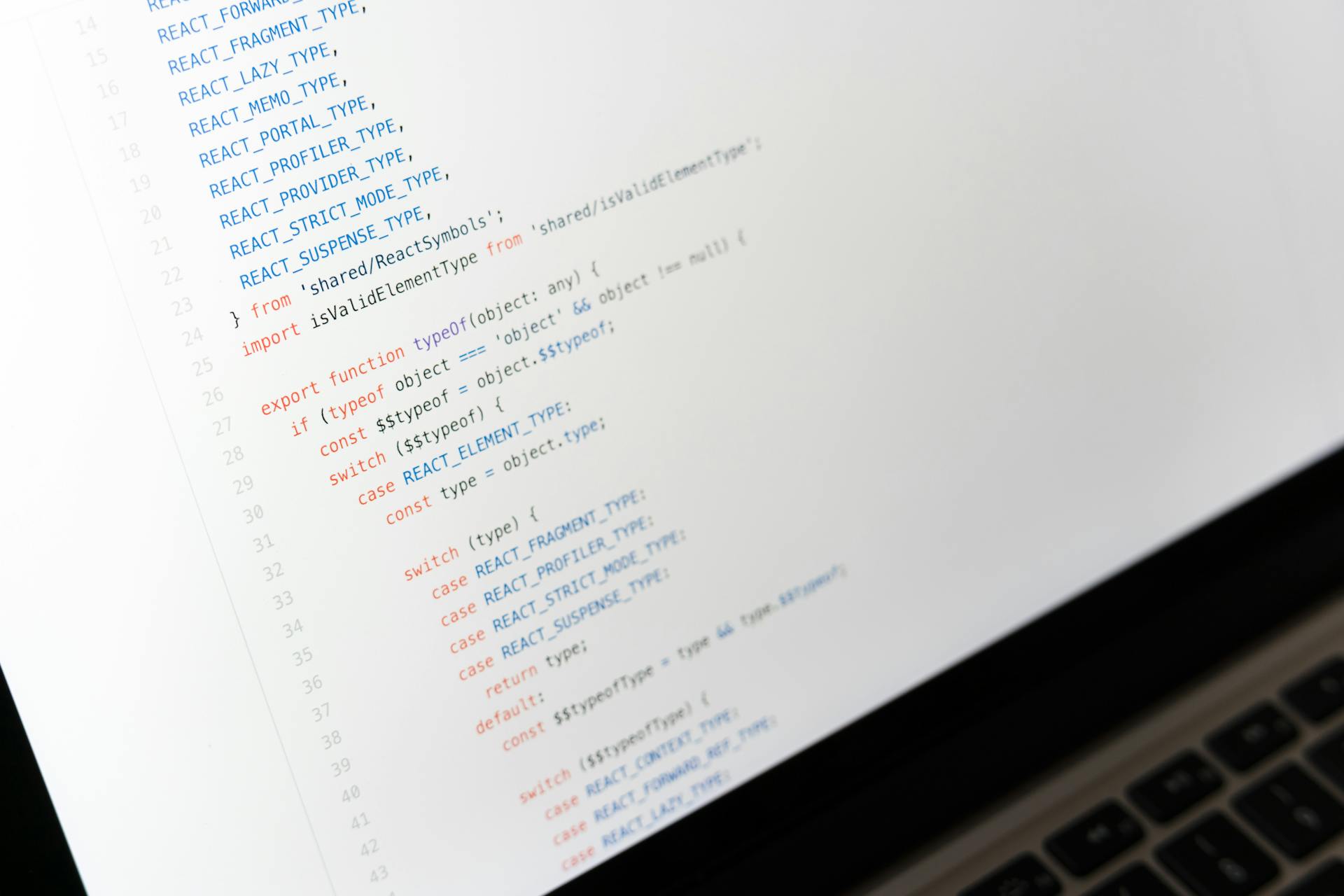
Next.js and Express can be used together to create a powerful and flexible web application. Both frameworks are built on top of Node.js, allowing for seamless integration.
Express is a popular Node.js web framework that provides a robust set of features for building web applications, including routing, middleware, and request handling.
By using Next.js with Express, you can leverage the strengths of both frameworks to create a fast, scalable, and maintainable application.
To get started, you'll need to set up a new Next.js project using the `npx create-next-app` command, and then configure Express to work with Next.js by creating a new Express instance and mounting it to the Next.js app.
Advantages of Using Next.js with Express
Using Next.js with Express brings a number of advantages that can improve your development experience and the final product.
One of the biggest advantages is the ease of adoption by other developers, thanks to a growing community of experienced developers and contributors. This means you can easily find solutions to any issues you might face while learning and using Next.js.
Express also allows you to integrate complex server-side logic or specific features that Next.js alone might not support. This makes it a great choice for applications that require advanced functionality.
