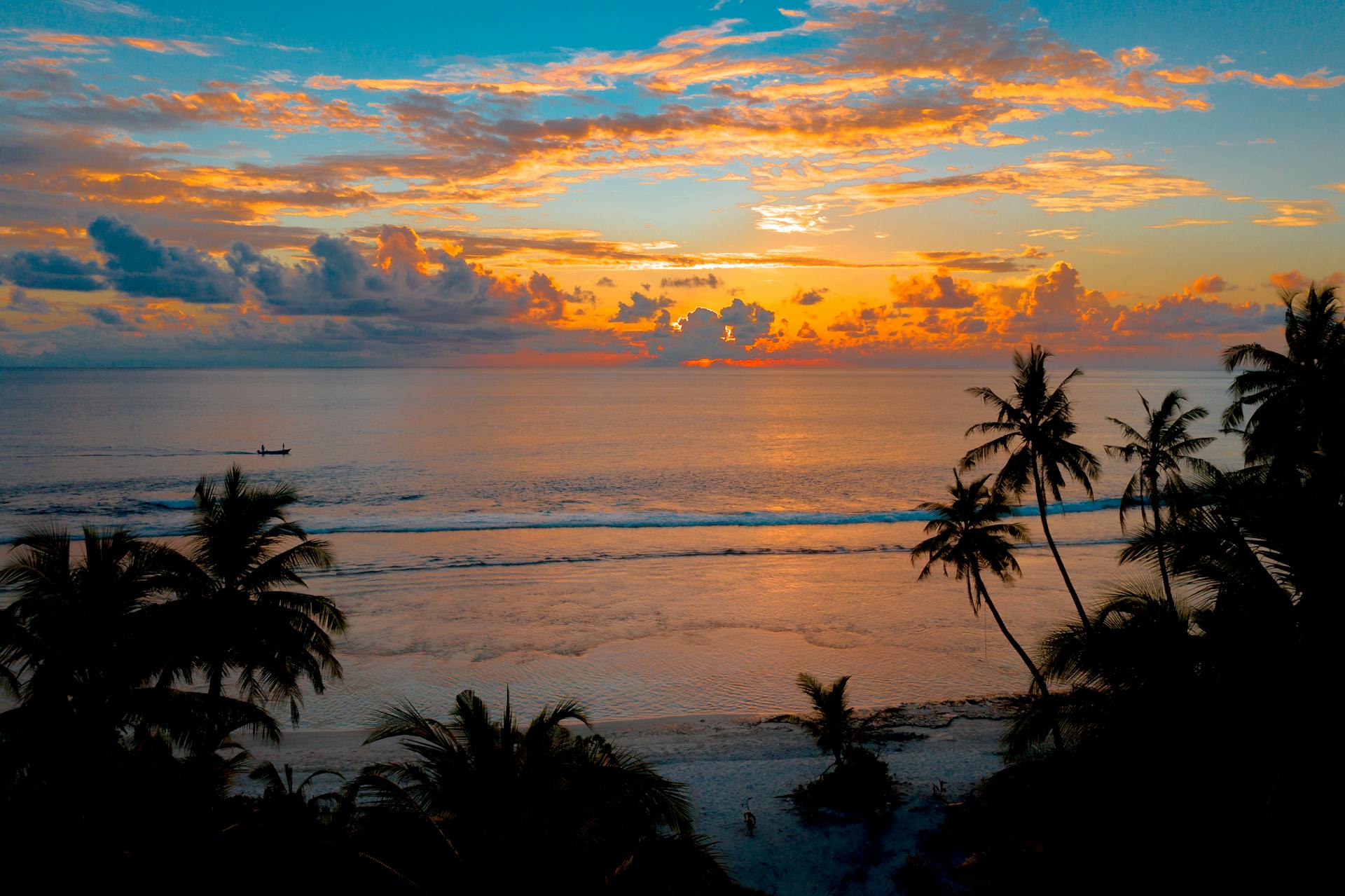
Implementing Next.js dark mode can significantly enhance the user experience, improving readability and accessibility. This is especially true for applications that require extended usage periods, such as productivity tools or social media platforms.
By following best practices, developers can ensure a seamless transition between light and dark modes, reducing the cognitive load on users. This can be achieved by carefully considering the color palette and typography.
A well-designed dark mode should be more than just a color scheme change; it should also take into account the accessibility features of the application. For instance, using high contrast colors can help users with visual impairments.
Next.js provides a built-in feature to enable dark mode, allowing developers to easily toggle between modes. This feature can be leveraged to create a more dynamic user experience.
Enabling Dark Mode
Enabling Dark Mode in Next.js is a straightforward process, but it requires a different approach than what we're used to in static sites. To get started, we need to enable dark mode in Tailwind CSS by adding `darkMode: 'class'` to the Tailwind configuration file.
This will allow us to use the `dark` class on the `html` tag to apply dark mode styles to all children. With this step, we're all set to move forward with implementing dark mode in our Next.js app.
To make the most of this feature, it's essential to understand how Tailwind CSS applies dark mode styles. Whenever we use the `dark` class, Tailwind magically applies styles prefixed with `dark:` to override default styles.
Here's a quick rundown of the key classes we'll use for dark mode:
- `dark`: Applies dark mode styles to all children
- `dark: prefix`: Used to override default styles
By using these classes, we can create a seamless dark mode experience for our users.
