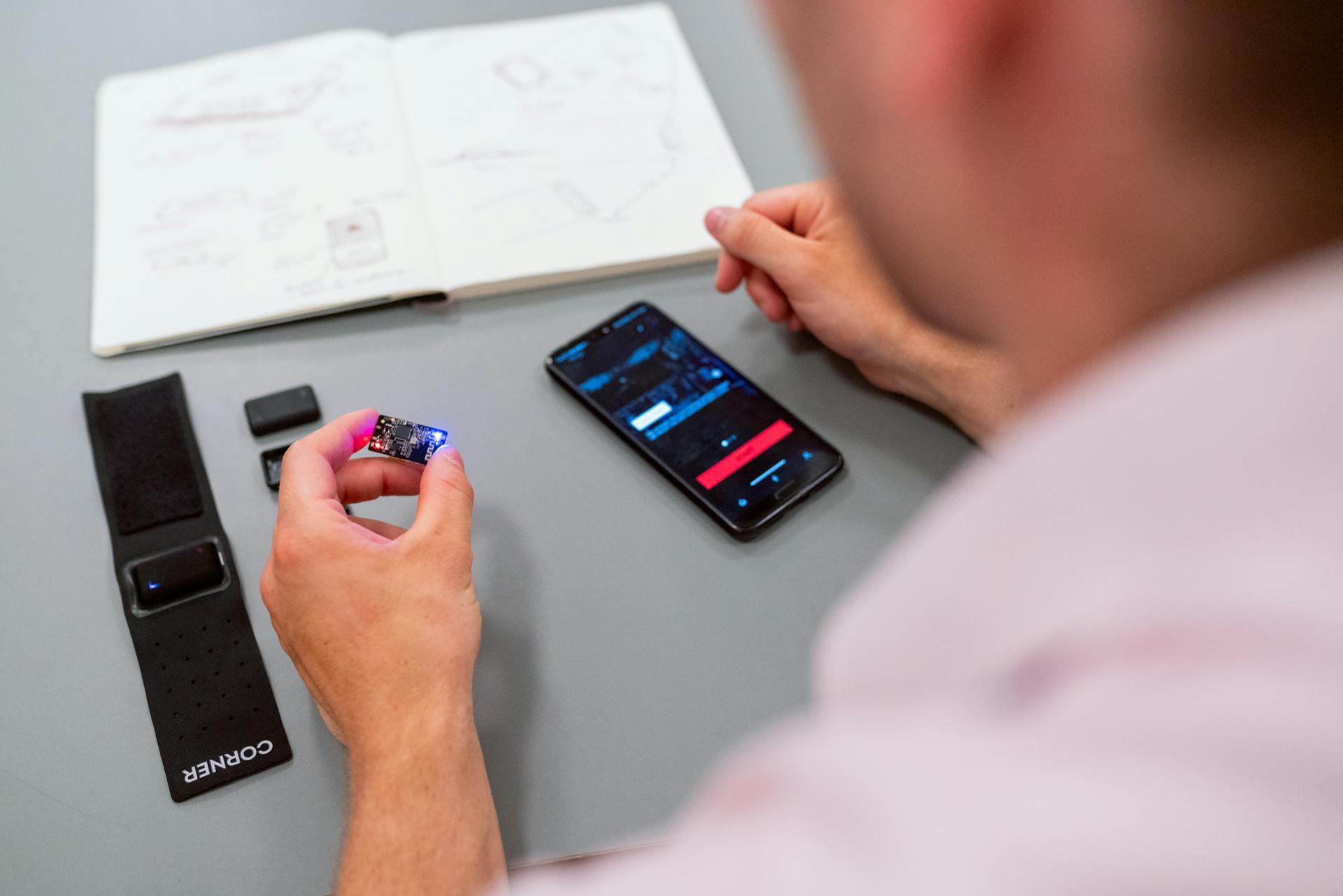
Nextjs route configuration is a crucial aspect of building robust and scalable applications. By understanding how to configure routes effectively, developers can create seamless user experiences and improve the overall performance of their application.
One of the key benefits of Nextjs is its support for dynamic routes, which allow developers to create routes that are generated at runtime. This is particularly useful for applications that require a high degree of flexibility in their routing configuration.
To get started with Nextjs route configuration, developers need to create a new page in the project directory. This can be done by running the command `npx create-next-app my-app` and then creating a new file in the `pages` directory. For example, a new file named `[id].js` can be created to handle dynamic routes.
In Nextjs, routes are defined using a combination of file names and directory structures. For instance, a file named `about.js` in the `pages` directory will be matched by the route `/about`. By creating routes in this way, developers can create a clear and intuitive structure for their application.
Take a look at this: Nextjs Intercepting Routes
Basic Project Structure
In a Next.js application, the basic project structure is crucial for supporting authentication and protected routes. The project directory should be organized in a way that makes sense for your specific needs.
The /pages directory contains your Next.js pages, including your login page, home page, and any other pages you plan to include in your app.
Each file in the /pages directory corresponds to a route in your Next.js application. For example, pages/index.js is associated with the home page.
The /pages/api directory is where you'll define your API routes that handle server-side logic, such as signing in and out, and checking the user's auth state.
You'll also need a /components directory to store your React components, including any client components that you'll protect.
If you're using a context for authentication, you'll store your AuthContext in the /context directory.
Reusable libraries and functions, such as those for managing authentication tokens or connecting to a database, can be stored in the /lib directory.
Finally, if you're using Next.js middleware for route protection, you'll define it in the /middleware directory.
Here's an example of how your project directory might look:
Authentication
Authentication is a crucial aspect of Next.js route protection.
