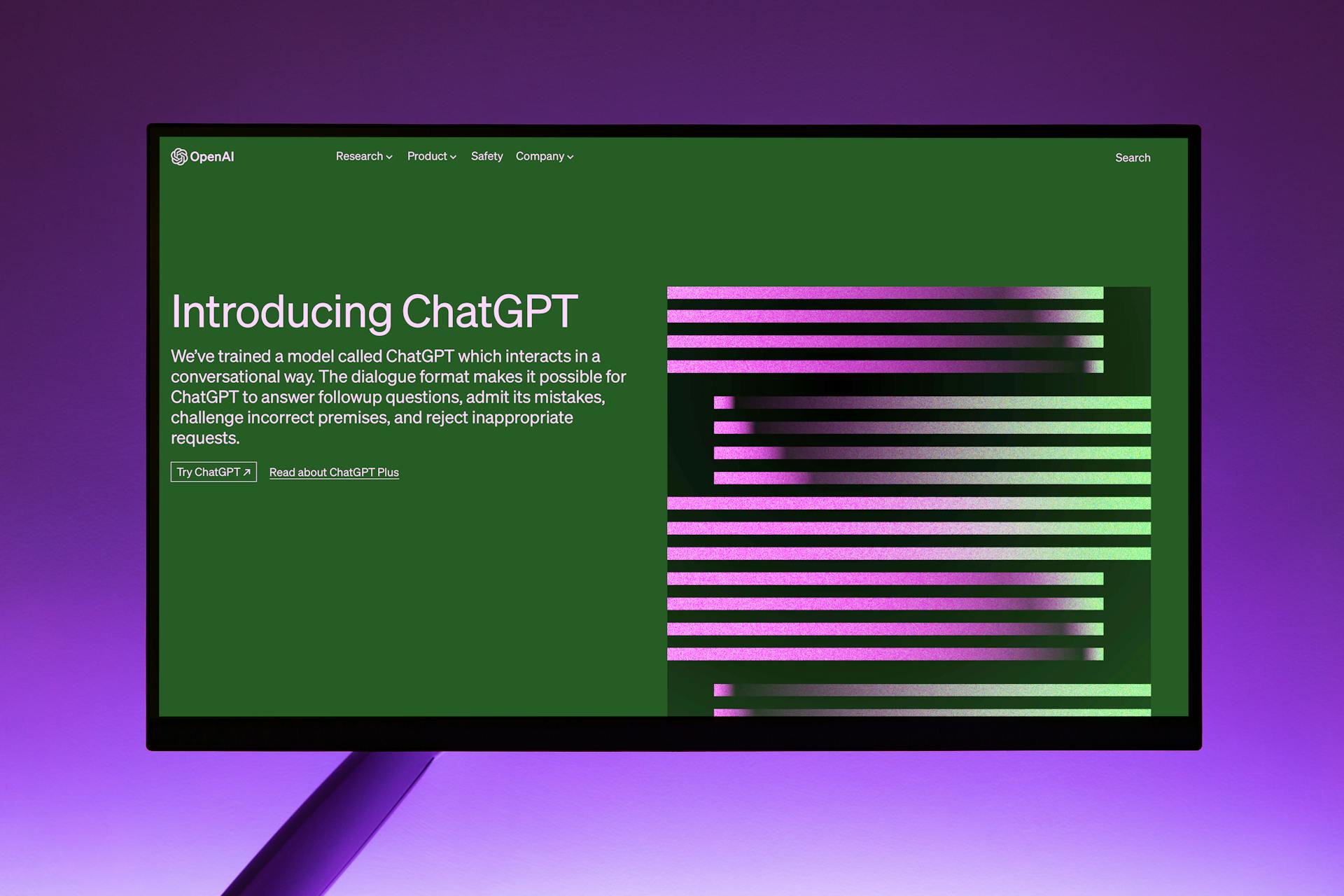
Next.js provides a flexible and powerful way to handle redirects and routing, making it easy to manage complex website structures.
You can use the `next.config.js` file to configure redirects, which is a simple and straightforward process.
Redirects can be permanent (301) or temporary (302), and you can specify a custom status code if needed.
In Next.js, routing is handled by the `getStaticProps` and `getServerSideProps` methods, which allow you to dynamically generate pages based on user input or other factors.
These methods can be used to create a seamless user experience, even for complex websites with multiple levels of navigation.
