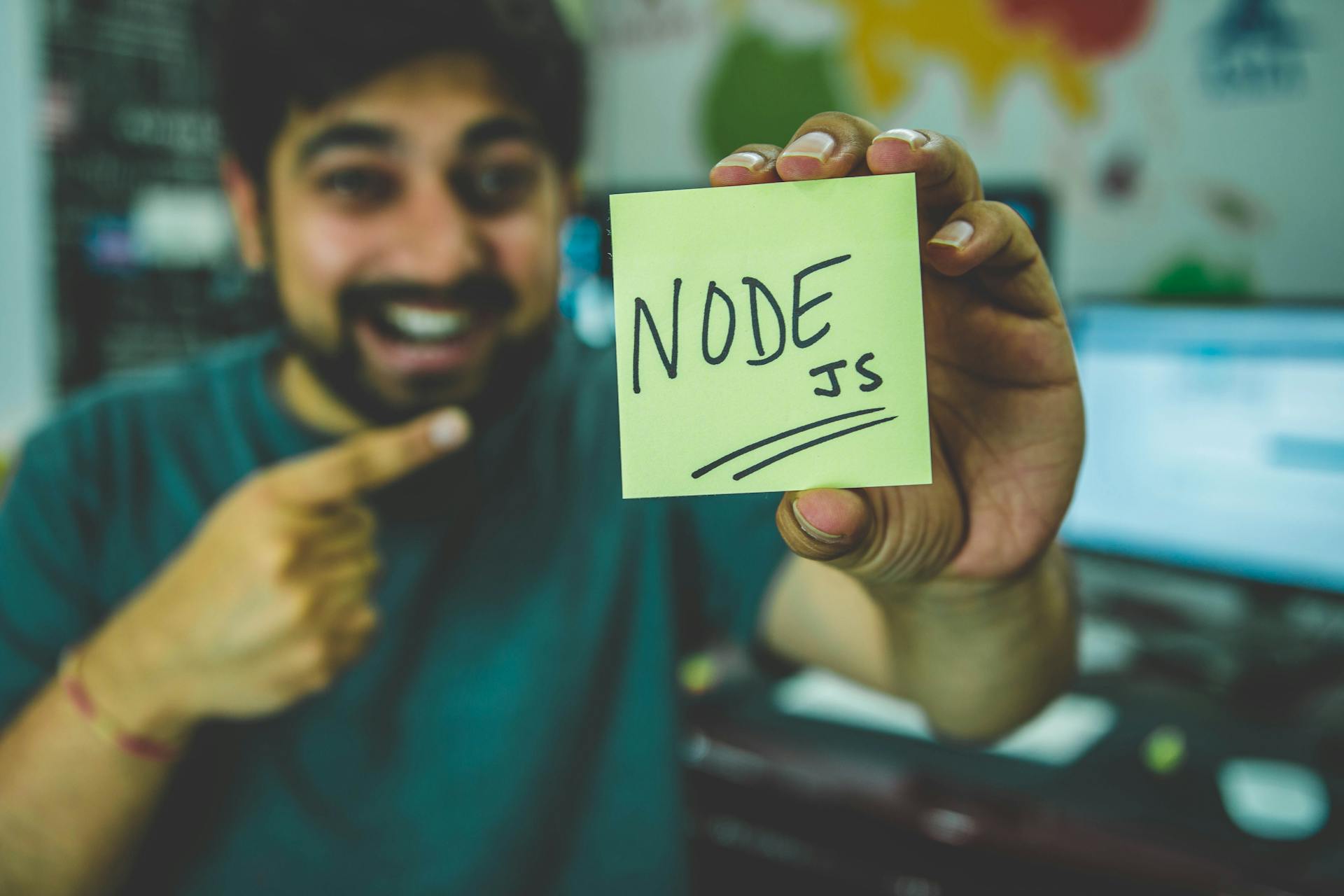
Next Js offers a seamless video streaming experience with automatic setup and storage. This feature is made possible by the Next Js Video component, which allows for easy integration of video streaming into your application.
With Next Js Video, you can upload videos to a server and then display them in your application. This is achieved through the use of a server-side rendered (SSR) approach, which enables fast and efficient video playback.
