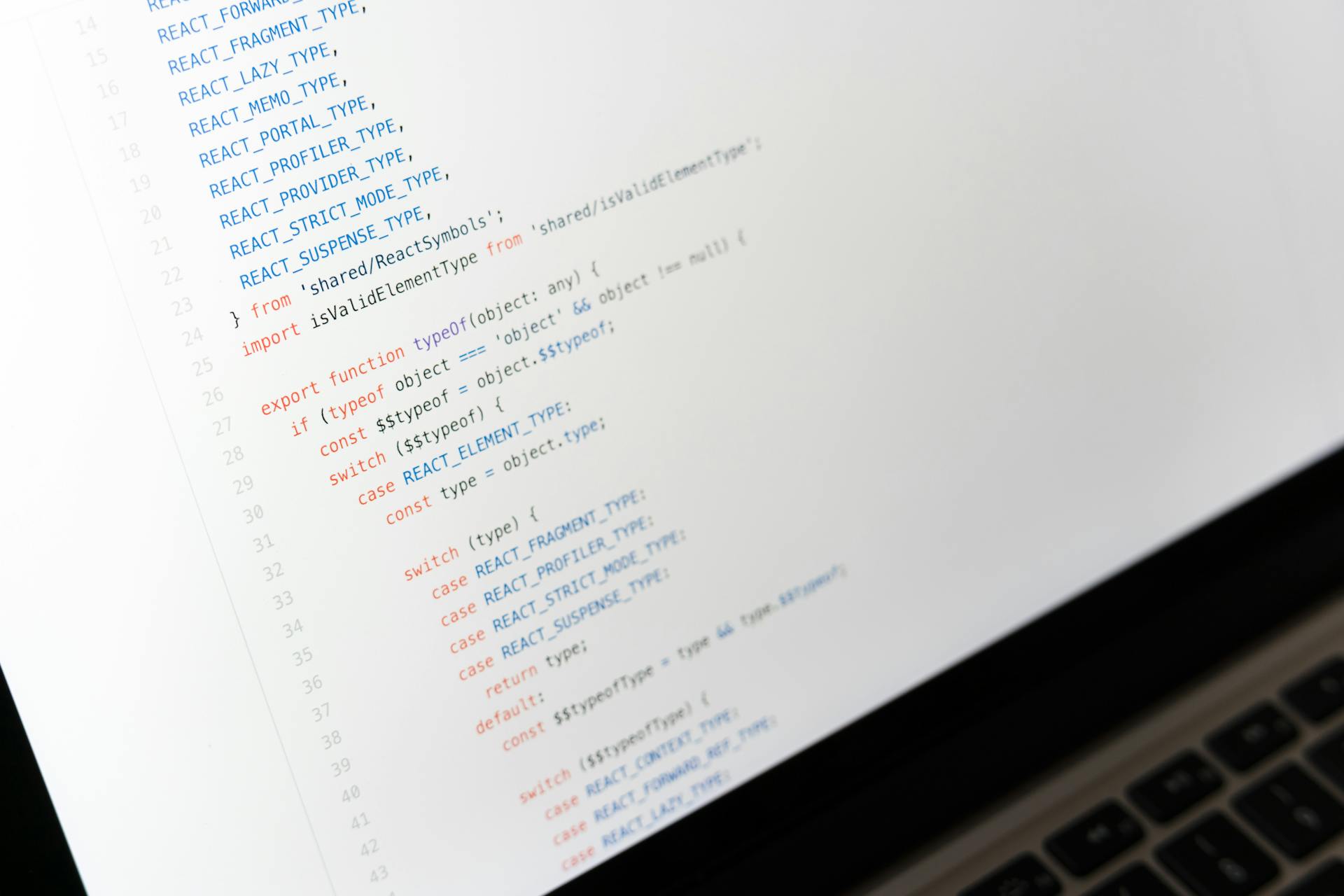
The React Cache API is a game-changer for Next.js developers, allowing you to cache frequently-used data and improve application performance.
By implementing the React Cache API, you can reduce the number of requests made to your server, resulting in faster page loads and a better user experience.
In Next.js, the React Cache API can be used to cache API responses, reducing the load on your server and improving performance.
Caching with the React Cache API is especially useful for applications with a high volume of traffic, where every millisecond counts.
Understanding the Cache API
Next.js cache operates by storing cache data and delivering it upon subsequent data requests, aiming to balance up-to-date content with reduced load times and server demands.
The cache data works by creating cache entries for each unique request, storing the fetched data. This can represent database query results or API responses.
Developers can choose to opt out of caching for specific fetch requests to ensure only fresh data is served. This is done by setting headers to prevent the client and intermediate caches from storing the fetch request response.