For more insights, see: Webflow Redirect
Redirect Methods
Redirects in Next.js can be performed using several methods, each with its own strengths and use cases.
You can define Next.js redirects in the next.config.js configuration file, which applies to the entirety of your application and all users accessing it. This method is best for permanent redirects on the server side, where you need to inform search engines of a changed URL.
Using middleware for Next.js redirects is another option, which is useful when performing redirects related to authentication, authorization, language, time, or location that can't be done using basic URL pattern matching.
Temporary redirects can be created using the redirect function in the Next.js App Router, which returns a 307 HTTP status code and is best for when you need to conditionally redirect based on a user action or other trigger.
Here are some of the methods available:
- next.config.js
- Middlewares
- getStaticProps/getServerSideProps for PageRoute
- redirect function for AppRouter
Each of these methods has its own use cases, such as permanent redirects, temporary redirects, and redirects based on user actions. By understanding these methods, you can choose the best approach for your Next.js application.
Route Configuration
Route configuration is a crucial part of Next.js redirects. You can define routes in the next.config.js file, which applies to the entirety of your application and all users accessing it.
Catch-all routes are a feature in Next.js that allow you to match multiple routes with a single page. They're useful for creating dynamic routes or handling multiple redirects with a single rule. You can use them to redirect users from old URLs to new ones without having to specify each redirect individually.
You might like: Nextjs Multiple Middlewares
To define routes in next.config.js, you can add them to the return value of the redirects function exported by the module. This is best for permanent redirects on the server side, where you need to inform search engines of a changed URL. For example, if you have a page that was created early in development with a misspelled URL, you can create a permanent redirect in next.config.js to redirect the old URL.
Here are some key things to keep in mind when defining routes in next.config.js:
- Use the source property to specify the requested route, the destination property to specify the route you want to redirect to, and the permanent property to control whether or not the redirect route is cached for the client machine and search engines.
- Each route should have up to 1000 elements in the array for better performance.
Rewrites in next.config.js allow you to map an incoming request path to a different destination path, making it appear like the user hasn't changed their location on the site. This can be useful for masking the destination path, and can be achieved by adding a rewrite to the next.config.js file.
Check this out: Nextjs Layouts
Defined Routes
You can define routes in Next.js using the source, destination, and permanent properties. The source property is the requested route, the destination is the route you want to redirect the user to, and permanent controls whether or not the redirect route should be cached for the client machine and search engines.
Curious to learn more? Check out: Nextjs Redirect from Server Component