With Next.js and Express, you get automatic feature that helps you optimize images, including lazy-loading, preloading of critical images, correct sizing across devices, and support for modern formats. This can greatly improve the user experience and performance of your application.
Here are some of the key features you can expect when using Next.js with Express:
Using Express with Next.js also allows for easy creation of API routes or endpoints during application development, which can greatly simplify the development process.
Setting Up Next.js with Express
To set up Next.js with Express, start by initializing your project with `npm init` or `yarn init` to create a `package.json` file. This will be the foundation of your project.
Next, install the necessary dependencies, including Next.js and Express, using `npm install next react react-dom express` or `yarn add next react react-dom express`.
Once you've installed the dependencies, create your Next.js app using `npx create-next-app` and follow the prompts. This will set up the basic structure of your project.
To integrate Express into your Next.js app, you'll need to create a server folder and a server entry point. Then, install Express and its TypeScript definitions using `npm install express` and `npm install @types/express`.
However, you'll encounter an issue with the `tsconfig.json` file, which is set to "esnext" by default. To resolve this, create a new `tsconfig.server.json` file and extend it from `tsconfig.json`. This will allow you to use the "commonjs" module pattern, which is required for Express.
Here are the key options you'll need to include in your `tsconfig.server.json` file:
- "extends": "./tsconfig.json" - Gets all options from tsconfig.json
- "compilerOptions.module": "commonjs" - Use commonjs as the module pattern
- "compilerOptions.outDir": "dist" - Compile .ts files to .js in the dist directory
- "compilerOptions.noEmit": true - Override the value to tell TS compiler to compile and output server files
- "include": ["server"] - Only compile .ts files in the server/ directory
By following these steps, you'll be able to set up Next.js with Express and take advantage of the benefits of using both frameworks together.
Handling Backend Tasks
Next.js provides a robust solution for handling backend tasks, thanks to its built-in server-side rendering capabilities and API routes.
You can write server-side code directly within your Next.js application using API routes, which can handle tasks like interacting with a database, processing forms, or integrating with third-party APIs.
Next.js's built-in server can handle SSR and SSG, but if you need more flexibility, you can use a custom server with Express.
Here are the key features of Next.js's backend handling:
- Built-in Server: Handles SSR and SSG internally
- API Routes: Allows server-side code within the Next.js application
- Custom Server: Uses Express or any other Node.js server framework
With Next.js and Express, you can efficiently manage diverse API requests while maintaining top-notch performance.
Custom Server vs Cloud Hosting
Using a custom server for Next.js can be a good fit if you have custom needs and infrastructure.
Next.js websites are static, which means they don't have a direct connection with your application database, user information, third-party integration keys, and any other sensitive information. This is a significant advantage for data security.
You can use Next.js to build across several platforms, including Static web applications, Progressive Web Apps (PWA), and even mobile apps. This flexibility is a major benefit of using Next.js.
Here are some key features that are sacrificed when using a custom Next.js server:
- Performance optimizations
- Automatic static optimization
- Serverless functions
- Other features
Because Next.js websites load fast and are easy to scan by search engine bots, this improves organic traffic to the website and can translate to conversions and sales for the owner of the website.
Routing and SEO
Next.js and Express play nicely together, and one of the key benefits is how they handle routing and SEO.
Express seamlessly handles API routes and routing, maintaining a clean architecture that integrates SEO optimization naturally into your development process.
With Next.js' built-in Server-Side Rendering (SSR), your content is rendered on the server, making it easily accessible to search engine crawlers.
This ensures your web pages are highly crawlable and indexable, ultimately boosting your site's search engine rankings.
This combination of Express and Next.js excels in SEO optimization, making it a great choice for web applications that need to be search engine friendly.
Authentication and Security
Using Next.js with Express provides a powerful combination for authentication and security. This alliance ensures that your application remains resilient against security threats.
Express offers a plethora of middleware options to bolster your application's security. This makes implementing authentication, authorization, and data validation a seamless process.
Security is paramount in the digital landscape, and this powerful combination of Express and Next.js safeguards sensitive user data. Providing a secure and trustworthy user experience is essential for any application.
Troubleshooting and Deployment
When integrating Next.js with Express, you may encounter issues with routing. To resolve this, verify that you're using the correct middleware order, with Express.js before Next.js.
Using a single instance of Express.js for all routes can simplify your application structure. This is especially useful for smaller applications with a straightforward routing system.
To avoid potential conflicts, ensure that you're not using the same route path in both Express.js and Next.js. This can lead to unexpected behavior and errors.
Fix 404 Error
When you visit an unknown page, you'll get an Internal Server Error instead of a 404 not found message. This is because Next.js no longer handles errors when integrating with a custom server.
To fix this, create a custom error page and populate it with code that will display a user-friendly error message.
Visit an unknown page again, and you should see your custom error message instead of the Internal Server Error.
Let's Go Production
Before we can put our application into production, we need to compile both Next.js and server code to .js.
