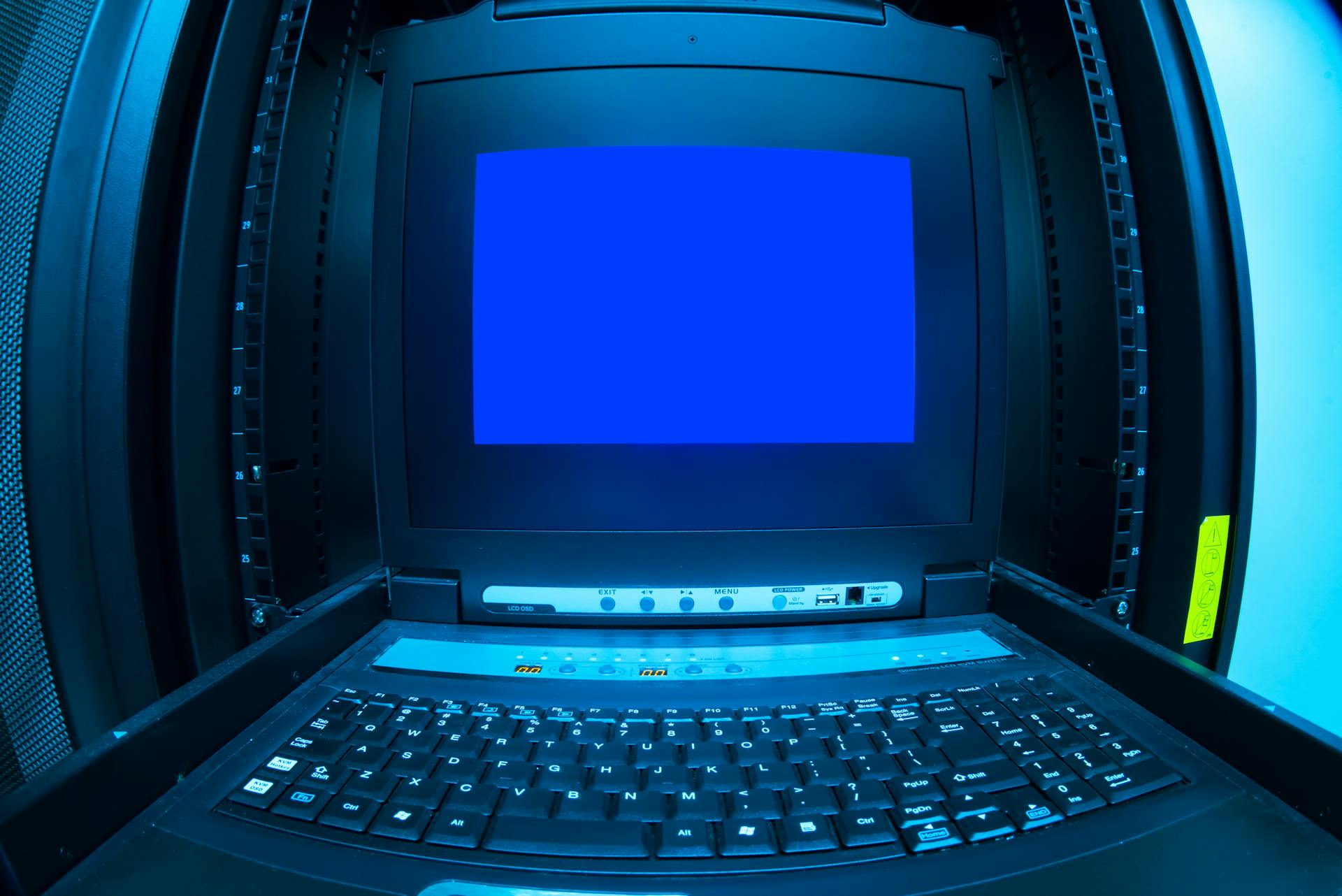
Azure Playwright is a browser automation tool that allows you to write and run tests for web applications. It's a game-changer for developers who want to ensure their apps work flawlessly.
To get started with Azure Playwright, you'll need to install it on your machine. This can be done using npm or yarn by running the command "npm install azure-playwright" or "yarn add azure-playwright".
Prerequisites
To get started with Azure Playwright, you'll need to meet some prerequisites. First, you'll need an Azure account with an active subscription, and if you don't have one, you can create a free account.
A Microsoft Playwright Testing workspace is also required, which you can create by following the quickstart: run Playwright tests at scale.
You'll also need a GitHub account, which you can create for free if you don't already have one. Next, you'll need a GitHub repository that contains your Playwright test specifications and GitHub Actions workflow.
To create a repository, see Creating a new repository. You'll also need to set up authentication from GitHub Actions to Azure, which you can find instructions for in Use GitHub Actions to connect to Azure.
In addition to GitHub, you'll need an Azure DevOps organization and project, which you can create for free if you don't already have one. You'll also need a pipeline definition, which you can create by following the steps in create your first pipeline.
Finally, you'll need an Azure Resource Manager Service connection to securely authenticate to the service from Azure Pipelines, which you can find instructions for in Azure Resource Manager service connection.
Here's a summary of the prerequisites:
Setting Up Azure Playwright
To set up Azure Playwright, you'll need to create a new file called PlaywrightServiceSetup.cs in the root directory of your project. This file will facilitate authentication of your client with the service.
First, create a new file called PlaywrightServiceSetup.cs in the root directory of your project. This file will be used to facilitate authentication of your client with the service.
