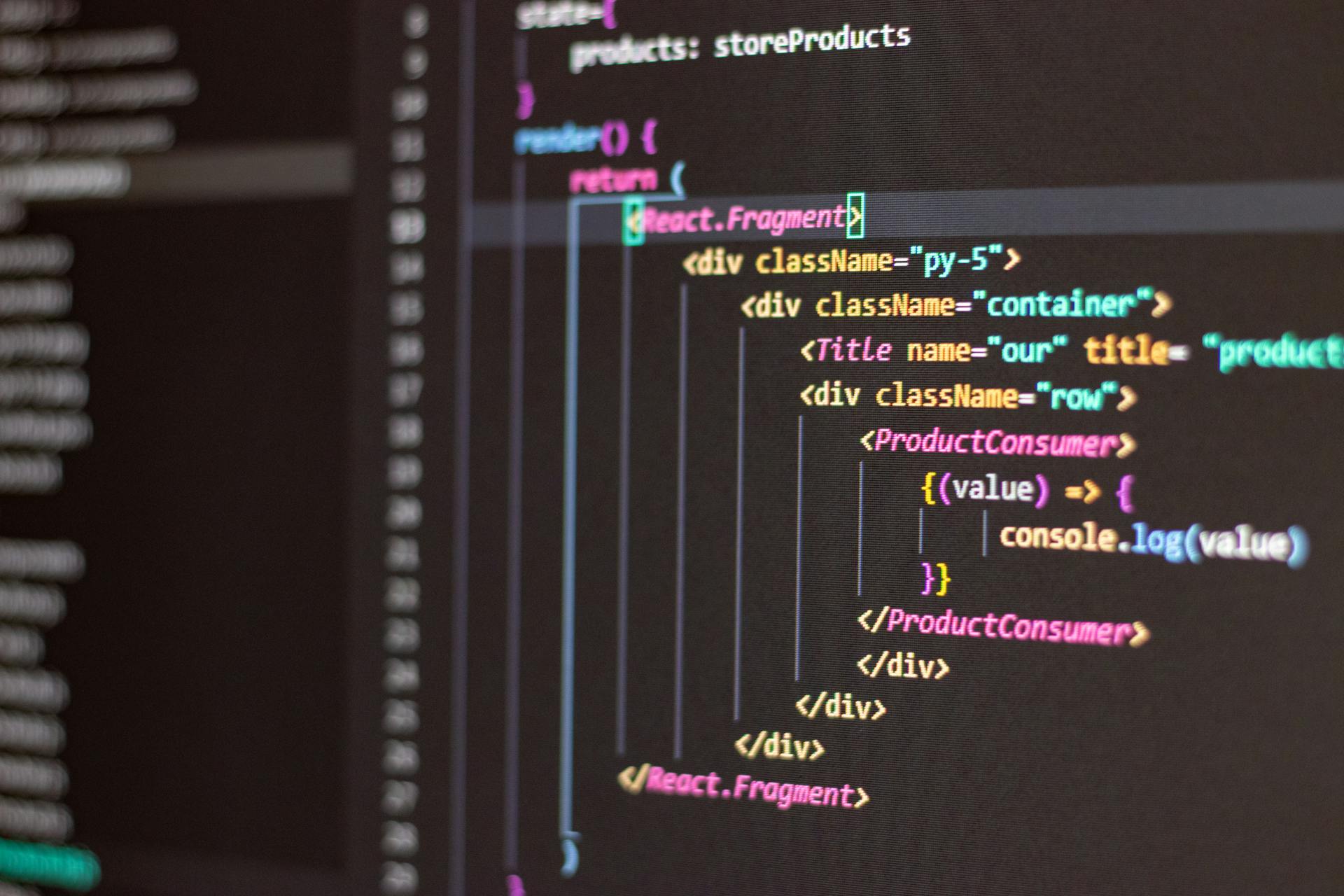
Next.js Styled Components are a powerful tool for building custom user interfaces in your React applications. This guide will walk you through the basics of using Next.js Styled Components.
Styled Components provide a way to write CSS as JavaScript, making it easier to manage complex styles and layouts. By using the `styled` function, you can create reusable, composable styles that can be easily maintained.
To get started with Next.js Styled Components, you'll need to install the `styled-components` package and import it in your Next.js project. This will give you access to the `styled` function and other features of the library.
In the next section, we'll dive deeper into the benefits of using Next.js Styled Components and explore some real-world examples of how to use them effectively.
Intriguing read: Using State in Next Js
Getting Started
To get started with Next.js styled components, you'll need to install the styled-components library and its peer dependency, babel-plugin-styled-components.
First, open your terminal and make sure you're in your Next.js project directory.
If you're using yarn, run `yarn add styled-components babel-plugin-styled-components` to install both packages.
Discover more: Next Js Install
Using Styled Components
Styled-components is a practical choice for Next.js, allowing developers to create components with style automatically injected. It was created for React, making it geared for client-side rendering.
To use styled-components in Next.js, you need to install the library and create a new pages/_document.js file to add the necessary code for server-side rendering. This is easily fixed by creating a ServerStyleSheet and populating the styles to the StyleSheetManager.
