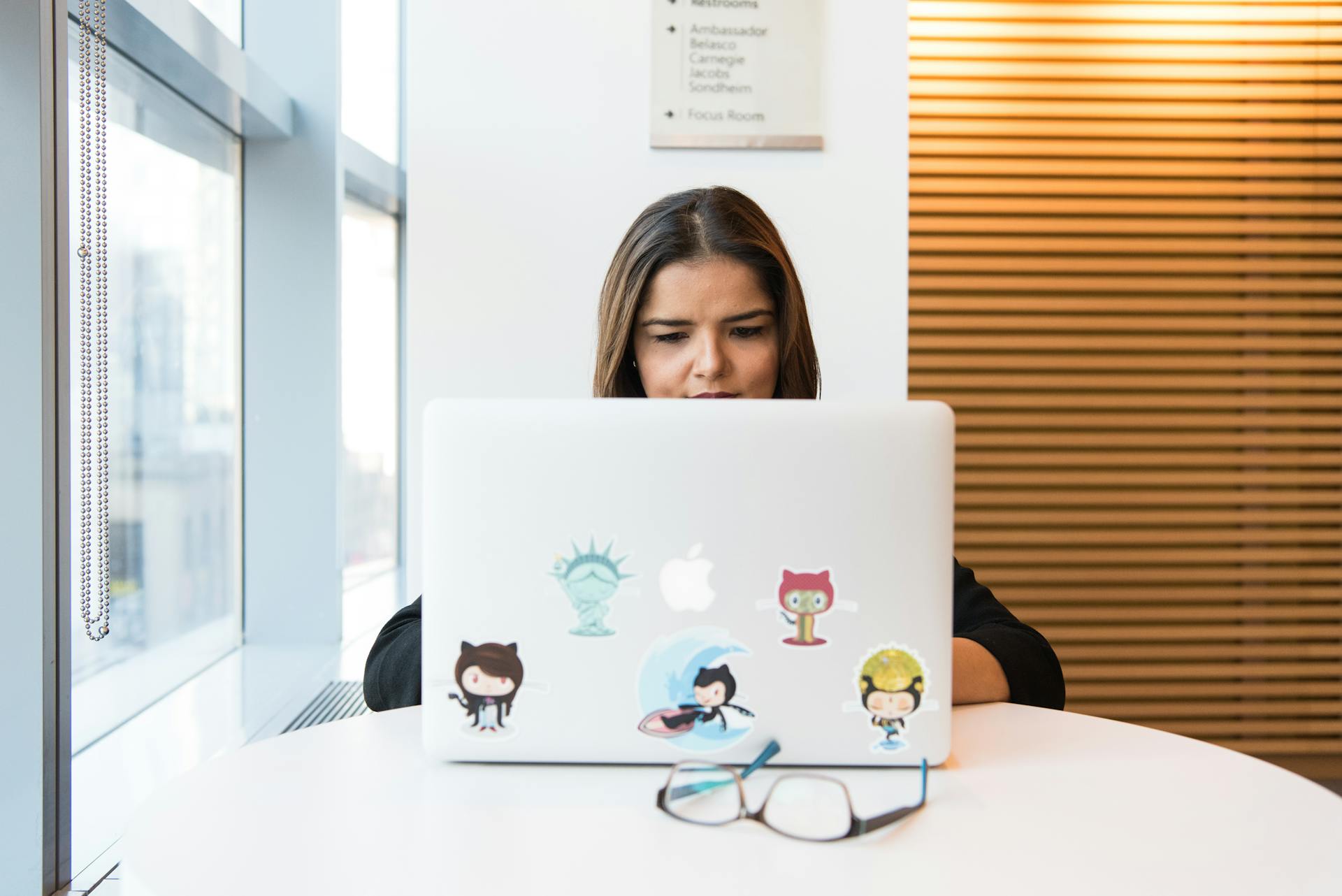
Let's start with a fresh Next.js project and set up Playwright testing from scratch. To begin, create a new Next.js project using the command `npx create-next-app my-next-app --experimental-app`.
Next, initialize a new Node.js project by running `npm init` in the project directory. This will create a `package.json` file that we'll use to manage our project's dependencies.
In the `package.json` file, add a new script called `test` that runs Playwright using the command `playwright test`.
For Playwright to work correctly, we need to install the `@playwright/test` package by running `npm install --save-dev @playwright/test`.
Worth a look: Testing-library/jest-dom How to Test with Next Js
Setting Up Nextjs
Setting up Nextjs involves installing the necessary packages and configuring the playwright.config.ts file. This file is created by default when you run the npm init playwright command, and it's where you'll configure the testDir to ./src/pages.
