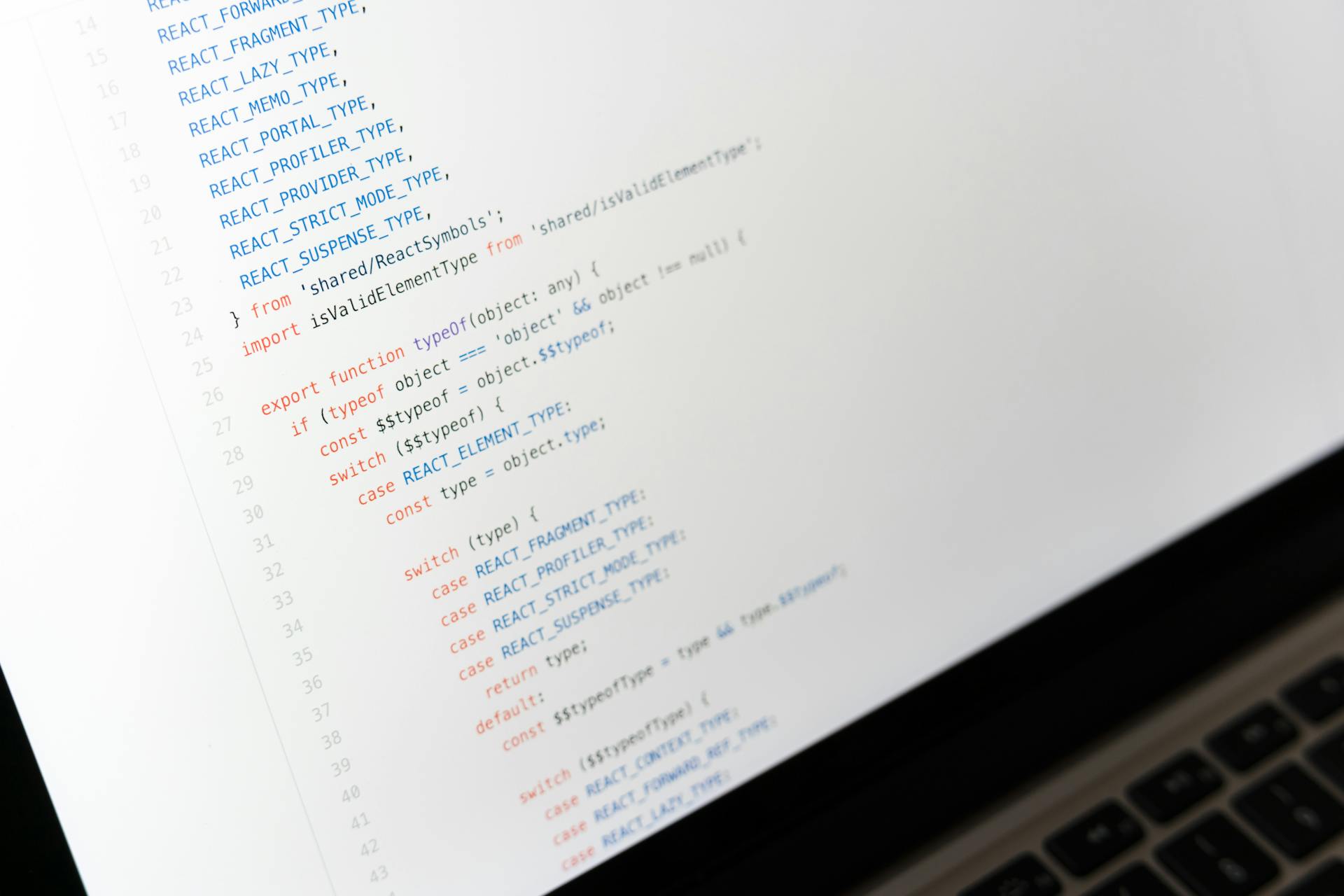
Creating a Nextjs starter project from scratch requires setting up a new project with npm or yarn, and then running the command npx create-next-app my-app to get started.
You can then navigate to the project directory and install the dependencies with npm install or yarn install.
To start the development server, run npm run dev or yarn dev, and access your app at http://localhost:3000.
Building a production-ready Nextjs app involves running npm run build or yarn build to create a static HTML file in the out directory.
If this caught your attention, see: Install Next Js 13
Getting Started
To start a Next.js project, you can use create-next-app, a CLI tool that sets up a new project with everything configured according to best practices. This tool handles complex configurations for you, so you can start coding your app right away.
The default Next.js template sets up a production-ready app with React, ESLint, TypeScript, a basic App Router, and development and production builds. You can also kickstart your project using one of the official Next.js examples, which provide pre-built templates for common use cases like blog sites and e-commerce stores.
