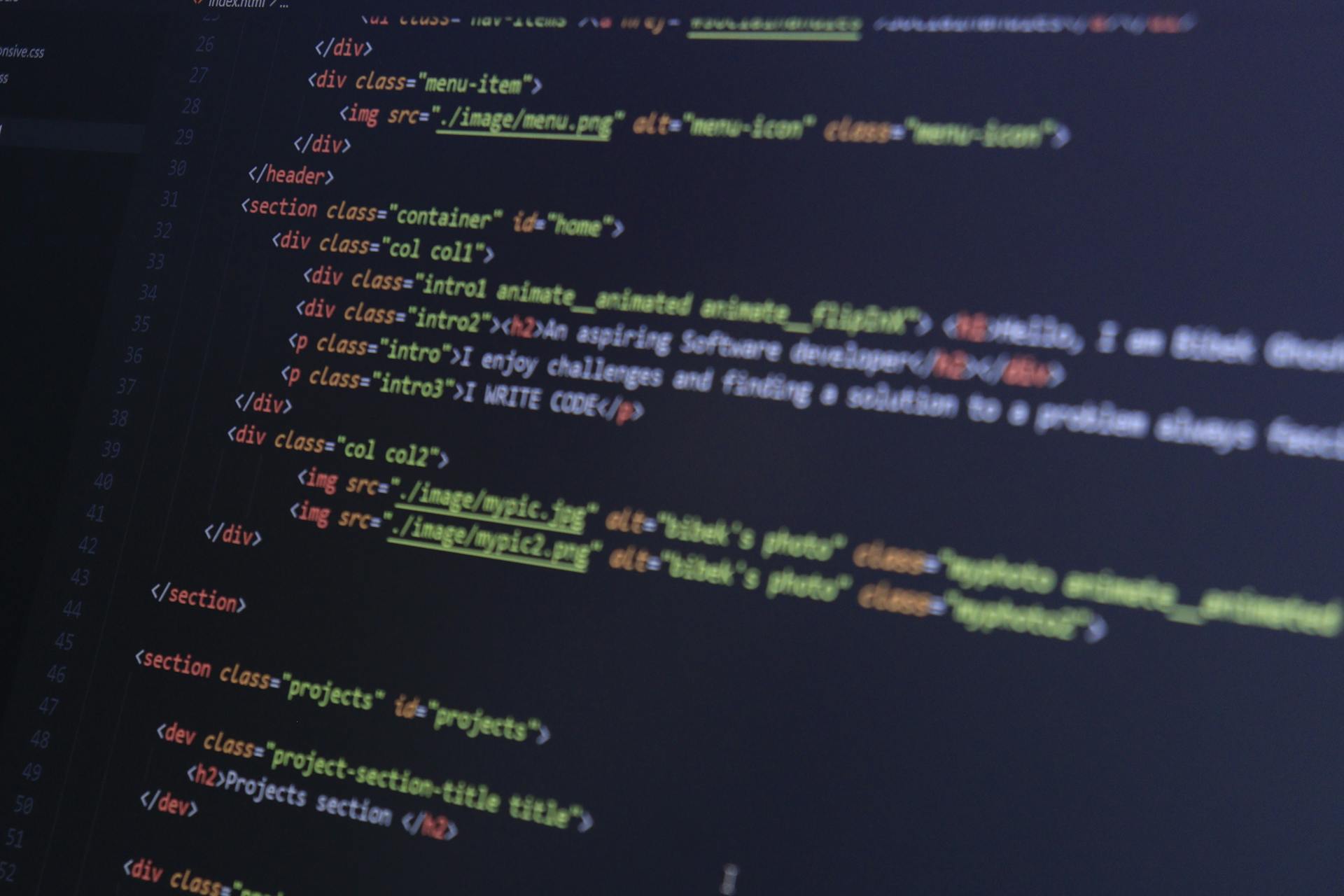
Nextjs Generatestaticparams is a powerful tool that allows you to pre-render pages at build time, improving performance and reducing server load.
By using GetStaticProps, you can fetch data on the server and pass it to the page component at build time, making it possible to pre-render pages with dynamic data.
This approach is particularly useful for sites with a large number of pages or complex data fetching logic.
With Nextjs Generatestaticparams, you can also take advantage of automatic code splitting, which reduces the amount of code that needs to be loaded on the client-side, resulting in faster page loads.
Consider reading: Speed up a Next Js Application Fetching Data
Dynamic Routing
Dynamic Routing is a powerful feature in Next.js that allows you to create flexible and reusable routes. By using dynamic segments, you can generate static paths for your pages, making them accessible via URLs with parameterized values.
For example, in a route like `/student/[id]`, the `[id]` is a dynamic segment that can be replaced with a specific value, such as `1`, `2`, or `3`, resulting in three different versions of the page being statically generated.
You can have multiple dynamic segments in a route, like in `/student/[name]/[subject]/page.tsx`, where `[name]` and `[subject]` are both dynamic segments that can take various combinations of values.
A catch-all dynamic segment, denoted by `[...slug]`, allows for variable path lengths, enabling you to create routes like `/student/[...slug]/page.tsx` that can handle different combinations of values.
