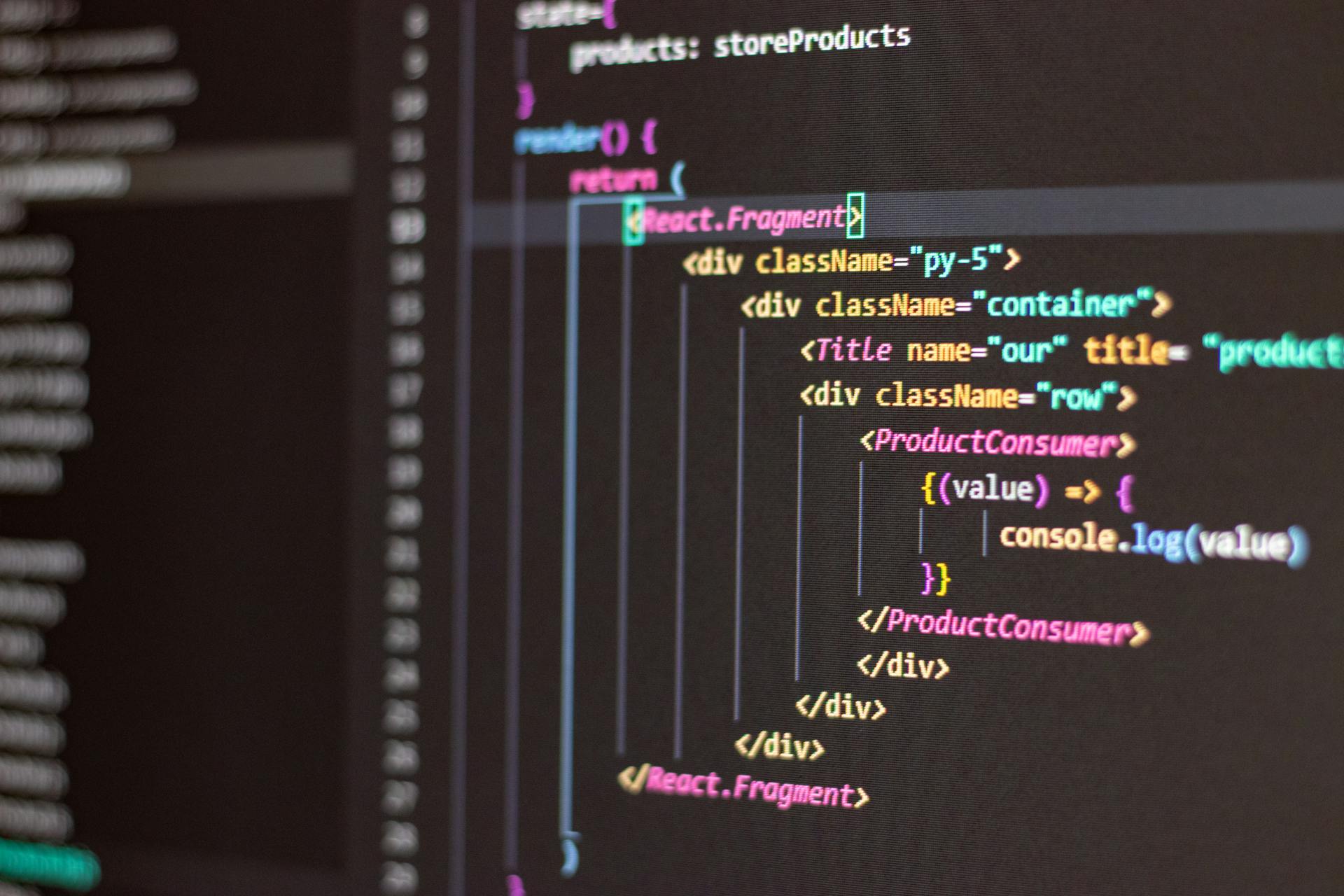
Next Js Performance Analyzer is a powerful tool that can significantly boost your app's performance. It's designed to help developers identify and fix performance bottlenecks in their Next Js applications.