Here are some key advantages of using create-next-app:
- Sets up Webpack, Babel, ESLint automatically
- Creates optimal production and development builds for you
- Easy customization for plugins, styling tools like Sass
- Gets you from zero to deploying an app quickly
Initializing the Project
You can start a Next.js project using create-next-app, a CLI tool that sets up everything for you. This tool is the fastest way to initialize a Next.js project with everything configured according to best practices.
Create-next-app handles complex configurations like Webpack, Babel, and ESLint automatically, so you can focus on coding your app. It also creates optimal production and development builds for you.
To get started, run create-next-app in your terminal, and it will install dependencies and set up the project. You'll have a production-ready Next.js app configured with React, ESLint, TypeScript, and a basic App Router for navigation.
Some key advantages of using create-next-app include setting up Webpack, Babel, ESLint automatically, creating optimal production and development builds, and easy customization for plugins and styling tools like Sass.
Here's a step-by-step guide to initializing a Next.js project:
- Run create-next-app in your terminal to install dependencies and set up the project.
- Install dependencies using npm install or yarn.
- Start the local development environment using npm run dev.
- Modify code in the pages/ directory to build application views.
- Add additional libraries and features as needed.
With the starter initialized locally, you can begin developing your app on top of the provided foundation. Be sure to consult the starter documentation if you get stuck, and many starters also provide demos showcasing implemented features.
Check this out: Production Ready Starter Next Js
Key Takeaways
Using a Next.js starter kit can simplify project configuration and eliminate decision fatigue early on. This is especially true when you're just starting out with a project.
By leveraging a Next.js starter kit, you can accelerate development and get to the good stuff faster. I've seen it myself, and it's amazing how much time you can save.
However, be careful not to add unnecessary libraries too soon, as this can lead to "starter bloat". This can make your project harder to maintain and update in the long run.
Deploying to production is a whole different ball game, but services like Vercel can make it a breeze. They handle infrastructure management for you, so you can focus on what matters most – creating value for your users.
Adopting SRE principles from the start can also help instill reliability best practices in your project. This is crucial for maintaining application stability post-launch.
Curious to learn more? Check out: Next.js
Understanding Next.js
Next.js is a versatile React framework that quickly became popular due to its extensive feature set. It renders React components server-side, providing increased performance and search engine optimization (SEO).
For your interest: React Next Js
Automatic code splitting is one of Next.js' key features, which improves page load speed by splitting code into separate bundles loaded on demand.
Next.js also supports client-side routing, which provides dynamic routing and page transitions without full page reloads. This streamlines the development process and makes it easier to build complex apps.
Here are some of the key features of Next.js:
- Automatic code splitting - Improves page load speed
- Client-side routing - Provides dynamic routing and page transitions
- API routes - Build backends and API endpoints directly within Next.js
- Static site generation - Pre-renders pages at build time for blazing fast performance
Next.js comes with built-in support for TypeScript and ESLint, which makes it easier to develop and maintain complex apps.
Discovering React Framework
So you're looking to discover Next.js, a versatile React framework that's gained immense popularity due to its extensive feature set. Next.js renders React components server-side, providing increased performance, search engine optimization (SEO), and more.
Automatic code splitting is one of the features that improves page load speed by splitting code into separate bundles loaded on demand. This is especially useful for complex apps.
Client-side routing provides dynamic routing and page transitions without full page reloads. This feature is a game-changer for developers who want to create seamless user experiences.
A fresh viewpoint: Next Js React Fundamentals
Next.js comes with built-in support for TypeScript, ESLint, and other tools that streamline development. This means you can focus on building features without worrying about setting up a bunch of extra tools.
API routes allow you to build backends and API endpoints directly within Next.js. This feature is perfect for developers who want to create robust and scalable applications.
Static site generation pre-renders pages at build time for blazing fast performance. This feature is ideal for developers who want to create fast and secure websites.
Here are some of the key features of Next.js:
- Automatic code splitting
- Client-side routing
- Developer experience
- API routes
- Static site generation
Ssr Vs. Ssg
Next.js offers two main approaches to generating static sites: Static Site Generation (SSG) and Server-Side Rendering (SSR).
Static Site Generation allows Next.js to generate HTML for each page at build time, which can then be cached by a CDN for a super performant site.
This approach is suitable for sites that don't need to update frequently, like a blog.
However, if your site needs to update dynamically, Server-Side Rendering is the way to go.
To use SSR instead of SSG, you would replace getStaticProps and getStaticPaths with getServerSideProps.
Keep in mind that this change requires an API to work, which we didn't create in our example.
If this caught your attention, see: Static Nextjs Site
Project Customization
Don't change the defaults of your Next.js starter kit just for the sake of it - understand why the defaults exist first.
To customize your starter kit effectively, question defaults and isolate overrides to reusable modules. This helps maintain a clean and organized codebase.
Here are some key principles to keep in mind:
- Document changes - Adding comments explaining made changes helps future maintainers.
