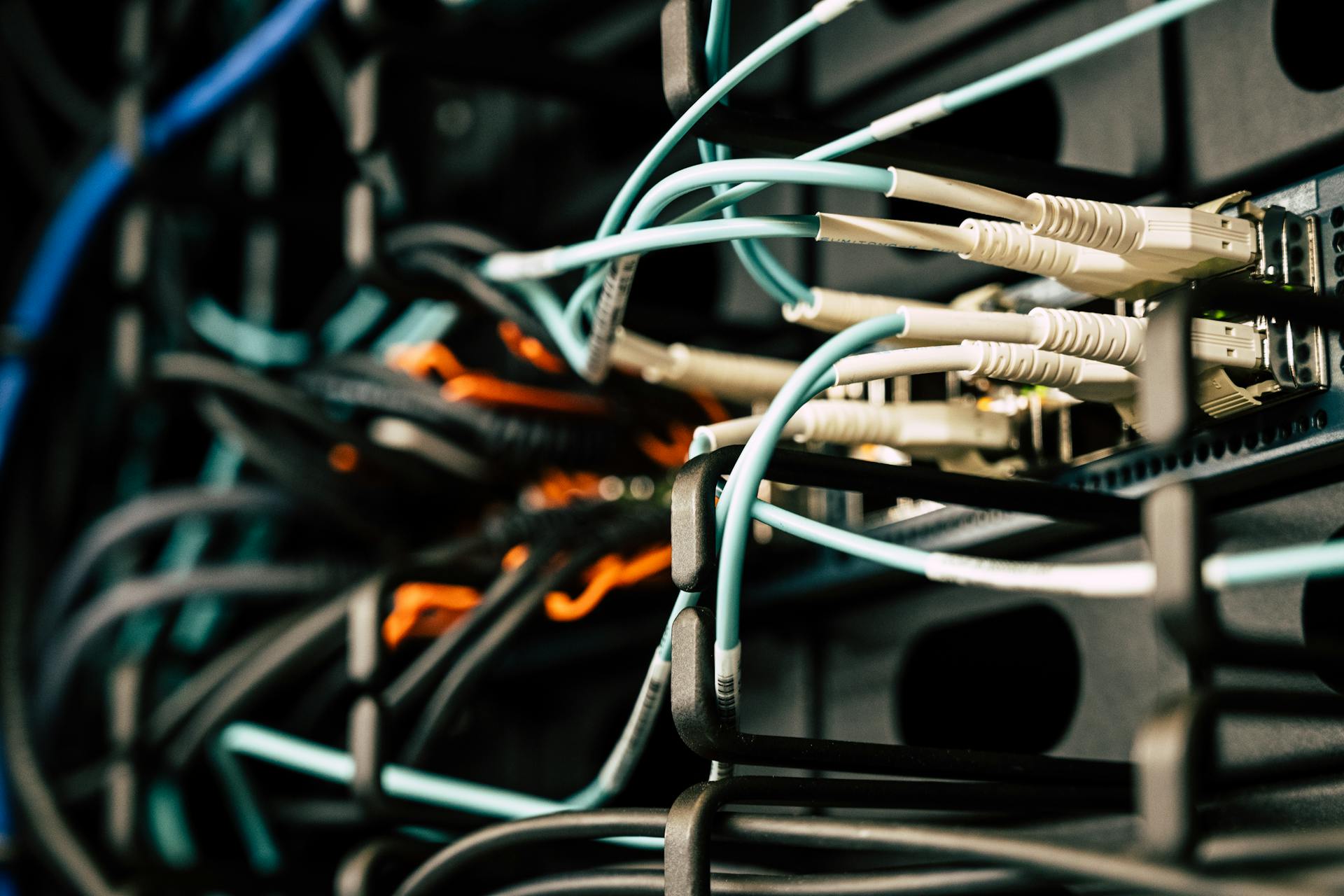
Real-time applications are all about providing an immersive experience for users. They allow for instant updates and interactions, making them ideal for applications like live chat, gaming, and news feeds.
WebSockets enable real-time communication between a client and a server by establishing a persistent connection. This allows for bi-directional communication, making it possible for the server to push updates to the client.
With Next.js, you can easily integrate WebSockets into your application using the `ws` library. This library provides a simple and efficient way to handle WebSocket connections.
Real-time applications can be complex and challenging to implement, but with the right tools and knowledge, you can create seamless and engaging experiences for your users.
What Are?
WebSockets are a communication protocol that enables two-way, full-duplex communication channels over a single, long-lived connection.
Unlike traditional HTTP, WebSockets allow for constant data exchange between a client and a server without the overhead of initiating multiple new connections. This persistent connection makes WebSockets ideal for real-time applications like chat applications, online gaming, and live data updates.
The main features of Next.js WebSockets include:
- Bi-directional Communication: WebSockets facilitate real-time, two-way communication, allowing the client and server to send and receive data anytime.
- Low Latency: The connection remains open, eliminating the need to establish relationships, reducing latency, and making real-time communication smoother repeatedly.
- Efficiency: WebSockets are efficient in data transfer, as they don't require the overhead of HTTP headers for every exchange.
