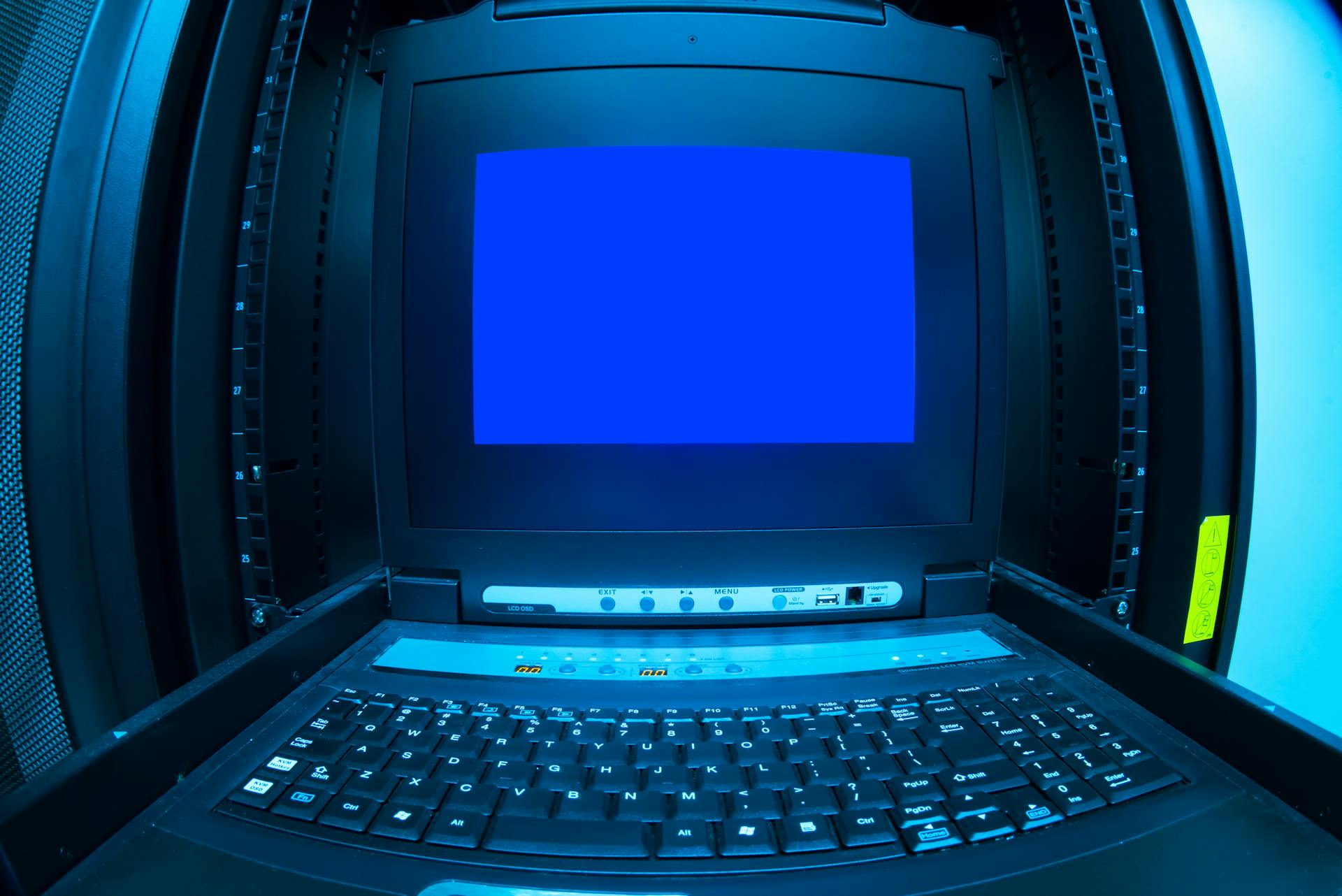
Azure Middleware offers a robust set of features and functionality that enable developers to build scalable and secure applications.