Next Js Video also supports automatic storage of videos, which means you don't have to worry about managing video files manually. This is a huge time-saver and makes it easier to manage large video libraries.
To get started with Next Js Video, you'll need to install the necessary packages and configure your application to use the video component.
Related reading: Next Js Use Context
Next.js Setup
To set up Next.js for video, you'll need to install next-video as a dependency. This will get you started with video functionality in your project.
The next step is to update your next.config.js file, which is where you'll configure Next.js. This file is crucial in setting up your project's configuration.
Once you've updated your next.config.js file, you'll need to add types for your video file imports if you're using TypeScript. This ensures that your code is properly typed and catches any errors before runtime.
To prepare your project for video, you'll also need to create a /videos directory, where you'll store all your video source files. This is where all your video files will live.
Here's a quick rundown of the steps:
- Install next-video as a dependency
- Update your next.config.js file
- Add types for your video file imports (if using TypeScript)
- Create a /videos directory for your video source files
By following these steps, you'll have your Next.js project set up for video functionality.
Automatic Setup
To set up Next.js automatically, you'll need to install next-video as a dependency. This will get you started with the basics.
First, update your next.config.js file to include the necessary settings. Don't worry if you're not sure what this file does - just make sure to update it according to the instructions.
Explore further: Update Nextjs Version
If you're using TypeScript, you'll also need to add types for your video file imports. This will help catch any errors and make your code more maintainable.
Next, create a /videos directory in your project, where you'll store all your video source files. This is where all your video files will live.
Here's a quick rundown of the steps:
- Install next-video as a dependency
- Update your next.config.js file
- Add types for your video file imports (if using TypeScript)
- Create a /videos directory for your video files
Your .gitignore file will also be updated to ignore video files in the /videos directory. This is a good thing, as videos can take up a lot of space and don't need to be tracked by git.
.js
In Next.js, you can use various components to enhance your application's functionality and user experience.
Video.js is a component that's used for displaying and playing videos once they've been uploaded.
For a seamless user experience, it's essential to show or hide components based on specific conditions, which can be achieved using React's useState and useEffect.
Spinner.js displays a spinner to indicate the upload in progress, making it a crucial component for user engagement.
By leveraging these components and hooks, you can create a more interactive and engaging experience for your users.
See what others are reading: Next Js Components
Storage and Optimization
For storage and optimization, it's recommended to use a dedicated content platform for video due to their large file size and potential for excessive bandwidth usage. This is why Vercel suggests using Mux, a video API for developers.
To get started with Mux, you'll need to sign up, create an access token, and add environment variables to your .env.local file. This will allow you to integrate Mux into your next-video project.
If you want to store asset metadata in a database or elsewhere, you can customize the storage hooks in a separate next-video config file. This will give you more control over how your metadata is stored and retrieved.
Remote Storage Optimization
Remote Storage Optimization is a game-changer for video-heavy websites. You can use a dedicated content platform like Mux to store and optimize your videos, reducing bandwidth usage and improving deliverability.
Mux is a video API for developers, built by the creators of Video.js, and powers popular streaming apps like Patreon. It's used on the largest live events in the world for video performance monitoring.
To get started with Mux, sign up for an account and create an access token, then add environment variables to your .env.local file. This will enable you to use Mux's features, such as compressed streaming and automatic placeholder posters.
If you already have videos hosted remotely, you can import the remote URL and refresh the page to start processing. This will create a local JSON file in the /videos folder and begin uploading the video.
If you don't have the remote video URLs available, you can create a new API endpoint in your Next.js app for /api/video with the following code. This will allow you to set the src attribute to the URL of the remote video, and the video will start processing.
Here's a comparison of the provider feature sets:
Asset Metadata Storage Hooks
Asset metadata storage hooks allow you to customize where your asset metadata is stored. By default, it's stored in a JSON file in the /videos directory.
You can store metadata in a database or elsewhere by customizing the storage hooks in a separate next-video config file. This is done by changing the body of the loadAsset, saveAsset, and updateAsset functions.
The default storage hooks for JSON file storage can be seen in the example config. You can customize these hooks to fit your needs by modifying the functions.