You can utilize the Authentication Hook in the Protected Route to access the user's authentication status by calling useAuth, which determines whether the user is authenticated.
The useEffect hook is used to perform side effects, such as navigating to a new URL if the user is not authenticated.
This ensures that unauthenticated users are redirected to the login page to sign in, preventing them from viewing the content of protected routes.
The combination of a higher-order component and the authentication hook provides a powerful and reusable solution for protecting routes.
It abstracts away the complexity of checking authentication status and handling redirection, making it easier to secure specific routes and ensure that only authenticated users can access them.
By using this approach, you can easily protect routes in your Next.js application and maintain a secure authentication flow.
Explore further: Nested Routes in Next Js
Routing
Next.js file-based routing system is a powerful tool for defining common route patterns. Each route is separated based on its definition, making it easy to manage routes without complex configurations.
The initial/default route in Next.js is pages/index.js with path /. This can be configured to make your default route a sub-path of the domain. With this change, all / paths will be routed to /dashboard.
Here are the features of Next.js routing:
- File-Based Routing: Each file in the pages directory corresponds to a route in the application.
- Dynamic Routing: You can create dynamic routes using parameterized file names.
- Nested Routing: Nested routes are supported through a simple directory structure.
- Client-Side Navigation: The built-in Link component enables fast client-side navigation with prefetching capabilities.
- Custom Routes: You can define custom routes, aliases, and redirects in the next.config.js file.
- Built-In Optimization: Next.js automatically optimizes routing and navigation.
Custom Configuration with Next.js
You can define custom routes, aliases, and redirects in the next.config.js file, allowing you to create more flexible and SEO-friendly URLs. This is a powerful feature that lets you tailor your application's routing to your specific needs.
Remember to restart your server anytime you update next.config.js, as changes won't take effect without a restart. This ensures that your application reflects the latest configuration changes.
Here are some key aspects of custom configuration with Next.js:
- Custom routes: Define custom routes in the next.config.js file.
- Aliases: Create aliases for routes to make them more SEO-friendly.
- Redirects: Set up redirects to handle changes in your application's routing.
By leveraging these features, you can create a more efficient and user-friendly routing system for your application.
The API
The API in Next.js is a powerful feature that allows you to create API routes/endpoints by adding files under the pages/api directory.
A fresh viewpoint: Nextjs Proxy Api Route
Each file in this directory is mapped to an API endpoint, making it easy to manage API routes without complex configurations. This is similar to how Next.js handles client-side routes, but with a focus on server-side API endpoints.
To create an API route, simply add a file to the pages/api directory, and Next.js will automatically map it to an API endpoint. For example, if you add a file called users.js to the pages/api directory, Next.js will create an API endpoint at /api/users.
You can use the useRouter hook to manually perform routing, but for API routes, it's more common to use the getInitialProps method to get the dynamic route passed in. This allows you to access the route parameters and render content accordingly.
Here's a summary of the key points to keep in mind when working with API routes in Next.js:
- API routes are created by adding files to the pages/api directory.
- Each file in the pages/api directory is mapped to an API endpoint.
- API routes can be accessed using a URL like /api/users.
- You can use getInitialProps to get the dynamic route passed in.
Rendering
Rendering is a crucial part of the Next.js ecosystem, and it's closely tied to routing. Next.js uses a process called hydration to make each page fully interactive with minimal JavaScript code.
In Next.js, rendering is highly dependent on the form of pre-rendering, which can be either Static Generation or Server-side rendering. These two methods are highly coupled to the data fetching technique used.
Pre-rendering happens before rendering to the UI, and it's complementary to routing. Data fetching requirements determine which method to use, and built-in functions like getStaticProps, getStaticPaths, and getServerSideProps are often employed.
Next.js also supports client-side data fetching tools like SWR and react-query, as well as traditional approaches like fetch-on-render, fetch-then-render, and render-as-you-fetch with Suspense.
Recommended read: Next Js Fetch
Nested
Nested routes are a powerful feature of Next.js routing. By creating a nested folder structure, you can eliminate redundancies and make your routes more organized.
You can access files in a nested structure by visiting a URL that mirrors the folder structure. For example, if you create a new folder called users and a new file called about.js within it, you can access this file by visiting http://localhost:3000/users/about.
Related reading: Nextjs Parallel Routes
Dynamic nested routes are also supported in Next.js. With the useRouter hook, you can pull out route parameters from the query object and use them in your application.
Here are some key features of nested routes in Next.js:
- Nested routes are supported through a simple directory structure.
- Subdirectories inside the pages directory create nested routes.
- Nested routes can be accessed by visiting a URL that mirrors the folder structure.
By using nested routes, you can create a more organized and efficient routing system for your application.
Network
In the world of routing, one key aspect is how routes are defined and separated. Each route is separated based on its definition.
The Next.js file-based routing system allows for the definition of common route patterns. This is a great approach for accommodating a variety of routes.
With this system, each route is separated from the others, making it easier to manage and maintain. This separation is based on the unique characteristics of each route.
The file-based routing system is a powerful tool for handling a large number of routes. It's especially useful when working with complex routing patterns.
Sources
- https://www.dhiwise.com/post/implementing-next-js-protected-routes-a-step-by-step-guid
- https://nextjs.org/docs/app/building-your-application/routing
- https://www.smashingmagazine.com/2021/06/client-side-routing-next-js/
- https://www.geeksforgeeks.org/next-js-routing/
- https://www.freecodecamp.org/news/routing-in-nextjs-beginners-guide/
Featured Images: pexels.com