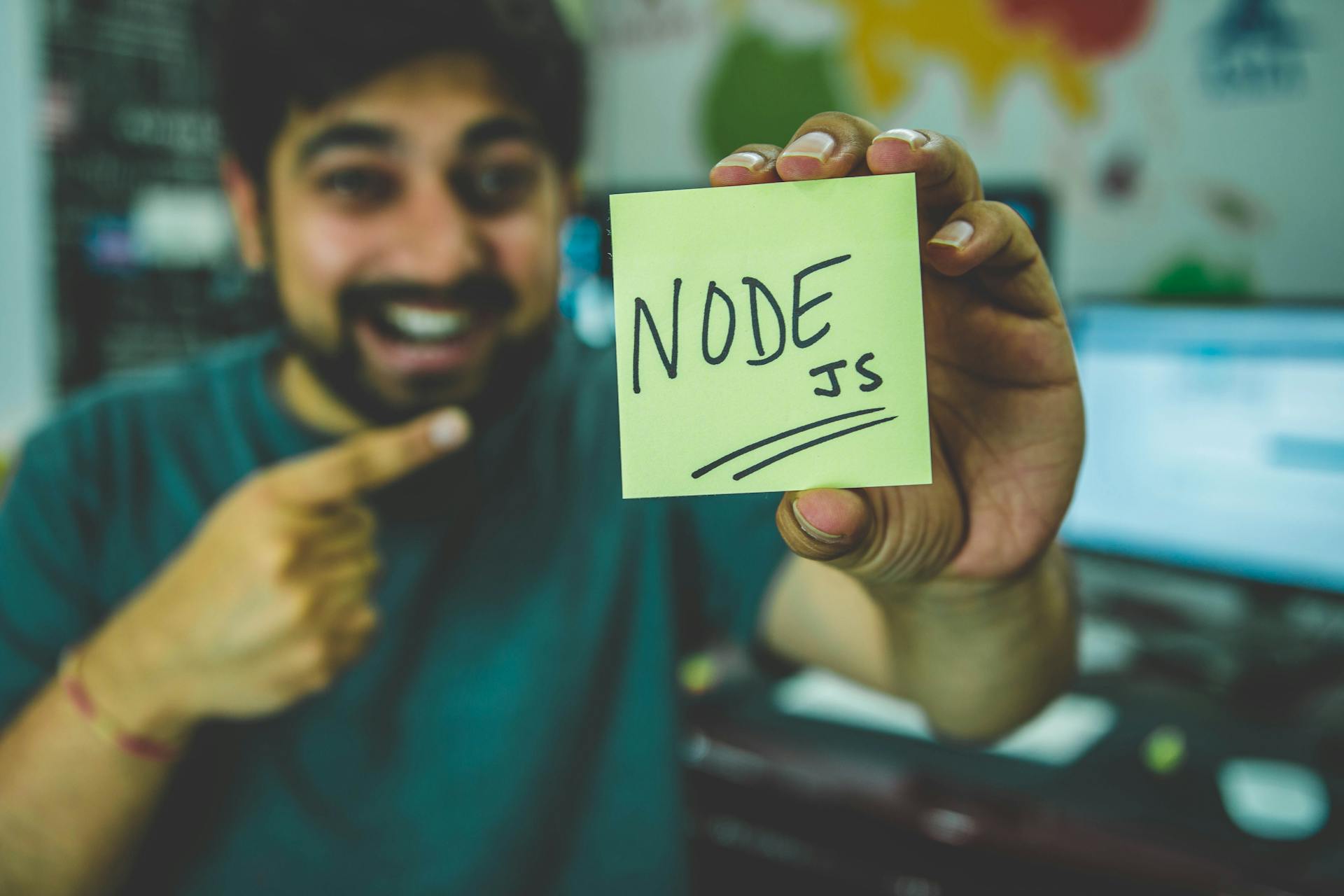
The useContext hook in Next.js allows you to share state across pages in a React application. This is especially useful for managing user preferences or settings that should be consistent across the entire application.
By using the useContext hook, you can create a centralized store for your application's state, making it easy to access and update the state from any page. This approach also helps to avoid prop drilling, which can make your code more maintainable and efficient.