By using Next Js Performance Analyzer, you can optimize your app's code and improve its loading speed, which is essential for providing a good user experience.
To get the most out of Next Js Performance Analyzer, you need to understand how it works, and what features it offers.
Check this out: Next Js Component Rendering Analyzer
Understanding Performance
New Relic's APM page provides a visual representation of your application's server-side data, including transaction time, Apdex score, throughput, and error rate.
These metrics help you identify performance bottlenecks and areas for improvement. By analyzing this data, you can optimize your application's performance and provide a better user experience.
The Next.js Bundle Analyzer provides a graphical representation of your JavaScript bundles, helping you identify large modules and areas for optimization.
To optimize your app's performance, focus on the following key areas: vendor code, common code, and duplicate code. By identifying and targeting the largest chunks of your bundle, you can reduce overall size and improve load times.
If this caught your attention, see: Nextjs Performance
Here are some common issues that can affect your app's performance:
- Large image file sizes
- Duplicate npm packages
- Unused npm packages
- Heavy JavaScript files
To address these issues, you can try the following:
- Compress or resize images with large file sizes
- Remove duplicate npm packages
- Remove unused npm packages
- Use minification, tree shaking, and code splitting to optimize JavaScript files
Understanding the Output
The Next.js Bundle Analyzer provides a graphical representation of your JavaScript bundles, making it easier to identify areas for optimization. Each module is represented as a rectangle, with larger rectangles indicating heavier modules that take up more space and time to load.
To get the most out of the Bundle Analyzer, focus on the following key areas: Vendor Code, Common Code, and Duplicate Code. Vendor Code checks if third-party libraries like React or Lodash are excessively large. Common Code ensures that commonly used code is being shared effectively between pages. Duplicate Code looks out for duplicate code that appears in multiple bundles.
Large rectangles in the Bundle Analyzer indicate heavy modules that take up more space and time to load. To optimize your application's performance, identify and target the largest chunks of your bundle. By reducing the overall size of your bundle, you can improve load times and user experience.
Worth a look: Next Js Build Time Slow
Here are some key metrics to check in the Bundle Analyzer:
By analyzing these metrics and identifying areas for optimization, you can improve the performance of your Next.js application and provide a better user experience.
Features and Use Cases Comparison
Understanding Performance is crucial for any web application, and one key aspect is bundle size optimization. Next.js developers have three options for analyzing their bundles: Next.js Built-in Bundle Analyzer, Webpack Bundle Analyzer, and Source-map-explorer.
The Next.js Built-in Bundle Analyzer is a great choice for developers who want a quick and easy setup without leaving the Next.js ecosystem. It provides a straightforward visualization of your bundles, helping you to identify large modules and chunks.
Webpack Bundle Analyzer offers more detailed insights and customization options, making it a good choice for developers who want to dive deeper into their webpack bundles. This is especially useful for developers who are already familiar with Webpack's configuration.
A unique perspective: Next Js Performance Analyzer Library Npm
Source-map-explorer works with source maps to show you a more accurate representation of your source code before Webpack transforms it. This can be useful for analyzing the original size of your code and how it contributes to the overall bundle size.
Here's a comparison of the three tools:
Optimizing Performance
To optimize performance, start by analyzing your Next.js bundles using the @next/bundle-analyzer tool. This will help you identify large dependencies, duplicate code, and unused packages that can slow down your app.
Large image file sizes can significantly slow down an app's load speed, so consider compressing or resizing images with large file sizes. Duplicate npm packages and unused packages can also contribute to the app's bundle size, so remove them to improve performance.
By optimizing your bundles, you can improve load times, resulting in a snappier, more responsive app. Smaller bundles load faster, and optimized bundles reduce JS amounts that require parsing and execution. Faster websites may rank higher in search engines.