- Think long-term - Consider future developers working on the codebase down the line.
By following these principles, you can prevent customizations from eroding the benefits of your starter kit over time.
Customizing and Extending
Customizing and extending a project can be a delicate process, and it's essential to understand the underlying goals of the starter kit before making any changes.
Don't change defaults just for the sake of it - understand why they exist first.
Customizing your project requires careful consideration to prevent eroding the benefits of the starter kit over time. Stick to the principle of isolating overrides to limit customization to isolated, reusable modules.
Avoid scattering one-off changes throughout your codebase. Documenting changes by adding comments explaining made changes helps future maintainers.
Consider future developers working on the codebase down the line and think long-term.
Nextless.js
Nextless.js is a Next.js SaaS starter template that helps developers quickly build scalable and production-ready SaaS products.
It includes a wide range of features such as authentication, payment, teams, dashboards, landing pages, and email functionality.
Nextless.js uses Next.js for static site generation, enabling developers to create fast and efficient websites optimized for performance.
TypeScript is used for type checking, catching errors early and improving code quality.
Tailwind CSS, a popular utility-first CSS framework, makes it easy to create consistent and customizable styles throughout the application.
Nextless.js also includes React Strict Mode, which can help identify and address potential problems with your app.
A linter with ESLint is used to maintain code quality and consistency.
End-to-end (E2E) testing with Cypress is incorporated, helping to ensure the application is working correctly and catching bugs early on.
A different take: Apply Css Nextjs
Deployment and Routing
You can deploy your Next.js app to production using platforms like Vercel or Netlify, which provide quick deployment integration.
To deploy with Vercel, you need to connect to your GitHub repo, trigger deployments on push automatically, serve your app over a global CDN network, and preview changes with unique URLs.
Take a look at this: Nextjs by Vercel Meaning
With Vercel, you can also rollback anytime and add custom domains and environments.
If you're using another platform, you'll need to create production build files with next build, start the Next.js production server, configure a reverse proxy, set up SSL certificates, and add custom domain and environments.
Next.js apps can be deployed to AWS Amplify with support for both SSR and SSG apps.
To deploy to AWS Amplify, change your build script to next build && next export for statically generated apps, or stick with the scripts generated by Next.js for server-side rendered apps.
You can create routes in Next.js by creating files within the pages folder, which makes those files into routes.
For example, creating a file called about.js will create a /about page, and creating a file called [color].js will dynamically create a page for each color in your array.
Here are the steps to create routes with Next.js:
- Create a file within the pages folder
- Make that file into a route
- Use folders to create routes like /blog/my-post-title
- Put the file name in [] to make it a parameter name
Components and Features
The Next.js Starter kit is built on top of a solid foundation, with several key components and features that make it a great starting point for your project.
It includes Next.js, a popular React-based framework for building server-rendered, statically generated, and performance-optimized websites and applications. Next.js provides features like automatic code splitting, server-side rendering, and static site generation.
One of the standout features of the Next.js Starter kit is its use of TypeScript, a statically typed language that helps catch errors early and improves code maintainability. This ensures that your project is built with a robust and scalable architecture from the start.
The kit also includes a pre-configured ESLint and Prettier setup, which helps maintain a consistent code style and catches errors in your code. This ensures that your code is not only functional but also readable and maintainable.
Expand your knowledge: Nextjs Code Block
GetStaticProps
GetStaticProps is a function that provides props to the React component for the page. It's what makes it possible to fetch data for a specific page without having to hardcode it.
In Next.js, this function gets the params passed to it, which in our case is the color name. We can then find the color in our array that matches the color in the params.
The data we return in getStaticProps is nested within { props }. This is a requirement for Next.js, and it's what makes it possible to render the page with the correct data.
You might like: Nextjs Params
Link Component
The Link component is a powerful tool in Next.js that allows for client-side route transitions, making page transitions smoother for users. It's used in place of an anchor tag, but with additional functionality.
To use the Link component, you'll need to update your index.js file with the list of colors. This is where the Link component really shines, making it easy to link to each color's page from the home page.
The Link component is a Next.js specific component, which means it's designed to work seamlessly with the Next.js framework. This makes it a great choice for building fast and efficient applications.
By using the Link component, you can create a seamless user experience that's both fast and intuitive. This is especially important for applications where page transitions are frequent, such as e-commerce sites or social media platforms.
Intriguing read: Link Nextjs
Sources
- https://nextjsstarter.com/blog/next-js-starter-project-guide-from-init-to-deployment/
- https://blog.logrocket.com/best-next-js-starter-templates/
- https://strapi.io/blog/introducing-the-new-strapi-starter-with-nextjs13-tailwind-and-typescript
- https://hygraph.com/docs/app-framework/quick-starters/nextjs
- https://welearncode.com/beginners-guide-nextjs/
Featured Images: pexels.com