- Real-time Updates: They are the backbone of modern applications that need to deliver real-time updates to users.
By using WebSockets, developers can create seamless real-time communication in their Next.js applications, making it perfect for applications that require instant updates.
Setting Up a Next.js Project
To set up a Next.js project, you'll need to have Node.js installed on your computer.
First, you'll initialize the project by running the command `npx create-next-app@latest real-time-app` in your terminal. This will create a new Next.js project in a directory called `real-time-app`.
Next, navigate into the project directory by running `cd real-time-app` in your terminal.
To set up the project for real-time updates, you'll need to install the `ws` library, which is a WebSocket library for Node.js. You can do this by running `npm install ws` in your terminal.
By following these steps, you'll have a basic Next.js project set up and ready to use with WebSockets.
Create a Server
To create a server in a Next.js application, you'll need to set up a custom server. This can be done by creating a file named server.js in the root of your project directory.
You can create a custom server by importing the required packages, including http, url, next, and WebSocket. This is shown in the code snippet below:
```javascript
const { createServer } = require('http');
const { parse } = require('url');
const next = require('next');
const WebSocket = require('ws');
```
In the server.js file, you'll also need to create an instance of the Server class from the WebSocket package. This is done by creating a new WebSocket server, as shown in the code snippet below:
```javascript
const wss = new WebSocket.Server({ server });
```
Once you've created the server, you can configure it to listen for incoming connections. This can be done by using the on('connection') event, which is triggered when a new client connects to the server.
Here's an example of how to configure the server to listen for incoming connections:
```javascript
wss.on('connection', (ws) => {
console.log('New client connected');
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
ws.send(`Server: ${message}`);
});
ws.on('close', () => {
console.log('Client disconnected');
});
});
```
To start the server, you'll need to create a new instance of the createServer function from the http package. This is shown in the code snippet below:
```javascript
const server = createServer((req, res) => {
const parsedUrl = parse(req.url, true);
handle(req, res, parsedUrl);
});
```
Once you've created the server, you can start it by listening on a specific port, such as port 3000. This is shown in the code snippet below:
```javascript
server.listen(3000, (err) => {
if (err) throw err;
console.log('> Ready on http://localhost:3000');
});
```
To update your project to use the custom server, you'll need to modify the scripts section in your package.json file. This can be done by adding the following lines of code:
```javascript
"scripts": {
"dev": "node server.js",
"build": "next build",
"start": "NODE_ENV=production node server.js"
}
```
This will allow you to start the server by running the command `npm run dev` or `npm start` in your terminal.
Integrating Client-Side
Integrating client-side WebSockets in a Next.js application is a straightforward process that can be achieved using the WebSocket API provided by modern web browsers. To get started, you'll need to create a Next.js WebSocket instance and add event listeners for open, message, close, and error events.
The client-side integration involves creating a WebSocket Hook, which can be done by importing the necessary dependencies, such as `useEffect` and `useState` from React. The `useWebSocket` hook can then be created, which takes a `url` parameter and returns an object with `messages` and `sendMessage` properties.
Here's a high-level overview of the steps involved in client-side integration:
By following these steps, you can integrate client-side WebSockets in your Next.js application and enable real-time communication between the client and server.
Client-Side Integration
Client-side integration is where the magic happens, and you get to see your WebSocket application come to life. This is where you'll use the WebSocket API provided by most modern web browsers to create a Next.js WebSocket instance and add event listeners for open, message, close, and error events.
You can use the message event to handle data received from the server and the send method to send data to the server. For example, in Next.js, you can create a WebSocket Hook using the WebSocket API to establish a connection with the server.
Here's a step-by-step guide to creating a WebSocket Hook:
- Create a WebSocket Hook: In the hooks directory, create a file named useWebSocket.js.
- Use the WebSocket Hook in a Component: Create a pages/index.js file to use the WebSocket hook.
By following these steps, you can set up a real-time chat application that allows users to send and receive messages in real-time. This is just one example of how you can use client-side integration to create a WebSocket application.
TRPC Client Configuration
You have two main options for client configuration in tRPC: createTRPCNext or createTRPCProxyClient. The higher-level client, createTRPCNext, integrates with TanStack React Query hooks for a more streamlined developer experience.
This implementation simplifies data fetching and management, making it easier to build reactive user interfaces. The lower-level client, createTRPCProxyClient, provides direct calls to the server but lacks additional features.
Both clients need to be configured with ending links, which can be either the default httpBatchLink for traditional HTTP communication or a wsLink for WebSocket communication. Using both clients will result in separate WebSocket connections throughout the application's lifetime.
The higher-level api client leverages React Query hooks for efficient data management, while the lower-level trpcClient offers a more direct connection to the server. Maintaining multiple WebSocket connections can introduce additional overhead and complexity.
It's essential to carefully evaluate the trade-offs and only implement both clients if the application's requirements truly justify the added complexity.
Real-Time Updates and Chat Applications
Real-time updates are a crucial aspect of modern web applications, and Next.js WebSockets make it easy to implement. With Next.js WebSockets, you can create real-time features in your Next.js application, such as a chat application where messages are instantly delivered to all connected clients.
