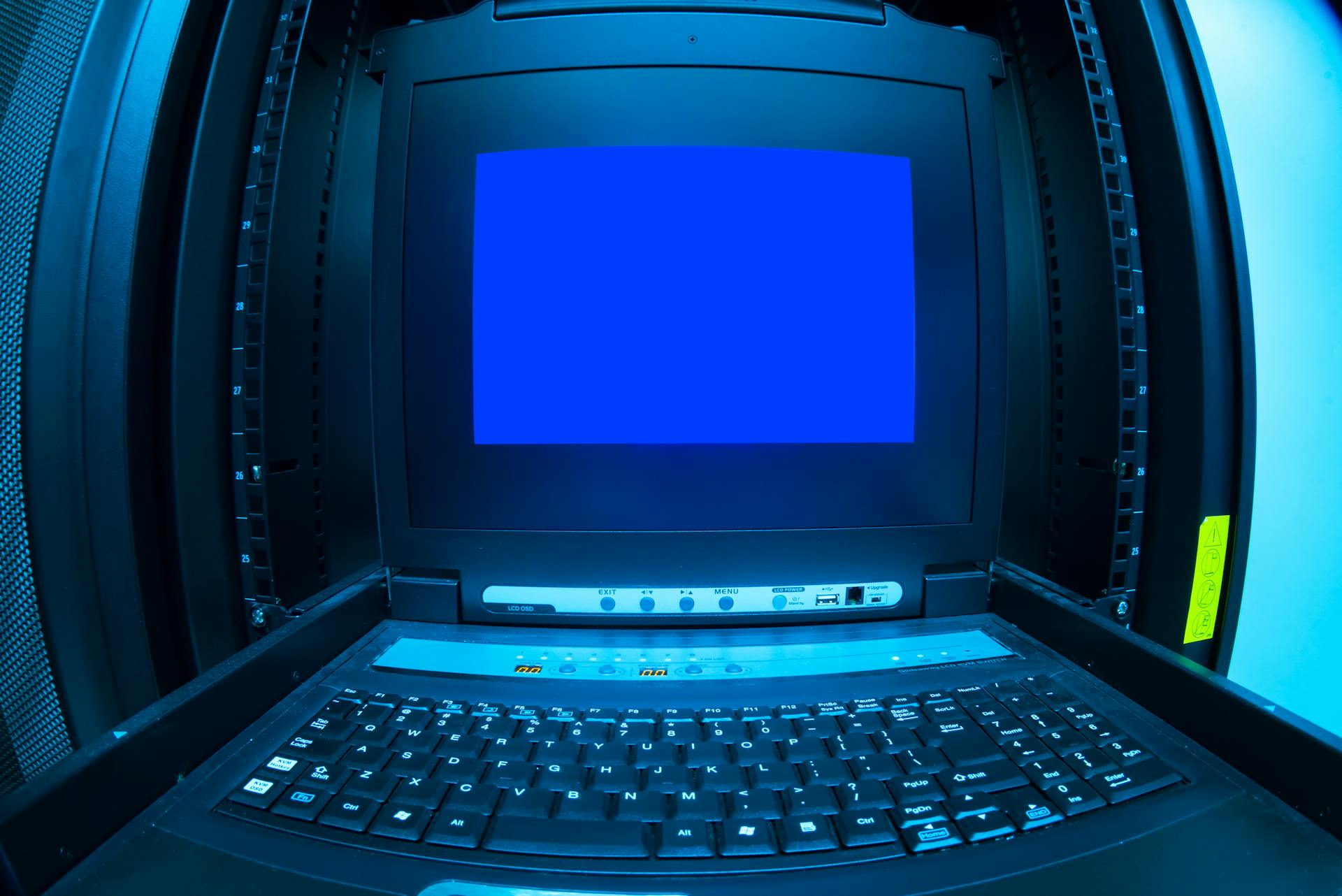
API versioning is a crucial aspect of Azure API management. Azure supports two primary methods of API versioning: URI-based and header-based.
URI-based versioning involves appending the version number to the API's base URI. For instance, if the base URI is `https://example.com/api`, the version 2 of the API would be `https://example.com/api/v2`.
Header-based versioning, on the other hand, uses a custom header to specify the API version. This approach is more flexible and allows for multiple versions to be used simultaneously.
Azure also supports content negotiation, which enables clients to specify the version of the API they want to use. This is done by setting the `Accept` header to the desired version.
API Versioning
API versioning is a crucial aspect of Azure API management. Each published version of an API is identified by a date value in YYYY-MM-DD format, called the api-version.
Newer versions have later dates, and all API operations require clients to specify a valid API version for the service via the api-version query string parameter in the URL. For example: https://management.azure.com/subscriptions?api-version=2020-01-01.
Client SDKs and tools include the api-version value automatically, making it easy to use the latest version of an API. However, in most scenarios, a service client will only need to interact with a single version of a service to access all of the functionality it requires.
Stable service versions generally remain available and supported for many years, even as newer versions become available. This means you can use a stable version of an API for a long time without worrying about it being deprecated.
Here are some of the stable versions of Azure API, along with their specifications and API updates:
In most cases, the only time you should adopt a new service version within existing code is to take advantage of new features.
Consider reading: Azure Create New App Service
Azure API Deployment
Continuous deployment of APIs can be achieved through Azure API Management Services, but it requires a series of manual steps, including deploying new versions of the API and updating the API Management Service.
To automate the deployment process, you can use a combination of REST API calls and PowerShell cmdlets, as demonstrated in Example 2. This approach allows you to create and update versioned APIs inside Azure API Management Services with ease.
