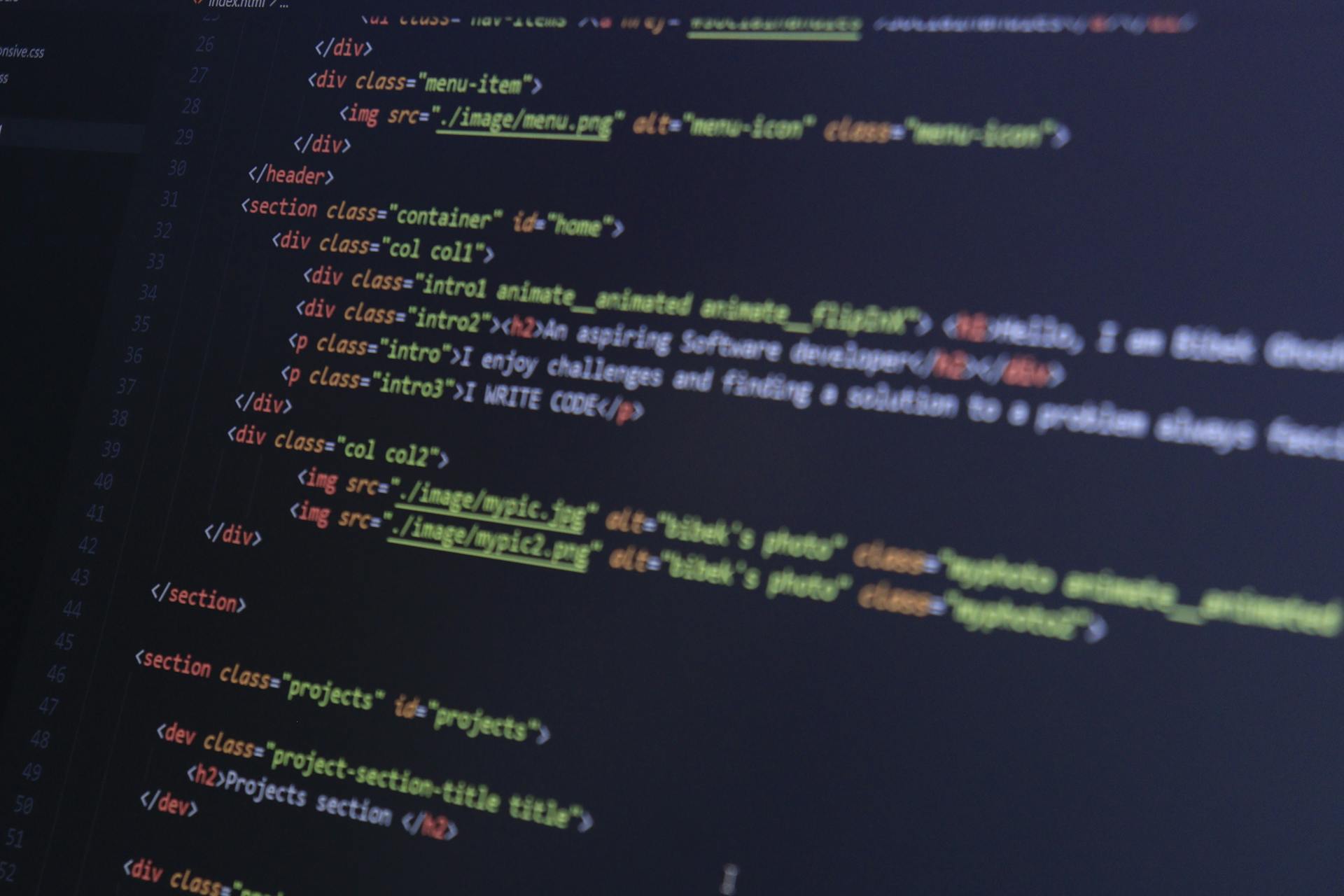
In Angular 8, you can dynamically add and remove CSS classes using the NgClass directive. This directive allows you to bind a class to a component's host element.
To add a CSS class dynamically, you can use an expression in the NgClass directive. For example, if you have a variable called `isDisabled` that determines whether a button should be disabled, you can use the NgClass directive like this: `[ngClass]="{'disabled': isDisabled}"`.
This will add the `disabled` class to the button's host element if `isDisabled` is true.
For more insights, see: Css Hide Class
Binding CSS Classes
Binding CSS classes in Angular 8 is a breeze, thanks to various methods and directives available.
