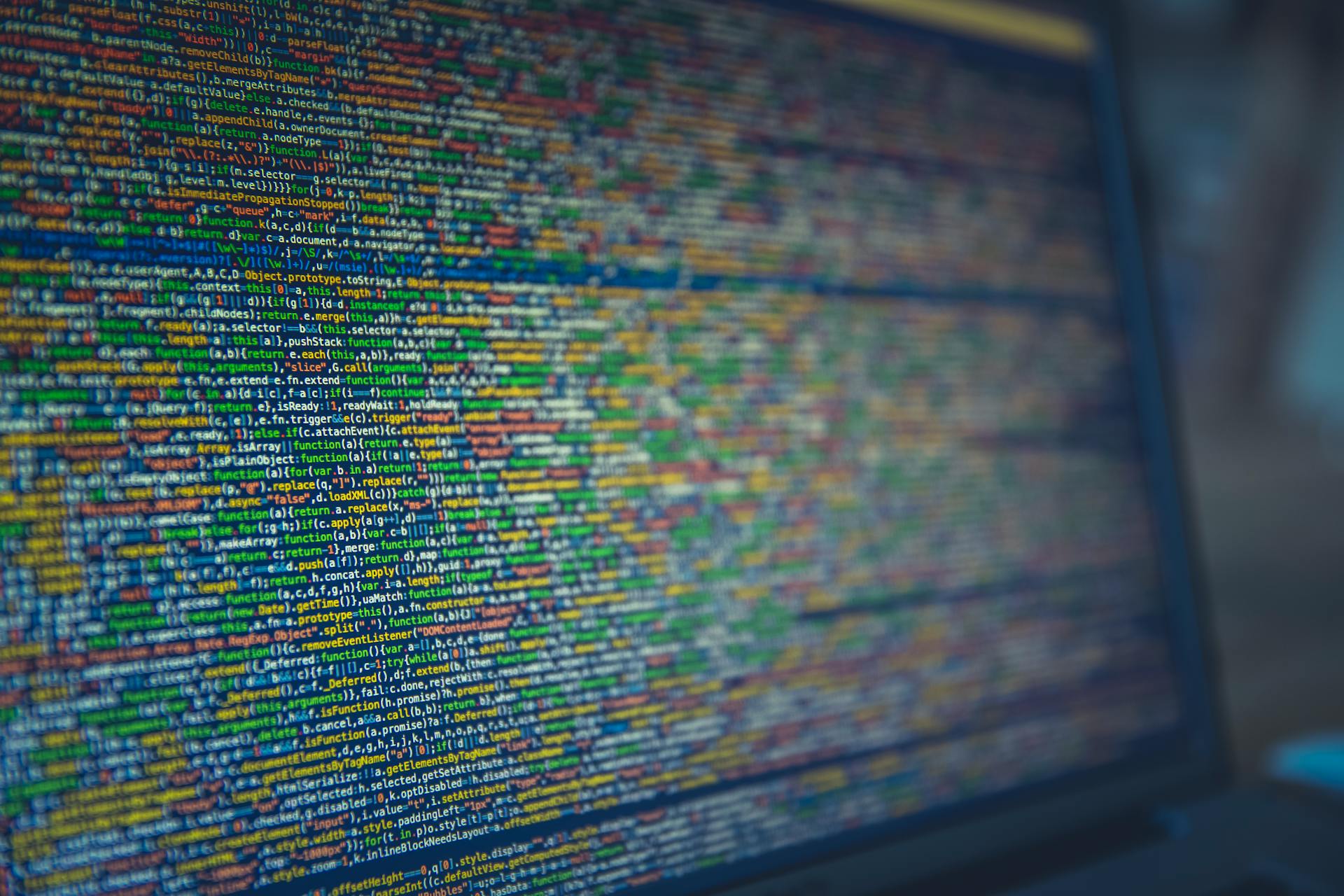
Next.js and ORMs are a match made in heaven for web development.
Next.js is a popular React-based framework for building server-rendered and statically generated websites and applications.