Implementing Dark Mode
Implementing Dark Mode is a breeze with Next.js and Tailwind CSS. You can use the dark variant in Tailwind CSS by prepending dark: to properties, such as dark:bg-gray-900 to change the background color to gray-900 when the theme is set to dark.
To toggle between light and dark modes, you need to set the darkMode strategy to class in the tailwind.config.js file. This will apply the theme when the dark class is present in the HTML tree.
Next.js uses the system preferences to set the theme by default, but you can override this behavior by adding the dark class to the html tag or any other element.
Here's a quick rundown of the steps to enable dark mode in Tailwind CSS:
- Add `darkMode: 'class'` to the Tailwind configuration file
- Use the `dark` class on the html tag or any other element to apply the dark mode styles
- Use the `dark:` prefix on properties to override default styles
With these simple steps, you'll be able to implement dark mode in your Next.js application using Tailwind CSS.
Testing and Accessibility
Testing your website with next.js dark mode is a straightforward process. Open your developer tool in your browser, click on the [Application] tab, and choose [Local Storage] from the left menu bar.
For the first visitor, the theme value is stored in localStorage as "theme:system". You can verify this by checking the storage and switching the appearance mode.
To ensure your application is accessible in both light and dark mode, use a tool like the Accessibility Insights browser extension to test the contrast between text and background colors.
Broaden your view: Tutorial Building Useful Nextjs Tool
Testing
Testing is an essential part of ensuring your website is accessible and functional for all users.
Open your developer tool in your browser to inspect the code.
The next-themes code stores the active theme value in localStorage for the first visitor.
For example, the theme:system value is stored in the Local Storage for the first visitor.
Switching the appearance mode in System Settings should reflect the theme change.
Try clicking on the toggle button to update and store the theme value in the storage.
Your website now supports the system theme and can switch between Light and Dark mode.
Expand your knowledge: Next Js Free Code Camp
Accessibility Considerations
Accessibility is crucial for a smooth user experience.
Ensure your application is accessible in both light and dark mode.
Choosing text and background colors with sufficient contrast is key.
You can test the accessibility of your application using a tool like the Accessibility Insights browser extension.
Global Styles and Layout
You can add global styles to a file like global.css to set the tone for your entire application. We can set the background of the body to white in light mode and dark gray in dark mode.
Global styles are a great way to define the look and feel of your application, and can be applied to all components.
To add some global styles, you can use a file like global.css, where you can set the background of the body to white in light mode and dark gray in dark mode.
Dark Mode Implementation
Implementing dark mode in Next.js is a breeze with Tailwind CSS. You can simply prepend `dark:` to properties to indicate they're for dark mode, like `dark:bg-gray-900` to change the background color to gray-900.
To toggle between light and dark modes, you can set the `darkMode` strategy to `class` in the `tailwind.config.js` file. This allows you to use the `dark` class on the HTML tag or any other element to apply dark mode styles to all children.
Here are the steps to enable dark mode in Tailwind CSS:
- Add `darkMode: 'class'` to the Tailwind configuration file
- Use the `dark` class on the HTML tag or any other element to apply dark mode styles
With these simple steps, you're all set to implement dark mode in your Next.js app.
Wrap Up
You've now successfully implemented a functioning dark mode toggle button on your website or app. This is a cool implementation that avoids a common pitfall of dark mode: the annoying flashy-ness.
Tailwind makes it super easy to tailor your design for dark mode. This is a big help, especially when dealing with light and dark mode color scale mapping.
It can be tough to create a neat 1:1 color scale mapping, as you'll often need special treatment to preserve the interface's depth and hierarchy.
Dark Mode
Dark mode is a feature that allows users to switch between light and dark color schemes on a website or app. You can implement dark mode using Tailwind CSS, which makes it easy to create a dark variant of your theme.
To enable dark mode in Tailwind CSS, you need to add `darkMode: 'class'` to your Tailwind configuration file. This will allow you to use the `dark` class on the HTML tag to apply dark mode styles.
You can also use the `dark:bg-gray-900` property to change the background color to gray-900 when the theme is set to dark. This is an example of how you can use the `dark` variant in Tailwind CSS.
To toggle between light and dark modes, you need to create a button that lets users switch between the two. This can be done using a checkbox input, which is the most accessible and straightforward solution.
Here are some key points to consider when implementing dark mode:
The next step is to handle the JavaScript side of things. You can either create a separate JavaScript file or add the code directly to your HTML just before the closing body tag. This will allow you to add event listeners and save the user's preference in localStorage.
To add the dark class to the HTML element based on the current theme, you need to create a new file named `theme-provider.tsx` and add the following code. This will ensure that the dark class is added or removed from the HTML element based on the value of the checkbox input.
You can also use the `next-themes` package to handle dark mode in Next.js. This package allows you to handle dark mode very easily without worrying about blocking page rendering to check user preferences.
Sources
- https://daniloleal.co/my-world/thinking/darkmode-next-tailwind
- https://webtech-note.com/posts/how-to-implement-light-dark-mode-css-vars-next-js
- https://dev.to/chinmaymhatre/implementing-dark-mode-in-nextjs-with-tailwind-css-and-next-themes-a4e
- https://tailwindcss.com/docs/dark-mode
- https://cruip.com/implementing-tailwind-css-dark-mode-toggle-with-no-flicker/
Featured Images: pexels.com