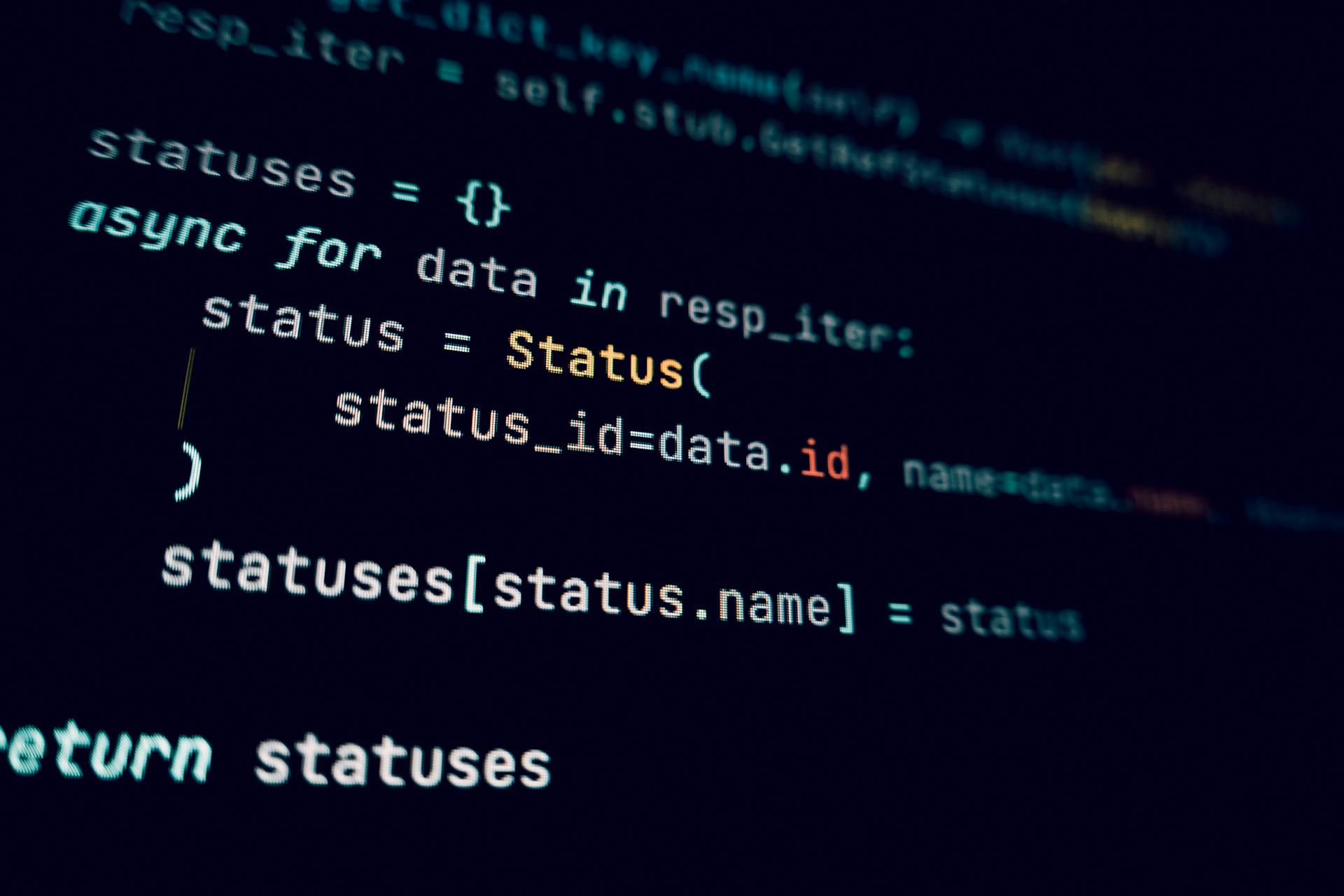
To create an Azure Function using .NET 8, you'll need to install the Azure Functions Core Tools. This can be done through the .NET CLI by running the command `dotnet tool install -g Azure.Functions.Cli`.
