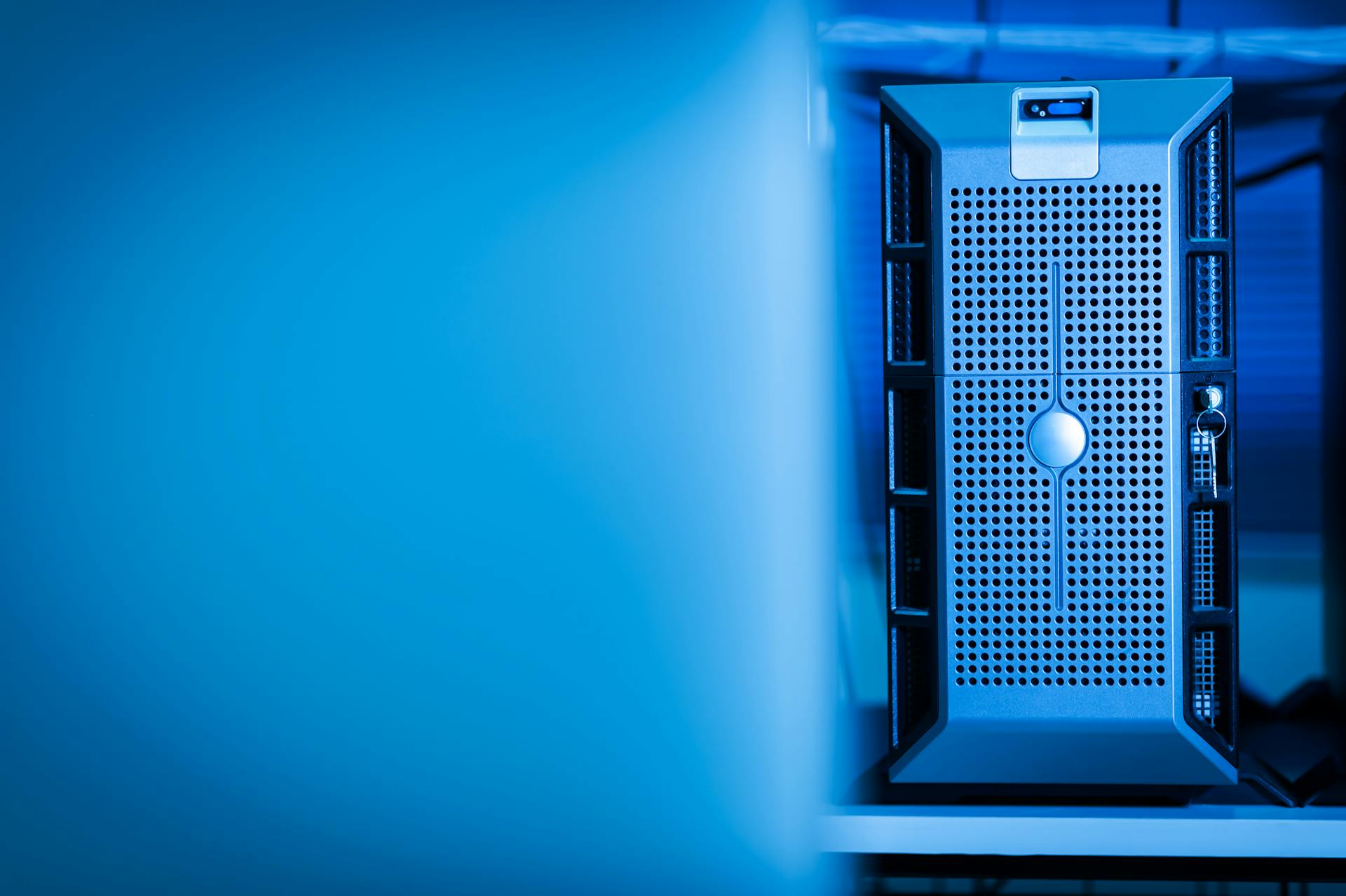
The Azure Functions Core Tools make local development a breeze.
With the Core Tools, you can create, test, and debug Azure Functions directly on your machine.