Here are some key takeaways about dynamic routing in Next.js:
- Dynamic segments can be used to create reusable routes.
- Multiple dynamic segments can be used in a single route.
- Catch-all dynamic segments can handle variable path lengths.
By leveraging dynamic routing, you can create more flexible and maintainable routes in your Next.js application.
Static Site Generation
Static Site Generation is a powerful feature in Next.js that allows you to pre-render pages at build time, making your site super fast and efficient.
By default, any page component in the app folder is a Server Component, and its data is fetched and cached by Next.js every time we build a new version of our application. This is equivalent to exporting a getStaticProps function from a component in the pages directory.
Broaden your view: Next Js Build Time Slow
Server Components support async/await by default, and suspend the rendering of the component until the data has been fetched. This means we don’t need to handle error or loading states in our component, keeping our rendering logic clean.
To statically render all paths at build time, you can supply the full list of paths to generateStaticParams. However, to statically render a subset of paths at build time, and the rest the first time they're visited at runtime, you should return a partial list of paths.
If you want to prevent unspecified paths from being statically rendered at runtime, you can add the export const dynamicParams = false option in a route segment. This will ensure that only paths provided by generateStaticParams will be served, and unspecified routes will 404 or match (in the case of catch-all routes).
You can generate params for dynamic segments above the current layout or page, but not below. For example, given the app/products/[category]/[product] route, a child route segment's generateStaticParams function is executed once for each segment a parent generateStaticParams generates.
Here are some examples of how to use generateStaticParams:
Paths and Routes
Next.js allows you to define static paths for your application, which are generated based on dynamic data using the getStaticPaths function. This function returns a list of paths that should be statically generated, including parameters for dynamic content generation.
Static paths can include parameters, enabling you to generate dynamic content. For example, in /posts/[id], [id] is a parameter representing a dynamic value.
Next.js supports dynamic routes, where parts of the URL are treated as parameters. This allows for variable path lengths, such as in the route `app/student/[...slug]/page.tsx`.
The generateStaticParams function returns an array of objects, each containing an array within the slug property. This represents different combinations of values, resulting in multiple versions of the page being statically generated.
Here are some key differences between static paths and dynamic routes:
By combining static paths and dynamic routes, you can create catch-all dynamic segments like `[...slug]`, allowing for variable path lengths and multiple versions of the page to be statically generated.
Data Fetching
Data Fetching is a crucial step in Next.js, and it's used to pre-render pages with data. This process starts after static paths are defined.
Next.js uses the getStaticProps function to fetch data for a specific path based on its parameters. This function runs at build time and receives the parameters of the current path as input.
The getStaticProps function is essential for fetching data, and it's used in conjunction with dynamic routes to fetch data for each path.
Here's a quick rundown of how data fetching works in Next.js:
- Once the static paths are defined, Next.js needs to fetch data for each path to pre-render the pages.
- The getStaticProps function is used to fetch data for a specific path based on its parameters.
- This function runs at build time and receives the parameters of the current path as input.
Implementation and Errors
Implementing generateStaticParams in Next.js can be a game-changer for optimizing performance. By pre-generating all possible blog post pages during the build process, we can ensure that each page is readily available without the need for additional API calls.
This approach eliminates the need to hit the API every time a user accesses a blog post URL, significantly reducing loading times and minimizing the number of API requests. By serving pre-rendered pages, we enhance the overall performance and user experience of the website.
To implement generateStaticParams, we'll follow Step 4 of the process, which involves implementing this feature in Next.js. This will allow us to generate static parameters at build time, saving them in the .next folder.