By combining Next.js with an Object-Relational Mapping (ORM) tool, developers can create robust and efficient data access layers for their applications.
This powerful combination enables developers to interact with databases in a more intuitive and scalable way.
Next.js provides a set of built-in features and APIs that make it easy to integrate with ORMs, including support for server-side rendering and static site generation.
This allows developers to build fast, secure, and maintainable applications that are optimized for performance.
Here's an interesting read: Next Js Api Call
Setting Up ORM
To set up an ORM (Object-Relational Mapping) tool in your Next.js project, you'll need to install Prisma, a server-side library that facilitates reading and writing data to the database in an intuitive, efficient, and safe way.
Prisma serves as the ORM tool for interacting with your CockroachDB database. You can install it by running the command: `npx prisma` (if you used npm) or `yarn prisma` (if you used yarn).
A new Prisma project will be initialized within the current directory, creating a "prisma" directory with a "schema.prisma" file and a ".env" file. The ".env" file contains the startup codes for database environment variables.
In the "schema.prisma" file, you'll create database models that will be migrated to CockroachDB. For the purpose of this article, you can copy a simple model and paste it into your "schema.prisma" file.
To update the DATABASE_URL in the ".env" file, replace the string with the connection string of your CockroachDB database. This will connect your Next.js project to your CockroachDB database.
You can change the Prisma provider from "postgresql" to "cockroachdb" in the "schema.prisma" file.
To migrate the models to the CockroachDB database, run the command: `npx prisma migrate dev --name initial`. Give your migration a name, and ensure each migration has a different name.
Once you've completed these steps, your Next.js project is now synchronized with your CockroachDB database. You can confirm this by running the command to start Prisma Studio, a dashboard-like environment where you can see your models and model objects.
For your interest: Nextjs File Upload Api
ORM Definition
An ORM, or Object-Relational Mapping, is a bridge between your application and the database.
It simplifies database interactions by allowing you to work with database records using code instead of raw queries.
This means you can focus on building application features without getting bogged down in complex queries.
Here are the benefits of using an ORM:
- Simplified database interactions: No need for complex queries.
- Improved code readability and maintainability: Work with familiar object structures.
- Portability: Easily switch between databases without major code changes.
Relational Data Queries
Drizzle provides a convenient object-oriented SQL-like querying API with Drizzle Queries, which maps select queries to an object prototype represented by a schema entity. This allows for friendlier methods to query the posts table with methods like findMany() and findFirst().
Drizzle Queries also supports relational navigation, giving easy single query access to related table data as nested objects. For example, it can fetch all categories each with their related posts.
To use relational navigation, we need to map the relations explicitly in the schema file with table relation definitions using the relations() function. This ensures that entity relations get mapped to the db.query prototype.
Here's an interesting read: Query in Nextjs
Prisma also offers a way to retrieve related data with its findMany() and findFirst() methods. However, it requires a more explicit approach, where we need to specify the related data in the query.
Here's a comparison of the two approaches:
Relational data queries can be filtered using the where clause in both Drizzle and Prisma. For example, we can retrieve users whose names contain a search query.
TypeScript Entity Types
Drizzle schemas can generate TypeScript entity types from entity table definitions. This feature is a game-changer for developers working with Next.js ORM.
With Drizzle, you can derive Zod schemas from table definitions by passing the table definition to the createInsertSchema() and createSelectSchema() functions. This allows you to create type definitions for your entities, such as posts, with ease.
The type definitions for posts are derived like this, using zod.infer<>. This approach enables you to work with your data in a more type-safe and efficient way.
For more insights, see: Best Next Js Database with Drizzle
Next.js Integration
Prisma and Next.js form an efficient and developer-friendly full-stack solution, making building full-stack applications with server-side rendering and secure database operations more reliable. This is because Prisma simplifies database management with type-safe queries, integrating seamlessly with Next.js' TypeScript-first approach.
