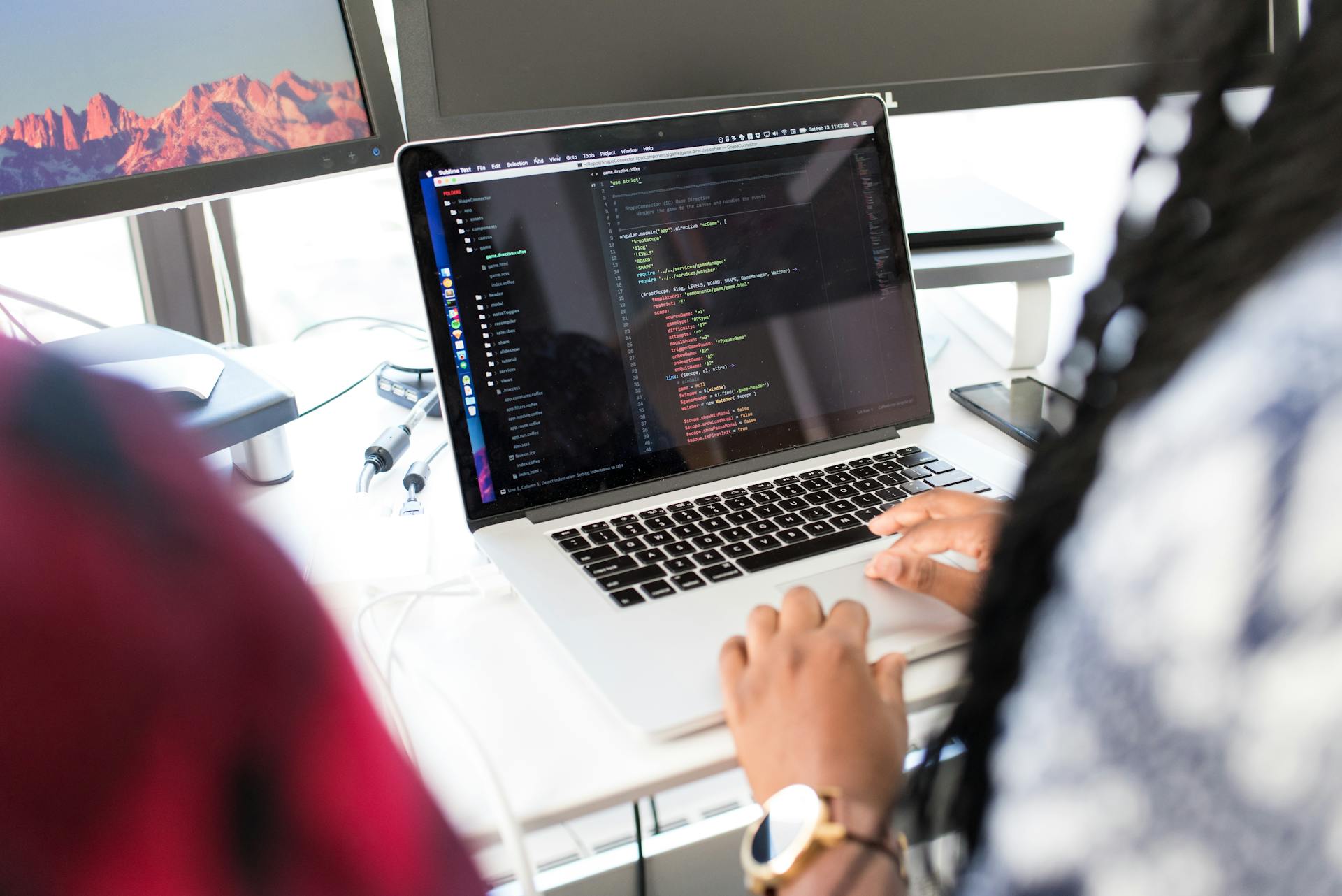
The Azure DevOps API is a powerful tool that allows developers to automate and integrate various aspects of their development workflow. It provides a comprehensive set of endpoints and APIs that can be used to manage projects, repositories, build pipelines, and more.
With over 100 APIs available, the Azure DevOps API offers a wide range of possibilities for customization and automation. This guide will walk you through the basics of getting started with the Azure DevOps API and provide a reference for common APIs and endpoints.
To get started with the Azure DevOps API, you'll need to authenticate your requests using a personal access token or a service principal. This can be done by creating a new token in the Azure DevOps organization settings.