Import the GET and POST handler functions in your API routes as needed. These handlers expect a url query or body parameter with the video source URL.
You would typically add more error handling and validation to the handlers, as well as authentication and authorization. This is not shown in the minimal examples.
Expand your knowledge: Next Js Hooks
ScyllaDB for Low-Latency Applications
ScyllaDB is a low-latency and high-performance NoSQL database that's perfect for video streaming applications. It's compatible with Apache Cassandra and DynamoDB, making it a great choice for large-scale data storage and retrieval.
ScyllaDB has drivers in all the popular programming languages, which means it integrates well with modern web development frameworks like NextJS. This makes it easy to use in real-world applications.
Low latency is crucial for video streaming services, as it delivers a seamless user experience. To achieve this, you need to design a data model that fits your needs.
For example, ScyllaDB is suitable for handling large-scale data storage and retrieval requirements of video streaming applications. It's a great choice for applications that require high performance.
Here are some key benefits of using ScyllaDB for low-latency applications:
- Low latency for seamless user experience
- High-performance NoSQL database
- Compatible with Apache Cassandra and DynamoDB
- Drivers in popular programming languages
Customization
You can add a custom poster and blurDataURL to the video by passing them as props, but this won't provide the same level of optimization as the automatic poster and blurDataURL by the default provider.
To get the same level of optimization, you can use a slotted poster element, which is a good solution but has its limitations. The thumbnailTime query parameter can be used to generate a poster image and blur up image at a specified time in the video, but this is limited to usage with the mux provider.
You can customize the player by passing a custom player component to the as prop, which accepts the following props: asset: The asset that is processed, contains useful asset metadata and upload status.src: A string video source URL if the asset is ready.poster: A string image source URL if the asset is ready.blurDataURL: A string base64 image source URL that can be used as a placeholder.
Additional reading: Next Js Provider
Lazy Loading Named Exported Components
Lazy loading named exported components is a technique that allows you to load components on demand, rather than loading them all at once. This approach is particularly useful when dealing with a large number of components.
To lazy load a named exported component, you need to use the dynamic import with the next/dynamic function. This function returns a promise that resolves to the module.