The Azure Functions Core Tools will allow you to create and manage Azure Functions from the command line. You can then use the `func new` command to create a new Azure Function.
To configure your Azure Function, you'll need to specify the runtime version, which in this case is .NET 8. This can be done by adding the `Microsoft.NET.Sdk.Functions` NuGet package to your project and setting the `TargetFramework` to `net8.0`.
A fresh viewpoint: Azure Devops Create New Area
Prerequisites
To get started with creating an Azure Function in .NET 8, you'll need to meet a few prerequisites.
You'll need to have Visual Studio 2022 installed, specifically with the Azure development workload selected during installation.
An Azure subscription is also required, and if you don't already have one, you can create a free account before beginning.
Here are the specific requirements:
- Visual Studio 2022 with Azure development workload
- Azure subscription
Creating the Azure Function
To create an Azure Function, start by adding a new function to your project in Visual Studio. Right-click your project node and select Add > New Azure Function. Enter a name for the class and select Add. Choose your trigger, set the required binding properties, and then select Add.
The trigger attributes are applied for you when you create your function triggers from the provided templates. Each function in the project can have a different trigger, but a function must have exactly one trigger.
To create a Queue storage trigger function, check the Configure connection box and you're prompted to choose between using an Azurite storage emulator or referencing a provisioned Azure storage account. Select Next and if you choose a storage account, Visual Studio tries to connect to your Azure account and get the connection string. Choose Save connection string value in Local user secrets file and then Finish to create the trigger class.
Here's an interesting read: Create Sample Azure Function for .net Frame Work
Here's an example of a basic Queue storage trigger function in C#:
```csharp
public class QueueTriggerCSharp
{
[Function(nameof(QueueTriggerCSharp))]
public void Run([QueueTrigger("PathValue", Connection = "ConnectionValue")] QueueMessage message)
{
_logger.LogInformation($"C# Queue trigger function processed: {message.MessageText}");
}
}
```
This function uses a QueueTrigger attribute, which indicates a Queue storage trigger function. The queue name and connection string setting name are passed as parameters to the QueueTrigger attribute.
Take a look at this: Computers Function
Publishing and Deployment
To publish your Azure Function .NET 8 project, you'll need to have a function app in your Azure subscription. You can create one in Visual Studio by right-clicking the project and selecting Publish.
You'll select Azure as the target and then Azure Function App (Windows), which will create a function app that runs on Windows. Next, you'll create a new instance of the function app by specifying the name, subscription, resource group, plan type, location, Azure Storage, and Application Insights instance.
To deploy your project to Azure, select Create to create the function app and its related resources. Then, make sure the Run from package file checkbox is selected, and select Finish. On the Publish pane, select Publish to deploy the package that contains your project files to your new function app in Azure.
You can also use the Azure portal to configure your project for local development. To do this, set the Values.AzureWebJobsStorage key to a valid Azure Storage account connection string, or use the Azurite emulator by setting the value to UseDevelopmentStorage=true.
Take a look at this: Create Windows Azure Account
Publish to Azure
Publishing to Azure is a straightforward process, but it does require some setup. You'll need to have a function app in your Azure subscription, which Visual Studio can create for you if you don't already have one.
To publish to Azure, start by right-clicking your project in Solution Explorer and selecting Publish. Then, select Azure as the target and click Next. Next, select Azure Function App (Windows) as the specific target, and click Next again.
When creating a new instance, you'll need to specify values for several settings, including Name, Subscription, Resource Group, Plan Type, Location, Azure Storage, and Application Insights. The Name should be a globally unique name that identifies your function app, and the Subscription should be your Azure subscription.
Here's a summary of the required settings:
Once you've specified these settings, select Create to create a function app and its related resources in Azure. Then, make sure the Run from package file checkbox is selected, and select Finish to deploy the package that contains your project files to your new function app in Azure.
If this caught your attention, see: How to Create Azure Function
Next Steps
Now that you've published and deployed your Azure Functions, it's time to take it to the next level.