You can use the Core Tools to create a new Azure Function project in just a few seconds, and start writing code right away.
The Core Tools also allow you to run your Azure Functions locally, without needing to create a new Azure Function App in the Azure portal.
This means you can test and iterate on your code quickly, without incurring any additional costs or waiting for Azure resources to become available.
You might enjoy: Azure Functions .net 8
Development Environments
If you're looking to develop Azure Functions, you have several local development environments to choose from.
Visual Studio Code is a popular choice, supporting languages like C#, JavaScript, PowerShell, and Python, and can be used on Linux, macOS, and Windows with version 2.x of the Core Tools.
You can also use the Command prompt or terminal, which supports the same languages and can be used on the same platforms as Visual Studio Code.
Here's an interesting read: Code Interpreter Azure
If you prefer to use Visual Studio, you can use it to develop C# functions, and it's included in the Azure development workload starting with Visual Studio 2019.
Maven is another option, specifically for Java functions, and supports development on Linux, macOS, and Windows with version 2.x of the Core Tools.
All of these environments use the Core Tools for local testing and development, and can be used to create function app projects and publish them to Azure.
Here are the local development environments that support Azure Functions development:
These environments will get you up and running with Azure Functions development, and you can use them to create and publish function app projects to Azure.
File Management
File Management is a crucial aspect of Azure Functions, and Core Tools can help you manage your files efficiently. Azure Functions Core Tools allows you to run, debug, and manage your Functions locally.
You can use the `func host start` command to start your Functions host, which will allow you to run and debug your Functions locally. This command also enables file watching, which means that your Functions will automatically restart if you make any changes to your code.
Recommended read: Debug Azure Function Locally
With Core Tools, you can manage your files using the `func` command, which provides a set of subcommands for creating, deleting, and updating Functions. You can also use the `func host` command to manage your Functions host.
Azure Functions Core Tools also includes a feature called "watch" that allows you to run your Functions and automatically restart them if you make any changes to your code. This feature is especially useful when you're developing and testing your Functions.
Worth a look: Azure Feature Flags
Project Deployment
To deploy your Azure Functions project, you can use the `func azure functionapp publish` command, which publishes project files from the current directory to the function app in Azure as a .zip deployment package.
This command overwrites existing files in the remote function app deployment, so make sure to back up any important data before deploying. You'll also need to have already created a function app in your Azure subscription.
You can verify your deployment by using the `az aks browse` command to start the Kubernetes dashboard, then looking for the namespace "azure-functions" and checking that a pod has been deployed successfully with your container.
Check this out: Azure Function Disappeared after Deployment
Here are some key considerations to keep in mind when deploying your project:
- Publishing overwrites existing files in the remote function app deployment.
- You must have already created a function app in your Azure subscription.
- A project folder may contain language-specific files and directories that shouldn't be published, which are listed in a .funcignore file.
- Use the --publish-local-settings option to automatically create app settings in your function app based on values in the local.settings.json file.
Deploy Project Files
To deploy your project files, you'll need to use a command-line tool, such as Core Tools or Maven.
The func azure functionapp publish command is used to publish project files from the current directory to the FunctionAppName as a .zip deployment package. If your project requires compilation, it's done remotely during deployment.
You must have already created a function app in your Azure subscription. If you haven't, you can create one using the Azure CLI or Azure PowerShell.
To exclude items that shouldn't be published, such as language-specific files and directories, you can use a .funcignore file in the root project folder.
By default, your project is deployed so that it runs from the deployment package. To disable this, use the --nozip option.
Here are some additional options you can use when deploying your project:
- --no-build: This option controls whether a remote build is performed on compiled projects.
- --publish-local-settings: This option automatically creates app settings in your function app based on values in the local.settings.json file.
- --slot: This option allows you to publish to a specific named slot in your function app.
Deploy Containers
Deploying containers is a crucial step in project deployment, and Azure Functions provides a seamless experience for doing so. You can deploy your containerized function app to both managed Azure Container Apps environments and Kubernetes clusters that you manage.