On a similar theme: Next Js 13 Api Routes
Implementing
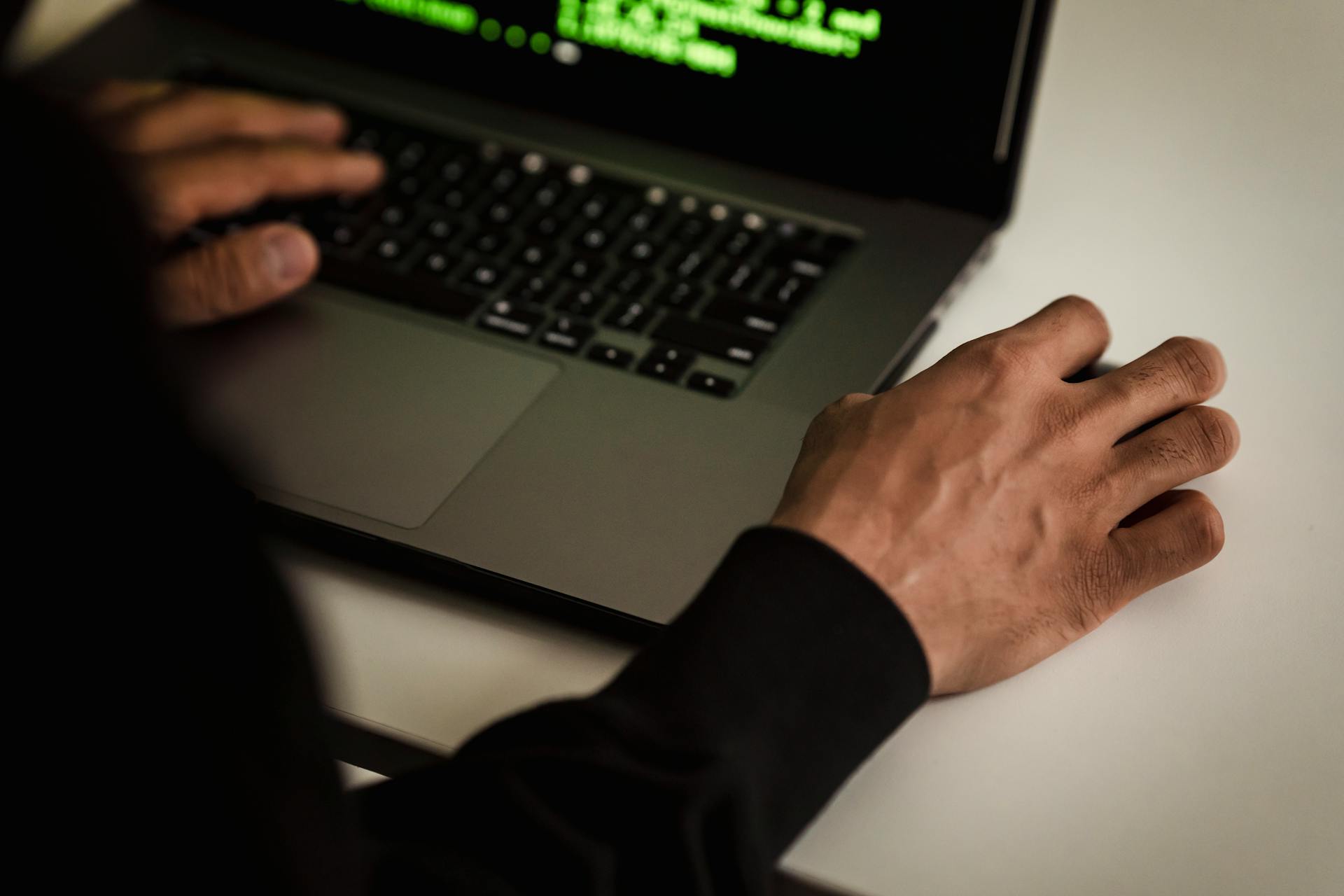
Implementing static parameter generation in Next.js is a great way to optimize performance by serving pre-rendered pages. This feature, known as static parameter generation, allows us to pre-generate all possible blog post pages during the build process.
To do this, we'll implement the generateStaticParams function, which is used to define a list of paths that should be statically generated based on dynamic data. Each path can include parameters, allowing for dynamic content generation.
The getStaticPaths function is used to define a list of paths that should be statically generated based on dynamic data. This function is typically used in combination with the generateStaticParams function.
Here's a key point to remember: Next.js generates the generateStaticParams at build time, saving them in the .next folder. This means that the HTML files for each blog post page are pre-generated during the build process.
To optimize performance, we need to run the build command to generate the optimized production build of the Next.js project. By doing so, we ensure that each page is readily available without the need for additional API calls, reducing loading times and minimizing the number of API requests.
Readers also liked: Next Js Performance Analyzer
Export Fails Building Paths
Exporting fails can be a real challenge when building paths. It's essential to understand the common pitfalls to avoid.
A failed export can occur when the path is not properly defined, as seen in the "Path Not Found" error. This can happen when the path is misspelled or doesn't exist.
Exporting fails can also occur when the export format is not supported. For example, if you're trying to export a file to a format that your software doesn't support, the export will fail.
The "Invalid File Path" error can occur when the path contains special characters or is too long. This can be a common issue when working with long file names or paths with special characters.
In some cases, exporting fails can be caused by a lack of permissions to access the export location. This can happen when you're trying to export a file to a location that requires administrative privileges.
Next.js Features
Next.js has built-in support for internationalized routing, which allows you to create routes that can handle different languages and locales.
The framework also includes a powerful API route feature that allows you to create serverless APIs with ease.
Next.js has a built-in feature called getStaticProps that allows you to pre-render pages at build time, which is useful for SEO and performance.
The framework also supports internationalized pages, which can be used to create pages that can handle different languages and locales.
Next.js has a feature called getStaticPaths that allows you to pre-render pages at build time, which is useful for SEO and performance.
This feature is particularly useful when working with data that requires dynamic routes, such as blog posts or products.
Check this out: Next Js Performance Analyzer Library Npm
Sources
- https://supabase.com/blog/fetching-and-caching-supabase-data-in-next-js-server-components
- https://nextjs.org/docs/pages/api-reference/functions/get-static-paths
- https://nextjs.org/docs/app/api-reference/functions/generate-static-params
- https://www.geeksforgeeks.org/next-js-functions-generatestaticparams/
- https://stackoverflow.com/questions/tagged/generatestaticparams
Featured Images: pexels.com