The testDir is set to src/pages by default, which means all .spec files in this directory will be run as tests. You can check out the repo for this stage of the setup to see the default configuration in action.
In the playwright.config.ts file, you can also change the default reporter from 'html' to 'list' to see the test results in the terminal instead of a browser window.
Suggestion: Azure Playwright
Configuring a Web Server
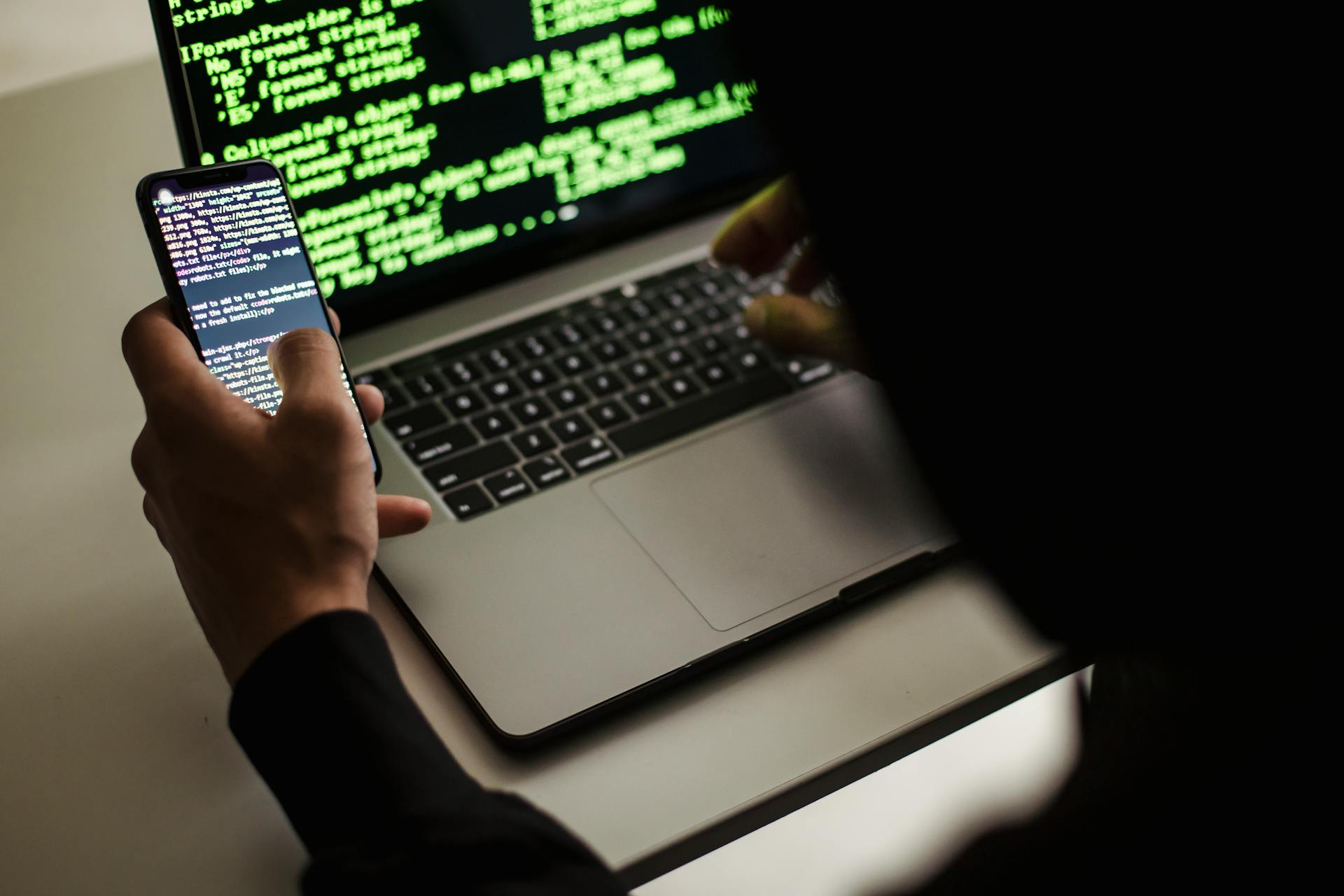
Configuring a web server is a crucial step in setting up Next.js for End-to-End (E2E) testing. You can use the webserver property in your Playwright config to launch a development web server during the tests.
To configure a web server, you'll need to specify the command to start the local dev server of your app, as well as the URL of your http server that is expected to return a 2xx, 3xx, 400, 401, 402, or 403 status code when the server is ready to accept connections.