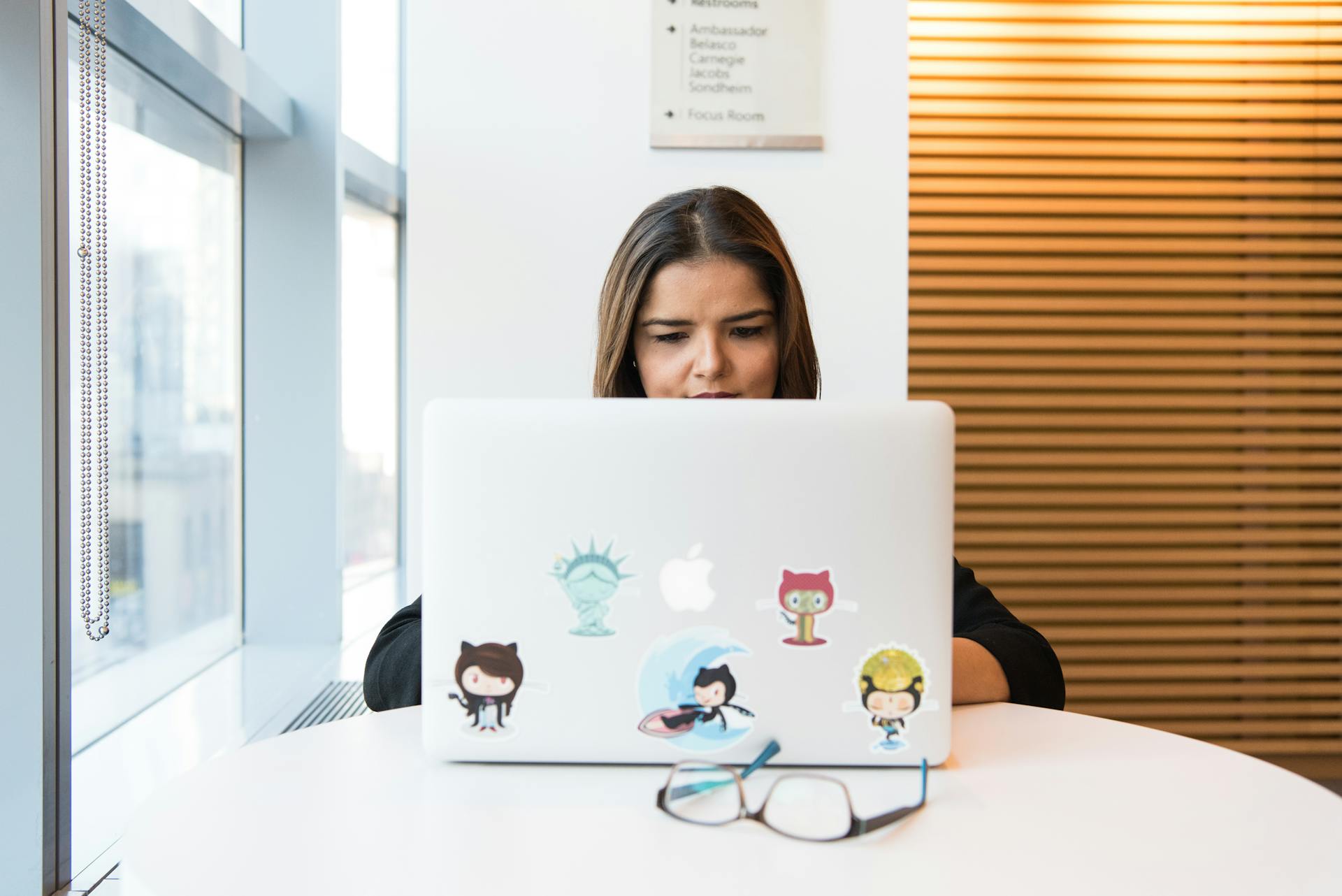
To define a route, you need to specify the source and destination properties. For example, if you want to redirect all requests to the old-blog page to the new-blog page, you can use the following code: source: "/old-blog", destination: "/new-blog", permanent: true.
This type of route is useful for permanent redirects on the server side, where you need to inform search engines of a changed URL. You can also use this type of route to redirect users from old URLs to new ones without having to specify each redirect individually.
Here's an example of a route that includes numbers: source: "/blog/123", destination: "/blog/456", permanent: true. This route will redirect all requests to the page with the URL "/blog/123" to the page with the URL "/blog/456".
Expand your knowledge: Nextjs Route
Unauthenticated Users
In full-stack development, you'll often want to restrict user access to private URLs that require authentication to be viewed. To achieve this, create a new route that will be protected.
Redirecting unauthenticated users is crucial in this scenario. You'll want to redirect them back to the login screen. This is where server-side redirects come in handy.
To implement this, create a new file in the root of the pages directory and include the necessary code. For this tutorial, a sample variable for the user was created. In a real-life scenario, you would probably use something like swr to make an API call to check if there is an active session.
Trying to access the /profile route without an active user will redirect you to the / route. This is because there is no active user available in the application.
API and Server-Side
API calls can be handled in Next.js using the built-in redirect method, which can be really handy when logging in users, submitting forms, and other use cases.
To perform a redirect, you can use the res.redirect() method in API routes, but make sure to call it before sending any response, and provide the correct syntax with the status code and destination URL.
Next.js also allows you to set redirects via its built-in pre-render methods, getStaticProps and getServerSideProps, which can be used for server-side rendering (SSR) or static site generation (SSG).
Expand your knowledge: Api Route Nextjs
Middleware
Middleware is a powerful tool in Next.js that allows you to run code before a request is completed.
You can define middleware in a file named middleware.ts (or .js) in the root of your project, at the same level as pages or app, or inside src if applicable.
Middleware can be used for site-wide redirects where multiple conditions must be checked, such as authentication and user roles.
This is useful when you need to perform redirects related to authentication, authorization, language, time, or location that can't be done using basic URL pattern matching.
By convention, use the file middleware.ts (or .js) in the root of your project to define Middleware.
GetStaticProps and GetServerSideProps
You can use getStaticProps to set redirects via Next's built-in pre-render methods, which will pre-render the page at build time. This is useful for static site generation (SSG).
To use getStaticProps, you can include the redirect rules in the index.js file, as shown in the example. The page will be automatically redirected to the specified URL.
