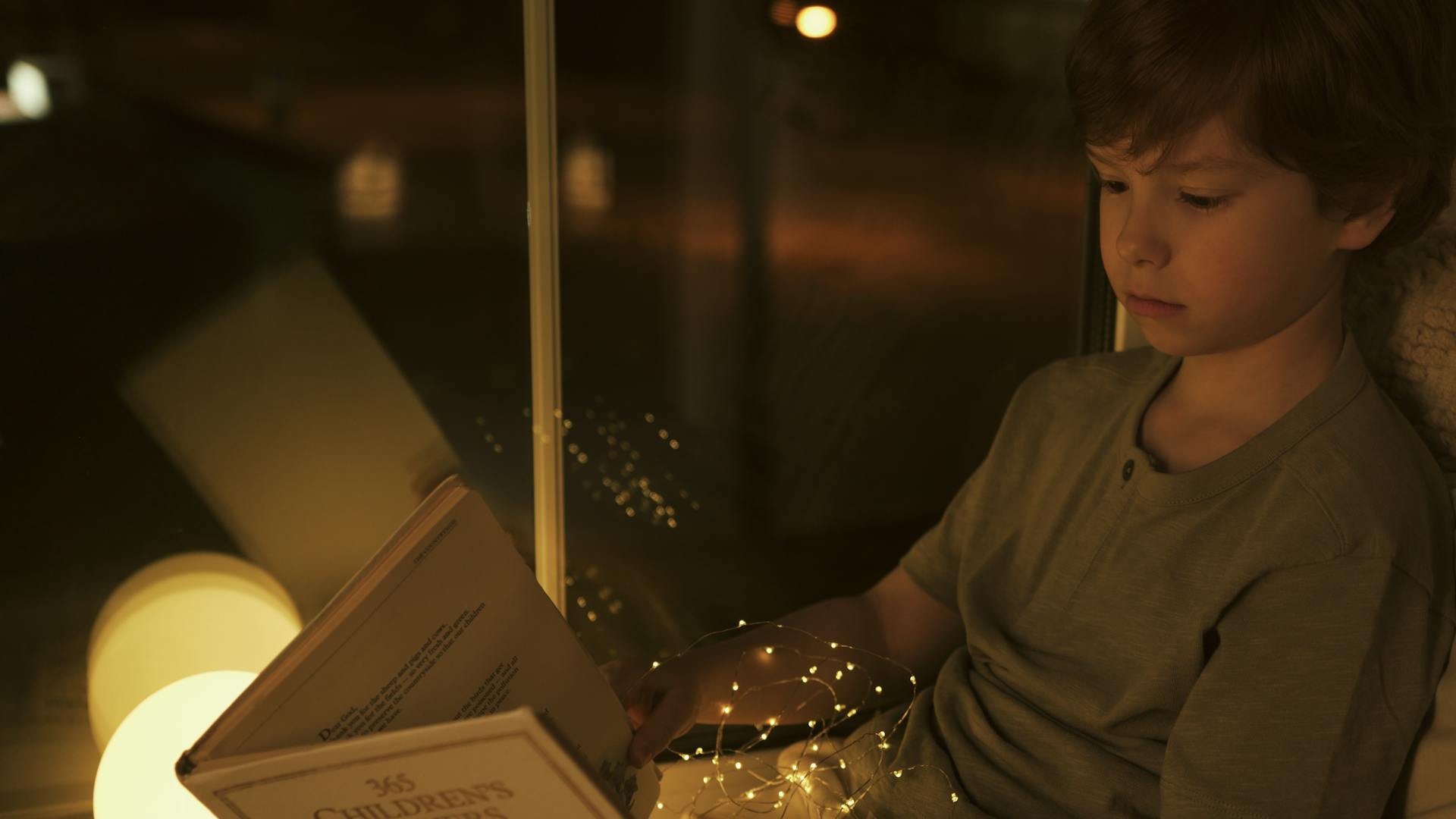
To get started with Storybook Next.js for Next.js projects, you'll need to install the Storybook Next.js package using npm or yarn.
Storybook Next.js is a tool that allows you to develop and test UI components in isolation.
You can create a new Storybook instance by running the command `npx storybook init` in your project directory.
This will create a basic Storybook configuration for you to work with.
Project Setup
To set up a Storybook for your Next.js project, start by initializing it with the command `npx sb init`. This script detects your project type and adapts to it.
You can also give it a few hints, such as specifying the use of Webpack 5, which is used in Next.js v11 and later. To do this, run the command `npx sb init` with the `builder` option.
With your Storybook set up, you can now import your Home page and start customizing it.
New Project Setup
To set up a new project, start by initializing a new Storybook with Webpack 5 using the command npx sb init. This script will detect your project type and adapt to it, but you can also give it a few hints.
You can use the builder option to specify that you want to use Webpack 5, which is especially useful for Next.js v11 and later projects. This will get you improved integration and performance.
Now that your Storybook is set up, you can start working on your Home page. But first, you need to import global styles to make it look decent.
Project Structure
The project structure is where Storybook adds a stories folder with example components like the button. This folder is located inside the src directory.
The button component itself is implemented in a file called Button.tsx. Its stories are defined in a separate file called Button.stories.ts.
In the Button.stories.ts file, you'll find a meta block that specifies metadata for the component, like its title and location in the navigation bar. The component itself is also defined in this file.
The meta block also includes an argTypes section that defines the available props for the button and how they should appear in the Storybook UI.
Individual stories for the button component are defined after the meta block, each showing a different use case or variation of the component with specific props.