In a Next.js application, you can use the useContext hook in combination with the useReducer hook to manage state changes. This combination provides a robust and scalable way to handle state updates.
Setting Up React in Next.js
To set up React in Next.js, you'll need to create a new project with the command `npx create-next-app my-app`. This will give you a basic Next.js project structure.
The `pages` directory is where you'll create your React components, which will be rendered as pages in your Next.js app. For example, you can create a new page in the `pages` directory with the command `npx next create page about`.
You might enjoy: Next Js Pages
Next.js uses a file-based routing system, where each file in the `pages` directory corresponds to a route in your app. For instance, if you create a file called `about.js` in the `pages` directory, Next.js will automatically create a route for it at `/about`.
In a Next.js project, you can use React hooks like `useState` and `useEffect` to manage state and side effects in your components. For example, you can use `useState` to store a counter value in a component's state, and then update it with a button click.
To use React Context API in Next.js, you'll need to create a context provider component that wraps your app. This can be done by creating a new file in the `pages` directory, such as `_app.js`, and using the `createContext` function from the `react` library to create a context.
Recommended read: Next Js Usestate
Understanding React Context
React Context is a great feature for sharing global state, making it easily accessible throughout the component tree. This is especially true when dealing with numerous components that need to access the same state.
React Contexts allow us to reach global states without having to worry about passing props multiple levels deep. This makes global state neatly structured.
Contexts are particularly useful when some components only pass props through to their children without using or mutating them. This can be error-prone and tiresome.
To create a new context and provider for our global state, we can use the React Context API. This involves creating a context and provider for our state and handler.
By wrapping all the pages with our provider in our main app component, every page and/or component can now access the exposed state using the useContext hook. This makes it easily accessible, no matter how deep they are in the tree.
The React Context store can be created and placed in a separate file, such as app/context/theme.js. This file can store and manipulate the color value, for example.
However, the introduction of React's Server Components presents a challenge for the Context API. Server components do not inherently manage or understand 'state' as the client does, making it incompatible with the Context API.
Here's an interesting read: Next Js Component
Implementing React Store
React Contexts are a great feature for sharing global state, making it easily accessible to components throughout the application.
Global state can become tiresome to manage when passed multiple levels deep through props, leading to errors and code duplication.
Contexts allow us to reach these global states without worrying about passing props, making it a neat and structured way to manage state.
To implement a React store, you can create a new context and provider for your global state, as shown in Example 1.
A different take: React Next Js
Theory Will Only Take You So Far
Theory will only take you so far when it comes to implementing complex features like theming in a React application. You can't simply rely on theory and expect it to magically solve the problems you'll encounter.
In a traditional setup, a theme provider would be rendered at the root of the application, using hooks like useState to toggle between themes. This approach doesn't work when you're dealing with server components, as you can't import a server-rendered component and nest it within the theme provider.
A unique perspective: Next Js Provider
The solution lies in separating concerns, just like we did with the client component and server component framework. By encapsulating our theme context and state within a client component, we can ensure that the theming logic resides solely on the client side.
The 'use client' declaration in the client component instructs React to treat it as a client-side component, enabling it to use context and stateful logic. This is a crucial step in making our theming system work seamlessly with server-rendered components.
A unique perspective: Nextjs Can Layouts Be Server Components
Adding React Store to an App
To add a React store to an app, you'll need to create a React Context store, which is essentially a centralized store that manages and shares data across your application.
You can place the React Context store in a specific file, such as app/context/theme.js, as demonstrated in the example.
The theme context store is designed to store and manipulate only the color value, making it a simple and focused solution.
By creating a React Context store, you'll be able to share data between components and make your app more manageable and scalable.
To get started, follow the example and create your own React Context store, customizing it to fit your app's specific needs.
Worth a look: Trpc Nextjs App Directory
Sources
- https://tuffstuff9.hashnode.dev/using-context-and-state-in-nextjs-server-components
- https://reactician.com/articles/sharing-state-between-nextjs-page-navigations-using-react-contexts
- https://www.js-craft.io/blog/using-react-context-nextjs-13/
- https://medium.com/geekculture/how-to-use-context-usereducer-and-localstorage-in-next-js-cc7bc925d3f2
- https://upmostly.com/next-js/streamline-your-next-js-apps-with-the-context-api
Featured Images: pexels.com