Next, add the following content to the PlaywrightServiceSetup.cs file: using Azure.Developer.MicrosoftPlaywrightTesting.NUnit; using NUnit.Framework; namespace PlaywrightTests; // Remember to change this as per your project namespace [SetUpFixture] public class PlaywrightServiceSetup : PlaywrightServiceNUnit { };
To confirm that Rich diagnostics using reporting setting is ON for your workspace, go to the workspace settings and look for the "Rich diagnostics using reporting" setting. If it's not enabled, enable it to avoid failures.
Here are the steps to set up the service configuration:
- Create a new file PlaywrightServiceSetup.cs in the root directory of your project.
- Add the following content to it: using Azure.Developer.MicrosoftPlaywrightTesting.NUnit; using NUnit.Framework; namespace PlaywrightTests; // Remember to change this as per your project namespace [SetUpFixture] public class PlaywrightServiceSetup : PlaywrightServiceNUnit { };
- Save and commit the file to your source code repository.
Running Tests
Running tests with Azure Playwright is a breeze, and one of the key features is the ability to shard tests between multiple machines. This allows you to execute your test suite on multiple machines, making it faster and more efficient.
You can set up sharding in your configuration file, which is a crucial step in getting the most out of Azure Playwright's test capabilities.
Sharding can significantly reduce the overall test execution time, which is especially important for large test suites.
Performance and Optimization
Azure Playwright's performance optimization is key to getting the most out of your automation tests. By using the browser context, you can significantly reduce the time it takes to run your tests.
The browser context allows you to run multiple browser instances within a single process, which can lead to a substantial performance boost.
Using the playwright.config file, you can configure the browser context to run in headless mode, which can further improve performance by reducing the overhead of rendering the browser UI.
Browser contexts can also be reused, which can help reduce the number of browser instances created, resulting in improved performance and reduced resource usage.
By leveraging these features, you can significantly improve the performance of your Azure Playwright tests and make them more efficient.
Customizing Test Behavior
You can customize the behavior of your tests by using the various options available in Azure Playwright. For example, you can use the `timeout` option to set a timeout for your test, as shown in the article section where it's mentioned that "You can also set the timeout to 30 seconds by passing the `timeout` option to the `test` method".
To customize the behavior of your tests, you can also use the `retry` option to specify how many times a test should be retried before failing. This can be useful when dealing with flaky tests that occasionally fail due to external factors.
By customizing the behavior of your tests, you can make them more robust and reliable, and ensure that they run smoothly and efficiently.
Control Test Order
Control Test Order is crucial for ensuring your tests run smoothly and efficiently. Playwright Test runs tests from a single file in the order of declaration.
If you parallelize tests in a single file, there's no guarantee about the order of test execution across the files, because Playwright Test runs test files in parallel by default.
You can control test order by disabling parallelism. This allows you to name your files in alphabetical order or use a "test list" file.
It's worth noting that running tests in parallel can be beneficial, but it also means you'll have to adjust your naming conventions or use a test list file to ensure a specific order.
Isolate Test Data Between Workers
You can leverage process.env.TEST_WORKER_INDEX or testInfo.workerIndex to isolate user data in the database between tests running on different workers. All tests run by the worker reuse the same user.
To differentiate between workers, create a dbUserName fixture in a playwright/fixtures.ts file that initializes a new user in the test database. This ensures each worker has its own unique user.
You can't communicate between workers, so it's essential to isolate test data to avoid conflicts. Playwright Test reuses a single worker as much as it can to make testing faster, so multiple test files are usually run in a single worker one after another.
Here's a simple way to ensure data isolation between workers:
- Create a dbUserName fixture in playwright/fixtures.ts to initialize a new user in the test database.
- Use testInfo.workerIndex to differentiate between workers and isolate user data.
Reporting and Output
You can use multiple reporters at the same time to get different types of output, such as a nice terminal output with 'list' and a comprehensive json file with the test results.
The HTML reporter produces a self-contained folder that contains a report for the test run that can be served as a web page.
By default, the HTML report is written into the playwright-report folder in the current working directory, but you can override this location using the PLAYWRIGHT_HTML_OUTPUT_DIR environment variable or a reporter configuration.
You can control the behavior of the HTML report via the open property in the Playwright config or the PLAYWRIGHT_HTML_OPEN environmental variable, which can be set to always, never, or on-failure.
The HTML report supports the following configuration options and environment variables:
You can also use the JSON reporter to produce an object with all information about the test run, which can be written to a file using the PLAYWRIGHT_JSON_OUTPUT_NAME environment variable.
The JSON reporter supports the following configuration options and environment variables:
The Playwright report can also be used as an artifact, which can be downloaded and opened as an HTML file, but this requires additional steps and may take up space on your computer.
Frequently Asked Questions
What is Microsoft Playwright used for?
Microsoft Playwright is a tool for end-to-end testing of modern web applications, ensuring reliability and accuracy. It enables developers to automate browser interactions and verify their web app's functionality.
What is Azure Playwright testing?
Azure Playwright testing is a scalable solution for end-to-end web app testing, providing comprehensive reporting and streamlined continuous integration workflows. It offers a unified dashboard and cloud browsers for efficient testing and analysis.
Is Playwright a framework or tool?
Playwright is a framework for Web Testing and Automation, providing a unified API for testing multiple browsers. It's not a tool, but a comprehensive framework for automating web interactions.
Does Microsoft own Playwright?
Microsoft co-develops and maintains Playwright with a global community of contributors. While Microsoft is involved, Playwright is an open-source project.
Sources
- https://learn.microsoft.com/en-us/azure/playwright-testing/quickstart-automate-end-to-end-testing
- https://playwright.dev/docs/test-reporters
- https://playwright.dev/docs/test-parallel
- https://marcusfelling.com/blog/2023/handling-azure-ad-authentication-with-playwright/
- https://ultimateqa.com/playwright-reporters-how-to-integrate-with-azure-devops-pipelines/
Featured Images: pexels.com