To deploy to Container Apps, you'll need to use the func azurecontainerapps deploy command. This command will deploy an existing container image to a Container Apps environment.
The environment and storage account must already exist, and you'll need to provide the storage account connection string. You don't need to create a separate function app resource when deploying to Container Apps.
Storage connection strings and other service credentials are important secrets that should be securely stored. You can encrypt the local.settings.json file for added security.
Here are the considerations to keep in mind when deploying to Container Apps:
- The environment and storage account must already exist.
- You don't need to create a separate function app resource.
- Storage connection strings and other service credentials should be securely stored.
Alternatively, you can deploy to a Kubernetes cluster using the func kubernetes deploy command. This command will use the Dockerfile to generate a container in the specified registry and deploy it to the default Kubernetes cluster.
To deploy to Kubernetes, you'll need to have a Dockerfile for your project. You can generate one using the Azure Functions tool.
Here's a step-by-step guide to deploying to Kubernetes:
- Generate a Dockerfile for your project.
- Use the func kubernetes deploy command to deploy to Kubernetes.
Once you've deployed your container, you can verify its deployment by using the Kubernetes web dashboard. To start the dashboard, use the az aks browse command.
In the dashboard, look for the namespace "azure-functions" and make sure that a pod has been deployed successfully with your container.
Curious to learn more? Check out: Azure Functions Are Built and Deployed
Deploy to Knative
Deploying to Knative is a great way to scale your functions. You can deploy Azure Functions to Knative with the --platform knative flag.
The Core Tools CLI has your back, and it identifies non-HTTP trigger functions. It annotates the Knative manifest with the minScale annotation to opt out of scale-to-zero.
Deploy the Project
To deploy your project, you'll need to use the `func azure functionapp publish` command, which publishes project files from the current directory to the function app in Azure as a .zip deployment package. This command is the standard way to deploy your project to Azure.
If you're using Java, you'll need to use Maven to publish your local project to Azure instead of Core Tools. The Maven command to use is `mvn azure-functions:deploy`. This command creates Azure resources during the initial deployment based on the settings in your `pom.xml` file.
You can also use the `func azurecontainerapps deploy` command to deploy your containerized function app to a managed Azure Container Apps environment or a Kubernetes cluster. This command is useful if you have a containerized function app that you want to deploy to Azure.
Before deploying to Azure, make sure you have already created a function app in your Azure subscription. If you try to publish to a function app that doesn't exist, you'll get an error.
Here are some key things to keep in mind when deploying to Azure:
- Publishing overwrites existing files in the remote function app deployment.
- You must have already created a function app in your Azure subscription.
- A project folder may contain language-specific files and directories that shouldn't be published.
- Excluded items are listed in a `.funcignore` file in the root project folder.
- By default, your project is deployed so that it runs from the deployment package.
- A remote build is performed on compiled projects.
- Use the `--publish-local-settings` option to automatically create app settings in your function app based on values in the local `settings.json` file.
- To publish to a specific named slot in your function app, use the `--slot` option.
Runtime and Instances
To start the Azure Functions runtime, you need to run the `func start` command from the root directory of your project. This command enables triggers for all functions in the project.
By default, authorization isn't enforced locally for HTTP endpoints, so all local HTTP requests are handled as authLevel = "anonymous". However, you can use the `--enableAuth` option to require authorization when running locally.
You can use the local Azurite emulator when running functions that require access to Azure Storage services without having to connect to these services in Azure. Start Azurite before starting the local host to use local emulation.
To start a new orchestration instance, you can use the `start-new` method on the orchestration client binding. This method writes a message via the Durable Functions storage provider and then returns, asynchronously triggering the start of an orchestrator function with the specified name.
The parameters for starting a new orchestration instance are as follows:
You can use a random identifier for the instance ID whenever possible to ensure an equal load distribution when scaling orchestrator functions across multiple VMs.
Storage and Bindings
You can use the local Azurite emulator when testing functions with Azure Storage bindings, such as Queue Storage, Blob Storage, and Table Storage, without having to connect to remote storage services.
To use the Azurite emulator, you must have it installed and running, and you should test with an actual storage connection to Azure services before publishing to Azure.