You might like: Why Use Next Js
getStaticProps is ideal for static site generation, but if you're working with server-side rendering (SSR), you should use getServerSideProps instead. This will make sure Next.js pre-renders the page on each request.
getServerSideProps will return the data from the server when pre-rendering pages on each request. You can use this function to authenticate server-rendered pages.
You can also use getStaticProps to authenticate statically generated pages, where the user is fetched from the client side. However, if you need dynamic redirects at the user request level, use getServerSideProps.
The limitation of using getStaticProps for redirects is that the data is only fetched at build time. If you need to fetch data at runtime, use getServerSideProps instead.
Suggestion: Nextjs Rendering
User Authentication and Access Control
User Authentication and Access Control is a crucial aspect of building a secure API and server-side application. Redirects are instrumental in user authentication flows, allowing you to reroute users to a login page if they attempt to access restricted areas of your site.
You can use server-side redirects to ensure that only authenticated users can access sensitive content. This is a simple yet effective way to implement access control and prevent unauthorized access to your application.
Error Handling
Error Handling is crucial when dealing with redirects in Next.js. You don't want your application to crash unexpectedly due to an unhandled error.
Unhandled runtime errors like NEXT_REDIRECT can occur when a redirect is triggered on the client side, but the redirect is not set up to catch and manage the operation. This usually happens when redirect logic in API routes or client-side scripts is not encapsulated within try...catch blocks.
To prevent this, ensure your redirect logic is wrapped in try...catch blocks to handle any potential errors gracefully. This will help your application recover from unexpected issues.
Redirect loops can also cause NEXT_REDIRECT errors. This happens when a redirected URL leads back to the original URL, causing an infinite loop. Redirect loops can be caused by misconfigured redirect rules or logic not accounting for certain user states or request parameters.
Carefully review your redirect rules in next.config.js and dynamic redirect logic in your application to identify and resolve circular patterns.
Curious to learn more? Check out: Nextjs Url Parameter Is Not Allowed
Best Practices and Performance
To ensure your Next.js redirects are effective, follow these best practices and performance considerations.
Use permanent redirects for SEO purposes, as they pass their SEO score on to the new page, whereas temporary redirects do not.
For optimal performance, limit the use of client-side redirects as they can cause additional render time and lead to a slower perceived performance for the user.
Server-side redirects can minimize delays, making them ideal for critical redirects that need to happen before the page loads.
To handle redirects at the server level and avoid client-side delays, define redirects in your next.config.js file when using static generation.
Use a composable content platform to deliver optimized media from a high-speed CDN, which can improve the performance of your Next.js app.
Here are some tips to optimize your redirects for performance:
Testing and Security
Testing your redirects is crucial to ensure they work as intended. Regularly testing your redirects can catch any potential issues before they become problems.
Automated tests are a great way to verify that your redirect function is working correctly. Write tests to check the correct status code and destination path are returned for different scenarios.
Manually testing redirects in development and staging environments is also essential. This helps catch any potential issues before deploying to production.
You should also monitor your application's logs and analytics to identify any broken redirects or unexpected behavior. This will help you stay on top of any issues and fix them quickly.
Here's a quick checklist to help you remember the important steps:
- Write automated tests for your redirect function
- Manually test redirects in development and staging environments
- Monitor your application's logs and analytics
Troubleshooting
Troubleshooting is an essential part of testing and security in Next.js. Make sure your redirects work, because dead links can compromise your app experience.
You don't want to add redirects for pages that no longer exist, as this can confuse browsers and search engines. Instead, use custom 404 pages to let them know the page has been removed.
