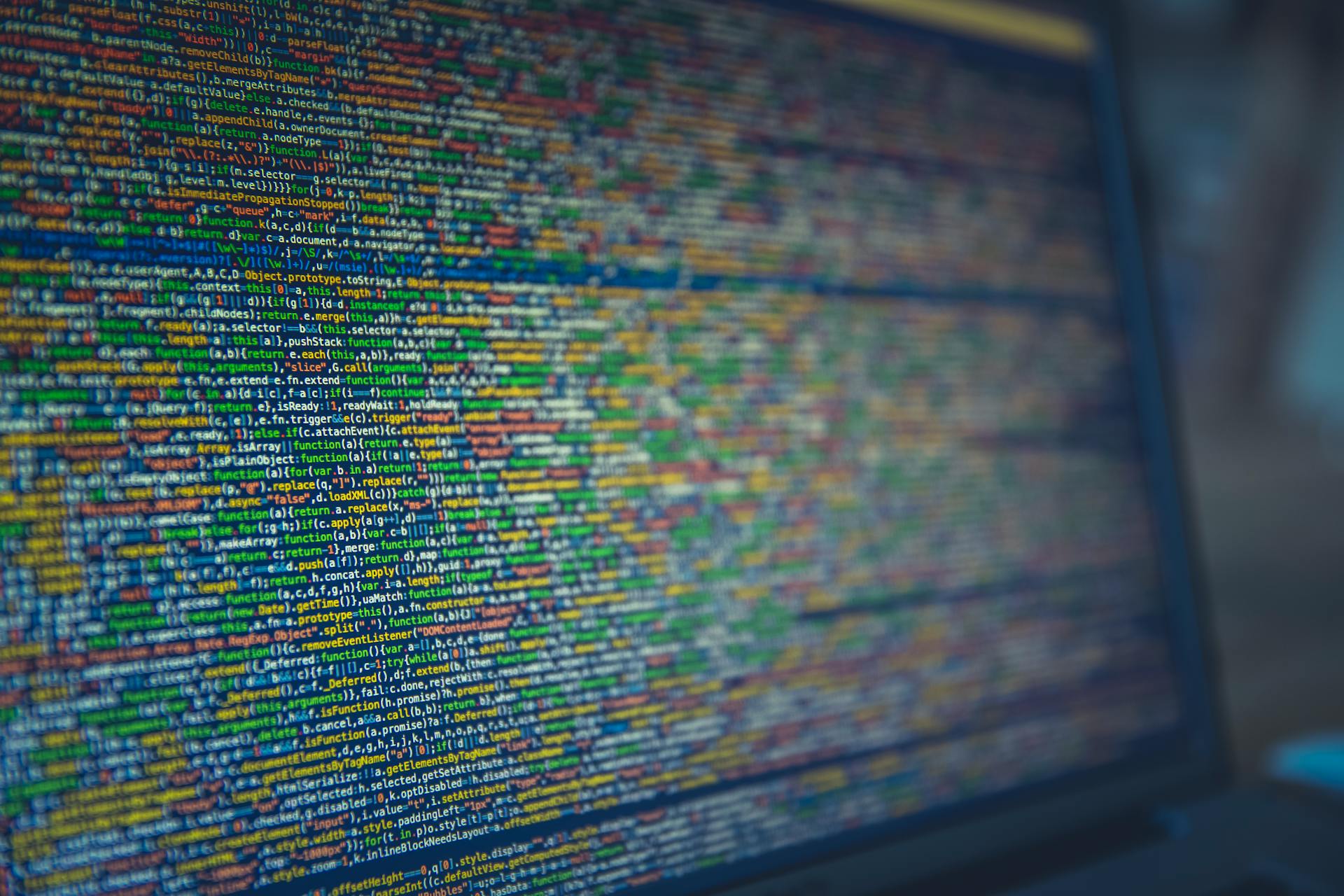
Building a Todo App with Next.js 13 and Prisma ORM is a great way to get started with the Prisma Nextjs stack. Next.js 13 provides a robust framework for building server-side rendered (SSR) and statically generated websites and applications.
To create a Todo App, you'll need to set up a new Next.js project and install Prisma. Prisma is an ORM (Object-Relational Mapping) tool that allows you to interact with your database using a more intuitive and efficient way.