You can download a storage connection string using the func azure storage fetch-connection-string command, which adds a setting named mystorage12345_STORAGE to the local.settings.json file.
Here are some key considerations for using storage emulation during local execution:
- You must have Azurite installed and running.
- You should test with an actual storage connection to Azure services before publishing to Azure.
- When you publish your project, don't publish the AzureWebJobsStorage setting as UseDevelopmentStorage=true.
Storage Emulator
You can use the local Azurite emulator when testing functions with Azure Storage bindings, such as Queue Storage, Blob Storage, and Table Storage, without having to connect to remote storage services. This emulator integrates with Visual Studio Code and Visual Studio, and you can also run it from the command prompt using npm.
To use Azurite, you must have it installed and running. You can install it and run it from the command prompt using npm. Azurite tells the local Functions host to use it for the default AzureWebJobsStorage connection.
For your interest: Azure Cosmos Db Emulator
To set up Azurite in your local.settings.json file, add the following setting value: "AzureWebJobsStorage": "UseDevelopmentStorage=true". This tells the local Functions host to use Azurite for the default AzureWebJobsStorage connection.
Here are some considerations to keep in mind when using storage emulation during local execution:
- You must have Azurite installed and running.
- You should test with an actual storage connection to Azure services before publishing to Azure.
- When you publish your project, don't publish the AzureWebJobsStorage setting as UseDevelopmentStorage=true.
Remember, when you publish your project, the AzureWebJobsStorage setting must always be the connection string of the storage account used by your function app.
Add a Binding
Adding a binding to your function is a straightforward process. You can add an input or output binding to an existing function by manually updating the function definition.
You'll need to define the output binding based on your process model. For example, when adding a Queue Storage output binding to an HTTP triggered function, you'll need to update the function definition to include the output binding.
The way you define the output binding depends on your Node.js model. For example, if you're using ASP.NET Core integration, you might need to change `HttpRequest` to `HttpRequestData` and `IActionResult` to `HttpResponseData`.
You can also use Visual Studio Code to simplify the process of adding bindings to an existing function definition.
Here are some considerations to keep in mind when adding bindings to a function:
- For languages that define functions using the function.json configuration file, Visual Studio Code simplifies the process of adding bindings to an existing function definition.
- You must also add an application setting that references a connection string or managed identity to the local.settings.json file.
- You might need to add a reference to a specific binding extension in your C# project.
- Non-HTTP functions can be triggered locally without connecting to a live service.
To add a binding to your function, you'll need to manually update the function definition and add the necessary application settings and references to your project.
Installation and Updates
To install Azure Functions Core Tools, you need to choose the right installer based on your operating system.
If you're using Windows, you can download and run the Core Tools installer, which is available in both 64-bit and 32-bit versions. The 64-bit version is recommended, as it's required for Visual Studio Code debugging.
On macOS, you'll need to install Homebrew if it's not already installed, and then run the command `brew tap azure/functions` followed by `brew install azure-functions-core-tools@4`. If you have an older version installed, you'll need to run `brew link --overwrite azure-functions-core-tools@4` as well.
Curious to learn more? Check out: Install Azure Cli Windows
For Linux distributions, you can use APT to install Core Tools, but you'll need to check the Core Tools readme for specific instructions.
You can also install or update Core Tools using the Azure Functions extension for Visual Studio Code, by running the command `Azure Functions: Install or Update Core Tools` from the command palette.
Before installing, make sure to uninstall any old versions of Core Tools from Add Remove Programs on Windows.
A unique perspective: Azure Linux Cli
Upload
You can upload local settings to Azure by using the --publish-local-settings option or the --publish-settings-only option. The --publish-local-settings option uploads local project files and settings, while the --publish-settings-only option uploads just the settings without republishing the project files.
The --publish-settings-only option is useful for updating settings in your function app without redeploying your project files. This can save you time and effort, especially if you only need to update a few settings.
You can use the --publish-settings-only option to upload settings from the local.settings.json file to your function app in Azure. For example, you can upload just the settings from the Values collection in the local.settings.json file to a function app named myfunctionapp12345.
