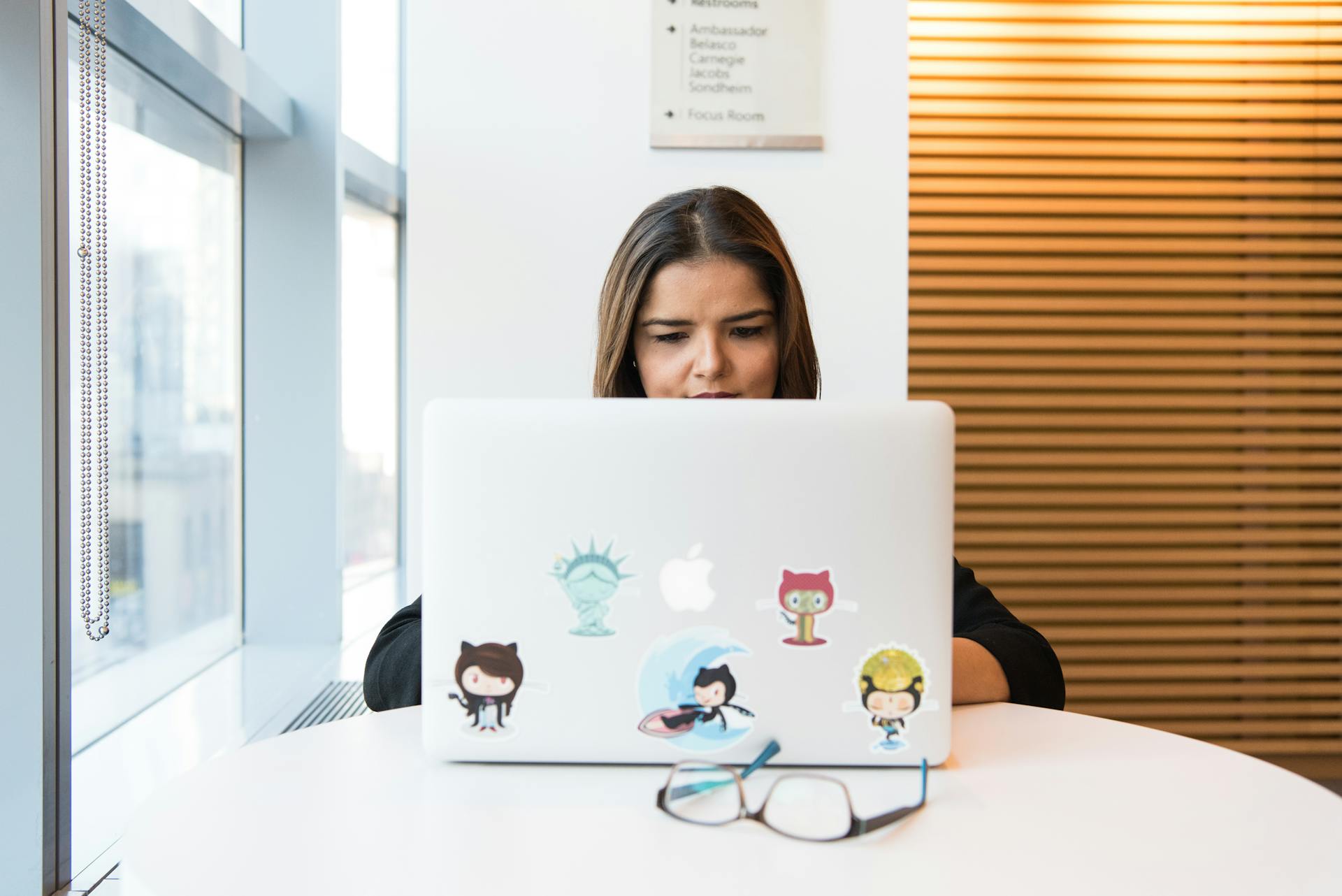
Let's get started with building a useful Next.js tool. You can create a Next.js project using the `npx create-next-app` command, which is covered in more detail in the "Setting Up a Next.js Project" section.
To get started, you'll need to have Node.js installed on your computer. Next.js projects are built on top of React, so you'll also need to have a basic understanding of React fundamentals.
The "Understanding Next.js Pages" section explains how Next.js handles pages in a project. This is an important concept to grasp, as it will help you build a robust and scalable tool.
By following these steps and learning from the examples in the article, you'll be well on your way to building a useful Next.js tool that meets the needs of your users.
