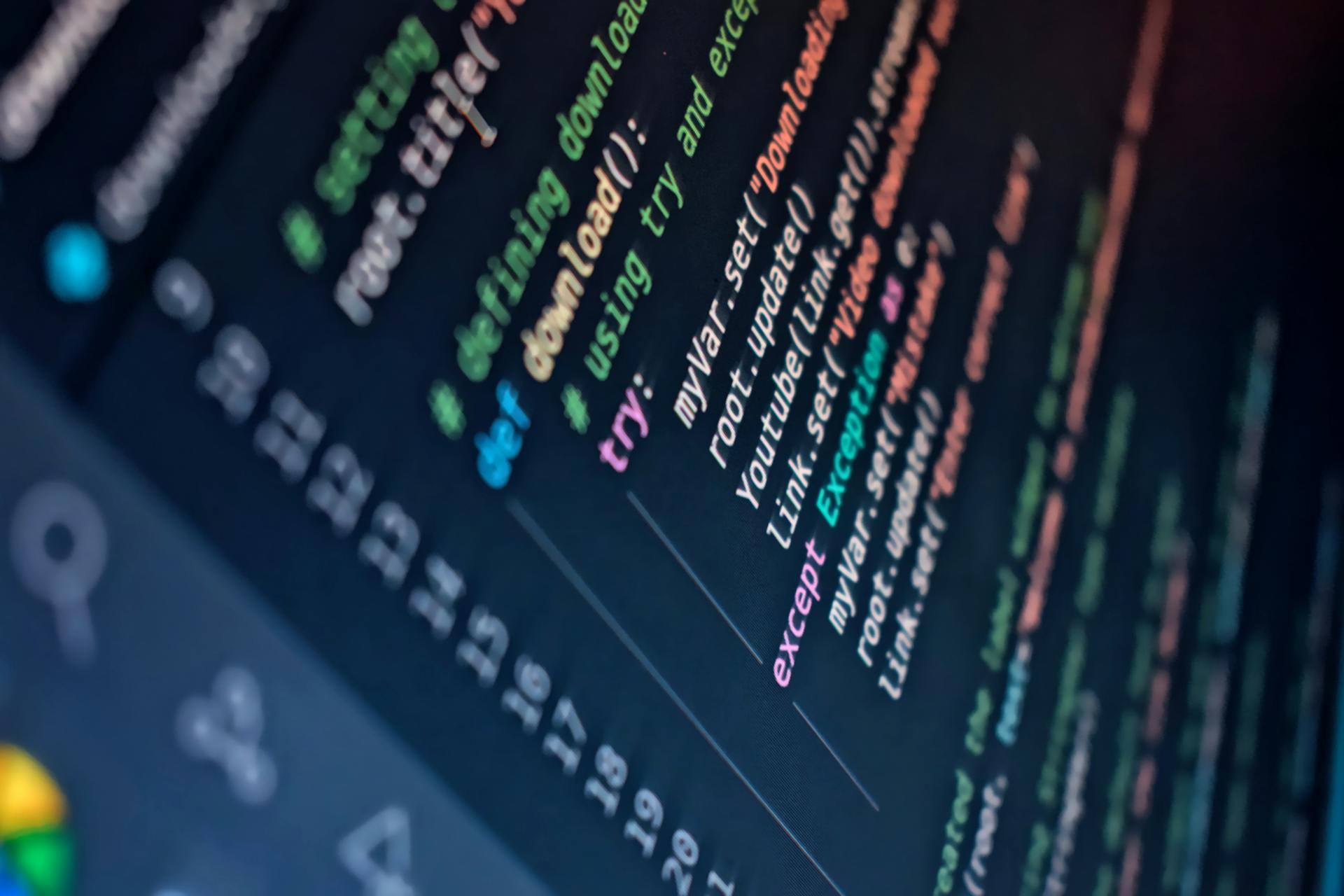
Next JS Loading Initial Props and Props is a crucial concept for developers to grasp, especially when building server-side rendered (SSR) applications.
In Next JS, initial props are the data passed to a page component during the initial render. This data is typically fetched from an API or a database.
The key difference between initial props and props is that initial props are only used during the initial render, while props are used throughout the component's lifecycle.
Initial props are a convenient way to pre-populate page components with data, but they can also lead to performance issues if not used carefully.
What Is Next.js?
Next.js is an open-source React-based framework for building server-side rendered (SSR) and statically generated websites and applications. It was created by Guillermo Rauch and was first released in 2016.
Next.js allows developers to create server-side rendered (SSR) applications, which means that the initial HTML of a page is generated on the server rather than in the browser. This can improve SEO and provide a faster user experience.