In this guide, we'll walk through the process of building a Todo App using Next.js 13 and Prisma ORM. We'll cover setting up the project, creating a database, and implementing CRUD (Create, Read, Update, Delete) operations. By the end of this guide, you'll have a fully functional Todo App that showcases the power of Prisma Nextjs.
Recommended read: Nextjs Orm
Set Up
Setting up Prisma and Next.js is a crucial step in building a robust and scalable application. You'll need to install the Prisma CLI as a development dependency in your project.
Broaden your view: Nextjs Prisma Docker
To initialize Prisma, run `npx prisma init` in your terminal. This command creates a new directory called `prisma` that contains a file called `schema.prisma`, which contains your Prisma schema with your database connection variable and schema models. It also creates the `.env` file in the root directory of your project, which is used for defining environment variables.
The Prisma schema file should look like this:
```prisma
datasource db {
provider = "mysql"
url = env("DATABASE_URL")
}
model Todo {
id String @id @default(cuid())
title String
completed Boolean
}
```
Make sure to update the `DATABASE_URL` environment variable to point to a PostgreSQL compatible database. This database can be different from what you'll use when you deploy the application.
To implement password rotation lifecycles, use AWS Secrets Manager. You can rotate, manage, and retrieve database credentials, API keys, and other secrets throughout their lifecycle using Secrets Manager.
Here are the steps to set up Prisma:
1. Install the Prisma CLI as a development dependency.
2. Initialize Prisma using `npx prisma init`.
3. Update the `DATABASE_URL` environment variable to point to a PostgreSQL compatible database.
4. Create a `schema.prisma` file with your Prisma schema and schema models.
5. Create a `.env` file to define environment variables, such as your database connection.
By following these steps, you'll have a solid foundation for building a Next.js application with Prisma.
A different take: Using State in Next Js
Prisma Components
Prisma Components are a key part of making Next.js apps work seamlessly with databases.
Next.js blurs the lines between client and server, and Prisma is the perfect companion to work with a database in a Next.js app. You can access your database with Prisma at build time using getStaticProps, at request time using getServerSideProps, or by using API routes.
Prisma Accelerate is a great tool to speed up your database queries when deploying your app to a Serverless or Edge environment. Its scalable connection pool ensures that your database doesn't run out of connections, even during traffic spikes.
You can cache the results of your database queries at the Edge with Prisma Accelerate, making for faster response times while reducing the load on your database.
Here's an interesting read: Next Js Db
Static Site Generation
Static site generation is a powerful feature in Next.js that allows you to pre-render pages at build time.
You can use the getStaticProps function to send queries to your database with Prisma, making it a great combo for React apps.
Next.js will pass the props to your React components, enabling static rendering of your page with dynamic data.
This approach is commonly used for static pages, like blogs and marketing sites, where performance and flexibility are key.
A unique perspective: Next Js React Fundamentals
Todo App with Hanko Auth and Next.js
In a Todo app using Hanko Auth, Next.js 13, and Prisma, the first step is to set up the Next.js app. This involves initializing the Next.js app and understanding the project structure.
To build the user interface, you need to set up Prisma, which is a modern alternative to traditional ORMs. Prisma allows you to define your database schema using a Prisma schema file.
The Todo page is a crucial part of the Todo app, where users can view and interact with their todos. To display the right todos, you need to link them to the correct user ID. This involves updating the Todo model in the Prisma schema and the API/todo/route.tsx file to get the user ID from the token.
Here's a brief overview of the project structure:
- Initialize the Next.js app
- Set up Prisma
- Build the user interface
- App structure
- The Todo page
- Todos in the making
- API Routes in Next.js 13
- New Todo
- Update and Delete todo by ID
- Authentication with Hanko
- Hanko Cloud setup
- Adding Hanko to the Next.js app
- Verifying JWT with jose library
- Middlewares
- Securing the application and redirecting
- Time to display the right Todos
- Try it yourself
Security and Deployment
To secure your Prisma-powered Next.js application, you can add protected paths to the Middleware configuration. This involves copying code to the bottom of your middleware.tsx file.