Here are some ways to optimize your app's performance:
- Compress or resize images with large file sizes
- Remove duplicate npm packages
- Remove unused npm packages
- Use minification, tree shaking, and code splitting to optimize JavaScript files
Configuring
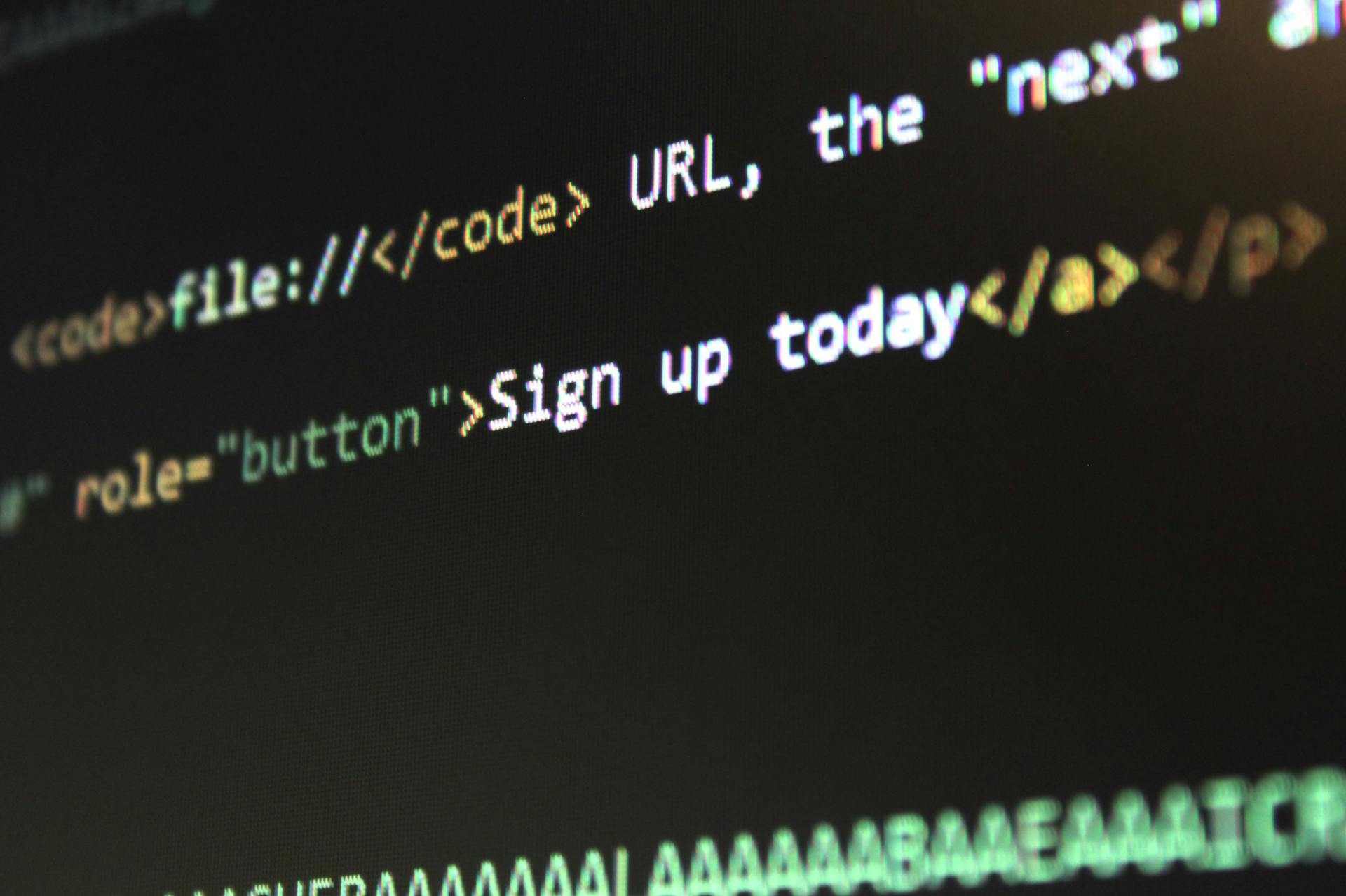
To configure your Next.js application for optimal performance, you need to modify the next.config.js file. This configuration ensures that the modules supported by New Relic are not mangled by webpack, and it externalizes those modules.