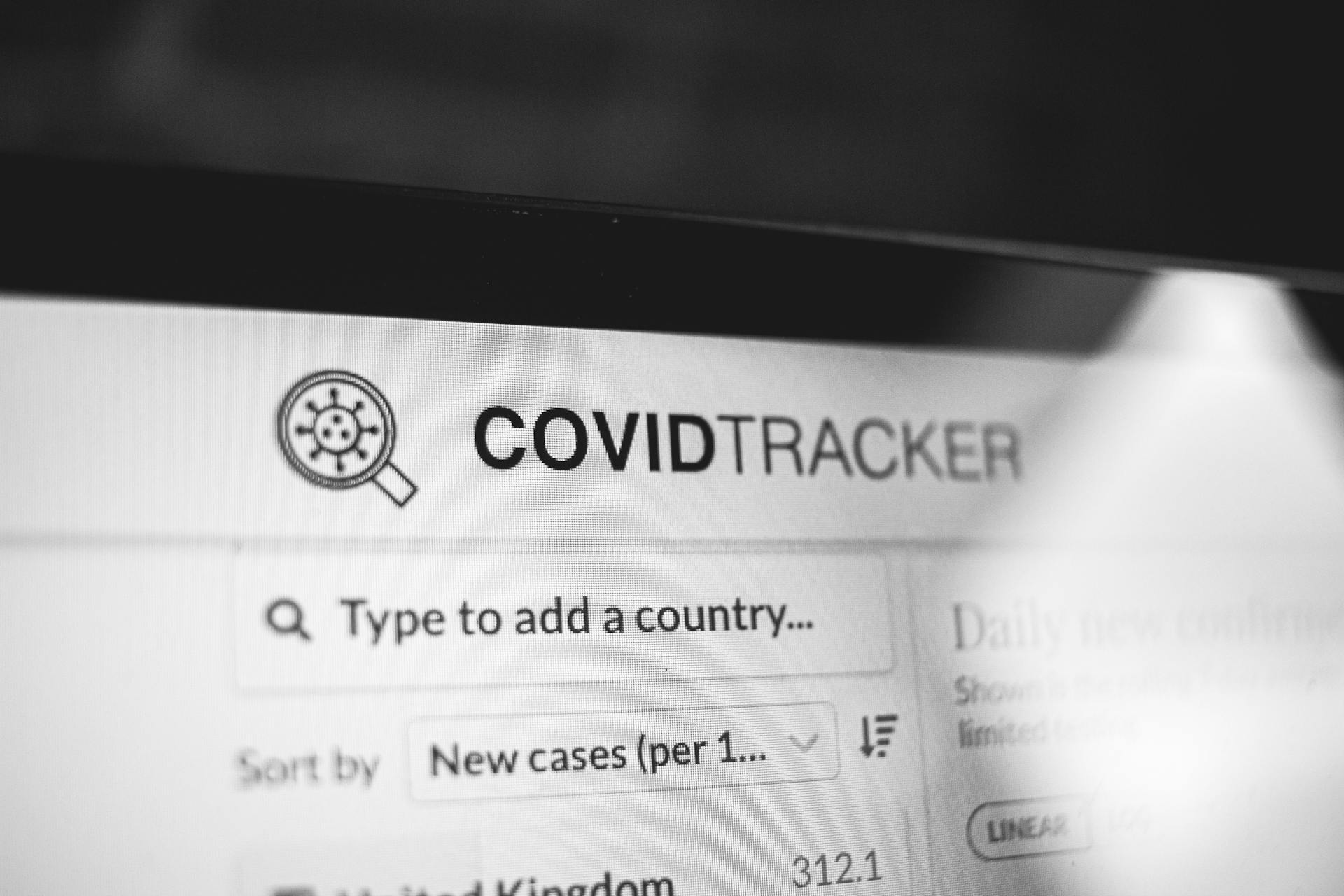
If you have a lot of redirects, consider using a redirect map stored in a database or JSON file. This will make them easier to manage and debug.
Check for conflicting paths or syntax errors, especially when pattern matching. This can cause unexpected redirect behavior.
Infinite redirect loops are a nightmare to debug, so avoid them at all costs. If you're seeing an unexpected redirect, try clearing your web browser cache or testing in an incognito window.
Here are some common errors to watch out for:
Testing Your
Testing your redirects is a crucial step in ensuring they work as intended. Regular testing helps catch any potential issues before they affect your application.
Automated tests can be written for the redirect function to verify the correct status code and destination path are returned for different scenarios. This is a key part of maintaining a smooth user experience.
Manually testing redirects in development and staging environments before deploying to production is also essential. It helps identify any problems that might arise.