The Azure DevOps API is built on top of REST, making it easy to use and integrate with other services.
API Basics
API Basics are essential to understanding how Azure DevOps works.
To interact with Azure DevOps, you'll need to know the HTTP verbs, which are used to perform different actions. For example, GET is used to get a resource or list of resources, while POST is used to create a resource or get a list of resources using a more advanced query.
Here are the HTTP verbs in a quick reference table:
Components of a Rest Pair
A REST API request/response pair can be broken down into five key components. The request URI is the first component, which is typically in the form of VERB https://{instance}[/{team-project}]/_apis[/{area}]/{resource}?api-version={version}. This format is specified in Example 3.
The HTTP request message header fields are the second component, which may include optional fields to support the URI and HTTP operation. For example, POST operations contain MIME-encoded objects that are passed as complex parameters.
The optional HTTP request message body fields are the third component, which can contain data to support the request. This data is typically passed as complex parameters in the request body.
Expand your knowledge: Azure Api Version
The HTTP response message header fields are the fourth component, which provide information about the response. These fields can include status codes, headers, and other metadata.
The optional HTTP response message body fields are the fifth component, which can contain data returned in the response. This data can be in the form of JSON, XML, or other formats.
In summary, a REST API request/response pair consists of five key components: request URI, HTTP request message header fields, optional request body fields, HTTP response message header fields, and optional response body fields.
For your interest: Azure Data Factory Testing
Http Verbs
HTTP verbs are the foundation of API interactions, and understanding them is crucial for building robust APIs.
The most common HTTP verbs are GET, POST, PUT, PATCH, and DELETE.
GET is used to retrieve a resource or a list of resources. For example, you can use a GET request to fetch a list of users from a database.
POST is used to create a new resource or to retrieve a list of resources using a more advanced query. This is useful when you need to send data in the request body.
For more insights, see: Azure Devops Parallelism Request
PUT is used to update an existing resource. If the resource doesn't exist, PUT will create it. This is useful when you need to update a resource with all the available information.
PATCH is similar to PUT, but it only updates specific parts of the resource, rather than replacing the entire resource.
DELETE is used to remove a resource from the system. This is useful when you need to delete a resource that's no longer needed.
Here's a quick summary of the HTTP verbs:
Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing (CORS) is supported by Azure DevOps Services, allowing JavaScript code to make Ajax requests to Azure DevOps Services REST APIs from any domain.
Each request must provide credentials, such as a personal access token or an OAuth access token.
Azure DevOps Services REST APIs can be accessed from a domain other than dev.azure.com/*.
To make an Ajax request, you'll need to replace "myPatToken" with your actual personal access token.
See what others are reading: Azure Data Factory Rest Api
Input Base
In the world of APIs, the foundation of any successful interaction is a well-defined input. The Input Base is the starting point for this process, and it's crucial to understand its components.
The Input Base is defined by the TaskInputDefinitionBase class, which includes several key properties.
The aliases property is an array of strings that provides alternative names for the input.
The defaultValue property is a string that specifies the default value for the input.
The groupName property is a string that identifies the group of inputs that this input belongs to.
The helpMarkDown property is a string that contains the help text for the input in Markdown format.
The label property is a string that displays a user-friendly name for the input.
The name property is a string that uniquely identifies the input.
The options property is an object that contains additional configuration options for the input.
The properties property is an object that contains custom properties for the input.
Explore further: How to Connect to Azure Cosmos Db Using Connection String
The required property is a boolean that indicates whether the input is mandatory or not.
The type property is a string that specifies the data type of the input.
The validation property is an object of type TaskInputValidation that defines the validation rules for the input.
The visibleRule property is a string that specifies the condition under which the input should be visible.
TFS Version Mapping
Azure DevOps Server vNext uses REST API Version 7.2, which works on server versions mentioned as well as later versions.
Azure DevOps Server 2022.1 uses REST API Version 7.1, and is compatible with build versions 19.225.34309.2 and later.
Azure DevOps Server 2022 uses REST API Version 7.0, and is compatible with build versions 19.205.33122.1 and later.
Azure DevOps Server 2020 uses REST API Version 6.0, and is compatible with build versions 18.170.30525.1 and later.
Azure DevOps Server 2019 uses REST API Version 5.0, and is compatible with build versions 17.143.28621.4 and later.
TFS 2018 Update 3 uses REST API Version 4.1, and is compatible with build versions 16.131.28106.2 and later.