First, create or update the next.config.js file in your project root with the following content:
```javascript
module.exports = {
// ... other configurations ...
experimental: {
externalDir: true,
},
};
```
Modify your dev and start npm scripts by amending the scripts section of package.json file to allow your application to run with Node’s -r option, which will preload @newrelic/next middleware.
Add the newrelic.jsAMP agent configuration file to the root directory of your project, and use NEW_RELIC_APP_NAME and NEW_RELIC_LICENSE_KEY in your .env file as shown in an example .env file for your application.
To customize your webpack configurations in Next.js, you can add the webpack property to your next.config.js file and pass your configurations on to it. This allows you to modify your webpack settings by adding loaders, plugins, or other optimization configurations.
Explore further: Next Config Js
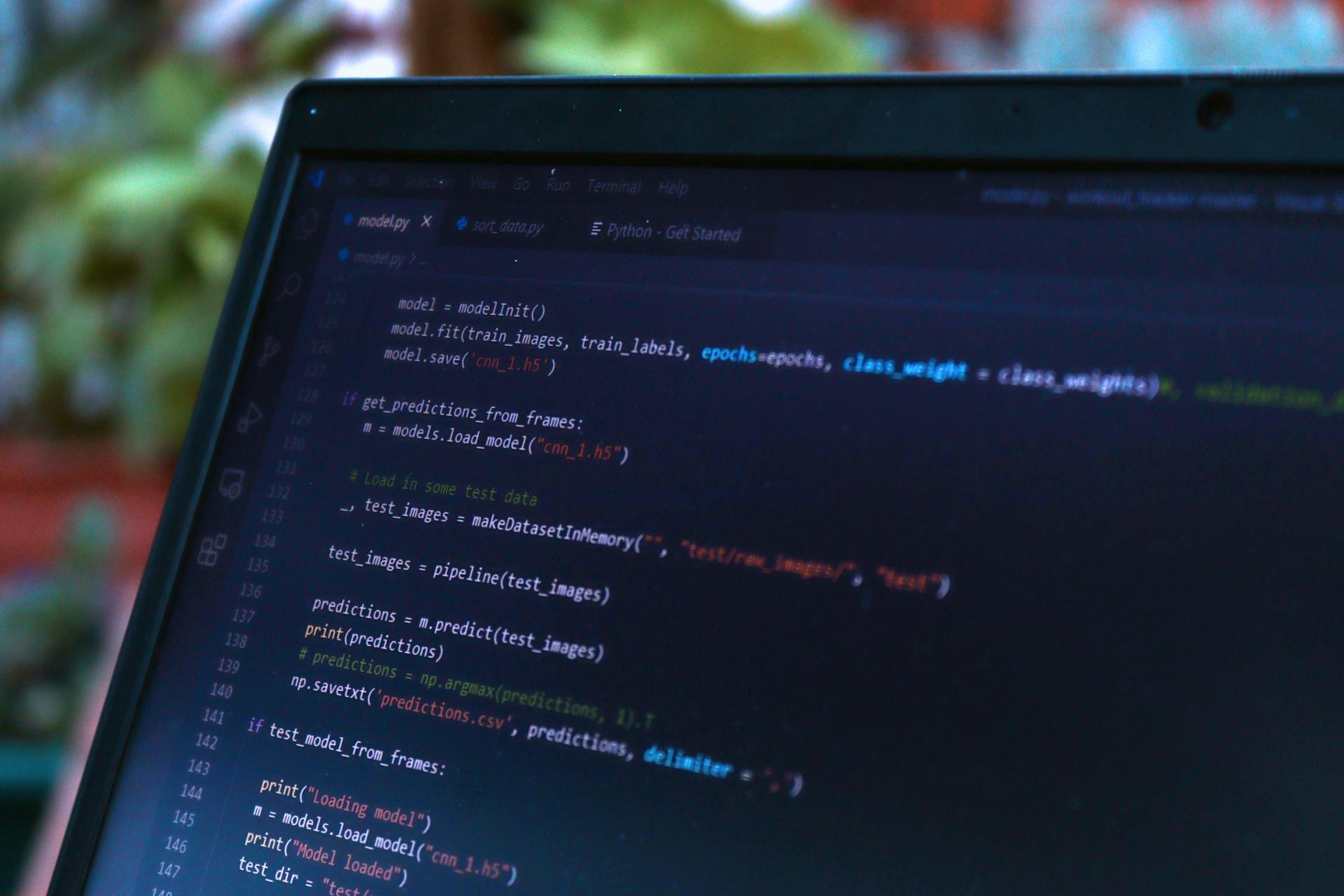
Here are some options you can customize in your webpack configuration:
By following these steps, you can configure your Next.js application for optimal performance and gain insights into your application's bundle sizes and assets.