If this caught your attention, see: Nextjs Ssr Websocket
Next.js is built on top of React and provides a set of tools and features that make it easy to build fast, scalable, and maintainable applications. It includes features like automatic code splitting, server-side rendering, and static site generation.
Next.js supports a range of features that make it a popular choice for building fast and scalable applications. These features include automatic code splitting, server-side rendering, and static site generation.
Additional reading: Next Js Component Rendering Analyzer
Getting Initial Props
getInitialProps will run on the server during the initial page load and, subsequently, run in the browser if you make client-side transition to other parts of the application.
Using getInitialProps for data fetching and SSR in Next.js is outdated and deprecated because its behavior can be confusing for beginners and could also lead to unintentional bugs and errors if used the wrong way.
getInitialProps and getServerSideProps are both used in fetching data for server-side rendering in Next.js, but they have different behaviors and use cases.
Explore further: Next Js Build Collecting Page Data
Both functions share similarities, such as being used in page components and not in children components.
However, getInitialProps will run on the server and in the browser, whereas getServerSideProps will only run on the server.
This difference in behavior makes getServerSideProps a more reliable choice for data fetching and SSR in Next.js.
Take a look at this: Next Js Component
GetInitialProps in Next.js
GetInitialProps in Next.js is an asynchronous function used for fetching data on the server and pre-rendering the resulting data in Next.js page components.
This function runs only on the server at the initial page load, but if you make client-side navigation to other parts of your application and come back to the page, the function will then run in the browser.
Any data you are fetching in getInitialProps is returned as an object and is used by Next.js to populate the props parameter in the default export in a page component.
You can use getInitialProps in a page component, as shown in the example below.
There are specific scenarios where you might still need to use getInitialProps, including:
- Complex data fetching logic: If your data fetching logic is complex and can’t be easily achieved with getStaticProps or getServerSideProps, you might continue using getInitialProps.
- Custom SSR logic: If you need custom server-side rendering logic that can’t be achieved with the newer methods, you might choose to use getInitialProps.
- Legacy projects: If you’re working on a legacy project that was built using older versions of Next.js and heavily relies on getInitialProps, it might be more practical to continue using it rather than rewriting everything.
Transitioning to Static Site Generation
Static Site Generation (SSG) is a game-changer for Next.js applications, offering faster page loads and reduced server load. It's ideal for pages with content that doesn't change frequently.
With SSG, Next.js pre-renders pages at build time, generating static HTML files with fetched data. This approach is particularly useful for blog posts, where content won't change often.
To migrate to SSG, you need to remove getInitialProps and replace it with getStaticProps in your page components. The structure of getStaticProps is different from getInitialProps, and it returns an object with the props key containing the fetched data.
You can also include the revalidate option in the returned object to specify how often the data should be revalidated and regenerated. This is useful when you want to update the data periodically without redeploying your application.
Take a look at this: Getstaticprops Next Js
Here's a brief comparison of getServerSideProps and getStaticProps:
By using getStaticProps, you can take advantage of SSG and improve the performance of your Next.js application.
Server-Side Rendering and Props
getServerSideProps will always run on the server, unlike getInitialProps which can run on both the server and the browser. This means you can use server-side only code, like the fs module, to load files to pass as props.
You can use getServerSideProps to fetch data from APIs, similar to getInitialProps. However, getServerSideProps is guaranteed to run on the server, giving you greater control over where the code is ran.
Here are the key differences between getServerSideProps and getInitialProps:
- getServerSideProps will always run on the server, whereas getInitialProps can run on both the server and the browser.
- getServerSideProps can use server-side only code, like the fs module, whereas getInitialProps cannot.
Context Parameter
The context parameter is a crucial part of server-side rendering, and it's essential to understand what it entails. It's an object containing several keys that provide valuable information about the request.
The req key is an instance of the HTTP request object, which is only available when getInitialProps runs on the server. This is a significant point, as it highlights the importance of server-side rendering.
The res key is also an instance of the HTTP response object, available only when getInitialProps runs on the server. This object is critical for responding to the client's request.
The pathname key returns the current route, which is particularly useful for navigating between pages. For example, if you have a file pages/example.js, pathname will return /example.
The query key parses the query string as an object if the URL contains query parameters. This is a convenient way to access query parameters without having to manually parse the URL.
The aspath key returns a string of the actual path, including the query, as shown in the browser. This is a useful tool for debugging and testing purposes.
The err key contains an error object if any error is encountered during rendering. This is a critical aspect of server-side rendering, as it allows you to handle and display errors to the user.