To do this, we'll modify the scripts in our package.json file. We'll create three new scripts: build:server, build:next, and build. The build script will run both build:server and build:next.
Here's a breakdown of the changes we'll make to our package.json file:
- build:server: This script will build our server code to the dist directory.
- build:next: This script will build our Next.js application.
- build: This script will run both build:server and build:next.
- start: This script will run our server using node dist/index.js, instead of next start.
Once we've made these changes, we'll be able to run our application in production mode.
Why Use Next.js with Express
Using Next.js with Express is a fantastic idea because it allows you to easily build web socket features in your applications. You can also develop custom or extra logic for your Next.js routes, build Express middleware and fetch data for Next.js pages before they are rendered, and easily build backend features, such as file uploading, media file compression, and more.
Express's middleware system enables you to handle various aspects of your application's request-response cycle efficiently. This means you can create custom routes effortlessly, enhancing your application's routing capabilities.
One of the main advantages of using Express with Next.js is that it provides a growing community of experienced developers and contributors, making it easy to adopt and find solutions to any issues you might face. This community also means you can leverage the automatic feature that helps you optimize images, and has automatic lazy-loading, preloading of critical images, correct sizing across devices, and supports modern formats.
Here are some of the key benefits of using Express with Next.js:
- Easily build web socket features in your applications
- Develop custom or extra logic for your Next.js routes
- Build Express middleware and fetch data for Next.js pages before they are rendered
- Easily build backend features, such as file uploading, media file compression, and more
Incorporating Express into your Next.js application brings a host of advantages that can significantly improve your development experience and the final product.
Using Next.js with Express
Using Next.js with Express, you can create a custom server that integrates Express to handle complex routing, middleware, and server-side functionalities. This is especially useful for migrating existing Express applications to Next.js.
Express can be used alongside Next.js to manage parts of your application that haven't been migrated yet. This incremental adoption approach helps you transition smoothly to Next.js.
For applications requiring complex server-side logic or specific Express-only features, using a custom Express server with Next.js makes sense. This allows you to leverage the strengths of both frameworks.
Some advantages of using Express with Next.js include a growing community of experienced developers and contributors, easy adoption, and easy-to-find solutions to any issues you might face.
Express with Next.js also provides automatic image optimization, lazy-loading, and preloading of critical images. This improves performance and user experience.
You can use Express with Next.js to achieve clean application routing, as seen in the example where we use Express routing to define routes and pass the page that should be loaded and the id as a query param to the main Next app.
Here are some key differences between using Express with Next.js and using Next.js alone:
By using Express with Next.js, you can create dynamic routes using Express route parameters, implement custom route handling, and easily manage redirects and error handling with Express middleware.
Key Takeaways
If you're considering using Next.js with Express, here are some key takeaways to keep in mind.
Express and Next.js are a dynamic duo for server-side rendering, allowing you to build efficient web applications.
By combining these technologies, you get better performance, routing flexibility, and security.
Don't just be a developer; be a Next.js-ninja with Express!
CodeWalnut: Your Companion
CodeWalnut is your perfect companion for using Express with Next.js. It offers a comprehensive set of tutorials, guides, and resources specifically tailored to help you harness the power of Express with Next.js.
Express.js simplifies server-side development, while Next.js streamlines frontend development. This makes CodeWalnut's guidance invaluable for building web applications that are both functional and user-friendly.
CodeWalnut's curated content will walk you through the process of setting up a robust server-client architecture. This enables you to create efficient and performant web applications effortlessly.
With CodeWalnut, you'll be able to leverage the best of both Express.js and Next.js. Say goodbye to integration woes and hello to a seamless development experience.
Frequently Asked Questions
Does NextJS use Express under the hood?
Yes, NextJS uses Express under the hood, allowing for server-side rendering and other server capabilities. This integration enables NextJS to provide a robust server environment for your applications.
Can I use an Express session in NextJS?
Yes, you can use Express sessions in NextJS, but it requires serving your NextJS app through an Express server and using the Express middleware to handle sessions.
Is NextJS a replacement for Express?
NextJS is not a direct replacement for Express, as it's specifically designed for React-based web applications with server-side rendering. If you need a general-purpose server framework, Express is still a better choice.
Is next JS a replacement for Express?
No, Next.js is not a direct replacement for Express.js, as it's specifically designed for React-based web applications with server-side rendering. While it shares some similarities, Next.js serves a different purpose than Express.js.
Sources
- https://www.rlogical.com/blog/nextjs-vs-expressjs-performance/
- https://blog.stackademic.com/backend-in-next-js-with-without-express-f4daf012caf6
- https://blog.logrocket.com/build-server-rendered-react-app-next-express/
- https://levelup.gitconnected.com/set-up-next-js-with-a-custom-express-server-typescript-9096d819da1c
- https://www.codewalnut.com/learn/using-express-with-next-js
Featured Images: pexels.com