Here's an interesting read: Speed up a Next Js Application Fetching Data
Optimizing Performance
Optimizing your Next.js app's performance is crucial for delivering a seamless user experience. To start, make sure you're using the next/image component, which automatically optimizes images on-demand.
Large images and fonts can significantly inflate your bundle size, but Next.js has built-in support for optimizing them. Compress or resize images with large file sizes to reduce load times.
Unused code can also contribute to a bloated bundle size. Use tree shaking to remove unused code, and avoid full library imports to reduce unnecessary code.
Code splitting is another technique to reduce load times. Next.js already does this for you, but you can also manually optimize your pages by using dynamic imports.
A bundle analyzer can help you detect duplicate code across bundles, which can lead to unnecessary bloat and increased js bundle sizes. Regularly audit your dependencies to remove any that are no longer used or are unnecessarily large.
Here are some key metrics to monitor for improving user experience:
- LCP (Largest Contentful Paint): measures the time it takes for the largest element on the page to load
- FID (First Input Delay): measures the time it takes for the page to respond to user input
- CLS (Cumulative Layout Shift): measures the visual stability of the page
By monitoring these metrics and making informed decisions, you can improve the loading, interactivity, and visual stability of your application.
Analyzing Performance
To analyze performance in Next.js, you can use tools like New Relic, which provides a UI to view server-side data and performance metrics. The APM page in New Relic offers visualizations of important metrics like transaction time, Apdex score, throughput, and error rate.
You can also use the Next.js bundle analyzer to identify large dependencies and optimize app performance. This tool helps you spot large modules, detect duplicate code, and analyze bundle size metrics.
To get started with the bundle analyzer, you need to set the ANALYZE environment variable to true and run the analysis command. The command will create an analyze folder with three files: client.html, nodejs.html, and edge.html.
The bundle analyzer report provides valuable insights into your app's performance, including the size of each module and the files contributing to the minified code. You can use this information to optimize JavaScript files, compress or resize images, and remove unused npm packages.
Worth a look: Next Js Build Time Analyzer
Here are some key metrics to look out for in the bundle analyzer report:
- Large image file sizes can slow down an app's load speed
- Duplicate npm packages increase the app's bundle size
- Unused npm packages contribute to the app's bundle size and slow down its load speed
- Heavy JavaScript files add to the app's bundle size
To optimize these issues, you can consider the following steps:
- Compress or resize images with large file sizes
- Remove duplicate npm packages
- Remove unused npm packages
- Use minification, tree shaking, and code splitting to optimize JavaScript files
By following these steps and using the Next.js bundle analyzer, you can identify potential bottlenecks and areas for improvement in your app's performance. Regularly running the analyzer will help you monitor changes in bundle size and catch any regressions.
Sources
- https://newrelic.com/blog/how-to-relic/how-to-monitor-app-based-router-nextjs-application
- https://www.catchmetrics.io/blog/reducing-nextjs-bundle-size-with-nextjs-bundle-analyzer
- https://blog.logrocket.com/how-to-analyze-next-js-app-bundles/
- https://www.dhiwise.com/post/next-bundle-analyzer-your-key-to-optimizing-next-js-app
- https://www.qed42.com/insights/optimizing-your-next-js-application-using-analytics-metadata-and-bundle-analyser
Featured Images: pexels.com