You can resolve the promise explicitly using the .then() handler function. This is different from lazy loading a default exported component, where you don't need to resolve the promise explicitly.
For example, if you have a component named LazySpike, you would use import("./spike") to get the module, and then pick the exported component by its actual name using the .then() function.
Lazy loading named exported components can be useful when you have a large number of components and you only want to load the ones that are actually needed. This approach can also help improve the performance of your application by reducing the amount of code that needs to be loaded upfront.
On a similar theme: Tutorial Building Useful Nextjs Tool
Custom Poster
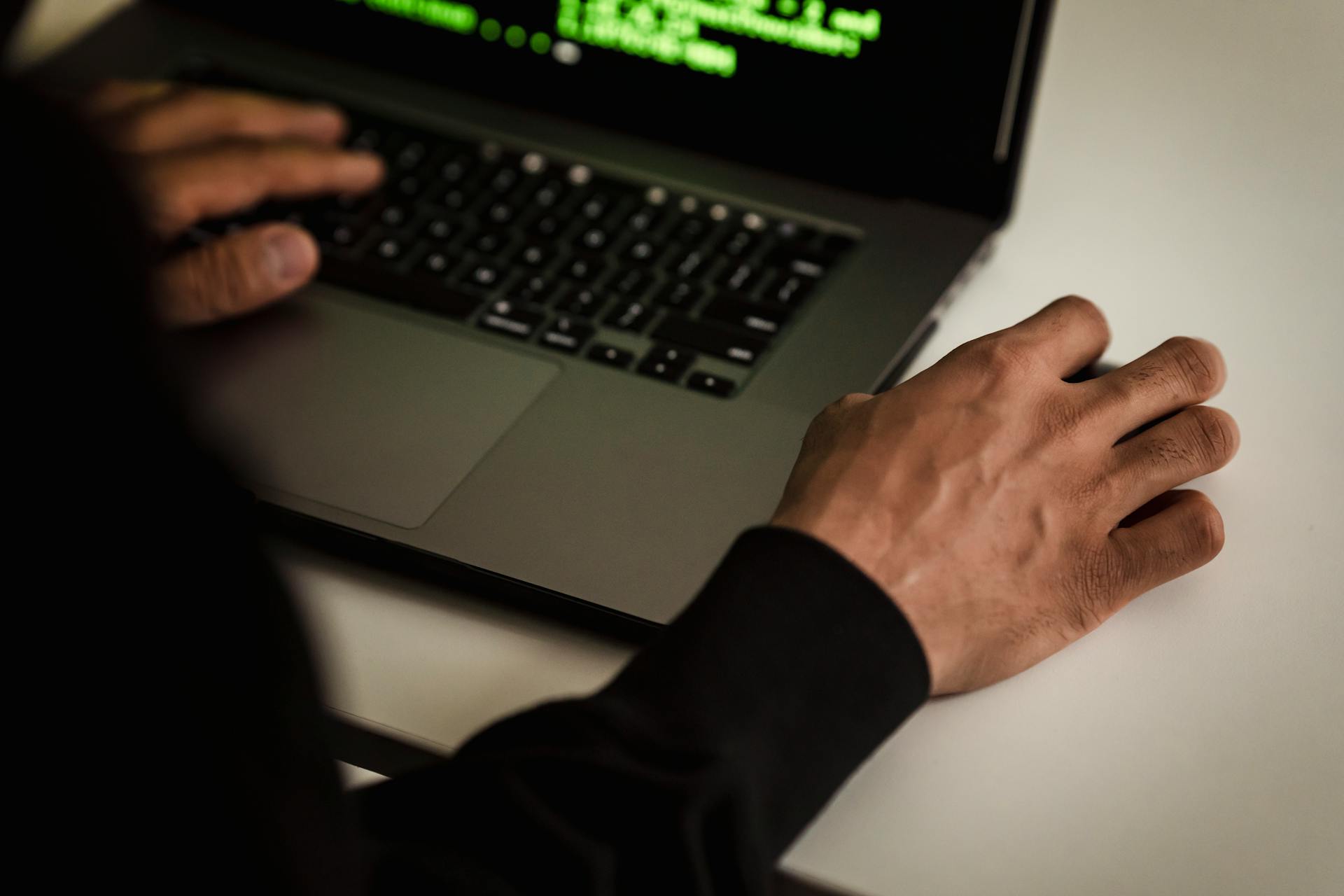
You can add a custom poster to your video by passing it as a prop. This is a good solution, but it won't provide the same level of optimization as the automatic poster and blurDataURL by the default provider.
To get the same level of optimization, you can use a slotted poster element. This approach is particularly useful when you need to generate a poster image and blur up image at a specified time in the video.
If you're using the Mux provider, you can use the thumbnailTime query parameter to achieve this. This parameter is limited to usage with the Mux provider.
A slotted poster image element, like next/image, can be added to the video by passing it as a child with a slot="poster" attribute. This will give your image all the benefits of the used image component and place it nicely behind the video player controls.
Data Management
Data Management is crucial for Next.js video applications. Next.js has built-in support for data fetching, making it easy to manage data.
See what others are reading: Next Js Fetch Data save in Context and Next Route
With Next.js, you can use API Routes to create server-side APIs that fetch data from external sources. This is especially useful for handling large datasets.
API Routes in Next.js allow you to handle requests and responses in a serverless environment. This means you don't have to worry about server maintenance or scaling.
Next.js also provides a built-in caching mechanism to improve performance. This caching mechanism can be configured to store data in memory or on disk.
By using API Routes and caching, you can build high-performance Next.js video applications that handle large datasets efficiently.
Here's an interesting read: Next Js Rest Api
Sources
- https://github.com/muxinc/next-video
- https://tpiros.dev/blog/uploading-and-displaying-videos-with-nextjs/
- https://nextjs.org/docs/app/building-your-application/optimizing/videos
- https://www.freecodecamp.org/news/next-js-performance-optimization/
- https://www.scylladb.com/2024/01/09/build-a-low-latency-video-streaming-app/
Featured Images: pexels.com