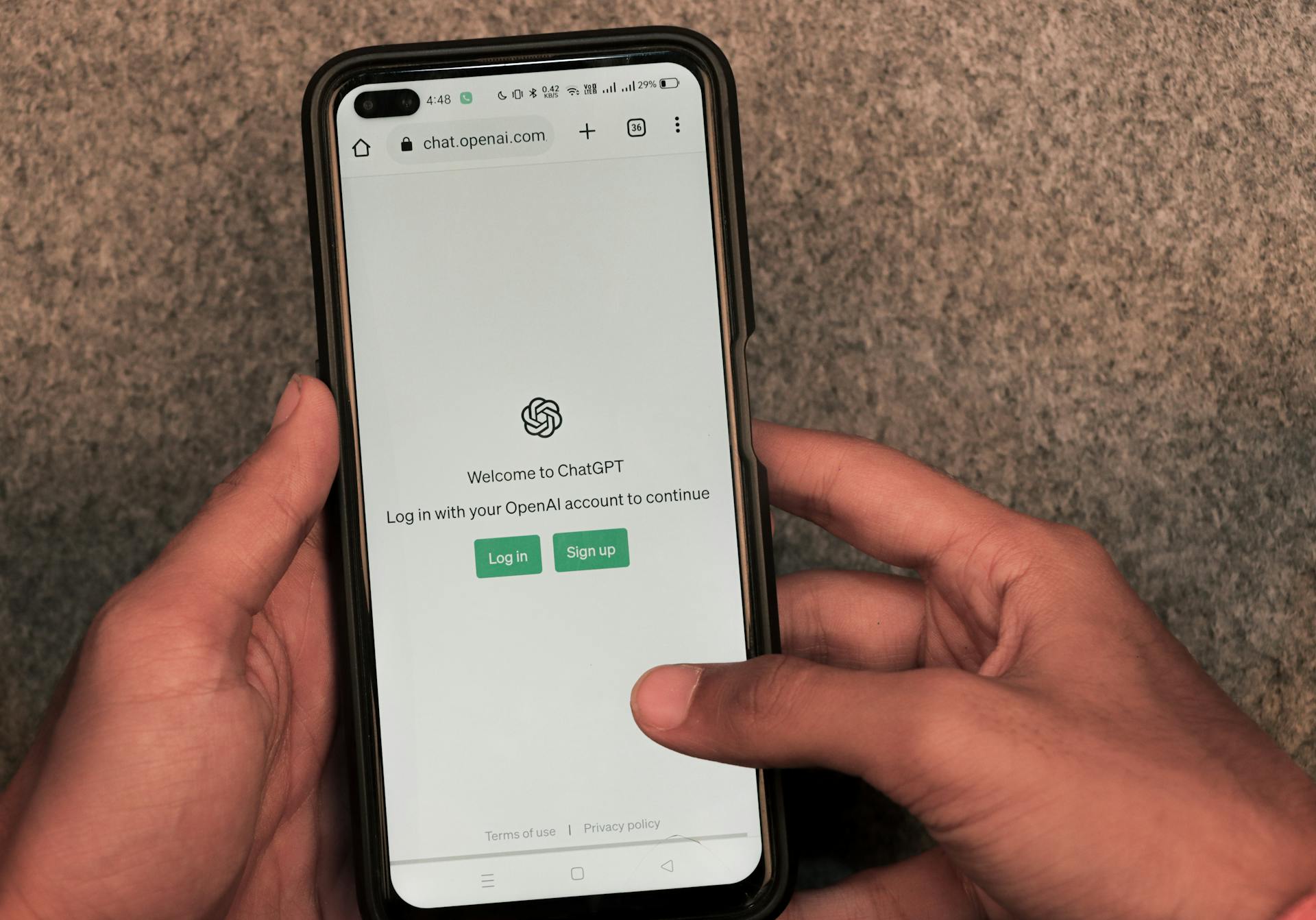
Monitoring your application's logs and analytics is another important step. It allows you to identify broken redirects or unexpected behavior, enabling you to take corrective action.
Here are some key steps to follow:
- Write automated tests for your redirect function.
- Manually test redirects in development and staging environments.
- Monitor your application's logs and analytics.
Frequently Asked Questions
How do I switch between pages in Nextjs?
To switch between pages in Next.js, you can use the Link component, the useRouter hook, the redirect function, or the native History API, each with its own approach and use case. Choose the method that best fits your development needs to navigate seamlessly between pages.
How to redirect in Next.js programmatically?
To programmatically redirect in Next.js, use the `Router.push()` method or the `useRouter()` hook, both of which allow you to navigate to a specific route when a button or event is triggered.
What is the difference between next JS 308 and 301?
Next.js 308 and 301 redirects differ in that 308 doesn't allow method changes, whereas 301 does. This means 308 is a safer choice for most permanent redirects
Featured Images: pexels.com