The webserver property in your Playwright config allows you to launch a development web server during the tests. This is particularly useful when testing Next.js applications.
You can configure the web server by specifying the command to start the local dev server of your app, which is typically `npm run dev`. You can also specify the URL of your http server, which should return a valid status code when the server is ready to accept connections.
Additional reading: Next.js
Here are the properties you can use to configure a web server in your Playwright config:
Step 2 - Setup
Setting up Next.js involves several key steps, and in this section, we'll focus on Step 2 - setting up Playwright.
You can install @playwright/test and create a playwright.config.ts file with the default configuration using a script. This script also sets the testDir to ./src/pages, which means all .spec files in this directory will be run as tests.
The default reporter is 'html', but you might prefer to change it to 'list' in your playwright.config.ts file, as it will open a browser window with the test results, which you might not prefer.
You can configure the projects array in your playwright.config.ts file to run your tests in one browser, by commenting out any additional entries. This will control the devices that will be used in your tests.
To run your tests, you'll need to define the test script in your package.json, which will run the tests in the src/pages folder.
A unique perspective: Run Nextjs on Bluehost
Here are the common configuration options for Playwright:
You can find the full setup in our testing-setup-nextjs-playwright repo, and check it out if you find it useful.
Configuring Playwright for Next.js
To configure Playwright for Next.js, you'll want to make a few adjustments to the configuration. First, enable the automatic launch of your development server when running E2E tests by uncommenting the line and setting the command to `npm run dev`.
The base URL for your tests is crucial for resolving relative paths. Update the `baseURL` property to `http://127.0.0.1:3000`, as shown in the example. This will ensure that your tests run smoothly.
Playwright fixtures are used to configure the testing environment for each test. They extend the test function from `@playwright/test` with additional functionalities, such as opening the correct page before every test. This is achieved by using the location of the test file in the file system to navigate to the corresponding webpage.
You can configure various options in the `testConfig` object, including `forbidOnly`, `fullyParallel`, `projects`, `reporter`, `retries`, `testDir`, `use`, `webServer`, and `workers`. These options allow you to customize the behavior of your tests and improve their performance.
Here's a list of some common configuration options:
The timeout for each test is enforced by Playwright, with a default value of 30 seconds. You can adjust this value by setting the `timeout` property in the `testConfig` object.
Testing Setup
To set up Playwright, you'll need to install @playwright/test and create a playwright.config.ts file with the default configuration. This script will create a playwright.config.ts file with the default configuration, including setting the testDir to ./src/pages.
The testDir is set to ./src/pages, which means all .spec files in this directory will be run as tests. You can check out the repo for this stage of the setup in Step 2 - setting up Playwright.
You might want to change the default reporter from 'html' to 'list' in your playwright.config.ts file, as the 'html' reporter will open a browser window with the test results. This is a good idea because you probably don't want to see the results in a browser window.
Related reading: Html File Upload Multiple Files
You can also comment out any additional entries in the projects array in your playwright.config.ts file, which controls the devices that will be used in your tests. This will ensure that your tests run in one browser.
To run your tests, you'll need to define the test script in your package.json file. This script will run the tests in the src/pages folder, which should have a single file, example.spec.ts, generated by the npm init playwright command.
Here's an interesting read: Nextjs Script
Configuring Tests
Configuring tests is a crucial step in setting up Playwright for Next.js. You want to make sure your tests run efficiently and effectively, without any unnecessary configuration.
To achieve this, you can use Playwright fixtures to configure the testing environment for each test. A fixture is some code that's configuring the testing environment, and it extends the test function from @playwright/test with additional functionalities.
Playwright fixtures can be used to open the correct page for each test, without writing this explicitly in every test. You can navigate to a webpage based on the location of the test file in the file system, assuming it will have the same path as the test file.
Here's an interesting read: Testing Azure
Some common configuration options include:
By configuring your tests correctly, you can ensure that they run smoothly and efficiently, without any unnecessary configuration.
Basic Configuration
Let's dive into the basics of configuration for tests. You can exit with an error if any tests are marked as test.only, which is useful on CI.
This feature is controlled by the testConfig.forbidOnly option. I've found this to be a useful setting when running tests on continuous integration servers, as it helps catch any issues early on.
Here are some common configuration options for tests:
These options can be a bit overwhelming at first, but once you understand what each one does, you'll be able to configure your tests with ease. I recommend taking a closer look at each option to see which ones you need to enable or disable for your specific use case.