TFS 2017 Update 2 uses REST API Version 3.2, and is compatible with build versions 15.117.26714.0 and later.
TFS 2015 Update 4 uses REST API Version 2.3, and is compatible with build versions 14.114.26403.0 and later.
The REST API version mapping is essential to ensure compatibility and accurate usage of the API.
A unique perspective: Azure 2
API Endpoints
Azure DevOps API endpoints allow you to interact with various services and retrieve data programmatically.
The API endpoints are organized into several categories, including projects, work items, and builds.
You can use the Projects - Get Project API endpoint to retrieve information about a project, including its ID, name, and description.
This endpoint returns a Project resource, which contains properties such as the project ID, name, and description.
To create a new project, you can use the Projects - Create Project API endpoint, which requires a Project resource as its request body.
This endpoint returns a 201 Created response with the newly created project's ID in the Location header.
Broaden your view: Azure Projects
Options
Options play a crucial role in API endpoints, allowing you to fine-tune how your application behaves. In the context of approvals, you can specify whether the approval can be skipped if the same approver approved the previous stage.
You can also enforce identity revalidation of the approver before completing the approval, which is a good practice to ensure the right person is approving the release. The execution order of approvals is another important option, which determines the sequence in which approvals are executed.
In some cases, you may want to allow the user requesting a release or deployment to also be an approver, which is a useful option to consider. The number of approvals required to move a release forward is also an important option, with '0' meaning all approvals are required.
Here's a summary of some key options:
In terms of gates, you can enable or disable them, and also set the minimum duration for steady results after a successful gates evaluation. The time between re-evaluation of gates, as well as the delay before evaluation, are also options you can configure.
DataSourceBindingBase
The DataSourceBindingBase represents the binding of a data source for a service endpoint request. It's a crucial part of API endpoints, allowing you to connect to external data sources.
The callbackContextTemplate property specifies the pagination format supported by the data source, which can be either ContinuationToken or SkipTop. This is important to consider when designing your API endpoints.
You can use the callbackRequiredTemplate property to determine if subsequent calls are needed. This helps with API endpoint management and optimization.
The dataSourceName property gets or sets the name of the data source, while the endpointId property gets or sets the endpoint ID. These properties are essential for identifying and accessing specific data sources and endpoints.
Here's a summary of the key properties of DataSourceBindingBase:
The endpointUrl property gets or sets the URL of the service endpoint, while the headers property gets or sets the authorization headers. These properties are vital for establishing a connection to the external data source.
The initialContextTemplate property defines the initial value of the query parameters, which helps with API endpoint configuration. The parameters property gets or sets the parameters for the data source, while the requestContent property gets or sets the HTTP request body.
The requestVerb property gets or sets the HTTP request verb, which is essential for specifying the action to be taken on the data source. The resultSelector property gets or sets the result selector, while the resultTemplate property gets or sets the result template.
Finally, the target property gets or sets the target of the data source, which is crucial for identifying the specific data source being accessed.
On a similar theme: Azure Data Studio for Mac
Azure Services
Azure Services use the instance dev.azure.com/{organization} and collection DefaultCollection.
You can get a list of projects in an organization by using the Azure DevOps Services pattern.
To authenticate with Azure DevOps Services, you can use personal access tokens (PATs) or other authentication mechanisms like Microsoft Authentication Library (MSAL), OAuth, and Session Tokens.
If this caught your attention, see: How to Use Azure Key Vault C#
To provide a PAT through an HTTP header, you must first convert it to a Base64 string.
The resulting string can then be provided as an HTTP header in the format.
Most samples use PATs for compact authentication.
The HttpClient class in C# can be used to make requests to Azure DevOps Services.
For more information on authentication mechanisms, see the Authentication guidance.
Frequently Asked Questions
What is rest API in DevOps?
A REST API is a type of web service that provides access to resources through standard HTTP methods, enabling create, read, update, and delete operations. It's a fundamental component in DevOps, facilitating communication between systems and applications.
Sources
- https://learn.microsoft.com/en-us/rest/api/azure/devops/release/releases/get-release
- https://learn.microsoft.com/en-us/rest/api/azure/devops/
- https://learn.microsoft.com/en-us/azure/devops/integrate/how-to/call-rest-api
- https://learn.microsoft.com/en-us/rest/api/azure/devops/wit/work-items/create
- https://stackoverflow.com/questions/71068040/azure-devops-api-authentication
Featured Images: pexels.com