The cache approach ensures the most current data is always served, avoiding stale data.
Web Performance
Caching is a game-changer for web performance, and it's a crucial element in modern web development.
By utilizing various caching mechanisms, such as Next.js's integrated functionalities and third-party libraries, developers can efficiently manage data requests and enforce caching behavior that aligns with their application's needs.
Caching optimizes both the speed and reliability of content delivery, which are essential factors in improving the user experience. This is especially true for scenarios requiring real-time or highly dynamic data.
The 'no-store' caching policy, for instance, avoids storing responses, making it perfect for fetch function scenarios where data is fetched but not stored for subsequent use.
React's cache function, an experimental feature within React, provides developers with the ability to cache data at the component level, reducing the frequency of fetch requests and optimizing the overall performance of the application.
Unstable_createResource creates a resource with a fetcher function that Next.js’s server components or client components can use, allowing for efficient data cache management.
In coordination with Next.js features, the React cache function can streamline data cache management, ensuring that commonly accessed data is preserved and reused efficiently.
Server Components and Setup
Server components in Next.js transform server-side logic, enabling developers to use React components without sending their logic or state to the browser. This abstraction is advantageous for caching as it separates dynamic user-specific content from the more static structure of an application.
The ServerProfile is a server-side component that leverages the data cache to avoid redundant data fetches, taking advantage of the server request handling to preserve server resources and reduce response times.
Caching mechanisms for server components often involve react server component payload, which encapsulates the minimal data needed to render the components on the client side.
Server Components
Server components in Next.js transform server-side logic, enabling developers to use React components without sending their logic or state to the browser.
This abstraction is advantageous for caching as it separates dynamic user-specific content, which shouldn't be cached, from the more static structure of an application. Caching mechanisms for server components often involve react server component payload, which encapsulates the minimal data needed to render the components on the client side.
To optimize caching behavior, identify what can be cached and what requires revalidate data on every request. In the ServerProfile example, the server-side component leverages the data cache to avoid redundant data fetches.
Caching rendering work reduces the server load by reusing previously computed output, known as react's cache function. This is particularly useful for server components, where revalidation of data can be expensive.
Developers should consider the cache lifetime and potential cache data staleness when designing server components with caching in mind. This is crucial to ensure that the cached data remains relevant and up-to-date.
Client components, in contrast, run in the browser and can utilize built-in data cache techniques enabled by React like 'React Query' or 'SWR'. These libraries provide a declarative approach to fetch requests and caching.
By strategically placing cache at different levels of the component tree, developers can avoid unnecessary re-renders and demand revalidation of components that rely on the same cached value. This significantly improves the efficiency of server requests and minimizes the occurrence of duplicate requests throughout the react component tree.
Next.js also allows for minimal configuration to customize caching behaviors per application needs, including settings for headers like ETag and Cache-Control.
Server Setup
A dedicated cache server can be a game-changer for reducing duplicate requests and managing cache invalidation logic.
Configuring a dedicated cache server typically involves integration with existing database clients or leveraging third-party cache services.
It offers the flexibility to create complex caching rules based on data cache works and request memoization.
For a Next.js application, a data cache server can provide cache entries for frequently accessed data.
Setting up a data cache server might require custom logic for cache misses and hits, such as the initialization of a data cache server with custom logic for cache misses onMiss and hits onHit.
A specified cache lifetime is often required for data retention, ensuring that data is not stored indefinitely.
Persisting Server-Side State
Persisting server-side state is a crucial aspect of building efficient applications. React Query provides a persistQueryClient function that allows us to persist the data store in memory.
This is an experimental feature, so be aware it is subject to change. To use it, we need to pass QueryClient a cacheTime value to override the default during hydration.
The function does most of the heavy lifting for us, so defining this is all we need to go. However, as noted in the docs, for persist to work properly, you need to pass QueryClient a cacheTime value.
React Query provides a persistQueryClient function that allows us to persist the data store in memory. We can use this function to dehydrate and rehydrate the in-memory data store.
Here are the steps to persist server-side state:
- When your query/mutation cache is updated, it will be dehydrated and stored by the persistor you provided.
- When the page is refreshed, the persistor will rehydrate the data store from the dehydrated data.
The data will be stored in local storage by default, but we can customize this behavior as needed. If a cache is found that is older than the maxAge, it will be discarded.
We can confirm that the data has been stored in local storage by checking the browser's local storage. The data will be persisted even after the page is refreshed.
However, be aware that incorrect implementation of the persistor can lead to undesired outcomes. We can mitigate this by passing a buster value for cache busting.
By using the persistQueryClient function, we can efficiently persist server-side state and improve the performance of our application.