At the core of Azure Middleware is its ability to handle integration, API management, and service bus capabilities.
With Azure Middleware, developers can create event-driven architectures that allow for real-time communication between services.
This is achieved through the use of Azure Service Bus, which provides a messaging platform for integrating applications and services.
Azure Middleware also includes API Management, which enables developers to create scalable and secure APIs that can be consumed by a wide range of applications.
This is particularly useful for developers who need to expose their services to external partners or customers.
In addition to these features, Azure Middleware also includes support for message queues, which allow developers to handle high volumes of messages and events in a scalable and reliable manner.
Additional reading: Azure Devops Apis
Installation and Setup
To get started with Azure middleware, you'll need to install the necessary packages. Ensure that you have the Microsoft.Azure.Functions.Worker package installed, as it's already included in your project.
The NuGet package is a crucial component for Azure Functions and middleware. It's essential to have it installed to proceed with the setup.
To install the required packages, follow the instructions provided earlier. This will help you ensure that you have all the necessary components for Azure middleware.
Check this out: Azure Functions Core Tools
Customization and Configuration
You can register a custom middleware to enable capabilities like the Server Timing API, which allows for injecting logic into the invocation pipeline.
Custom middleware can be registered using the UseMiddleware() extension method, which is a convenient way to add middleware to the pipeline.
In Azure Functions, you can't use the convention-based middleware like in ASP.NET Core, but you can use the factory-based approach by implementing the IFunctionsWorkerMiddleware interface.
To register middleware, call the UseMiddleware() extension method inside the ConfigureFunctionsWorkerDefaults method, which registers the middleware as a singleton.
FunctionContext provides valuable information about the invoked function and the invocation itself, and its extension methods make it easier to work with.
You can access the HTTP response and modify it using the GetHttpResponseData() extension method, which returns an instance of HttpResponseData if the function has been invoked by an HTTP trigger.
To make this functional, you need to gather values for the header during the invocation, which requires a shared service between the function and the middleware.
You can add one or more custom middlewares to the execution pipeline using MiddlewareBuilder.
To define custom middlewares, try to implement the InvokeAsync(HttpContext) method for HTTP triggers and the InvokeAsync(ExecutionContext) method for non-HTTP triggers.
A unique perspective: Azure Function Core Tools Vscode
Function and Pipeline Management
To register your custom middleware in an Azure Functions app, use the UseMiddleware method, which ensures the middleware is invoked for every incoming request before any function logic is executed.
The order in which handlers are added to the middlewareHandler determines the order in which they'll be executed in the runtime. You can add a middleware using the use method.
Error handling functions will only be executed if there's an error thrown or returned to the context.next method, at which point normal function handling stops.
Here's a quick rundown of how middleware execution works:
Custom Exception Handling
Custom Exception Handling is a crucial aspect of Function and Pipeline Management. It allows you to catch and handle errors in a centralized way, making your application more robust and user-friendly.
To create a custom exception handling middleware, you'll need to wrap the next middleware or function execution in a try-catch block. This is done in the Invoke method, which catches any exceptions that occur during execution.
The middleware then creates an HTTP response with a 500 status code and serializes a custom error message, including a generic message and the exception details. This ensures that users receive a consistent error response, even if the error occurs in different parts of the application.
Here's a breakdown of the key features of custom exception handling middleware:
By implementing custom exception handling middleware, you can ensure that your application provides a better user experience, even in the face of errors. This is especially important for applications that handle sensitive data or have a high volume of users.
Registering in the Function App
To register your custom middleware in the function app, you'll need to use the UseMiddleware method. This method registers your middleware in the function app's pipeline, ensuring it's invoked for every incoming request before any function logic is executed.
The UseMiddleware method is a convenient extension provided by Azure Functions that enables registering inline or factory-based middleware. It's an alternative to working directly with FunctionExecutionDelegate, which represents the invocation pipeline in isolated worker process Azure Functions.