Readers also liked: Azure App Insights vs Azure Monitor
Install or Update
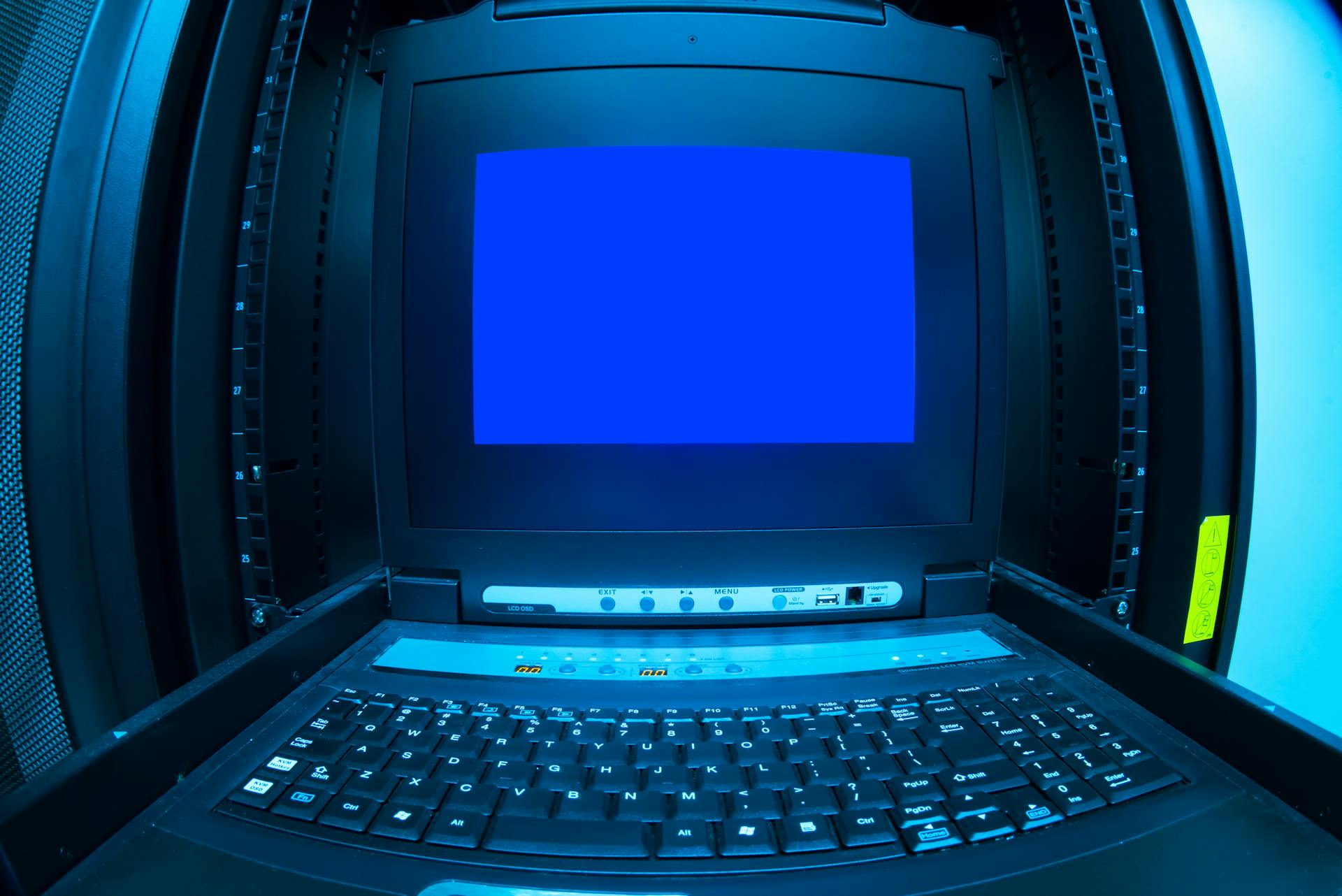
To install or update Core Tools, you have a few options. You can use the Azure Functions extension for Visual Studio Code to install or update Core Tools by selecting F1 to open the command palette and searching for and running the command Azure Functions: Install or Update Core Tools.