Recommended read: What Is Seo Link Building
Getting Started
To get started with this tutorial, I highly recommend using the provided starter boilerplate that I specifically created for this tutorial. It saves your valuable time by already including the necessary dependencies and folder structure, eliminating the need to set up your project from scratch.
Simply clone the starter boilerplate from the GitHub repository and then follow along with the tutorial. This way, you can focus on learning and implementing the concepts without getting caught up in setup details.
Once you have set up the starter boilerplate and successfully run it on your local machine, you should be able to see the initial page. This page marks the beginning of our tutorial and will serve as the starting point for our journey.
You might enjoy: Next Js Starters
Shared Layout
In Next.js, a shared layout is a convenient way to reuse elements across multiple pages, such as a navigation bar or footer, without manually adding them to each page.
The Root Layout is the top-most layout that serves as the consistent structure for the entire app and is required to include the necessary HTML and body tags.
You can define individual route segments within your application, each with its own layout shared across all pages within that segment.
To create a shared layout, open up app/layout.js and add the Root Layout component, which includes metadata tags like title and description for SEO purposes.
Shared Layout
Creating a shared layout in Next.js is a game-changer for your application. It allows you to reuse elements across multiple pages, making it easier to maintain consistency throughout your app.
You can create a shared layout by defining a Root Layout, which serves as the top-most layout and provides a consistent structure for your entire app. This layout is required and must include the necessary HTML and body tags.
The Root Layout is a crucial component that plays a key role in creating a shared layout for your entire application. It's the first type of layout you should consider when setting up your Next.js app.
You can define metadata tags for your application within the Root Layout component, including the title and description properties, which are important for search engine optimization (SEO). These metadata tags can be overridden for specific routes if needed.
The Navigation component is a great example of an element that can be shared across multiple pages. By including it in your Root Layout, you ensure that it's displayed consistently throughout your app.
The children prop is a special prop that represents the content rendered within the shared layout. This allows you to nest other components and content within the shared layout, making it easy to reuse elements across multiple pages.
Expand your knowledge: Can Nextjs Be Used on Traditional Web Application
Displaying Characters on the Homepage
To display characters on the homepage, you'll need to create a UI component that fetches data from the API endpoint. This component is responsible for rendering the homepage UI.
The API endpoint is created in the app/api/characters/route.js file, where an asynchronous function called GET() is defined to handle HTTP GET requests. This function returns a JSON response containing the character data from the characters.json file.
To fetch the character data, the Page component uses the "fetch" function to make an asynchronous HTTP request to the API endpoint. The response from this request is stored in the data variable.
The Page component awaits the result of calling the getAllCharacters function, which fetches the character data. If the HTTP response returned an error, the component throws an error indicating that the data fetch has failed.
The data is then used to dynamically render a grid layout of character avatars with clickable links to individual character pages. Each character's avatar image is displayed using an Image component within the Link component.
The Link component generates a URL based on the character's slug property, allowing users to navigate to individual character pages. This is achieved by mapping over the characters array in the data object and generating a list of items.
You might like: Nextjs Link
Custom Navigation Bar
Creating a custom navigation bar in Next.js is a straightforward process. You'll need to open up the components/Navigation.jsx file and add the code that includes a logo and a link to the quiz section.
This shared layout ensures consistency across all pages, making it easier to manage elements like the navigation bar throughout your application. The navigation bar will be a crucial part of your application's UI.
You can create a folder inside the app directory in Next.js, and it will automatically become a route. However, you have the flexibility to define whether it should be a UI route or an API route.
Related reading: Nextjs Ui
Custom Navigation Bar
Creating a custom navigation bar in Next.js is a straightforward process. You'll start by opening up the components/Navigation.jsx file and adding the necessary code.
The navigation bar will include a logo and a link that takes users to the quiz section. You'll be able to manage elements like the navigation bar consistently across all pages thanks to the shared layout.
Congratulations are in order - you've successfully created a shared layout with a navigation bar for your Next.js app. This shared layout is essential for maintaining consistency throughout your application.
To display characters on the homepage, you'll need to create an API route that retrieves all the characters from your local JSON file. This will allow you to dynamically populate the homepage with relevant information.
Routing in Next.js is a fundamental concept that determines how different parts of your application are accessed. You can define whether a folder inside the app directory should be a UI route or an API route.
Take a look at this: Next Js Use Context
What Is an App Router?
The App Router in Next.js is a new paradigm for developing applications that leverages the latest features of React.
It introduces a natural evolution of the existing Pages Router, which is based on the file system.