Request Management
Request management is a critical aspect of a Next.js application's performance, and it starts with controlling fetch requests. By developing a custom fetch function, developers can introduce caching behavior, implicitly resulting in router cache effectiveness.
This custom fetch function illustrates how to check for a cached response in sessionStorage before executing a new fetch request, preventing duplicate requests for the same data coming from the same URL.
Developers must adhere to best practices for handling data requests to ensure efficiently cached data and avoid stale data. This includes using ETags for demand revalidation, setting appropriate cache entries, and specifying cache lifetime.
Navigating route handlers should incorporate route cache principles, such as creating a route segment for each distinct set of data dependencies, allowing Next.js to cache the results at the route level and reducing server requests.
Implementing request memoization is a technical process that stores the results of fetch requests to avoid repeating identical calls, improving application performance by reducing load times and server load from data requests.
The goal of request memoization is to cache the specific fetch request response, tying it to a unique key, such as a URL or query parameters, so that subsequent user requests for the same data are served from the cache.
Leveraging a static route configuration can ensure that caching mechanisms are employed for content that does not change frequently, substantially reducing incoming requests to the server for uncached data.
The use of a custom hook, like useData, allows client components to request data without concern over redundant fetches, ensuring the no-store policy while elegantly deferring to cached results when available.
Router Management
Router Management is crucial for a Next.js application's performance. By leveraging page-level caching, also known as router cache, developers can ensure that entire pages are preserved effectively, minimizing load times for user requests that access the same data.
On-demand revalidation can be employed to refresh cached data when certain criteria are met. This is often handled via custom route handlers that dictate when and how cached data is updated based on incoming server requests and user requests.
Custom route handlers can be used to trigger cache updates without impacting the cache lifetime of other cache entries. Developers can implement route cache strategies by exporting custom handlers from their API routes, enabling specific fetch requests to trigger cache updates.
Router cache is applied in conjunction with server-side rendering, providing a balance between maintaining up-to-date content and leveraging cached versions to enhance the user's browsing experience.
Router Management
Router Management is crucial for a seamless user experience. Developers can implement route cache strategies by exporting custom handlers from their API routes.
This approach enables specific fetch requests to trigger cache updates without impacting the cache lifetime of other cache entries. By doing so, developers can ensure that entire pages or full route cache are preserved effectively.
Router cache is applied in conjunction with server-side rendering, providing a balance between maintaining up-to-date content and leveraging cached versions to enhance the user's browsing experience. This holistic caching rendering work saves snapshots of pages as they are rendered.
On-demand revalidation is a technique that refreshes cached data when certain criteria are met. This can be handled in Next.js via custom route handlers that dictate when and how cached data is updated based on incoming server requests and user requests.
How Routers Work and Their Benefits
Routers work by capturing the output from the initial request to a given URL and repurposing it for subsequent incoming requests to the same endpoint.
This approach allows routers to serve cached data with rapid consistency, reducing the demand for new data fetches from external data source endpoints.
Router cache streamlines the process of retrieving content by serving a cached response whenever possible, making it a crucial component of Next.js applications.
By managing same URL requests through router cache, Next.js applications can perform optimally and reduce the load on the backend architecture.
GetStaticProps utilizes router cache to cache static product data, with a revalidation time set, allowing for periodic updates while still favoring speed and efficiency for route segment deliveries.
Frequently Asked Questions
How do I cache API response in React js?
To cache API responses in React, use the `idb-keyval` library to store and retrieve data with the `get` and `set` functions, as shown in the code snippet. This allows you to fetch data from a URL, cache it locally, and return the cached result if available.
What is the difference between cache and Unstable_cache?
Cache stores data locally for a single rendering, while unstable_cache shares data across multiple requests, making it suitable for applications requiring persistent caching
Does Next.js cache data?
Next.js caches data by default, but you can opt out if needed. This caching helps improve performance and reduce costs, but may require adjustments to your application
What is the difference between React cache and Next.js cache?
Next.js cache persists responses on disk, reducing backend queries, whereas React cache doesn't modify globalThis, leading to duplicated fetch requests. This difference impacts performance and resource usage in your application
Sources
- https://nextjs.org/docs/app/building-your-application/data-fetching/fetching
- https://nextjs.org/docs/14/app/building-your-application/data-fetching/fetching-caching-and-revalidating
- https://www.dhiwise.com/post/leveraging-nextjs-cache-everything-you-need-to-know
- https://blog.dennisokeeffe.com/blog/2021-09-30-introduction-to-caching-with-nextjs
- https://www.pronextjs.dev/workshops/pro-next-js-workshop-hl06z/data-caching-and-revalidation-with-react-server-components-pkgrz
Featured Images: pexels.com