Expand your knowledge: Nextjs Middleware App Router
You'll also want to update the Login page to subscribe to the events of the Hanko client and redirect to the Todo page after a successful login.
To deploy your application, you can use Vercel, a platform built for Next.js apps. Alternatively, you can follow the steps outlined in Example 3, which includes creating a Next.js app, setting up Prisma and seeding the DB, and configuring Amplify Hosting to retrieve the production DB connection string from AWS Parameter Store.
Here's a list of prerequisites for deploying on Amplify Hosting:
- A GitHub account to deploy the app from (with Amplify CI/CD)
- A database supported by Prisma (PostgreSQL)
- An AWS account and some familiarity with AWS Systems Manager Parameter Store
Securing and Redirecting
To prevent unauthorized users from accessing private user data, you can add protected paths to the Middleware configuration. This is done by copying the code from the Next.js Docs to the bottom of your middleware.tsx file.
Adding the middleware configuration is a crucial step in securing your application. It helps prevent unauthorized access to sensitive information.
To redirect users after a successful login, update the Login page to subscribe to the events of the Hanko client. This will allow you to redirect to the Todo page once the login is complete.
A different take: Redirects Nextjs
The middleware configuration can be updated by adding the following code to the middleware.tsx file:
```html
// middleware.tsx
import { NextMiddleware } from 'next';
import { hankoClient } from '../lib/hankoClient';
export const middleware: NextMiddleware = async ({ req, res }) => {
// Add protected paths here
const protectedPaths = ['/private-data'];
// Check if the user is trying to access a protected path
if (protectedPaths.includes(req.url)) {
// Redirect to the login page if not authenticated
if (!hankoClient.isAuthenticated()) {
res.writeHead(302, { Location: '/login' });
res.end();
}
}
};
```
This code checks if the user is trying to access a protected path and redirects them to the login page if they are not authenticated.
You might enjoy: Nextjs Middleware Firebase Auth
Deploy App on Amplify
To deploy your app on Amplify Hosting, you'll need to push the application to GitHub, as Amplify Hosting uses continuous CI/CD.
First, you'll need to set up your GitHub account to deploy the app. You'll also need a database supported by Prisma, such as PostgreSQL, and an AWS account with familiarity with AWS Systems Manager Parameter Store.
A different take: Amplify Nextjs
To deploy the app, you'll need to retrieve the production database connection string from AWS Parameter Store. This can be done in a similar way to Secrets Manager, but the pattern we'll use works with either service.
The correct permissions for your application are required for Next.js apps on Amplify, and can be found in SSR IAM Permissions. These permissions will need to be set up in the Amplify build settings.
Here are the necessary steps to deploy your app:
- Push the application to GitHub
- Retrieve the production database connection string from AWS Parameter Store
- Set up the correct permissions for your application in SSR IAM Permissions
- Configure the Amplify build settings with the necessary permissions
Testing and Deployment
To deploy your Prisma-powered Next.js app, you'll need to create a GitHub account and a database supported by Prisma, such as PostgreSQL. You'll also need an AWS account and some familiarity with AWS Systems Manager Parameter Store.
Before deploying, you'll need to push your application to GitHub and retrieve the production database connection string from AWS Parameter Store. This can be done using the `update-env.sh` utility script, which will help parse the AWS CLI response from SSM and write the value to the `.env` file.
Discover more: Next Js Software Staging Environment Aws Amplify Gen 2
You can modify the Amplify Build settings in your app to include the `update-env.sh` utility script, which will run during the build process. The build settings should include the following steps:
- Install jq as a utility to help parse the AWS CLI response from SSM
- Verify jq is installed by printing version
- Switch to Node.js 16
- Execute the `update-env.sh` utility to retrieve the database connection string from SSM and write the value to the `.env` file
- Generate the Prisma client using the connection string SSM parameter
Migrating Changes
Migrating changes is a crucial step in testing and deployment. To create tables in the database, use the prisma migrate dev command.
This command generates a new SQL migration called init, applies the migration to the database, installs Prisma Client if it's not yet installed, and generates Prisma Client based on the current schema. If Prisma Client is not automatically installed, you can install it with the command npx prisma generate.
Inside the prisma directory, you'll notice a new folder called migrations. It should contain another folder that ends with init and contains a file called migration.sql. This file contains the generated SQL.
