revalidate specific data when required.
A common coding mistake in the context of revalidation is over-fetching, where developers omit conditional checks, leading to unnecessary requests even when cached data would suffice.
Proper revalidation ensures that additional network requests are only made when needed, which is crucial for maintaining a good user experience.
As applications scale, it's essential to consider the complexity and readability of your conditional revalidation logic. Abstraction into utility functions or hooks promotes modularity and eases maintenance.
To refine your revalidation strategy for large-scale Next.js applications, it's helpful to identify patterns in your application to abstract this revalidation logic effectively.
Sources
- https://nextjs.org/docs/app/api-reference/directives/use-cache
- https://borstch.com/blog/development/fetching-caching-and-revalidating-data-in-nextjs-14
- https://30dayscoding.com/blog/handling-data-fetching-and-caching-in-nextjs-applications
- https://blog.keshavdev.com/article/caching-in-nextjs-
- https://www.prisma.io/nextjs
Featured Images: pexels.com