Here's a summary of the context parameter keys:
- req: HTTP request object (server-side only)
- res: HTTP response object (server-side only)
- pathname: Current route
- query: Query string parsed as an object
- aspath: Actual path including query (browser view)
- err: Error object (if any error is encountered)
Optimizing for Performance
getServerSideProps runs on every request, which can slow down your site if you're doing a lot of data fetching or computations.
One way to optimize getServerSideProps is to return the required props object with the props property. This helps reduce the number of repetitive calls by memoizing the request and reusing the result for subsequent calls.
Returning the required props object can significantly improve performance by avoiding multiple requests to the server for the same data.
Smarter caching is another way to optimize getServerSideProps. It caches the results of your request based on the content that was requested.
This means that if a user requests a specific page, Next.js will only cache the data for that page, saving time and resources.
Smarter caching also allows for "incremental updates", where only the affected parts of the site will be updated in the cache if you make a small change to your code.
Intriguing read: Next Js Typescript Example Props
Server Side Props
getServerSideProps is a function in Next.js that runs on the server and is used for server-side rendering. It will always run on the server, even if you make client-side navigation or refresh the page.
Unlike getInitialProps, getServerSideProps is not deprecated and is the recommended way to do data fetching and SSR in Next.js. This is because it gives you greater control over where the code is run.
The context parameter is an object that contains the following keys: req, res, pathname, query, aspath, and err. The req and res objects are only available when getServerSideProps runs on the server.
Here are the details of the context parameter:
getServerSideProps runs on every request, which means it can slow down your site if you're doing a lot of data fetching or computations. To optimize its use, make sure to return the required props object with the props property. This will help reduce the number of repetitive calls and improve performance.
On a similar theme: Next Js Props
Per-Route State
Per-route state can be a challenge when using server-side rendering with Redux. This is because the store is preserved across route changes, which can lead to issues if you're using it for per-route data.
Curious to learn more? Check out: Nextjs App Router Tailwind Spinner Loading Page
If you're using Next.js's support for client-side SPA-style navigation, only the route component will be re-rendered when customers navigate from page to page. This means the store will be preserved, but that's not a problem if you're only using it for global, mutable data.
However, if you're using the store for per-route data, you'll need to reset the route-specific data in the store when the route changes. This is because the store is initialized on any route change to the product detail route.
You can use the same initialization pattern as before, of dispatching actions to the store, to set the route-specific data. The initialized ref is used to ensure that the store is only initialized once per route change.
Initializing the store with a useEffect would not work because useEffect only runs on the client. This would result in hydration errors or flicker because the result from a server-side render would not match the result from the client-side render.
For more insights, see: Next Js Caching
Checking Your Work
You've set up Redux Toolkit, but how do you know it's working correctly? The key lies in checking three crucial areas.
Server-side rendering is one of them. You need to ensure the data in the Redux store is present in the server-side rendered output. This might sound obvious, but it's easy to overlook.
Route changes are another area to focus on. Navigate between pages on the same route as well as between different routes to guarantee that route-specific data is initialized properly.
Mutations are the final piece of the puzzle. You should check that the store is compatible with the Next.js App Router caches by performing a mutation and then navigating away from the route and back to the original route to ensure that the data is updated.
Here are the three key areas to check in more detail:
- Server-side rendering
- Route changes
- Mutations
By checking these areas, you'll be confident that your Redux Toolkit setup is working as expected.
Loading Initial Props
getServerSideProps is the way to go for loading initial props in Next.js, as it always runs on the server and gives you greater control over where the code is ran.
Unlike getInitialProps, which is being deprecated, getServerSideProps is guaranteed to run on the server and can even use server-side only code, like the fs module to load files to pass as props.
You can use getServerSideProps to fetch a list of users from the JSONPlaceholder API for server-side rendering, and it will never run in the browser, even if you make client-side navigation or refresh the page.
Writing server-side specific code in getServerSideProps will work perfectly, but you'll run into errors if you try the same thing in getInitialProps.
Using getServerSideProps for data fetching and SSR in Next.js is the recommended approach, as it avoids unintentional bugs and errors that can occur with getInitialProps.
A unique perspective: Getserversideprops Nextjs
Sources
- https://refine.dev/blog/next-js-getinitialprops-and-getserversideprops/
- https://blog.logrocket.com/data-fetching-next-js-getserversideprops-getstaticprops/
- https://upmostly.com/nextjs/getserversideprops-vs-getinitialprops-getstaticprops-in-next-js
- https://egghead.io/lessons/react-leverage-next-js-getinitialprops-lifecycle-hook-to-load-individual-posts
- https://redux.js.org/usage/nextjs
Featured Images: pexels.com