To learn more about running C# functions in an isolated worker process, check out the Guide for running C# Azure Functions in an isolated worker process. This guide will walk you through the process and provide additional support.
You can also explore other versions of supported .NET versions in an isolated worker process by visiting .NET supported versions.
Adding an Azure Storage queue binding to your function is a great way to integrate with Azure Storage. This will allow you to send and receive messages from your function.
If this caught your attention, see: Which of the following Functions of a Computer Is Mostly?
Function App Settings
Function App Settings are a crucial part of Azure Functions development, and it's essential to understand how they work, especially when switching between local development and deployment to Azure.
The settings required by your functions are stored securely in app settings in Azure, but during local development, they're added to the Values collection in the local.settings.json file.
For another approach, see: Azure Create New App Service
Visual Studio doesn't automatically upload the settings in local.settings.json when you publish the project, so you'll need to upload them manually after publishing.
To do this, you can use the Manage Azure App Service settings link in the Hosting section of the Azure portal after successfully publishing your project.
This link displays the Application settings dialog for the function app, where you can add new application settings or modify existing ones.
You can also use the Azure CLI or the Azure Functions Core Tools to upload the settings.
Here are the three ways to upload the required settings to your function app in Azure:
- Use the Azure portal.
- Use the --publish-local-settings publish option in the Azure Functions Core Tools.
- Use the Azure CLI.
Remember, the local.settings.json file is not checked into source control by default, so if you clone a local Functions project from source control, you'll need to manually create the local.settings.json file in the project root for the Application settings dialog to work as expected.
Take a look at this: How to Create a File in Google Drive
Monitoring and Debugging
Remote debugging isn't recommended on a production service, so make sure to test your Azure function in a non-production environment first.
To debug your function app, you must publish a debug configuration of your project and enable remote debugging in your function app in Azure. This will allow you to attach a debugger to your function app and step through your code.
You can attach the debugger by following the steps outlined in the Azure documentation, which involve downloading a publish profile, attaching a debugger, and pasting a URL into the Attach to Process window. Be sure to allow Visual Studio access through your local firewall and provide the correct credentials.
If you're running into issues with remote debugging, be aware that Azure will automatically disable remote debugging after 48 hours, so you'll need to reenable it after that time.
To monitor the execution of your functions, it's recommended to integrate your function app with Azure Application Insights, which can be enabled during Visual Studio publishing or in Azure after the fact.
Explore further: Create Schema Azure Data Studio
Remote Debugging
Remote debugging is a powerful tool for identifying and fixing issues in your Azure Functions app. To debug your function app remotely, you must publish a debug configuration of your project and enable remote debugging in your function app in Azure.
Remote debugging is not recommended on a production service, as it can impact performance and security. If you have Just My Code debugging enabled, disable it before attempting remote debugging.
Remote debugging has some specific considerations to keep in mind. Here are some key points to be aware of:
- Remote debugging isn't recommended on a production service.
- If you have Just My Code debugging enabled, disable it.
- Avoid long stops at breakpoints when remote debugging.
- Remote debugging is automatically disabled in your function app after 48 hours.
To attach the debugger, you'll need to follow a specific process depending on your execution mode. If you're debugging an isolated worker process app, you'll need to attach the remote debugger to a separate .NET process and complete several other configuration steps.
Monitoring
Monitoring is a crucial part of ensuring your functions run smoothly.
You can monitor the execution of your functions by integrating your function app with Azure Application Insights.
This integration should be enabled during Visual Studio publishing.
If you missed this step, you can still enable Application Insights integration for your function app in Azure.
For your interest: Next Js Create App
Azure Function App and Project
To create an Azure Function App, you can start by creating an empty Function app from the Azure Portal. Simply click on Function App / Add and enter the App name, which will be used as the default domain name for the function.
Other parameters can be set according to your needs, but it's recommended to reuse the existing App Service Plan to save money. You can also choose .NET Core as the Runtime Stack.