To integrate Drizzle ORM with Next.js, you'll need to set up Drizzle ORM in a Next.js application, which involves a series of steps outlined in the article. Specifically, you'll need to install Drizzle ORM and related packages, configure Drizzle with a configuration file, and define Drizzle schemas, entity relations, and types.
Here's a brief overview of the steps involved in setting up Drizzle ORM in a Next.js application:
- Installing Drizzle ORM and related packages
- Configuring Drizzle with a configuration file
- Defining Drizzle schemas, entity relations, and types
- Creating a PostgreSQL client for Drizzle
Next.js: A Great Fit
Next.js is a popular framework for building server-rendered React applications, and one of its greatest strengths is its ability to integrate seamlessly with other tools and technologies. Next.js is a TypeScript-first approach, which means it's a great fit for developers who are already familiar with TypeScript.
Prisma is a database management tool that integrates perfectly with Next.js, simplifying database management with type-safe queries. This integration makes building full-stack applications more reliable.
Next.js allows developers to leverage its capabilities for both frontend and backend development, making it a great choice for building complex applications.
Related reading: Convert Next Js Project to Typescript
Client-Side Form Rendering
Client-Side Form Rendering is a must when working with Drizzle in Next.js.
To render forms client-side, you need to use the "use client" directive.
This is particularly important if your forms use dynamic libraries like React Hook Form and Zod.
The form data can be handled dynamically using React Hook Form and Zod inside the form component, as seen in the CreatePostForm component.
You need to import the mutation function and use it in the form handler.
For example, the createPost() function, which performs a db.insert() call with Drizzle, is imported and used in the createNewPost() handler.
Discover more: Formdata Nextjs
Move Mutation Actions to Server Side
When working with Drizzle ORM and Next.js, it's essential to move mutation actions explicitly to the server side. This is because Drizzle's db connection is not accessible from the client side.
You'll need to split your /new pages to have forms render client side, and define mutation actions server side. This is particularly important if your forms need to use dynamic libraries like React Hook Form and Zod.
Recommended read: Next Js Client Portal
By moving the db.insert() operation out into a server side action using the "use server" directive, you can make the client invoke the action and perform the operation successfully from the server side. This is a crucial step in ensuring that your forms and mutations work seamlessly together.
To achieve this, you'll need to refactor your code to explicitly move the mutation function to the server side. This might involve creating a new server side action, like the createPost() function, which performs the db.insert() operation.
Related reading: Nextjs Pathname Server Component
Database Setup
To set up a database for your Next.js project using an Object-Relational Mapping (ORM) tool, you'll need to install Prisma, a server-side library that facilitates reading and writing data to the database in an intuitive, efficient, and safe way. For this tutorial, Prisma serves as the ORM tool for interacting with our CockroachDB.
To install Prisma, run the following command: `npx prisma`. This command will create a new directory named "prisma", containing a "schema.prisma" file. Additionally, a ".env" file will be created in the root directory, containing the startup codes for database environment variables.
On a similar theme: Next Js Prisma
The "schema.prisma" file is where we create database models that will be migrated to CockroachDB. A simple model is shown below and should be copied into your schema.prisma file.
Here are the steps to update the database setup:
- Replace the "DATABASE_URL" string in the ".env" file with the connection string of your CockroachDB database.
- Change the provider from "postgresql" to "cockroachdb" in the "schema.prisma" file.
- Migrate the models to the CockroachDB database by executing the following command: `npx prisma migrate dev --name initial`.
By following these steps, you'll be able to synchronize your Next.js project with your CockroachDB database.
Frequently Asked Questions
Does Next.js have an ORM?
Yes, Next.js supports popular ORMs like Prisma and TypeORM, which offer type-safe clients for TypeScript and JavaScript applications. These ORMs provide flexibility and support for various databases, making them a great choice for Next.js projects.
Does Next.js have an ORM?
Next.js does not have a built-in ORM, but it supports various third-party ORMs that can be used with its applications. Developers can choose from popular ORMs like TypeORM, Sequelize, and Prisma to interact with their databases.
Featured Images: pexels.com