Filtering Tests
Filtering Tests is an essential part of writing effective tests. You can filter tests by glob patterns or regular expressions.
The `testConfig.testIgnore` option allows you to specify glob patterns or regular expressions that should be ignored when looking for test files. For example, you can use `*test-assets` to ignore any files with the word "test-assets" in their name.
You can also use the `testConfig.testMatch` option to specify glob patterns or regular expressions that match test files. By default, Playwright runs `.*(test|spec).(js|ts|mjs)` files, but you can customize this to suit your needs.
Here's a breakdown of the `testConfig.testMatch` option:
The `expect` option allows you to configure web first assertions like `expect(locator).toHaveText()`. By default, these assertions have a separate timeout of 5 seconds, but you can adjust this to suit your needs.
Advanced Configuration
In the world of Playwright testing, configuration is key. You can configure advanced options to suit your needs.
The `testConfig.globalSetup` option allows you to specify a path to a global setup file, which will be required and run before all tests. This file must export a single function.
When running tests, it's essential to have a clear output directory. The `testConfig.outputDir` option helps you specify a folder for test artifacts like screenshots, videos, and traces.
Playwright enforces a timeout for each test, defaulting to 30 seconds. You can adjust this with the `testConfig.timeout` option. Time spent by the test function, test fixtures, and `beforeEach` hooks is included in the test timeout.
Here are some key advanced configuration options at a glance:
Naming and Organization
Configuring the naming convention in Playwright is crucial to automatically navigate to the correct page for each test. This is achieved by using Playwright fixtures to configure the testing environment for each test.
A fixture extends the test function from @playwright/test with additional functionalities, mainly opening the correct page before every test. This is based on the location of the test file in the file system, assuming it will have the same path as the test file.
The same port should be used in the Playwright configuration file for consistency. A smaller test timeout can also be set, as the default is 30 seconds.
You can check out the repo for this stage of the setup, which includes configuring the Playwright fixture and naming convention.
Intriguing read: Next Js 14 Redirect to Another Page Loading Indicator
Build
In a Next.js project, the `jest.config.js` file is used to configure Jest, the testing framework used by playwright.
The `jest.config.js` file is located in the project root directory, alongside the `next.config.js` file.
To configure Jest, you can add custom configurations to the `jest.config.js` file.
The `moduleDirectories` option in `jest.config.js` specifies the directories Jest should search for modules.
The `moduleFileExtensions` option in `jest.config.js` specifies the file extensions Jest should consider as modules.
The `setupFilesAfterEnv` option in `jest.config.js` specifies the files Jest should run after the environment has been set up.
The `testEnvironment` option in `jest.config.js` specifies the testing environment used by Jest.
The `testMatch` option in `jest.config.js` specifies the files Jest should match for testing.
The `moduleNameMapper` option in `jest.config.js` specifies the mapping of module names to paths.
The `setupTestFrameworkScriptFile` option in `jest.config.js` specifies the file Jest should run to set up the testing framework.
In a Next.js project, the `playwright.config.js` file is used to configure Playwright, the browser automation framework used for testing.
If this caught your attention, see: Why Testing Software Is Important
The `playwright.config.js` file is located in the project root directory, alongside the `jest.config.js` file.
To configure Playwright, you can add custom configurations to the `playwright.config.js` file.
The `use` option in `playwright.config.js` specifies the browser or browser type to use for testing.
The `width` and `height` options in `playwright.config.js` specify the dimensions of the browser window.
The `slowMo` option in `playwright.config.js` specifies the slow motion factor for testing.
The `timeout` option in `playwright.config.js` specifies the timeout for testing.
The `reporter` option in `jest.config.js` specifies the reporter used by Jest.
The `reporter` option in `playwright.config.js` specifies the reporter used by Playwright.
The `testPathPattern` option in `jest.config.js` specifies the pattern for test file paths.
The `testPathPattern` option in `playwright.config.js` specifies the pattern for test file paths.
The `projects` option in `jest.config.js` specifies the projects to include in the Jest configuration.
The `projects` option in `playwright.config.js` specifies the projects to include in the Playwright configuration.
Additional reading: Can Nextjs Be Used on Traditional Web Application
Sources
- https://infinite-table.com/blog/2024/04/18/the-best-testing-setup-for-frontends-playwright-nextjs
- https://playwright.dev/docs/test-configuration
- https://playwright.dev/docs/test-webserver
- https://playwright.dev/docs/api/class-testconfig
- https://www.pronextjs.dev/workshops/next-js-production-project-setup-and-infrastructure-fq4qc/e2-e-testing-with-playwright-d3eyw
Featured Images: pexels.com