For your interest: Azure App Insights vs Azure Monitor
To use this method, you'll need to call it inside the ConfigureFunctionsWorkerDefaults method as part of the host preparation steps. This registers the middleware as a singleton, giving it the same lifetime as convention-based middleware in ASP.NET Core.
Here are the key steps to register your middleware:
- Use the UseMiddleware method to register your custom middleware.
- Call the UseMiddleware method inside the ConfigureFunctionsWorkerDefaults method.
- Register the middleware as a singleton to ensure it has the same lifetime as convention-based middleware.
Add Custom to Pipeline
Adding custom middleware to the pipeline is a crucial step in Function and Pipeline Management. This allows you to inject logic into the invocation pipeline, enabling capabilities like the Server Timing API.
To add custom middleware, you can use the MiddlewareBuilder, as mentioned in Example 3. This method allows you to add one or more custom middlewares to the execution pipeline.
Here are the steps to add custom middleware:
- Use MiddlewareBuilder to add custom middlewares to the execution pipeline.
- Implement the InvokeAsync method for HTTP triggers (Example 4).
- Implement the InvokeAsync method for non-HTTP triggers (Example 4).
- Use FunctionsMiddleware for returning IActionResult (Example 4).
- Use TaskMiddleware for non-HTTP triggers (Example 4).
The order in which handlers are added to the handler determines the order in which they'll be executed in the runtime (Example 6).
If you want to define a predicate as the first argument, you can use the useIf method (Example 7). If the predicate resolves to false, the function handler won't be executed.
To validate function input, you can use Joi to create and validate schemas, as shown in Example 10.
Error handling functions will only be executed if there's an error has been thrown or returned to the context.next method (Example 11).
Azure Function Modes
Azure Function Modes are crucial to understand when managing your functions and pipelines. There are two execution modes in Azure Functions: in-process and isolated worker process.
The in-process mode runs the function code in the same process as the host, which was the original approach for .NET functions. This enabled unique benefits like rich bindings and direct access to SDKs, but came with a price: .NET functions could only use the same .NET version as the host.
Dependency conflicts were common with in-process mode, which is why Azure Functions fully embraced the isolated worker process mode for .NET functions in version 4. This mode is now the default option for .NET functions, offering more flexibility and fewer dependency conflicts.
In some cases, the isolated worker process mode is the only option, such as when using non-LTS versions of .NET or when you need to support custom middleware registration, like with the Server Timing API.
Additional reading: When Did Azure Launch
Port IoT C SDK Application
If you're migrating from Azure IoT C SDK, you can find the porting process in our document.
Porting from Azure IoT C SDK is a straightforward process, and our document details the steps you need to take.
You can find this document by following the link provided in the Azure IoT C SDK section.
Frequently Asked Questions
What is a middleware in Azure?
Middleware in Azure is a software layer that connects applications, enabling data and database interactions between them. It facilitates tasks like form submissions and dynamic web page rendering based on user profiles.
What are examples of middleware?
Middleware examples include web servers that connect websites to backend databases, and other software that facilitates communication between different systems or applications. These intermediaries enable data exchange and processing, making complex systems more efficient and scalable.
Is Azure Service Bus middleware?
Azure Service Bus is a type of middleware component used in cloud-based systems. It acts as a bridge between applications, enabling communication and data exchange.
Sources
- https://dev.to/codestreet/how-to-create-custom-middleware-in-azure-functions-a-step-by-step-guide-with-net-8-17gp
- https://www.tpeczek.com/2023/03/server-timing-api-meets-isolated-worker.html
- https://github.com/emanuelcasco/azure-middleware
- https://azure.github.io/azure-iot-middleware-freertos/
- https://www.nuget.org/packages/AzureFunctions.Extensions.Middleware/2.1.0
Featured Images: pexels.com