Next.js WebSockets provide low-latency communication, making them ideal for applications that require real-time updates. This means that your users can expect instant data transmission without the overhead of establishing multiple HTTP connections.
To broadcast chat messages to connected clients, you need to emit events from the WebSocket server. You can create a new function to handle incoming chat messages and emit an event to all connected clients using io.emit. This is a key feature of Next.js WebSockets that enables real-time communication.
Here are the benefits of using WebSockets:
- Low Latency: Instant data transmission without the overhead of establishing multiple HTTP connections.
- Efficient: Reduced bandwidth usage by maintaining a single connection.
- Real-Time Communication: Ideal for applications requiring live updates, such as chat applications, real-time dashboards, and collaborative tools.
Real-World Applications
Real-world applications of Next.js WebSockets are vast and exciting. With instant message delivery and read receipts, chat applications can be powered with ease.
Next.js WebSockets are perfect for chat applications, whether for business or personal use. They provide the real-time messaging that's essential for these types of applications.
Real-time updates are a game-changer in various industries. For instance, chat applications can be used in customer support, where instant responses are crucial.
Low latency and efficient data transmission make Next.js WebSockets ideal for applications requiring live updates. This is especially true for chat applications, real-time dashboards, and collaborative tools.
Here are some benefits of using WebSockets:
- Low Latency: Instant data transmission without the overhead of establishing multiple HTTP connections.
- Efficient: Reduced bandwidth usage by maintaining a single connection.
- Real-Time Communication: Ideal for applications requiring live updates, such as chat applications, real-time dashboards, and collaborative tools.
Real-Time Updates
Real-time updates are a crucial aspect of chat applications, allowing users to receive instant messages and stay connected with others. With Next.js WebSockets, you can implement real-time features in your application, making it ideal for chat applications.
Low-latency communication is provided by Next.js WebSockets, making them perfect for applications that require real-time updates. This means that messages are delivered instantly to all connected clients.
To broadcast chat messages to connected clients, you need to emit events from the WebSocket server. Create a new function to handle incoming chat messages, which takes a message parameter and emits an event to all connected clients using io.emit.
You can use the useState hook to store the messages and the current input value in your React component. When a new message is received from the server, you can update the messages state.
Here are the benefits of using WebSockets:
- Low Latency: Instant data transmission without the overhead of establishing multiple HTTP connections.
- Efficient: Reduced bandwidth usage by maintaining a single connection.
- Real-Time Communication: Ideal for applications requiring live updates, such as chat applications, real-time dashboards, and collaborative tools.
Libraries and Tools
When choosing a WebSocket library, select one that suits your needs. Some popular options include Socket.io and ws.
For this guide, we'll use the ws library, a simple and efficient WebSocket implementation for Node.js. You can install it with npm.
To enable real-time communication in your Next.js app, install the necessary WebSocket libraries. You'll need the socket.io-client and socket.io packages.
Here's a list of the required packages and installation commands:
With your Next.js project set up and the necessary WebSocket libraries installed, you're now ready to start building your real-time chat application.
Choose a Library
When choosing a WebSocket library, it's essential to select one that aligns with your project requirements. Consider the features and capabilities of each library to make an informed decision.
Socket.io and ws are two popular options. The ws library is a simple and efficient WebSocket implementation for Node.js, which is why it's used in this guide. You can install it with npm.
For real-time communication in your Next.js app, socket.io is a robust and easy-to-use WebSocket implementation. It's composed of two packages: socket.io-client and socket.io.
Here's a brief overview of the packages you'll need:
These packages will help you establish a WebSocket connection and handle real-time events on both the client-side and server-side.
TRPC
tRPC is a fantastic library that allows you to create typesafe subscriptions and WebSocket communication in your Next.js application.
It integrates seamlessly with WebSockets, which were previously implemented with a custom server, but now you can create WebSocket endpoints directly within the Next.js app router.
With tRPC, you can reuse the WebSocket connection provided by the next-ws package, eliminating the need for a custom server and keeping your application optimized and resource-efficient.
The tRPC package defines the applyWSSHandler function, which wires up the incoming WebSocketServer and creates a connection to the client.
You can define tRPC subscriptions on the server-side using the publicProcedure and .subscription methods from tRPC, which return an observable that maintains a stateful connection until it is closed.
To subscribe to a subscription endpoint from the client-side, you can use the useSubscription hook provided by tRPC, which allows you to receive data in real-time.
The useSubscription hook takes the input for the subscription and two callback functions: onData and onError, which are invoked when data is received or an error occurs during the subscription.
You can validate the input for a subscription using zod, ensuring that the correct data type is provided.
Sources
- https://kapsys.io/user-experience/real-time-updates-integrating-next-js-web-sockets-on-vercel
- https://nextjsstarter.com/blog/build-nextjs-real-time-chat-app-in-5-steps/
- https://www.pedroalonso.net/blog/websockets-nextjs-part-3/
- https://numvio.com/blog/software-dev/Practical-Implementation-of-WebSockets-with-Next.js-and-tRPC
- https://blog.bytescrum.com/real-time-applications-with-nextjs-and-websockets
Featured Images: pexels.com