This command will try to install or update the latest version of Core Tools using a package-based installation. If you don't have npm or Homebrew installed on your local computer, you'll need to manually install or update Core Tools.
The recommended way to install Core Tools depends on your operating system - Windows, macOS, or Linux. For Windows, you can download and run the Core Tools installer, while for macOS and Linux, you can use Homebrew or APT to install Core Tools.
Here are the specific steps for each operating system:
Core Tools and Versions
You can only install one version of Core Tools on a given computer. This means you can't have multiple versions running side by side.
To determine the version of your current Core Tools installation, run the following command. This will give you a clear idea of what version you're working with.
The recommended major version of both the Functions runtime and Core Tools is version 4.x. You can install this version by following the instructions in the Azure Functions Core Tools repository.
Here are some key considerations to keep in mind when working with Core Tools installations:
- You can only install one version of Core Tools on a given computer.
- When upgrading to the latest version of Core Tools, you should use the same method that you used for original installation.
- Version 2.x and 3.x of Core Tools have reached their end of support.
File
The File section of your Core Tools and Versions is where you'll find the essential tools for managing and editing files.
The File section includes a File Explorer, which allows you to browse and navigate through your files and folders.
You can use the File Explorer to create new folders, rename files, and even copy or move files to different locations.
The File section also includes a File Manager, which provides advanced features for managing your files, such as searching, sorting, and filtering.
See what others are reading: Azure Data Studio vs Azure Data Explorer
The File Manager is particularly useful for finding specific files quickly, especially when you have a large number of files to manage.
You can use the File Manager to view file properties, such as file size, creation date, and permissions.
The File section also includes a File Editor, which allows you to edit and modify file contents directly.
The File Editor provides a range of features, including syntax highlighting, code completion, and debugging tools.
Run the
To run a function in Azure, you can use the command palette in Visual Studio Code. Press F1 to display the command palette, then search for and run the command Azure Functions: Execute Function Now, and select your subscription if prompted.
To select your function, you'll need to choose your new function app resource and HttpExample as your function.
You'll need to enter a request body type, such as { "name": "Azure" }, and press Enter to send the request message to your function.
The response will be displayed in the notification area, and you can expand the notification to review the full response.
Core Tools
Core Tools are a must-have for any Azure Functions developer. You can start an instance directly using the `func durable start-new` command, which takes parameters such as function-name, input, id, connection-string-setting, and task-hub-name.
To run Core Tools commands, you need to be in the root directory of a function app. If you explicitly provide the connection-string-setting and task-hub-name parameters, you can run the commands from any directory.
Core Tools support only the default Azure Storage provider for persisting runtime state. This means that if you're using a different provider, you won't be able to use Core Tools.
Here are the parameters for the `durable get-runtime-status` command:
- connection-string-setting (optional): Name of the application setting containing the storage connection string to use.
- task-hub-name (optional): Name of the Durable Functions task hub to use.
To publish your local code to a function app in Azure, use the `func azure functionapp publish` command. This command publishes project files from the current directory to the function app as a .zip deployment package. If the project requires compilation, it's done remotely during deployment.
Additional reading: Azure Linux Function App
You can also use Maven to publish your Java project to Azure instead of Core Tools. Use the following Maven command to publish your project to Azure:
You can encrypt the local settings file using the `func encrypt-local-settings` command. This command encrypts the local.settings.json file, which improves security by protecting connection strings and other valuable data.
To decrypt an encrypted local setting, use the `func decrypt-local-setting` command. This command decrypts the setting so that you can work with it.
Discover more: Azure Function Local Settings Json
Sources
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-develop-local
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-run-local
- https://learn.microsoft.com/en-us/azure/azure-functions/create-first-function-vs-code-python
- https://learn.microsoft.com/en-us/azure/azure-functions/durable/durable-functions-instance-management
- https://github.com/Azure/azure-functions-core-tools
Featured Images: pexels.com