To publish your project to Azure, you should use the recommended deployment method, Zip Deploy with Run-From-Package mode enabled. This can be done by right-clicking the project in Solution Explorer and selecting Publish, then following the steps in the Azure Function App (Windows) section.
Azure Functions Project
To set up your Azure Functions project for local development, you need to configure the project to use an Azure Storage account. You can either use an actual Azure Storage account or the Azurite emulator.
The Functions runtime uses an Azure Storage account internally, so you need to set the Values.AzureWebJobsStorage key to a valid Azure Storage account connection string. This connection string can be obtained from the Azure portal.
To get the connection string, navigate to your storage account in the Azure portal, and then copy the Connection string of key1 from the Access keys tab. This string will be used to set the value of the AzureWebJobsStorage key in your project's local.settings.json file.
You'll also need to add unique keys to the Values array for any other connections required by your functions. To do this, repeat the process of copying the connection string and adding it to the local.settings.json file.
Here's a step-by-step guide to setting the storage account connection string:
- In the Azure portal, navigate to your storage account.
- In the Access keys tab, below Security + networking, copy the Connection string of key1.
- In your project, open the local.settings.json file and set the value of the AzureWebJobsStorage key to the connection string you copied.
- Repeat the previous step to add unique keys to the Values array for any other connections required by your functions.
Remember to change the AzureWebJobsStorage setting to an actual storage account connection string before deployment.
Azure Function App
You can create an Azure Function App using Visual Studio or the Azure Portal. To publish your project to Azure, you must have a function app in your Azure subscription. If you don't already have a function app, Visual Studio publishing creates one for you the first time you publish your project.
In Visual Studio, you can right-click the project and select Publish, then follow the steps to create a function app and its related resources in Azure. The function app must have a globally unique name, and you can choose a subscription, resource group, plan type, location, Azure Storage, and Application Insights instance.
Here are the settings you need to specify when creating a function app in Visual Studio:
In the Azure Portal, you can create a function app by clicking Function App / Add, and then entering the app name and other parameters according to your needs. It's recommended to reuse an existing App Service Plan to save money. You can also choose the runtime stack to be .NET Core.
Introduction
In this article, we'll be focusing on Azure Function App and Project, specifically looking at the latest changes made available with the release of .NET 8. This is the second installment of a two-part series.
We've previously discussed the features made available by .NET 8, but now we're shifting our aim to the latest changes made available to Azure Functions.
Explore further: Compute Net Income
Overview
Azure Functions offers two models for building function apps: the in-process model and the isolated model. The main difference between the two lies in how they operate.
The in-process model runs functions within the same process as the host, coupling the user's code as they must run the exact same library/.NET versions as the host. This coupling was useful initially, but it's now being phased out.
The isolated model runs functions in a separate, isolated process, offering flexibility, control, and compatibility with a broader range of .NET versions, including .NET Framework. This makes it a more forward-looking approach.
Azure Functions has finally achieved complete feature parity between the two models, with the isolated model now offering equivalent input/output/trigger bindings and model types as the in-process model.
Here are some of the key features supported by the isolated model:
- Support for .NET Framework 4.8
- Support for .NET 7/.NET 8
- Support for Durable Functions
- Support for Application Insights from the worker
- Support for binding to SDK types
- Preview support for ASP.NET Core integration
Microsoft's clear preference for the isolated model signals a shift towards greater flexibility and decoupling of the host process and the application process itself.
Frequently Asked Questions
Does Azure support .NET 8?
Yes, Azure supports .NET 8, specifically for isolated worker model applications. This support allows for enhanced functionality and scalability.
Sources
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-create-your-first-function-visual-studio
- https://edi.wang/post/2019/8/10/create-azure-function-app-with-net-core-and-cd-from-github
- https://arinco.com.au/blog/unveiling-net-8-part-2-seamless-azure-functions-integration/
- https://joonasw.net/view/azure-ad-jwt-authentication-in-net-isolated-process-azure-functions
- https://learn.microsoft.com/en-us/azure/azure-functions/functions-develop-vs
Featured Images: pexels.com