By using this automated approach, you can avoid manual operations and ensure that all versions of the API are fully synced to Azure API Management Service at each new release. This includes importing all versions of the API, cleaning up version definitions that no longer exist, and keeping deprecated versions in a deprecated state for a while.
Here's a step-by-step overview of the automated deployment process:
- Authenticate through Azure AD App Registration and get an AccessToken to be used in REST API calls.
- Download the main Swagger HTML file and detect all versions that an API contains and paths to their Swagger JSON files.
- Import all versions of the API into Azure API Management Service using the Swagger JSON files discovered earlier.
- Clean up version definitions that no longer exist in the actual API itself.
Zero Downtime Deployments
Zero Downtime Deployments are a must-have for any API deployment. It's a process that allows you to deploy new versions of your API without interrupting existing users.
To achieve this, you can deploy the new version of your API as a separate application under a different internal URL. This way, you can test and verify the new version without affecting existing users.
Explore further: Bulk Invite Guest Users Azure
The process involves creating a new entry in Azure API Manager and pointing it to the new Azure App Service internally. You should always use deployment slots and swapping during your deployments.
Here are the steps to follow:
- Deploy the new version of your API as a separate application under a different internal URL.
- Create a new entry in Azure API Manager and point it to the new Azure App Service internally.
- Deploy the new version of your SPA on top of the old one.
- Await until all old users are gone, then drop the old version from Azure API Management Service and remove the old App Service deployment.
This process works, but it requires a lot of manual steps and checks. A better way would be to introduce versioning inside the codebase as a first-class citizen. This way, you can automate the deployment process and make it easier to manage different versions of your API.
Client SDKs
Client SDKs are a crucial part of Azure API deployment, and they're designed to make service versioning a thing of the past.
Each SDK is composed of client libraries, one for each service, and each client library version targets a single version of the service it relies on.
New stable versions of services are accompanied by new point releases of client libraries, so you'll need to upgrade your client library version to take advantage of new features.
Only beta-version client libraries use preview service versions, so be aware of that if you're working with beta releases.
Overriding a client library's default service version is an advanced scenario that may lead to unexpected behavior, so use it with caution and test your application thoroughly.
Readers also liked: Azure Ad Connect Current Version
Built-in Policy Definition
Azure offers a built-in policy definition that ensures API Management minimum API version is set to 2019-12-01 or higher.
This policy definition is called "API Management minimum API version should be set to 2019-12-01 or higher" and has the ID 549814b6-3212-4203-bdc8-1548d342fb67. It's available in the Azure Portal.
The policy definition is categorized under API Management and has a description that explains its purpose: to prevent service secrets from being shared with read-only users.
Here are the details of the policy definition:
This policy definition is part of the Azure Governance Visualizer (AzGovViz) and is used to track policy changes in the tenant. It's also associated with three compliance controls: Azure Security Benchmark v3.0, New Zealand ISM, and Microsoft cloud security benchmark.
The policy definition has a version of 1.0.1 and supports versioning, with the built-in versioning feature enabled.
For your interest: Is Azure a Word
API Documentation
API documentation is a crucial aspect of Azure API versioning. It helps developers understand how to use the API, including the different versions and their associated features.
API documentation should include information about the API's endpoints, request and response formats, and error handling. This information is essential for developers to write code that interacts with the API.
Azure API Management provides a built-in documentation feature that generates documentation automatically. This feature includes information about API versions, methods, and parameters.
Developers can also create custom documentation using Azure API Management's documentation templates. These templates allow developers to add custom content, such as tutorials and examples.
API documentation should be easily accessible and maintainable. Azure API Management provides a centralized location for documentation, making it easy for developers to find the information they need.
Azure API Management also supports versioning of API documentation, allowing developers to keep track of changes between versions. This feature is particularly useful when working with multiple versions of an API.
You might enjoy: Azure Aks Versions
Preview and Stable Versions
Preview versions of Azure APIs are identified by a suffix "-preview" in their api-version, for example, 2022-07-07-preview. They include all the features of the most recent stable version and add new preview features.
Preview versions aren't intended for long-term use. They may become unavailable as early as 90 days from the availability of a new stable or preview version. Use preview versions only in situations where you're actively developing against new service features and you're prepared to adopt a new, non-preview version soon after it's released.
Stable versions of Azure APIs generally remain available and supported for many years. They are identified by a date value in YYYY-MM-DD format, called the api-version. Newer versions have later dates.
In most scenarios, a service client will only need to interact with a single version of a service to access all of the functionality it requires. Stable service versions include features that are generally available and supported.
Here are some key differences between preview and stable versions:
Preview versions may introduce breaking changes between versions, whereas stable versions typically do not. If some features from a preview version are released in a new stable version, remaining features still in preview will typically be published in a new preview version.
Frequently Asked Questions
How do I check Azure version?
To check your Azure version, run the command "az --version" in your terminal. Azure Cloud Shell always has the latest version preinstalled.
Sources
- https://www.hakantuncer.com/2018/09/16/api-versioning-with-swagger-azure-api-management-services-and-asp-net-core-a-frictionless-devops-experience/
- https://www.azadvertizer.net/azpolicyadvertizer/549814b6-3212-4203-bdc8-1548d342fb67.html
- https://learn.microsoft.com/en-us/rest/api/azure/
- https://learn.microsoft.com/en-us/azure/developer/intro/azure-service-sdk-tool-versioning
- https://learn.microsoft.com/en-us/rest/api/searchmanagement/management-api-versions
Featured Images: pexels.com