The App Router enables you to run React code on the server by default, fetching data on the server and only returning static HTML to the client.
For your interest: Use Theme in Server Components Nextjs
This means we have a Server Component that retrieves data from the server and renders its content on the server side.
You won't have access to client-side features like React state and React Hooks inside Server Components, since they're only running on the server.
To use client-side features, you need to specify that in your component file by adding "use client" at the top of the file.
On a similar theme: Next Js Use Client
Routing
Routing is a critical aspect of building a Next.js application, and it's essential to understand how to navigate through different routes. In Next.js, you can create dynamic API routes by using brackets to name your folders, indicating to Next.js that it is a dynamic route. This allows you to access the dynamic value within your code and retrieve the desired data.
To catch all routes in Next.js, you can use the catch-all route feature, which is denoted by a single asterisk (*). This is useful for handling routes that don't have a specific name. For example, you can create a catch-all route at the root of your API directory to handle requests to any API endpoint.
If this caught your attention, see: Nextjs save Data Pulled from Api Using State Context
Here's a list of ways to handle routes in Next.js:
- Catch all routes using a single asterisk (*)
- Optional catch all routes using a question mark (?)
- Nested routes by creating multiple levels of folders
- Dynamic API routes using brackets to name your folders
- Static API routes using a fixed file name
In addition to these routing methods, Next.js also provides a function called `generateStaticParams` that allows you to statically generate routes at build time. This is useful for optimizing performance and reducing the load on your server. By using `generateStaticParams`, you can pre-render your routes and make them available for static site generation.
Server-Side Rendering
Server-side rendering is a powerful feature in Next.js that allows you to generate HTML on the server. This can be done using three different methods: Static Site Generation (SSG), Server-Side Rendering (SSR), and Incremental Static Regeneration (ISR).
SSG is a great option for websites with content that doesn't frequently change. It generates HTML at build time, fetching data from APIs or other data sources and pre-rendering the pages. This means that pre-rendered pages can be served to the client upon request.
SSR is useful for websites with frequently updated content or personalized user experiences. It generates HTML on each request, fetching data and rendering the HTML on the server before sending it to the client.
Intriguing read: Nextjs Rendering
ISR is a feature in Next.js that allows you to statically generate pages on-demand, rather than at build time. This means that your site can be both statically generated and dynamic at the same time.
Here are the three server-side rendering methods in Next.js, summarized in a table:
By understanding these server-side rendering methods, you can choose the best approach for your Next.js project.
Enhancing Codebase
Isolating commonly used functions like getAllCharacters in a separate module can enhance code reusability and avoid code repetition.
By moving the function to a separate module, you can easily import and use it in different parts of your codebase. This approach makes it convenient to access and reuse functions throughout your codebase.
You can make a quick adjustment in your project by cutting out the getAllCharacters function from the app/page.jsx file and exporting it from the lib/characters.js file. Now you can import and use the function in different parts of your codebase.
On a similar theme: Next Js Free Code Camp
Enhancing Codebase Modularity and Maintainability
Modularizing your codebase is a game-changer for maintainability. By isolating commonly used functions, you can avoid code repetition and enhance code reusability.
A great place to start is by identifying functions that are used in multiple parts of your codebase. For example, the getAllCharacters function in a Next.js project can be isolated and reused throughout the codebase.
Cutting out the getAllCharacters function from the app/page.jsx file and moving it to a separate module is a quick adjustment that can make a big impact. This way, you can easily import and use the function in different parts of your codebase.
Now you can access the getAllCharacters function throughout your whole codebase by importing it from the lib/characters.js file. This makes it easy to reuse the function and avoid duplicating code.
Testing the endpoint is a great way to see the result of your modularization efforts. Simply open up http://localhost:3000/api/characters/peter-griffin in your browser and you should see the JSON data.
Additional reading: What Is Azure Used for
Prisma Site Generation
Next.js blurs the lines between client and server, making it the perfect fit for Prisma. It supports pre-rendering pages at build time (SSG) or request time (SSR), allowing you to decide when to access your database with Prisma.
You can use Prisma inside of getStaticProps to send queries to your database, enabling static rendering of your page with dynamic data. Next.js will pass the props to your React components.
Next.js and Prisma is the ultimate combo if you need a database in React apps! You can query your database with Prisma in Next.js API routes, in getServerSideProps or in getStaticProps for full rendering flexibility and top performance.
Readers also liked: Next Js Db
Featured Images: pexels.com