Content Creation
Next.js and Storybook have extremely compatible component models, making Storybook a great component-driven development environment for Next.js. This compatibility allows for seamless integration of components for pages in Next.js and documentation/testing components in Storybook.
To create a story for a full Next.js page, you'll need to create a new file in the /stories/pages directory, such as /stories/pages/home.stories.jsx. This file will contain the story for your page, including the component and any other relevant details.
Here's a step-by-step guide to creating a story for your Next.js home page:
- Create a new file /stories/pages/home.stories.jsx
- Import /pages/index.js
- Export a default story object, with title and component properties
- Export a story for Home
Create Full Page Content
You can create stories for full Next.js pages, which is a great way to drive component-driven development. This is made possible by the extremely compatible component models of Next.js and Storybook.
To get started, create a new file in the /stories/pages directory, for example, /stories/pages/home.stories.jsx.
Import the /pages/index.js file in this new file.
Export a default story object with title and component properties.
Export a story for Home.
This process is quite straightforward and allows you to create full page content with ease.
Project Story Creation
Creating stories for your Next.js project is a great way to showcase your components in a component-driven development environment. This makes it easy to document and test your components, as well as collaborate with others.
To create a story for a full Next.js page, you'll need to create a new file in the /stories/pages directory and import the corresponding page component. For example, to create a story for the home page, you'll need to create a new file called home.stories.jsx and import the /pages/index.js component.
Here's a step-by-step guide to creating a story for your Next.js home page:
- Create a new file called home.stories.jsx in the /stories/pages directory.
- Import the /pages/index.js component.
- Export a default story object with a title and component property.
- Export a story for the Home page.
By following these steps, you'll be able to create a story for your Next.js home page and start exploring the possibilities of Storybook.
If you're working on a Next.js and Tailwind project, you can create stories for your components by creating a new file in the /stories directory and importing the corresponding component. For example, to create a story for a card component, you'll need to create a new file called card.stories.js and import the card component.
Here's a table showing the steps to create a story for a Next.js and Tailwind project:
By following these steps, you'll be able to create stories for your components and start exploring the possibilities of Storybook.
Image Optimization
Image optimization is a crucial aspect of Next.js and Storybook integration. Next.js v10 and newer include the Next.js Image component, which optimizes file size, visual stability, and load times.
To handle images in Storybook, we need to configure it in two important ways. First, we need to serve the Next.js public directory in Storybook. Second, we need to add the unoptimized prop to the Next.js Image component in all stories.
The unoptimized prop is used to de-optimize Next.js Image only in stories. This is necessary because Storybook runs components in isolation of Next.js framework-integrations. By using the unoptimized prop, we can utilize Next Image's prop APIs and attributes without requiring the Next.js dev server to be running.
Here's a summary of the steps to de-optimize Next.js Image in stories:
- Serve the Next.js public directory in Storybook
- Add the unoptimized prop to the Next.js Image component in all stories
By following these steps, you can ensure that images are optimized for production, but not for Storybook. This allows you to test and develop your components without the overhead of Next.js Image optimization.
Tailwind CSS
To get started with Tailwind CSS in a Next.js application, you'll need to run a command to install it and its dependencies. This involves changing directory to the new app and running a command to generate the required configuration files.
You'll also need to update the tailwind.config.js file to include files from your project's /pages and /components directories. This allows Tailwind to access the necessary styles.
To enable Tailwind CSS in your Storybook environment, you'll need to add a webpackFinal option to the .storybook/main.js config file. This imports the global stylesheet and supports next-image, ensuring both Tailwind CSS and next-image function properly in Storybook.
Tailwind Basics
To get started with Tailwind CSS, you'll need to create a new Next.js application by running the command `npx create-next-app my-app`. This will create a basic Next.js project that you can then customize.
Tailwind CSS requires two configuration files: `tailwind.config.js` and `postcss.config.js`. You can generate these files by running the command `npx tailwindcss init -p`. This will create the necessary configuration files for Tailwind CSS to work properly.
To use Tailwind's utility classes, you'll need to update the `tailwind.config.js` file to include the directories where your project's components and pages are located. This will allow Tailwind to automatically apply its utility classes to your components.
The `@tailwind` directives should be added to the top of the `styles/global.css` file to enable Tailwind's utility classes in your project. This will give you access to Tailwind's extensive library of utility classes, which you can use to style your components.
To create a new story in Storybook, you'll need to create a new component on which the story will be based. This involves creating a new directory for the component, such as a `Card` folder, and then creating two files: one for the component's JavaScript code and another for its CSS styles.
Adding Tailwind CSS Support
To get Tailwind CSS working with Storybook, you need to add a webpackFinal option to the .storybook/main.js config file. This will instruct Storybook to build with the necessary dependencies required by Tailwind CSS.
Storybook doesn't work with Tailwind CSS by default, so you'll need to make this adjustment to get it up and running.
To support next-image, you'll also need to import the global stylesheet into Storybook. This will ensure that both Tailwind CSS and next-image function properly in your Storybook environment.
The fix for missing Tailwind styles in Storybook is simple: just import Tailwind styles into Storybook by adding an import statement at the top of the .storybook/preview.ts file.
Sources
- https://storybook.js.org/blog/get-started-with-storybook-and-next-js/
- https://blog.logrocket.com/building-next-js-app-tailwind-storybook/
- https://www.lost-pixel.com/blog/integrating-next-js-with-tailwind-and-storybook
- https://www.pronextjs.dev/workshops/pro-next-js-workshop-hl06z/setting-up-storybook-with-next-js-2vxd8
- https://www.npmjs.com/package/@storybook/nextjs/v/0.0.0-pr-24604-sha-a206c3eb
Featured Images: pexels.com