Here are some key benefits of using styled-components:
- No separation of content vs. structure because CSS and HTML are combined
- Time needed to learn all the different classes
You can create styled-components using the styled function from the styled-components library, which generates a React component with styles attached to it. This allows you to define and use styled elements within your React components.
You might enjoy: Next Js React Fundamentals
Using CSS Modules
Using CSS modules is a great way to keep your styles organized and efficient. You can create files for style-specific components, and they're easy to use with no setup required.
CSS modules are simple CSS files with the module.css extension, making them easy to identify. For example, a Home.module.css file can contain styles for a specific component.
A fresh viewpoint: Title of Specific Page Nextjs
In your components, you can import your stylesheet and use it, as shown in the example in pages/index.js. This approach allows you to isolate your CSS and make it easier to maintain.
One advantage of using CSS modules is that they can be used in addition to a global stylesheet, giving you more flexibility.
A fresh viewpoint: Apply Css Nextjs
The Plugin
Styled-components provides a Babel plugin for adding SSR support. To use it, install it using the command `npm install babel-plugin-styled-components`.
You can create a custom configuration file, such as `.babelrc`, and add the styled-components plugin to it.
Here are the steps to configure Babel with the styled-components plugin:
- Create or open the `.babelrc` file in the root directory of your project.
- Add the following to the file:
```json
{
"plugins": ["styled-components"]
}
```
Alternatively, you can use the styled-components SWC plugin, which can be configured by creating a `.swcrc` file in the root of your project and adding the following:
```json
{
"plugins": ["styled-components/swc"]
}
```
Note that the styled-components SWC plugin is an alternative to the Babel plugin and provides similar functionality.
Worth a look: Nextjs Swc
Theming and Styling
You can use themed-components to define a set of global styles that can be applied consistently across your application.
Theming in styled-components allows you to define a set of global styles that can be applied consistently across your application, making it easy to manage and switch between different themes.
To set up a theme, you first need to create a theme file where you can define your theme properties such as colors, fonts, and other design tokens. This is done by creating a file named theme.js (or any other name you prefer) in a suitable directory.
To use the theme properties within your styled-components, you can access the theme object from props. This ensures that the Button component adheres to the theme defined in the theme.js file.
Here are some ways to access theme properties within styled-components:
By using the ThemeProvider component provided by styled-components, you can make the theme available to all styled-components in your application. This is done by importing the necessary modules, wrapping your application with ThemeProvider, and passing your theme as a prop.
For more insights, see: Themeprovider Nextjs
Theming
Theming is a great way to maintain a unified look and feel across your application. This is particularly useful for managing and switching between different themes.
To set up a theme, you first need to create a theme file where you can define your theme properties such as colors, fonts, and other design tokens. This file is typically named theme.js and is placed in a suitable directory like a styles or theme folder.
You can define various properties for your theme in the theme.js file, such as color, font, and font size properties. Here's an example of what this might look like:
To use the theme properties within your styled-components, you can access the theme object from props. This ensures that the styled-components adhere to the theme defined in the theme.js file.
Theming also allows you to access global variables like colors, font sizes, or other design tokens across multiple components. You can use a theme to do this by creating a theme file and importing it into your components.
Expand your knowledge: Next Js Folder Structure
Using the ThemeProvider component provided by styled-components makes the theme available to all styled-components in your application. This is typically done by wrapping your application's root component with ThemeProvider and passing your theme as a prop.
By using theming, you can create a consistent look and feel across your application, making it easier to manage and switch between different themes.
A unique perspective: Next Js Single Page Application
Add Ssr Support
Adding SSR support to styled-components with Next.js can be a bit tricky, but don't worry, I've got you covered. The main issue is that styled-components was made for React, meaning it's geared for client-side rendering, not server-side rendering. This means that styles won't be applied on the server, resulting in a visible delay before the styles are applied on the client.
To fix this, you can create a new pages/_document.js file and add the necessary code to support server-side rendering. This is easily done by adding the following code to the file:
Check this out: File Upload Next Js Supabase
However, there are multiple ways to add SSR support to styled-components with Next.js, and this is just one of them. Another option is to use a different library, but if you're already invested in styled-components, it's worth exploring the other options first.
Here are some of the ways to add SSR support to styled-components with Next.js:
- Create a new pages/_document.js file and add the necessary code to support server-side rendering.
- Use a different library, but if you're already invested in styled-components, it's worth exploring the other options first.
In this case, creating a new pages/_document.js file is a simple and effective solution to add SSR support to styled-components with Next.js.
Sources
- https://blog.logrocket.com/best-styling-options-nextjs/
- https://www.dhiwise.com/post/step-by-step-guide-to-nextjs-styled-components
- https://medium.com/@felipe.nava/using-styled-components-in-nextjs-app-router-35295688e27a
- https://dev.to/rashidshamloo/using-styled-components-with-nextjs-v13-typescript-2l6m
- https://medium.com/@kapaak/next-js-styled-components-setup-9cc98b3df2a1
Featured Images: pexels.com