You can use class binding for simple cases where you want to add a class to an element or not. For example, adding a disabled class to a button in a form when the form is invalid.
To add a class using Angular Renderer2, you need to inject it into your component via the constructor and use the add and remove class methods. This is useful when you need to add a class to the body element or other elements that aren't directly accessible within your component.
A different take: Pseudo Element Css
Another way to assign a CSS class in Angular is by using the [className] property binding. This is similar to other attribute bindings like img-src.
However, when it comes to toggling CSS classes, the syntax can be a bit inconvenient. A better way to do this is by using the class.name directive, which makes it clear what you're trying to achieve.
Angular's *ngClass directive is a game-changer for conditional class application. It allows you to bind a class name or an object to an element and specify the conditions under which the class should be applied.
Here are the various aspects of *ngClass:
- Boolean Properties: Define boolean properties in your component class and use them in the *ngClass expression.
- String Expressions: Use a string expression directly in the *ngClass directive to apply a class based on a condition.
- Array Expressions: Use an array expression to apply multiple classes conditionally.
- Object Expressions: Use an object expression when you have multiple classes and conditions.
Lastly, if you want to update the styling of the component itself rather than an element inside, you can use HostBinding and the :host selector.
Dynamic Class Management
Dynamic Class Management is a crucial aspect of Angular development, and there are several ways to manage classes dynamically in Angular 8. You can use the `ngClass` directive to conditionally apply classes to an element. This directive allows you to bind a class name or an object to an element and specify the conditions under which the class should be applied.
Recommended read: Tailwind Css Angular
One of the simplest ways to use `ngClass` is to define boolean properties in your component class and use them in the `ngClass` expression. For example, you can define a property `isClicked` and use it in the `ngClass` expression like this: `[ngClass]="{'text-darkgreen': isClicked}"`.
You can also use string expressions directly in the `ngClass` directive, like this: `[ngClass]="{'primary': true}"`. This will add the `primary` class to the element. Another option is to use an array expression, which allows you to apply multiple classes conditionally. For example: `[ngClass]="['primary', 'secondary']"`.
Here's a summary of the different ways to use `ngClass`:
Host binding a class is another way to apply classes to an element, but it's more suitable for applying classes to the component selector itself. You can use the `:host` selector to host bind a class, like this: `:host { class: 'primary' }`. This will apply the `primary` class to the component selector.
In addition to `ngClass`, you can also use the `Renderer2` class to add and remove classes dynamically. This is useful when you need to add or remove classes from a specific element, like the `document.body` element. You can inject the `Renderer2` class into your component and use its `addClass` and `removeClass` methods to add or remove classes. For example: `renderer2.addClass(document.body, 'primary')`.
Overall, dynamic class management is an essential part of building responsive and interactive Angular applications. By using the `ngClass` directive and other methods, you can easily add or remove classes based on specific conditions, making your application more flexible and adaptable to different scenarios.
For another approach, see: Remove Dropbox Ubuntu
NgClass Conditional Class
Angular's NgClass directive is a powerful tool for dynamically adding or removing CSS classes from an element. It's like a supercharged version of the class binding syntax.
You can use NgClass to toggle a class on or off with a single line of code, like this: `[ngClass]="{'class-name': condition}"`. For example, in Example 4, we can see how to toggle a class using the class.name directive, which is similar to NgClass.
NgClass can also be used to apply multiple classes conditionally. You can pass an array of class names and conditions to the directive, like this: `[ngClass]="[{class-name: condition}, {another-class: another-condition}]"`. This is useful when you need to apply different styles or behavior to an element based on multiple conditions.
Here are some examples of how to use NgClass in different scenarios:
In Example 5, we can see how to use NgClass to conditionally apply classes to an element. This is a great way to create responsive and interactive Angular applications.
You can also use NgClass in conjunction with other directives like *ngIf or *ngFor to conditionally apply classes based on changing states or data conditions. This is a powerful feature that can help you create complex and dynamic UI components.
On a similar theme: Jquery Apply Css Class
Sources
- https://briantree.se/4-ways-to-conditionally-add-classes-in-angular/
- https://malcoded.com/posts/angular-ngclass/
- https://stackoverflow.com/questions/51187256/angular-add-class-dynamically
- https://www.geeksforgeeks.org/angular-conditional-class-with-ngclass/
- https://medium.com/swlh/6-ways-to-dynamically-style-angular-components-b43e037852fa
Featured Images: pexels.com