Expand your knowledge: Next Js Folder Structure
Application Deployment
To deploy a Next.js app with Prisma, you can use Vercel, a platform built for Next.js apps. This straightforward deployment option is a great starting point.
You'll need to create a Next.js app, set up Prisma and seed the DB, and store the production DB connection string in Parameter Store (SSM). This involves creating a database supported by Prisma, such as PostgreSQL.
To follow along, you should have a GitHub account for deploying the app from, an AWS account, and familiarity with AWS Systems Manager Parameter Store.
Here are the steps to deploy the app on Amplify Hosting:
1. Push the application to GitHub.
2. Retrieve the production database connection string from AWS Parameter Store.
3. Configure the Amplify Hosting build to retrieve the connection string from Parameter Store.
4. Make sure the Backend Role has the correct permissions for your application, as listed in SSR IAM Permissions.
5. Deploy the app!
Related reading: How to Build Next Js App for Production
Testing Locally
Testing locally is a great way to ensure your app is working as expected. To do this, you can add a simple database query using Prisma to the index.js page of your app.
Replace the contents of index.js with the snippet that selects all the posts from the Post table, specifying only the columns to return. This query will help you test the data fetching locally.
Test your app with yarn dev to see the results. The app should be working after making these changes.
User Interface and API
Building the user interface and API is a crucial part of a Next.js application. To create a Todo app, you'll need to set up Prisma, a powerful ORM for databases.
You'll create API routes to handle requests and return responses. For example, you'll create a GET and POST request handler in the 'users/route.ts' file to get user data and create new users. The API will be accessed under the 'localhost:3000/api' route.
To call a GET request and retrieve all users, use a tool like curl to make a request to 'http://localhost:3000/api/users'. You can also use the Prisma client to make direct queries from your Next.js components, but this is only available for server-side components.
Curious to learn more? Check out: Next Js New Project
User Interface Building
Building the user interface is an essential part of creating a seamless user experience.
The login page is where the Hanko-auth component will play its part in handling authentication.
To display all the todos, the todo page is where it happens.
Here's a breakdown of the key user interface components:
- Login page
- Todo page
The login page is a crucial part of the user interface, as it's where users will enter their credentials to access the application.
Create API Routes
API routes are the backbone of any web application, and in this section, we'll explore how to create them using Next.js 13. To get started, we need to create a new directory called 'api' inside our app directory, which will serve as the route for all our API endpoints.
We'll create a 'GET' and 'POST' request handler in the 'users/route.ts' file, which will handle requests and return responses to the client. This is where we'll define our API endpoints and their corresponding logic.
Take a look at this: Nextjs Cookie
To test our API endpoints, we can use a tool like curl to make a GET request to 'http://localhost:3000/api/users'. This will return all the users in our database.
If we want to access our API endpoints from a Next.js application, we can use the fetch or axios HTTP client. However, if we want to access our database directly from a server-side component, we can use the Prisma client's direct query feature.
Here's a quick rundown of the Prisma client's direct query feature:
By following these steps, we can create API routes that will serve as the foundation for our web application. In the next section, we'll explore how to build the user interface for our application.
For more insights, see: Next Js Spa
Community Examples
The Prisma Next.js community is thriving, with many examples to learn from. t3 is a web development stack that includes Next.js, tRPC, Tailwind, TypeScript, Prisma, and NextAuth.
t3 is known for its extensive type-safety guarantees, making it a robust choice for building web applications. This stack is taught by Francisco Mendes in a tutorial that covers full-stack development with end-to-end type-safety.
Broaden your view: Next Js Development Company
Blitz.js is an application framework built on top of Next.js and Prisma, bringing back the simplicity of server-rendered frameworks. It preserves the best of React and client-side rendering.
CoDox is a starter template for modern web development, featuring Next.js 13, TypeScript, Tailwind CSS, Shadcn, tRPC, Clerk Auth, and more. This template saves you the initial boilerplate for your next Next.js app.
This comprehensive 4-hour tutorial teaches you how to build a full-stack form application, complete with responsiveness, drag & drop functionality, and various field types. The form will be responsive and allow for drag & drop functionality.
Intriguing read: Nextjs Form Component
Featured Images: pexels.com